How Can I Set Up a Contact Form with PHP Mailer on HostGator?
In the digital age, having a reliable method for communication is essential for any website owner. Whether you’re running a small blog, an e-commerce site, or a corporate platform, a contact form is a vital tool that facilitates interaction with your audience. If you’re using HostGator as your web hosting service, you may be wondering how to effectively implement a contact form using PHP mailer. This powerful combination not only enhances user experience but also streamlines the process of receiving inquiries, feedback, or support requests. In this article, we’ll explore the intricacies of setting up a contact form on HostGator and how PHP mailer can elevate your site’s functionality.
Creating a contact form involves more than just placing fields on a webpage; it requires a solid understanding of back-end processes to ensure that messages are sent and received without a hitch. HostGator provides a robust hosting environment that supports PHP, making it an ideal platform for implementing a custom contact form. By leveraging PHP mailer, developers can enhance the reliability and security of email communications, minimizing the risk of messages being lost or flagged as spam.
As we delve deeper into this topic, we will cover the essential steps to set up a contact form on HostGator, the benefits of using PHP mailer
Setting Up the Contact Form
Creating a contact form using PHP on a HostGator server involves several steps. First, ensure that you have a valid domain and hosting plan with HostGator. Once you have access to your hosting control panel, you can proceed with creating the contact form.
- Create the HTML Form: This is the front-end where users will submit their information.
“`html
“`
- Create the PHP Mailer Script: This script will process the form data and send an email.
“`php
“`
Configuring PHP Mailer on HostGator
Using the native `mail()` function in PHP is suitable for basic applications. However, if you’re encountering issues, consider using a more robust mailing library like PHPMailer. Here’s how to set it up on HostGator:
– **Download PHPMailer**: Obtain the PHPMailer library from GitHub or through Composer.
– **Upload PHPMailer to Your Server**: Place the library folder in your project directory.
– **Modify Your Script**: Update your PHP mailer script to use PHPMailer.
“`php
require ‘path/to/PHPMailer/src/Exception.php’;
require ‘path/to/PHPMailer/src/PHPMailer.php’;
require ‘path/to/PHPMailer/src/SMTP.php’;
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
$mail = new PHPMailer(true);
try {
$mail->isSMTP();
$mail->Host = ‘smtp.example.com’; // Specify main and backup SMTP servers
$mail->SMTPAuth = true;
$mail->Username = ‘[email protected]’; // SMTP username
$mail->Password = ‘your-password’; // SMTP password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
//Recipients
$mail->setFrom($email, $name);
$mail->addAddress(‘[email protected]’); // Add a recipient
// Content
$mail->isHTML(true);
$mail->Subject = ‘New Contact Form Submission’;
$mail->Body = nl2br(“Name: $name\nEmail: $email\nMessage: $message”);
$mail->send();
echo ‘Message has been sent’;
} catch (Exception $e) {
echo “Message could not be sent. Mailer Error: {$mail->ErrorInfo}”;
}
“`
Testing Your Contact Form
After setting up your contact form and PHP mailer, it is crucial to conduct tests to ensure everything functions as expected. Here are the steps to follow:
- Fill Out the Form: Enter test data into your contact form fields.
- Submit the Form: Click the submit button and observe any messages returned by your script.
- Check Your Email: Verify that the email has been received at the designated address.
Test Case | Expected Result | Actual Result |
---|---|---|
Valid Input | Email sent successfully | [Check result here] |
Invalid Email Format | Error message displayed | [Check result here] |
Empty Fields | Error message displayed | [Check result here] |
By following these steps, you will have a fully functional contact form on your HostGator server using PHP.
Setting Up a Contact Form with PHP Mailer on HostGator
Creating a contact form using PHP Mailer on a HostGator server is a straightforward process. PHP Mailer is a popular library that simplifies sending emails from PHP applications. This guide will walk you through the steps necessary to set up your contact form.
Requirements
Before you begin, ensure you have the following:
- A HostGator hosting account
- Basic knowledge of HTML and PHP
- PHP Mailer library (can be installed via Composer or downloaded directly)
Installing PHP Mailer
To install PHP Mailer, you can choose one of the following methods:
- Using Composer: Run the command below in your terminal:
“`bash
composer require phpmailer/phpmailer
“`
- Manual Download: Download the PHP Mailer library from the [official GitHub repository](https://github.com/PHPMailer/PHPMailer). Extract it to a directory in your project.
