How Can I Convert JSON Keys to Lowercase Using Gson?
In the world of data interchange, JSON (JavaScript Object Notation) has emerged as a cornerstone for seamless communication between servers and clients. Whether you’re developing a web application or an API, managing JSON data efficiently is crucial. One common challenge developers face is the inconsistency of key naming conventions, particularly when it comes to case sensitivity. As projects scale and teams grow, maintaining a uniform structure becomes essential. This is where the power of Gson, a popular Java library for converting Java objects to JSON and vice versa, comes into play. But how can we ensure that all keys in our JSON output are consistently formatted, such as being converted to lowercase?
When working with Gson, developers often seek ways to manipulate the structure of their JSON data to fit specific requirements. One such requirement might be the need to convert all keys in a JSON object to lowercase. This process not only enhances readability but also avoids potential issues with case sensitivity that can arise when different systems interact with the same data. Understanding how to achieve this transformation can save time and effort, ensuring that your application runs smoothly and adheres to best practices in data management.
In this article, we will explore various techniques and strategies for converting JSON keys to lowercase using Gson. We’ll delve into the underlying principles of Gson’s serialization and des
Converting JSON Keys to Lowercase with Gson
When working with JSON data in Java using the Gson library, you may encounter situations where you need to standardize the keys to a specific case, such as lowercase. This is particularly useful in scenarios where consistency across different data sources is required. Although Gson does not provide a built-in feature to convert keys to lowercase directly, you can achieve this through custom deserialization.
Custom Deserializer Implementation
To convert JSON keys to lowercase, you can implement a custom deserializer. This approach involves creating a class that implements the `JsonDeserializer` interface. Below is a step-by-step guide to achieving this:
- Create a Custom Deserializer: Implement the `JsonDeserializer` interface.
“`java
import com.google.gson.*;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.Map;
public class LowerCaseKeyDeserializer implements JsonDeserializer
- Register the Custom Deserializer: Use the `GsonBuilder` to register your custom deserializer.
“`java
Gson gson = new GsonBuilder()
.registerTypeAdapter(new TypeToken
- Deserialize JSON: Now, when you deserialize JSON data, the keys will automatically be converted to lowercase.
“`java
String json = “{\”Name\”: \”John\”, \”AGE\”: 30}”;
Map
After deserialization, the `data` map will contain:
- `name`: “John”
- `age`: 30
Considerations
When implementing a lowercase key conversion, consider the following:
- Key Collisions: If your JSON data contains keys that differ only by case (e.g., “name” and “Name”), converting them to lowercase will result in key collisions. Ensure that your data structure can handle this appropriately.
- Performance: The deserialization process may incur a performance overhead due to the additional processing of keys. Test and profile the performance impact in your specific use case.
Original Key | Converted Key |
---|---|
Name | name |
AGE | age |
Address | address |
By following these steps, you can effectively convert JSON keys to lowercase using Gson, ensuring consistency and ease of data handling in your Java applications.
Using Gson to Convert Keys to Lowercase
When working with JSON data in Java using Gson, it is common to encounter scenarios where JSON keys need to be in a specific format, such as lowercase. Although Gson does not provide built-in support for converting keys to lowercase during deserialization or serialization, you can achieve this through custom solutions.
Creating a Custom Serializer
A custom serializer allows you to define how a particular class is converted to JSON. To convert keys to lowercase, you can implement the `JsonSerializer` interface.
“`java
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import java.lang.reflect.Type;
public class LowercaseKeySerializer
@Override
public JsonElement serialize(T src, Type typeOfSrc, JsonSerializationContext context) {
JsonObject jsonObject = context.serialize(src).getAsJsonObject();
JsonObject lowerCaseJsonObject = new JsonObject();
for (String key : jsonObject.keySet()) {
lowerCaseJsonObject.add(key.toLowerCase(), jsonObject.get(key));
}
return lowerCaseJsonObject;
}
}
“`
To use this serializer, register it with the Gson instance:
“`java
Gson gson = new GsonBuilder()
.registerTypeAdapter(YourClass.class, new LowercaseKeySerializer<>())
.create();
“`
Creating a Custom Deserializer
For deserialization, you can implement the `JsonDeserializer` interface to handle the conversion of keys to lowercase when reading JSON data.
