How Do You Multiply Numbers in Python? A Beginner’s Guide
Introduction
Python, a versatile and powerful programming language, has become a favorite among developers and data scientists alike. One of the fundamental operations in programming is multiplication, a concept that extends beyond simple arithmetic to more complex applications in data manipulation, algorithm design, and mathematical modeling. Whether you’re a novice coder looking to grasp the basics or an experienced programmer seeking to refine your skills, understanding how to multiply in Python is essential. In this article, we’ll explore the various methods of performing multiplication in Python, showcasing its simplicity and efficiency.
Multiplication in Python can be achieved through various means, each suited to different contexts and requirements. At its core, Python allows for straightforward arithmetic operations using the asterisk (*) operator, making it intuitive for anyone familiar with basic math. However, Python’s capabilities extend far beyond simple multiplication, offering powerful libraries and functions that can handle more complex scenarios, such as multiplying matrices or performing element-wise operations on arrays.
As we delve deeper into the world of multiplication in Python, we will uncover the nuances of using built-in functions, leveraging libraries like NumPy for advanced mathematical operations, and exploring best practices to enhance your coding efficiency. Whether you’re working on a small project or a large-scale application, mastering multiplication in Python will undoubtedly elevate your programming prowess and open
Basic Multiplication
In Python, multiplication is straightforward and can be performed using the asterisk (`*`) operator. This operator can be used to multiply integers, floats, and even sequences like lists and strings, though the latter two have specific behaviors.
For example:
- Multiplying integers:
python
result = 5 * 3 # result is 15
- Multiplying floats:
python
result = 2.5 * 4.0 # result is 10.0
- Multiplying a sequence (like a list) by an integer:
python
result = [1, 2, 3] * 2 # result is [1, 2, 3, 1, 2, 3]
- Multiplying a string by an integer:
python
result = “Hello” * 3 # result is “HelloHelloHello”
Multiplying with NumPy
For more advanced mathematical operations, especially with arrays or matrices, the NumPy library is highly recommended. NumPy provides a powerful array object and numerous functions to perform element-wise operations.
To multiply arrays using NumPy, you can utilize both the `*` operator for element-wise multiplication and the `numpy.dot()` function for dot products. Here’s how to do it:
python
import numpy as np
# Element-wise multiplication
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
result_elementwise = a * b # result is array([ 4, 10, 18])
# Dot product
result_dot = np.dot(a, b) # result is 32
Multiplication Table
The following table illustrates the results of multiplying integers from 1 to 5 with the number 3.
Number | Multiplication with 3 |
---|---|
1 | 3 |
2 | 6 |
3 | 9 |
4 | 12 |
5 | 15 |
Using Functions for Multiplication
In Python, you can also define a function to perform multiplication, allowing for additional logic or validation if necessary. Here’s an example of a simple multiplication function:
python
def multiply(x, y):
return x * y
result = multiply(7, 8) # result is 56
This approach is particularly useful when you need to encapsulate multiplication logic or extend it with additional functionality like error handling.
Python provides multiple methods to perform multiplication, catering to both basic arithmetic and more complex numerical operations, ensuring flexibility for various applications.
Multiplying Numbers in Python
In Python, multiplication can be performed using the asterisk (`*`) operator. This operator can be used with various numeric types, including integers, floats, and complex numbers.
Basic Multiplication
To multiply two numbers, simply use the `*` operator between them. Here are some examples:
python
# Multiplying integers
result_int = 5 * 3 # Output: 15
# Multiplying floats
result_float = 2.5 * 4.0 # Output: 10.0
# Multiplying a float and an integer
result_mixed = 3 * 2.5 # Output: 7.5
Multiplying Lists and Tuples
In Python, you cannot directly multiply lists or tuples using the multiplication operator. However, you can repeat a list or tuple a specified number of times:
python
# Repeating a list
repeated_list = [1, 2, 3] * 3 # Output: [1, 2, 3, 1, 2, 3, 1, 2, 3]
# Repeating a tuple
repeated_tuple = (1, 2) * 2 # Output: (1, 2, 1, 2)
Multiplying Elements in a List
To multiply each element in a list by a specific number, you can use a list comprehension or the `map()` function:
python
# Using list comprehension
numbers = [1, 2, 3, 4]
multiplied_numbers = [x * 2 for x in numbers] # Output: [2, 4, 6, 8]
# Using map function
multiplied_numbers_map = list(map(lambda x: x * 2, numbers)) # Output: [2, 4, 6, 8]
Using NumPy for Array Multiplication
For more complex mathematical operations, especially with large datasets, the NumPy library is often used. NumPy provides element-wise multiplication of arrays.