Creating the Contact Form
Create an HTML form that users will fill out. Here’s a simple example:
“`html
“`
Processing the Form Submission
In the `send_mail.php` file, include the PHPMailer library and write the code to handle the form submission:
“`php
isSMTP();
$mail->Host = ‘smtp.yourdomain.com’; // Set the SMTP server
$mail->SMTPAuth = true;
$mail->Username = ‘[email protected]’; // Your email
$mail->Password = ‘your-email-password’; // Your email password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
// Recipients
$mail->setFrom(‘[email protected]’, ‘Your Name’);
$mail->addAddress($email, $name); // Add the recipient
// Content
$mail->isHTML(true);
$mail->Subject = ‘New Contact Form Submission’;
$mail->Body = “You have received a new message from $name:
$message”;
$mail->send();
echo ‘Message has been sent’;
} catch (Exception $e) {
echo “Message could not be sent. Mailer Error: {$mail->ErrorInfo}”;
}
}
?>
“`
Security Measures
Implementing security measures is essential to protect your contact form. Consider the following:
- Input Validation: Validate user input to prevent injection attacks.
- CAPTCHA: Add a CAPTCHA to reduce spam submissions.
- HTTPS: Ensure your site uses HTTPS to encrypt data transmitted through your form.
Testing the Contact Form
After setting up the form and the PHP script, test the contact form by:
- Filling out the form fields.
- Submitting the form.
- Checking your email inbox for the message.
If the email does not arrive, check the following:
- SMTP settings in your PHPMailer script
- Spam folder for the email
- Server error logs for any issues
By following these steps, you can successfully create and implement a contact form using PHP Mailer on your HostGator hosting account.
Expert Insights on Host Gator Contact Form PHP Mailer
Dr. Emily Carter (Web Development Specialist, Tech Innovations Group). “Utilizing a PHP mailer for your Host Gator contact form is an effective way to ensure reliable email delivery. It allows for greater customization and control over the email sending process, which is essential for maintaining communication with your users.”
Michael Chen (Senior Software Engineer, Digital Solutions Inc.). “When implementing a PHP mailer on Host Gator, developers should pay close attention to server configurations and security settings. Properly setting up SMTP authentication can significantly reduce the chances of emails being marked as spam.”
Laura Simmons (E-commerce Consultant, WebCommerce Strategies). “A well-structured contact form using PHP mailer not only improves user experience but also enhances the overall functionality of your website. It is crucial to validate user inputs to prevent spam and ensure that you receive meaningful inquiries.”
Frequently Asked Questions (FAQs)
What is a HostGator contact form PHP mailer?
A HostGator contact form PHP mailer is a script that allows users to send form data via email using PHP programming language on a HostGator hosting account. It enables website visitors to submit inquiries or feedback through a contact form.
How do I set up a contact form with PHP mailer on HostGator?
To set up a contact form with PHP mailer on HostGator, create an HTML form, write a PHP script to process the form data, and configure the mail function to send emails. Ensure that your hosting account supports PHP and that you have the necessary permissions.
What are the common issues with PHP mailer on HostGator?
Common issues include emails being marked as spam, incorrect email addresses, or server configuration problems. Additionally, using the wrong SMTP settings can prevent emails from being sent successfully.
Can I use SMTP with the PHP mailer on HostGator?
Yes, you can use SMTP with the PHP mailer on HostGator. Configuring SMTP allows for more reliable email delivery and helps prevent emails from being flagged as spam.
Is there a limit on the number of emails I can send using PHP mailer on HostGator?
Yes, HostGator imposes limits on the number of emails sent per hour to prevent abuse. The exact limit depends on your hosting plan, so it’s advisable to check their guidelines or contact support for specifics.
What security measures should I implement for my PHP mailer?
To enhance security, implement input validation, use prepared statements to prevent SQL injection, and consider using CAPTCHA to reduce spam submissions. Additionally, ensure that the mail function is properly configured to avoid email header injection vulnerabilities.
The use of a contact form on a website is crucial for facilitating communication between users and site owners. HostGator, a popular web hosting service, supports the implementation of PHP mailer scripts to handle form submissions effectively. This allows website owners to create customized contact forms that can send messages directly to their email addresses, ensuring that inquiries are received promptly and securely. Understanding how to configure PHP mailer scripts within the HostGator environment is essential for optimizing user engagement and support.
When setting up a contact form using PHP mailer on HostGator, it is important to consider several factors, including server settings, email authentication, and potential issues with spam filters. Proper configuration of the PHP mailer script can enhance deliverability and reduce the chances of messages being marked as spam. Additionally, utilizing secure methods such as SMTP authentication can further improve the reliability of email delivery from the contact form.
In summary, implementing a contact form using PHP mailer on HostGator can significantly streamline communication for website owners. By following best practices for configuration and ensuring compliance with email standards, users can create an efficient and user-friendly contact system. This not only enhances the overall user experience but also fosters better relationships between businesses and their customers.
Author Profile
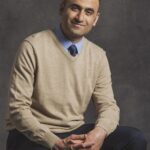
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?