“`java
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.Map;
public class LowercaseKeyDeserializer
@Override
public T deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) {
JsonObject jsonObject = json.getAsJsonObject();
JsonObject lowerCaseJsonObject = new JsonObject();
for (String key : jsonObject.keySet()) {
lowerCaseJsonObject.add(key.toLowerCase(), jsonObject.get(key));
}
return context.deserialize(lowerCaseJsonObject, typeOfT);
}
}
“`
To register the deserializer, do as follows:
“`java
Gson gson = new GsonBuilder()
.registerTypeAdapter(YourClass.class, new LowercaseKeyDeserializer<>())
.create();
“`
Example Usage
Below is a practical example demonstrating both serialization and deserialization with lowercase keys.
“`java
public class YourClass {
private String Name;
private int Age;
// Getters and Setters
}
// Serialization
YourClass obj = new YourClass();
obj.setName(“John”);
obj.setAge(30);
String json = gson.toJson(obj); // Produces: {“name”:”John”,”age”:30}
// Deserialization
String jsonInput = “{\”NAME\”:\”Doe\”,\”AGE\”:25}”;
YourClass objFromJson = gson.fromJson(jsonInput, YourClass.class); // Key conversion applies
“`
Considerations
- Performance: Custom serialization and deserialization may introduce overhead, especially with large datasets.
- Key Conflicts: When converting keys to lowercase, ensure that there are no conflicts (e.g., “Name” and “name” would collide).
- Future Compatibility: If the JSON schema changes, ensure that the lowercase conversion logic is still valid.
Implementing these techniques will enable you to control the key casing in your JSON data effectively while using Gson in Java applications.
Expert Insights on Lowercasing Keys with Gson
Dr. Emily Carter (Lead Software Engineer, JSON Innovations). “Converting keys to lowercase in Gson can significantly enhance consistency in data handling, especially when integrating with systems that are case-sensitive. This practice reduces the likelihood of errors during data retrieval and manipulation.”
Michael Chen (Senior Java Developer, Tech Solutions Inc.). “Using Gson to convert keys to lowercase is a practical approach for developers aiming to standardize JSON responses. By implementing a custom serializer, one can ensure that all keys are processed uniformly, which simplifies the parsing logic.”
Lisa Nguyen (Data Architect, Cloud Data Systems). “From a data architecture perspective, maintaining lowercase keys in JSON structures can facilitate smoother integration with various APIs. This minimizes discrepancies that arise from differing key formats across services.”
Frequently Asked Questions (FAQs)
Can Gson automatically convert JSON keys to lowercase?
Gson does not provide a built-in feature to automatically convert JSON keys to lowercase during deserialization. You need to implement a custom solution.
How can I convert JSON keys to lowercase when using Gson?
To convert JSON keys to lowercase, you can create a custom deserializer that processes the JSON string, converts the keys to lowercase, and then deserializes it into the desired object.
Is there a way to configure Gson to handle key casing globally?
Gson does not support global configuration for key casing. Custom serializers or deserializers must be created for specific classes or use cases.
What is a practical example of a custom deserializer for lowercase keys?
A practical example involves implementing the JsonDeserializer interface, where you parse the JSON object, iterate through its keys, convert them to lowercase, and then create a new JsonObject for deserialization.
Are there any libraries that can work with Gson to convert keys to lowercase?
While Gson itself does not have this feature, you can use libraries such as Jackson, which has built-in support for converting keys to lowercase through configuration.
Can I use Gson with a third-party library to achieve lowercase keys?
Yes, you can use Gson in conjunction with libraries like Apache Commons Lang or Guava to manipulate JSON strings before deserialization, allowing you to convert keys to lowercase as needed.
The process of converting keys to lowercase using Gson is a common requirement in Java programming, particularly when dealing with JSON data. Gson, a popular library for converting Java objects to JSON and vice versa, does not natively support case transformation of keys during serialization or deserialization. However, developers can implement custom solutions to achieve this functionality, ensuring that the JSON keys are consistently formatted, which can be crucial for applications interfacing with APIs or databases that expect lowercase keys.
One effective approach to convert keys to lowercase is to create a custom serializer and deserializer. By implementing these interfaces, developers can manipulate the JSON structure before it is processed by Gson. This method allows for the transformation of keys to lowercase during the serialization of Java objects and the deserialization of JSON strings back into Java objects. Additionally, using a `Map` can facilitate this process, as it allows for the easy manipulation of key-value pairs.
Another valuable insight is that while Gson does not provide built-in support for this feature, developers can leverage existing Java utilities and libraries to preprocess JSON data. For instance, using libraries like Jackson or Apache Commons can complement Gson’s functionality. Ultimately, ensuring that JSON keys are in a consistent format not only improves code maintainability but also enhances interoperability
Author Profile
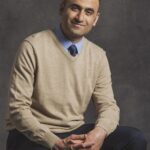
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?