How Do You Properly End a Program in Python?
In the world of programming, knowing how to gracefully end a program is just as crucial as understanding how to start one. Whether you’re debugging a complex application, managing resources efficiently, or simply ensuring that your code runs smoothly, mastering the art of program termination in Python can make all the difference. As you delve into the intricacies of Python programming, you’ll discover that there are various methods and best practices for concluding your scripts effectively, each tailored to different scenarios and needs.
Ending a program in Python isn’t just about stopping execution; it’s about doing so in a way that maintains the integrity of your application and its data. From using built-in functions to handling exceptions and ensuring that resources are released properly, there are several strategies to consider. Understanding these techniques can empower you to write cleaner, more efficient code while avoiding common pitfalls that may arise during the program’s lifecycle.
As you explore the different ways to terminate a program, you’ll also learn about the implications of each method. Some approaches may lead to abrupt exits, while others allow for a more controlled shutdown, preserving the state of your application. By equipping yourself with this knowledge, you’ll be better prepared to handle any situation that arises, ensuring that your Python programs not only run well but also end well.
Methods to End a Program in Python
In Python, there are several methods available to terminate a program. The choice of method can depend on the context of the program and the desired outcome. Below are the most common ways to end a program.
Using the `exit()` Function
The `exit()` function is one of the most straightforward ways to terminate a Python program. It is part of the `sys` module, which must be imported before using it. The `exit()` function can optionally take a status code, where a non-zero value typically indicates an error.
python
import sys
def main():
print(“Program is running.”)
sys.exit() # Exits the program
main()
Using the `quit()` Function
Similar to `exit()`, the `quit()` function also terminates the program and can be used in an interactive session. It behaves the same way as `exit()` and is primarily intended for use in the Python shell.
python
def main():
print(“This program will quit now.”)
quit() # Exits the program
main()
Using the `os._exit()` Function
For a more abrupt termination, the `os._exit()` function can be used. This method immediately stops the program without cleaning up resources or calling cleanup handlers. It’s primarily used in child processes after a fork.
python
import os
def main():
print(“Exiting immediately.”)
os._exit(0) # Exits the program without cleanup
main()
Raising an Exception
Another method to end a program is by raising an exception, which can be caught and handled appropriately. If the exception is not caught, it will terminate the program.
python
def main():
print(“An error occurred.”)
raise Exception(“Ending program with an error.”) # Raises an exception
try:
main()
except Exception as e:
print(e)
Using Control Statements
Control statements such as `return` in a function or `break` in loops can effectively end parts of a program. While they do not terminate the program outright, they can control the flow to exit from loops or functions.
python
def main():
for i in range(5):
if i == 3:
print(“Breaking the loop.”)
break # Exits the loop
print(i)
main()
Comparison of Exit Methods
The following table summarizes the various methods to end a program in Python along with their characteristics:
Method | Import Required | Exit Type | Cleanup |
---|---|---|---|
exit() | Yes (sys) | Graceful | Yes |
quit() | No | Graceful | Yes |
os._exit() | Yes (os) | Immediate | No |
Raising an Exception | No | Graceful (if caught) | Yes |
Each of these methods serves a specific purpose, and understanding their differences is essential for effective program control in Python.
Methods to End a Program in Python
There are several ways to terminate a Python program, each suitable for different scenarios. Below are the most common methods:
Using the `exit()` Function
The `exit()` function is part of the `sys` module and is often used to terminate a program. It raises the `SystemExit` exception, which can be caught in the outermost scope.
python
import sys
sys.exit()
- Optional Exit Code: You can also provide an exit code to indicate success or failure. By convention, a code of `0` indicates success, while any non-zero value indicates an error.
python
sys.exit(0) # Successful termination
sys.exit(1) # Indicating an error
Using the `quit()` Function
Similar to `exit()`, the `quit()` function is intended for use in the interactive interpreter. It can also be used in scripts but is less common in production code.
python
quit()
- Exit Code: Like `exit()`, it can also accept an optional exit code.
Using the `raise SystemExit` Statement
You can explicitly raise the `SystemExit` exception to terminate the program. This method is functionally equivalent to calling `exit()` or `quit()`.
python
raise SystemExit
- Exit Code: You can specify an exit code with the exception.
python
raise SystemExit(1) # Indicating an error
Using `os._exit()` Function
The `os._exit()` function is a lower-level termination function that exits the program without calling cleanup handlers, flushing stdio buffers, etc. It is generally used in child processes after a `fork()`.
python
import os
os._exit(0)
- Use Case: This method is not recommended for normal program termination due to its abrupt nature.
