How Can We Access Selectors in Actions? Exploring the Best Practices
In the ever-evolving landscape of web development, the ability to manipulate and interact with the Document Object Model (DOM) is crucial for creating dynamic, user-friendly applications. One of the key aspects of this interaction lies in understanding how to access selectors within actions. Whether you’re a seasoned developer or just starting your journey in front-end programming, mastering this skill can significantly enhance your workflow and the performance of your applications. In this article, we will delve into the intricacies of accessing selectors in actions, unraveling the techniques that empower developers to create seamless and responsive user experiences.
At its core, accessing selectors in actions involves leveraging JavaScript or frameworks like jQuery to target specific elements on a webpage. This capability allows developers to perform a variety of tasks, from simple style changes to complex event handling. Understanding the methods and best practices for accessing these selectors is essential for optimizing code efficiency and ensuring that your applications respond intuitively to user interactions.
Moreover, the integration of selectors into actions opens up a world of possibilities for dynamic content manipulation, enabling developers to create interactive features that enhance user engagement. As we explore this topic further, we will uncover the various approaches and tools available to streamline the process of accessing selectors, ensuring that you are well-equipped to tackle the challenges of modern web development
Understanding Selectors in Actions
In the realm of web development, particularly when working with frameworks like Redux or libraries that utilize a similar architecture, accessing selectors within actions can significantly enhance your application’s efficiency and organization. Selectors are functions that retrieve specific pieces of state from the store, allowing actions to operate based on the current application state.
To access selectors in actions, you can follow these strategies:
– **Direct Import of Selectors**: You can directly import the selector functions into your action creators. This method is straightforward and keeps the action logic clean and understandable.
– **Passing State as an Argument**: When dispatching actions, you can pass the relevant state as an argument. This allows the action to utilize the current state without needing to import the selectors directly.
– **Using Thunks**: If your framework supports thunk middleware, you can create asynchronous action creators that receive `dispatch` and `getState` as arguments. This approach allows you to access the current state and apply selectors within your action logic.
Here’s an example of how to structure an action creator with a selector:
“`javascript
// selectors.js
export const getUserData = (state) => state.user.data;
// actions.js
import { getUserData } from ‘./selectors’;
export const fetchUserData = () => {
return (dispatch, getState) => {
const state = getState();
const userData = getUserData(state);
// Perform actions based on userData
dispatch({
type: ‘FETCH_USER_DATA_SUCCESS’,
payload: userData,
});
};
};
“`
Best Practices for Accessing Selectors
When integrating selectors into actions, adhering to best practices can prevent common pitfalls and maintain code clarity:
- Keep Selectors Pure: Ensure that selectors do not have side effects and purely derive data from the state. This enhances testability and reusability.
- Memoization: Use memoized selectors (e.g., using libraries like Reselect) to avoid unnecessary recalculations, improving performance when accessing derived state.
- Consistency: Maintain a consistent naming convention for your selectors to make it easier to identify their purpose and usage throughout your codebase.
- Encapsulation: Keep selectors and actions closely related by encapsulating them in the same module when possible. This enhances maintainability and readability.
Here’s a table summarizing the different methods for accessing selectors in actions:
Method | Description | Pros | Cons |
---|---|---|---|
Direct Import | Import selectors into action creators | Simplicity and clarity | May lead to tight coupling |
Passing State | Pass state as an argument to actions | Decouples actions from selectors | Increases complexity |
Using Thunks | Access state within thunk actions | Flexibility and access to current state | Can lead to verbose action creators |
By understanding the various methods of accessing selectors within actions, developers can create more efficient and maintainable applications. This approach not only streamlines the interaction between state and actions but also enhances the overall architecture of the application.
Accessing Selectors in Actions
To access selectors within actions, particularly in frameworks like Redux or when using tools like Redux-Saga, it is essential to understand the structure and flow of your application state. Selectors provide a way to encapsulate the logic for retrieving slices of state, which can be particularly useful in action creators and middleware.
Using Selectors in Redux Actions
In Redux, actions can be created using action creators, which can be synchronous or asynchronous. To access selectors within these actions, you typically pass the `getState` function as a parameter.
– **Synchronous Actions**: These actions can directly call selectors to retrieve data from the state.
“`javascript
const myActionCreator = () => (dispatch, getState) => {
const state = getState();
const myData = mySelector(state);
dispatch({ type: ‘MY_ACTION’, payload: myData });
};
“`
– **Asynchronous Actions**: When using middleware like Redux Thunk or Redux Saga, selectors can be accessed in a similar manner.
“`javascript
const myAsyncActionCreator = () => async (dispatch, getState) => {
const state = getState();
const myData = mySelector(state);
await someAsyncFunction(myData);
dispatch({ type: ‘MY_ASYNC_ACTION’, payload: myData });
};
“`
Selectors and Redux-Saga
In Redux-Saga, accessing selectors involves using the `select` effect. This allows sagas to read the state without directly coupling them to the store.