python
import numpy as np
# Creating NumPy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Element-wise multiplication
result_array = array1 * array2 # Output: array([ 4, 10, 18])
Multiplying Matrices
Matrix multiplication can be performed using the `@` operator or the `dot()` method in NumPy:
python
# Matrix multiplication using @ operator
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
result_matrix = matrix1 @ matrix2 # Output: array([[19, 22], [43, 50]])
# Matrix multiplication using dot() method
result_matrix_dot = np.dot(matrix1, matrix2) # Output: array([[19, 22], [43, 50]])
Multiplying with Complex Numbers
Python natively supports complex numbers, which can also be multiplied using the `*` operator:
python
# Defining complex numbers
complex1 = 2 + 3j
complex2 = 4 + 5j
# Multiplying complex numbers
result_complex = complex1 * complex2 # Output: (-7+22j)
Summary of Multiplication Methods
Operation Type | Example | Result |
---|---|---|
Integer Multiplication | `5 * 3` | `15` |
Float Multiplication | `2.5 * 4.0` | `10.0` |
List Repetition | `[1, 2] * 2` | `[1, 2, 1, 2]` |
Element-wise List Multiply | `[x * 2 for x in [1, 2]]` | `[2, 4]` |
NumPy Array Multiply | `np.array([1, 2]) * np.array([3, 4])` | `array([3, 8])` |
Matrix Multiplication | `matrix1 @ matrix2` | `array([[19, 22], [43, 50]])` |
Complex Multiplication | `(2 + 3j) * (4 + 5j)` | `(-7 + 22j)` |
Expert Insights on Multiplication in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Multiplication in Python is straightforward, utilizing the asterisk (*) operator. This operator allows for both scalar and vector multiplication, making it versatile for various applications, including mathematical computations and data manipulation.”
Michael Chen (Data Scientist, Tech Innovations Inc.). “When performing multiplication in Python, it’s essential to understand the context. For instance, using libraries like NumPy can significantly enhance performance for large datasets, as it allows for element-wise multiplication and optimized operations.”
Sarah Patel (Educator and Python Programming Author). “For beginners, mastering the multiplication operator is crucial. However, I often emphasize the importance of understanding how Python handles data types, especially when multiplying integers and floats, to avoid unexpected results.”
Frequently Asked Questions (FAQs)
How do you multiply two numbers in Python?
You can multiply two numbers in Python using the asterisk (*) operator. For example, `result = 5 * 3` assigns the value 15 to `result`.
Can you multiply more than two numbers in Python?
Yes, you can multiply multiple numbers together by chaining the asterisk operator. For instance, `result = 2 * 3 * 4` will yield 24.
What happens if you multiply a number by zero in Python?
Multiplying any number by zero in Python results in zero. For example, `result = 7 * 0` will give you 0.
Is it possible to multiply different data types in Python?
Yes, Python allows multiplication between integers, floats, and even strings. For example, multiplying a string by an integer, like `’abc’ * 3`, results in `’abcabcabc’`.
How do you handle multiplication errors in Python?
To handle multiplication errors, you can use try-except blocks. This allows you to catch exceptions, such as TypeError, when attempting to multiply incompatible types.
Can you use libraries for advanced multiplication in Python?
Yes, libraries such as NumPy provide advanced multiplication features, including element-wise multiplication for arrays. For example, `numpy.multiply(array1, array2)` performs element-wise multiplication.
In Python, multiplication is a straightforward operation that can be performed using the asterisk (*) operator. This operator can be applied to various data types, including integers, floats, and even complex numbers. Additionally, Python supports multiplication of sequences, such as lists and strings, allowing for the repetition of elements or characters. The versatility of the multiplication operator makes it a fundamental aspect of Python programming, suitable for both simple calculations and more complex data manipulations.
Moreover, Python provides several built-in functions and libraries that enhance its mathematical capabilities. For instance, the NumPy library allows for element-wise multiplication of arrays, which is particularly useful in scientific computing and data analysis. Understanding how to effectively utilize these tools can significantly improve the efficiency and performance of mathematical operations in Python.
In summary, mastering multiplication in Python is essential for anyone looking to work with numerical data or perform mathematical computations. The simplicity of the multiplication operator, combined with the power of additional libraries, equips programmers with the necessary tools to handle a wide range of tasks efficiently. Embracing these capabilities will lead to more effective coding practices and better problem-solving skills in Python development.
Author Profile
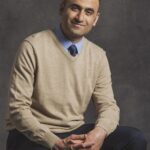
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?