Using Keyboard Interrupt
In a running program, you can typically stop execution by sending a keyboard interrupt, usually by pressing `Ctrl + C`. This raises a `KeyboardInterrupt` exception.
python
try:
while True:
pass # Simulating a long-running process
except KeyboardInterrupt:
print(“Program terminated by user.”)
- Handling: You can catch this exception to perform any cleanup before exiting.
Using Conditional Statements for Graceful Termination
You may want to end a program based on certain conditions, allowing for cleanup or finalization.
python
def main():
while True:
user_input = input(“Type ‘exit’ to end the program: “)
if user_input.lower() == ‘exit’:
print(“Exiting the program.”)
break
main()
- Best Practices: This approach is useful for ensuring that any necessary cleanup is performed before the program exits.
Summary of Exit Methods
Method | Description | Exit Code Support |
---|---|---|
`sys.exit()` | Raises `SystemExit` exception | Yes |
`quit()` | Similar to `exit()`, mainly for interactive use | Yes |
`raise SystemExit` | Explicitly raises `SystemExit` | Yes |
`os._exit()` | Forces program termination without cleanup | No |
Keyboard Interrupt | Stops a running program via `Ctrl + C` | No |
These methods provide flexibility in how you choose to terminate your Python programs, catering to both simple and complex requirements.
Expert Insights on Ending a Program in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovators Inc.). “To gracefully terminate a Python program, one can use the `sys.exit()` function, which allows for a clean exit and can optionally return a status code to the operating system. This method is particularly useful in larger applications where resource management is critical.”
Michael Chen (Python Developer, CodeCraft Solutions). “In addition to `sys.exit()`, utilizing exceptions to handle termination can provide a robust way to end a program, especially when dealing with unexpected conditions. Raising a `SystemExit` exception can also be an effective method to ensure that cleanup operations are performed before exiting.”
Linda Patel (Lead Instructor, Python Programming Academy). “For beginners, the simplest way to end a Python script is to let the script reach its natural conclusion, which occurs when the last line of code is executed. However, for scenarios requiring immediate termination, using `exit()` or `quit()` can also be effective, though they are best reserved for interactive sessions.”
Frequently Asked Questions (FAQs)
How do you end a program in Python?
To end a program in Python, you can use the `exit()` or `sys.exit()` functions. The `exit()` function is a built-in function, while `sys.exit()` requires importing the `sys` module. Both will terminate the program immediately.
What is the difference between exit() and sys.exit()?
The `exit()` function is designed for interactive use and is a part of the `site` module, while `sys.exit()` is intended for use in scripts and can accept an exit status code to indicate success or failure.
Can you end a Python program using a return statement?
Yes, you can use a return statement to exit a function, which will subsequently end the program if it is called in the main execution block. However, it does not terminate the entire program unless it is the main program’s entry point.
Is there a way to forcefully terminate a Python program?
Yes, you can use `os._exit()` from the `os` module to forcefully terminate a Python program. This method exits the program immediately without executing any cleanup code.
What happens if you don’t end a Python program properly?
If a Python program does not end properly, it may leave resources like files or network connections open, potentially leading to memory leaks or data corruption.
Can you end a program based on a condition in Python?
Yes, you can use conditional statements to check for specific criteria and call `exit()` or `sys.exit()` when those conditions are met, allowing for controlled termination of the program.
Ending a program in Python can be accomplished through various methods, each serving different purposes depending on the context of the program. The most straightforward way to terminate a Python script is by using the `exit()` or `sys.exit()` functions, which can be called at any point in the code to stop execution. Additionally, the `quit()` function serves a similar purpose, primarily in interactive environments. It is important to note that these functions can take an optional argument, which is an exit status code that indicates whether the program ended successfully or encountered an error.
Another common method to end a program is by allowing the program to reach its natural conclusion, where the last line of code is executed. In many cases, this is sufficient for simple scripts. However, for more complex applications, especially those that involve loops or waiting for user input, it may be necessary to implement control statements such as `break` or `return` within functions to exit early or to handle exceptions gracefully using `try` and `except` blocks.
In summary, understanding how to effectively end a Python program is crucial for developers to ensure that their applications run smoothly and terminate correctly. Utilizing functions like `exit()`, `sys.exit()`, and allowing natural program flow
Author Profile
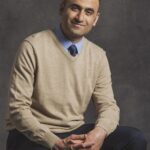
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?