- Example of Using `select` in a Saga:
“`javascript
import { select, call, put } from ‘redux-saga/effects’;
function* mySaga() {
const myData = yield select(mySelector);
const response = yield call(apiFunction, myData);
yield put({ type: ‘API_CALL_SUCCESS’, payload: response });
}
“`
Benefits of Using Selectors in Actions
Utilizing selectors within actions provides several advantages:
- Encapsulation: Selectors encapsulate the logic for state retrieval, making it reusable across different actions.
- Performance: Memoized selectors can improve performance by preventing unnecessary re-computations of derived state.
- Testing: Separating state access logic into selectors makes it easier to test actions independently.
Best Practices for Accessing Selectors
When implementing selectors in actions, consider the following best practices:
- Keep Selectors Pure: Ensure selectors do not have side effects and return the same output for the same input.
- Use Reselect: Utilize libraries like Reselect to create memoized selectors for better performance.
- Avoid Deep State Access: Use selectors to abstract away the structure of the state, preventing direct access to nested state properties in action creators.
Best Practice | Description |
---|---|
Keep Selectors Pure | Selectors should not modify state or have side effects. |
Use Reselect | Use memoized selectors to optimize performance. |
Avoid Deep State Access | Abstract state access to make actions cleaner and more maintainable. |
By adhering to these practices, developers can effectively leverage selectors in their action logic, enhancing both maintainability and performance of their applications.
Strategies for Accessing Selectors in Actions
Dr. Emily Carter (Software Development Lead, Tech Innovations Inc.). “To effectively access selectors in actions, it is essential to implement a structured approach that involves clearly defining the selectors in your codebase. Utilizing frameworks such as Redux or MobX can streamline this process by maintaining a centralized state, thus allowing actions to easily reference the required selectors.”
Michael Chen (Senior UI/UX Engineer, Design Dynamics). “In order to access selectors within actions, developers should consider using higher-order functions that encapsulate the logic needed to retrieve the selectors. This not only enhances code readability but also promotes reusability across different components of the application.”
Sarah Thompson (Frontend Architect, CodeCraft Solutions). “Integrating a modular architecture can significantly improve the accessibility of selectors in actions. By organizing your selectors into separate modules, you can ensure that actions have a clear and direct path to access the necessary data, thus improving performance and maintainability.”
Frequently Asked Questions (FAQs)
How can we access selectors in actions?
Selectors can be accessed in actions by importing the selector functions into the action file. This allows actions to retrieve the necessary state data before dispatching updates or performing side effects.
What is the role of selectors in Redux actions?
Selectors serve to encapsulate the logic for retrieving specific slices of the state. In Redux actions, they provide a clean way to access state data without directly referencing the state structure, promoting better maintainability.
Can selectors be used in asynchronous actions?
Yes, selectors can be utilized in asynchronous actions, such as those created with middleware like Redux Thunk. This enables you to access current state values before making API calls or dispatching further actions.
Are there any best practices for using selectors in actions?
Best practices include keeping selectors pure and memoized, using them to derive data only when necessary, and ensuring they are defined in a central location to maintain consistency across actions.
What are the benefits of accessing selectors in actions?
Accessing selectors in actions enhances code readability and maintainability, reduces duplication of state access logic, and allows for better separation of concerns between state management and business logic.
How do you test actions that use selectors?
Testing actions that use selectors involves mocking the selectors to return predefined values. This allows you to verify that the actions behave correctly based on different states without relying on the actual Redux store.
Accessing selectors in actions is a fundamental aspect of managing state and behavior in applications, particularly within frameworks like Redux or similar state management libraries. Selectors are functions that retrieve specific pieces of state from the store, allowing for a more organized and efficient way to manage data flow. When actions need to access these selectors, it typically involves using middleware or higher-order functions that facilitate the interaction between the action creators and the state.
One common approach to accessing selectors in actions is through the use of thunk middleware. This allows action creators to return a function instead of an action object. Within this function, developers can access the current state by passing the `getState` function as an argument, enabling them to call selectors directly. This pattern not only promotes better separation of concerns but also enhances the readability and maintainability of the code by keeping data retrieval logic encapsulated.
Another valuable insight is the importance of memoization in selectors, especially when they are accessed frequently within actions. Memoized selectors can improve performance by caching results and preventing unnecessary recalculations. This is particularly beneficial in large applications where state changes can occur frequently, and accessing the state efficiently becomes crucial. By leveraging memoized selectors, developers can ensure that their applications remain responsive and performant
Author Profile
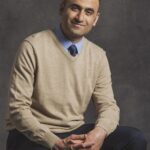
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?