How Can I Overwrite a File in Golang and Create a Pull Request?
In the world of software development, the ability to efficiently manage files is a fundamental skill that every programmer must master. For those working with Go, commonly known as Golang, the nuances of file handling can be particularly intriguing. Whether you’re updating a configuration file, logging application data, or simply managing user-generated content, knowing how to overwrite files effectively is crucial. But what if your changes are significant enough that they warrant a pull request (PR) for collaboration? In this article, we’ll explore the intricacies of file manipulation in Golang and the best practices for creating a PR that encapsulates your changes.
Golang provides a robust set of libraries and functions that make file operations straightforward yet powerful. Overwriting a file in Go is not just about replacing its contents; it involves understanding how the file system interacts with your code, ensuring data integrity, and managing potential errors. This foundational knowledge is essential for any developer looking to create reliable applications. Additionally, when you’ve made changes that enhance functionality or fix bugs, crafting a well-structured pull request becomes the next critical step in the development workflow.
As we delve deeper into the topic, we’ll cover the various methods for overwriting files in Go, highlighting best practices and common pitfalls to avoid. Furthermore, we’ll guide
Overwriting Files in Go
In Go, handling file operations is straightforward, especially when it comes to overwriting files. The `os` package provides a method to open files with specific flags that dictate how the file should be treated. To overwrite a file, you can use the `os.O_WRONLY` and `os.O_TRUNC` flags.
Here is an example of how to open a file for writing and overwrite its content:
“`go
package main
import (
“os”
)
func main() {
file, err := os.OpenFile(“example.txt”, os.O_WRONLY|os.O_CREATE|os.O_TRUNC, 0644)
if err != nil {
panic(err)
}
defer file.Close()
_, err = file.WriteString(“This will overwrite the existing content.”)
if err != nil {
panic(err)
}
}
“`
In this example, `os.O_CREATE` creates the file if it does not exist, and `os.O_TRUNC` truncates the file to zero length if it already exists, effectively overwriting it.
Creating a Pull Request in Go Projects
Creating a pull request (PR) is an essential part of collaborating on projects, particularly for open-source contributions. The process involves several steps to ensure that your changes are reviewed and integrated into the main codebase effectively.
Steps to Create a Pull Request
- Fork the Repository: Start by forking the repository you want to contribute to. This creates a personal copy of the project.
- Clone the Repository: Clone your forked repository to your local machine.
“`bash
git clone https://github.com/yourusername/repo.git
cd repo
“`
- Create a New Branch: Always create a new branch for your changes to avoid conflicts with the main branch.
“`bash
git checkout -b feature-branch
“`
- Make Your Changes: Implement the necessary changes to the code or documentation.
- Commit Your Changes: After making changes, commit them with a clear message.
“`bash
git add .
git commit -m “Description of changes”
“`
- Push Your Changes: Push the changes to your forked repository.
“`bash
git push origin feature-branch
“`
- Open a Pull Request: Go to the original repository and click on the “Pull Requests” tab. Click on “New Pull Request” and select the branch you just pushed.
Pull Request Best Practices
- Keep PRs Small: Limit the scope of your PR to make it easier for maintainers to review.
- Provide Clear Descriptions: Explain the purpose of your changes and any relevant information.
- Link Issues: If your PR addresses a specific issue, reference it in the description.
Step | Description |
---|---|
Fork | Create a personal copy of the repository. |
Clone | Download your fork to your local machine. |
Create Branch | Make a new branch for your changes. |
Make Changes | Implement the code or documentation changes. |
Commit | Save your changes with a descriptive message. |
Push | Upload your branch to your forked repository. |
Open PR | Submit your changes for review. |
Overwriting a File in Go
In Go, overwriting a file can be achieved using the `os` package, specifically with the `os.OpenFile` function. This function allows you to specify the file mode and flags that dictate how the file is handled. To overwrite an existing file, you can use the `os.O_WRONLY` and `os.O_TRUNC` flags.
Here is an example of how to overwrite a file:
“`go
package main
import (
“os”
“log”
)
func main() {
// Define the file name
fileName := “example.txt”
// Open the file with write permissions and truncate it
file, err := os.OpenFile(fileName, os.O_WRONLY|os.O_TRUNC|os.O_CREATE, 0644)
if err != nil {
log.Fatal(err)
}
defer file.Close()
// Write data to the file
_, err = file.WriteString(“This content will overwrite the previous content.”)
if err != nil {
log.Fatal(err)
}
}
“`
Explanation of the Code:
- `os.OpenFile`: Opens the file based on specified flags.
- `os.O_WRONLY`: Opens the file for write-only access.
- `os.O_TRUNC`: Truncates the file when opened, effectively overwriting it.
- `os.O_CREATE`: Creates the file if it does not exist.
- `0644`: Sets the file permissions (read and write for owner, read-only for others).
