How Do You Create a Hello World Program in COBOL?
In the realm of programming languages, few phrases are as iconic as “Hello, World!” This simple expression serves as a rite of passage for many coders embarking on their programming journeys. While modern languages like Python and JavaScript have popularized this tradition, the roots of “Hello, World!” can be traced back to the venerable COBOL (Common Business-Oriented Language). Developed in the late 1950s, COBOL has stood the test of time, powering critical business applications and maintaining relevance in today’s technology landscape. In this article, we will explore the significance of the “Hello, World!” program in COBOL, its syntax, and what it represents in the broader context of programming.
The “Hello, World!” program in COBOL is not just a simple output statement; it embodies the essence of learning a new programming language. For beginners, writing this program is often the first step towards understanding the structure and syntax of COBOL, a language designed with readability and business applications in mind. Unlike many contemporary languages that prioritize brevity, COBOL’s verbose nature reflects its purpose in enterprise environments, where clarity and maintainability are paramount.
As we delve deeper into the world of COBOL, we will uncover the unique characteristics that set it apart from
Hello World Program Structure in COBOL
The “Hello World” program is a simple yet essential exercise for beginners in COBOL, allowing them to understand the basic syntax and structure of the language. A typical COBOL program consists of four main divisions: Identification, Environment, Data, and Procedure. Each division serves a specific purpose in program organization.
- Identification Division: This division provides metadata about the program, including the program name.
- Environment Division: Specifies the environment in which the program runs, including the configuration of input and output devices.
- Data Division: Declares the variables and data structures that the program will use.
- Procedure Division: Contains the actual instructions or logic that the program will execute.
Sample Hello World Program
Below is a simple COBOL program that prints “Hello, World!” to the console.
“`cobol
IDENTIFICATION DIVISION.
PROGRAM-ID. HelloWorld.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-MESSAGE PIC X(20) VALUE ‘Hello, World!’.
PROCEDURE DIVISION.
BEGIN.
DISPLAY WS-MESSAGE.
STOP RUN.
“`
In this program:
- WS-MESSAGE is defined in the WORKING-STORAGE SECTION with a maximum length of 20 characters.
- The `DISPLAY` statement outputs the value of `WS-MESSAGE` to the console.
Key Components Explained
The above sample comprises several key components that are essential for understanding how COBOL operates:
Component | Description |
---|---|
IDENTIFICATION DIVISION | Declares the program name. |
PROGRAM-ID | Specifies the program’s unique identifier. |
ENVIRONMENT DIVISION | Defines the runtime environment. |
DATA DIVISION | Holds the variables used in the program. |
WORKING-STORAGE SECTION | Section where variables are declared and initialized. |
PROCEDURE DIVISION | Contains the executable statements of the program. |
DISPLAY | Command used to output data to the screen. |
STOP RUN | Terminates the program execution. |
Running the Program
To run a COBOL program, follow these general steps:
- Write the Code: Use a text editor to write the COBOL code and save it with a `.cob` or `.cbl` extension.
- Compile the Code: Use a COBOL compiler (e.g., GnuCOBOL) to compile the program. This may involve a command like `cobc -x hello_world.cob`.
- Execute the Program: Run the compiled program from the command line, typically by entering `./hello_world` in a Unix-like environment.
Common Errors and Troubleshooting
When writing and executing a COBOL program, you may encounter common errors. Here are a few along with potential solutions:
- Syntax Errors: Ensure that all statements follow COBOL’s strict syntax rules, including proper indentation and punctuation.
- Variable Declaration Issues: Confirm that all variables are declared in the DATA DIVISION before use.
- Compiler Errors: Review error messages generated by the compiler for hints on what might be wrong in the code.
By understanding these components and processes, you can effectively write and run a basic “Hello World” program in COBOL.
Understanding COBOL Syntax
COBOL (Common Business-Oriented Language) is known for its verbose syntax, which is designed to be easily readable. The structure of a COBOL program can be divided into four divisions:
- Identification Division: This section provides metadata about the program, such as its name.
- Environment Division: This division specifies the system environment in which the program will run.
- Data Division: This area defines the variables and data structures used in the program.
- Procedure Division: This is where the actual logic and instructions of the program are written.
Basic Structure of a Hello World Program in COBOL
The following code snippet illustrates a simple “Hello, World!” program in COBOL:
“`cobol
IDENTIFICATION DIVISION.
PROGRAM-ID. HelloWorld.
ENVIRONMENT DIVISION.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 HelloMessage PIC X(30) VALUE ‘Hello, World!’.
PROCEDURE DIVISION.
DISPLAY HelloMessage.
STOP RUN.
