How Many Bytes Are in a String? Understanding String Storage in Computing
In the digital age, where data flows seamlessly across devices and platforms, understanding the fundamental building blocks of information is essential. One of the most ubiquitous forms of data is the string—a sequence of characters that can represent anything from a simple word to complex data structures. But have you ever stopped to wonder how many bytes are actually consumed by a string? This seemingly straightforward question opens the door to a fascinating exploration of data representation, encoding standards, and the intricacies of computer memory.
When we talk about strings in programming and data management, we are not just discussing letters and symbols; we are delving into how these characters are stored and processed in a computer’s memory. The size of a string in bytes can vary significantly based on several factors, including the character encoding used and the specific content of the string itself. For instance, while a simple ASCII string may require just one byte per character, more complex encodings like UTF-8 can demand varying amounts of space depending on the characters represented.
Understanding the byte size of strings is not just an academic exercise; it has practical implications for software development, data storage, and network communication. As we navigate through the nuances of string representation, we will uncover how different programming languages handle strings, the impact of character encoding on memory usage,
Understanding String Size in Memory
When dealing with strings in programming, it is crucial to understand how they are stored in memory, particularly how many bytes they occupy. The size of a string can vary significantly based on the encoding used and the length of the string itself.
Character Encoding
The number of bytes a string occupies in memory is largely determined by its character encoding. The most common encodings include:
- ASCII: Each character is represented by 1 byte. ASCII supports 128 characters, including standard English letters, digits, and punctuation.
- UTF-8: This is a variable-length encoding scheme.
- Characters from the ASCII set (0-127) take 1 byte.
- Extended characters can take 2, 3, or 4 bytes.
- UTF-16: Typically uses 2 bytes for most characters, but some characters require 4 bytes.
- UTF-32: Every character is represented by 4 bytes, regardless of the character.
The choice of encoding can have a significant impact on the memory footprint of strings, especially when working with internationalization or special characters.
Calculating String Size
To calculate the size of a string in bytes, consider both its length and the encoding used. Here’s a simple formula:
- For ASCII: `Size (bytes) = Length of string`
- For UTF-8: `Size (bytes) = Count of ASCII characters + 2 * Count of 2-byte characters + 3 * Count of 3-byte characters + 4 * Count of 4-byte characters`
- For UTF-16: `Size (bytes) = 2 * Length of string` (for most characters)
- For UTF-32: `Size (bytes) = 4 * Length of string`
Here’s a practical example:
String | Encoding | Length | Size (bytes) |
---|---|---|---|
Hello | ASCII | 5 | 5 |
こんにちは | UTF-8 | 5 | 15 |
你好 | UTF-16 | 2 | 4 |
😀 | UTF-32 | 1 | 4 |
Practical Considerations
When programming, consider the implications of string size:
- Memory Usage: Larger strings consume more memory, which could impact performance, especially in memory-constrained environments.
- Data Transmission: When sending strings over networks, understanding their byte size is crucial for optimizing data transfer.
- Storage: The encoding affects how data is stored in databases or files, influencing storage requirements.
recognizing how many bytes a string occupies is essential for efficient programming and resource management.
Understanding String Storage
The number of bytes used to store a string depends on several factors, including the character encoding and the length of the string itself.
Character Encoding
Character encoding defines how characters are represented in bytes. Different encodings utilize varying amounts of bytes per character:
- ASCII:
- Uses 1 byte per character.
- Supports 128 characters (0-127), including standard English letters and control characters.
- UTF-8:
- Variable-length encoding.
- Uses 1 byte for standard ASCII characters.
- Uses 2 to 4 bytes for other characters (e.g., accented characters, emojis).
- UTF-16:
- Typically uses 2 bytes for most common characters.
- May use 4 bytes for less common characters (surrogate pairs).
- UTF-32:
- Fixed length of 4 bytes per character.
- Supports all Unicode characters.
Calculating Bytes in a String
To determine the total number of bytes in a string, consider both its length and the character encoding:
- Formula:
- Total Bytes = Length of String × Bytes per Character
For different encodings, this results in varying byte sizes.