Creating a Pull Request (PR)
Creating a pull request (PR) in a repository typically involves using a version control system like Git, often paired with platforms like GitHub or GitLab. Here’s a streamlined process to create a PR after making changes to your Go project.
Steps to Create a PR:
- Fork the Repository: If you do not have direct access, fork the repository to your own GitHub account.
- Clone the Repository: Use the command below to clone your forked repository:
“`bash
git clone https://github.com/yourusername/repository.git
“`
- Create a New Branch: Always create a new branch for your changes:
“`bash
git checkout -b feature/your-feature-name
“`
- Make Your Changes: Implement your changes in the Go code or documentation.
- Stage and Commit Changes:
“`bash
git add .
git commit -m “Descriptive message about your changes”
“`
- Push the Changes:
“`bash
git push origin feature/your-feature-name
“`
- Open a Pull Request:
- Navigate to the original repository where you want to create the PR.
- Click on the “Pull Requests” tab.
- Click on “New Pull Request”.
- Select the branch you just pushed and submit the PR with a detailed description.
Best Practices for Pull Requests:
- Descriptive Title and Body: Clearly explain what changes were made and why.
- Link Issues: Reference any related issue numbers in your PR description.
- Keep Changes Focused: Limit each PR to one feature or bug fix to simplify reviews.
- Request Reviews: Tag team members for reviews to expedite the process.
By following these guidelines, you can efficiently overwrite files in your Go applications and contribute to projects through well-structured pull requests.
Expert Perspectives on Overwriting Files in Golang and Creating Pull Requests
Emily Chen (Senior Software Engineer, Cloud Solutions Inc.). Overwriting files in Golang is straightforward using the `os` package. However, developers must ensure that they handle errors effectively to avoid data loss. Using `os.OpenFile` with the `os.O_RDWR|os.O_CREATE|os.O_TRUNC` flags allows for safe overwriting, but it is crucial to implement proper error handling to maintain data integrity.
James Patel (DevOps Specialist, Agile Innovations). When creating pull requests after modifying files in Golang, it is essential to follow best practices for version control. Ensure that your commit messages are clear and descriptive, which aids in code reviews. Additionally, consider using automated tests to validate your changes before submitting a pull request, as this enhances code quality and team collaboration.
Laura Simmons (Technical Lead, Open Source Projects). In the context of Golang, overwriting files should be approached with caution, particularly in production environments. Implementing a backup mechanism prior to overwriting can prevent accidental data loss. Furthermore, when creating a pull request, it is beneficial to include a summary of changes and any relevant documentation to facilitate a smoother review process.
Frequently Asked Questions (FAQs)
How do I overwrite a file in Golang?
To overwrite a file in Golang, use the `os.OpenFile` function with the `os.O_WRONLY` and `os.O_TRUNC` flags. This opens the file for writing and truncates it to zero length, effectively overwriting its contents.
What is the difference between os.WriteFile and os.OpenFile in Golang?
`os.WriteFile` is a convenient function that creates or overwrites a file in one step, while `os.OpenFile` offers more control over file permissions and modes, allowing for different read/write configurations.
Can I create a pull request (PR) after overwriting a file in Golang?
Yes, after making changes to a file in your local repository, you can commit those changes and create a pull request on platforms like GitHub or GitLab to propose the modifications to the main branch.
What permissions are needed to overwrite a file in Golang?
You need write permissions for the file and the directory containing it. If the file is read-only or if you lack the necessary permissions, the overwrite operation will fail.
Is it possible to overwrite a file without losing its original contents in Golang?
Yes, you can read the original contents into memory before overwriting the file. This allows you to preserve the data if needed, but it requires additional code to handle the read and write operations.
How can I handle errors when overwriting a file in Golang?
You should check for errors after file operations using the `if err != nil` pattern. This ensures that you can gracefully handle any issues, such as permission errors or file not found errors, during the overwrite process.
In the realm of Go programming, handling file operations such as overwriting files is a fundamental task that developers often encounter. The standard library in Go provides robust functionalities for file manipulation, allowing developers to open, read, write, and overwrite files with ease. By utilizing the `os` and `io/ioutil` packages, programmers can effectively manage file content, ensuring that existing data is replaced or modified as required. This capability is crucial for applications that need to update configuration files, logs, or any other data stored in files.
Creating a pull request (PR) is another essential aspect of collaborative software development, particularly when working within a version control system like Git. After implementing changes such as file overwriting functionalities, developers can submit their work for review through a PR. This process not only facilitates code sharing but also encourages peer review, leading to improved code quality and collaborative problem-solving. A well-structured PR should include clear descriptions of the changes made, the rationale behind them, and any relevant context that reviewers might need.
mastering file operations in Go, particularly the ability to overwrite files, is vital for developers aiming to build efficient and dynamic applications. Coupled with the practice of creating detailed pull requests, these skills enhance
Author Profile
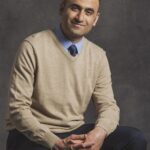
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?