“`
Code Breakdown
To better understand the components of the above program, here’s a breakdown of each section:
Section | Description |
---|---|
IDENTIFICATION DIVISION | Defines the program’s name. |
PROGRAM-ID | Specifies the unique identifier for this program. |
ENVIRONMENT DIVISION | (Empty in this example) Indicates the environment setup, if needed. |
WORKING-STORAGE SECTION | Declares variables and data used during execution. |
01 HelloMessage | A variable defined to hold the string “Hello, World!”. |
PIC X(30) | Specifies that `HelloMessage` can hold a string of up to 30 characters. |
VALUE ‘Hello, World!’ | Initializes `HelloMessage` with the string to be displayed. |
PROCEDURE DIVISION | Contains the executable statements of the program. |
DISPLAY | Command to output the value of `HelloMessage` to the console. |
STOP RUN | Terminates the program execution. |
Compiling and Running the Program
To compile and run a COBOL program, follow these steps:
- Write the Code: Use a text editor to create a file named `HelloWorld.cob`.
- Compile the Program: Use a COBOL compiler like GnuCOBOL.
- Command: `cobc -x HelloWorld.cob`
- Execute the Program: Run the compiled output.
- Command: `./HelloWorld`
A “Hello, World!” program in COBOL serves as an excellent to the language’s structure and syntax. This foundational example illustrates key concepts such as data declaration, output commands, and the overall program structure. By understanding this simple code, one can begin to explore more complex programming concepts within COBOL.
Understanding the Significance of the Hello World Program in COBOL
Dr. Emily Carter (Senior COBOL Developer, Legacy Systems Solutions). “The ‘Hello World’ program in COBOL serves as an essential to the language, illustrating basic syntax and structure. It allows new programmers to familiarize themselves with the environment and understand how COBOL handles output operations.”
James Thompson (Professor of Computer Science, University of Technology). “In teaching COBOL, starting with a ‘Hello World’ program is crucial. It not only simplifies the learning curve but also emphasizes the importance of clear and structured coding, which is a hallmark of COBOL’s design philosophy.”
Linda Martinez (COBOL Systems Analyst, Financial Services Corp). “The ‘Hello World’ example is a rite of passage for many COBOL programmers. It encapsulates the essence of programming in COBOL, where clarity and readability are paramount, setting the stage for more complex applications.”
Frequently Asked Questions (FAQs)
What is a “Hello World” program in COBOL?
A “Hello World” program in COBOL is a simple program that outputs the text “Hello, World!” to demonstrate the basic syntax and structure of the COBOL programming language.
How do you write a “Hello World” program in COBOL?
To write a “Hello World” program in COBOL, you define the program structure with the IDENTIFICATION, ENVIRONMENT, DATA, and PROCEDURE divisions, then use the DISPLAY statement to output the text.
What are the key components of a COBOL program?
The key components of a COBOL program include the IDENTIFICATION DIVISION, ENVIRONMENT DIVISION, DATA DIVISION, and PROCEDURE DIVISION, each serving a specific purpose in program organization and functionality.
Can you provide a sample code for a “Hello World” program in COBOL?
Certainly. A simple “Hello World” program in COBOL looks like this:
“`
IDENTIFICATION DIVISION.
PROGRAM-ID. HelloWorld.
PROCEDURE DIVISION.
DISPLAY ‘Hello, World!’.
STOP RUN.
“`
What is the significance of the DISPLAY statement in COBOL?
The DISPLAY statement in COBOL is used to output text or data to the console or standard output, making it essential for user interaction and program results.
Is COBOL still relevant for modern programming?
Yes, COBOL remains relevant, particularly in legacy systems within financial institutions and government organizations, where it continues to be used for critical applications and data processing.
The “Hello World” program in COBOL serves as an introductory example for those learning the language. It demonstrates the fundamental structure and syntax of COBOL, which is known for its readability and business-oriented applications. This simple program typically includes essential components such as the identification division, environment division, data division, and procedure division, showcasing how COBOL organizes its code into distinct sections.
One of the key takeaways from implementing a “Hello World” program in COBOL is the emphasis on clarity and structure. COBOL’s design prioritizes understandable code, making it accessible for beginners and ensuring that even complex business logic can be easily followed. This characteristic is particularly beneficial in enterprise environments where maintainability and collaboration are crucial.
Furthermore, the “Hello World” example highlights COBOL’s ability to interact with various data types and its strong emphasis on data processing. As a language that has stood the test of time, COBOL remains relevant in many legacy systems, and understanding its basic constructs through simple examples reinforces foundational programming skills that can be built upon in more advanced applications.
Author Profile
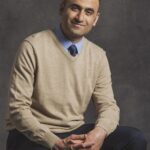
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?