Examples
Encoding | Sample String | Length | Bytes per Character | Total Bytes |
---|---|---|---|---|
ASCII | “Hello” | 5 | 1 | 5 |
UTF-8 | “Café” (with é) | 4 | 1 (C, a, f) + 2 (é) | 6 |
UTF-16 | “Hello” | 5 | 2 | 10 |
UTF-32 | “Hello” | 5 | 4 | 20 |
Practical Considerations
When working with strings in programming, be mindful of the following:
- Memory Usage: Choose the appropriate encoding based on the expected character set to optimize memory usage.
- Interoperability: Ensure consistency in encoding when exchanging data between systems.
- Performance: Consider the impact of encoding on performance, especially in large datasets or applications with high string manipulation.
Understanding these factors will help in effectively managing strings in various applications, ensuring accurate data representation and efficient memory usage.
Understanding the Byte Size of Strings in Computing
Dr. Emily Carter (Computer Scientist, Tech Innovations Lab). “The number of bytes in a string depends on the encoding used. For instance, in UTF-8 encoding, a single character can take between 1 to 4 bytes, while in UTF-16, it typically takes 2 bytes for most characters, but can take 4 bytes for others.”
Michael Chen (Software Engineer, CodeCraft Solutions). “When calculating the byte size of a string, it is essential to consider both the character set and the specific programming language being used, as different languages may have different default encodings.”
Laura Simmons (Data Analyst, ByteWise Analytics). “In practice, developers often overlook the impact of string encoding on memory usage. Understanding how many bytes a string occupies is crucial for optimizing storage and performance in applications.”
Frequently Asked Questions (FAQs)
How many bytes does a string use in memory?
The number of bytes a string uses in memory depends on the character encoding and the length of the string. For example, in UTF-8 encoding, each character can use 1 to 4 bytes, while in UTF-16, each character typically uses 2 bytes.
What is the byte size of a string in UTF-8 encoding?
In UTF-8 encoding, a string’s byte size is calculated by summing the byte sizes of each character. ASCII characters use 1 byte, while other characters may use up to 4 bytes.
How do programming languages calculate string size?
Programming languages typically provide built-in functions to calculate string size. For instance, in Python, the `len()` function returns the number of characters, while `sys.getsizeof()` returns the total memory usage, including overhead.
Does the length of a string affect its byte size?
Yes, the length of a string directly affects its byte size. A longer string will consume more bytes, especially if it contains characters that require more bytes due to the encoding used.
Are there differences in string byte size across different languages?
Yes, different programming languages may handle strings and their byte sizes differently based on their internal representation and character encoding standards. For instance, Java uses UTF-16, while JavaScript primarily uses UTF-16 but represents strings as sequences of UTF-16 code units.
How can I determine the byte size of a string in my code?
You can determine the byte size of a string by using language-specific methods to encode the string and then measure the resulting byte array. For example, in Python, you can use `len(my_string.encode(‘utf-8’))` to get the byte size in UTF-8 encoding.
In programming and data management, the size of a string in bytes is determined by several factors, including the encoding used and the length of the string itself. Common character encodings such as ASCII, UTF-8, and UTF-16 each represent characters differently, leading to variations in byte size. For instance, ASCII uses one byte per character, while UTF-8 can use one to four bytes depending on the character, and UTF-16 typically uses two bytes for most common characters.
To calculate the byte size of a string, one must consider both the number of characters and the encoding scheme applied. For example, a string containing only standard English letters will consume fewer bytes in ASCII than in UTF-8 or UTF-16. Conversely, strings that include special characters or symbols may require more bytes in UTF-8 due to its variable-length encoding. Understanding these differences is crucial for efficient memory management and data transmission.
In summary, the number of bytes in a string is not a fixed value but varies based on encoding and content. Developers and data analysts should be aware of these factors to optimize their applications and ensure compatibility across different systems. Properly calculating the byte size of strings is essential for performance, storage considerations, and data integrity
Author Profile
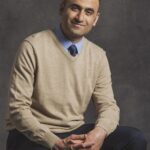
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?