How Can You Effectively Accept User Input in Python?
Introduction
In the world of programming, the ability to interact with users is a fundamental skill that can elevate your applications from static displays of information to dynamic, engaging experiences. Whether you’re developing a simple script or a complex software application, accepting user input in Python is a crucial step that allows your program to respond to real-time data and user preferences. This capability not only enhances functionality but also empowers users to personalize their interactions, making your application more versatile and user-friendly.
Understanding how to accept input from users in Python opens the door to a myriad of possibilities. At its core, user input allows your program to gather data that can influence its behavior, from basic calculations to more sophisticated decision-making processes. Python provides intuitive methods to capture this input, making it accessible even for those new to programming. As you delve into the nuances of input handling, you’ll discover various techniques that cater to different needs, ensuring your applications can adapt to diverse user scenarios.
In this article, we will explore the essential methods for accepting user input in Python, highlighting best practices and common pitfalls to avoid. By the end, you’ll have a solid understanding of how to effectively gather and utilize input, paving the way for more interactive and responsive programming experiences. Get ready to enhance your coding toolkit and bring your Python
Using the input() Function
The primary method to accept user input in Python is through the built-in `input()` function. This function prompts the user for input and captures their response as a string. Here is a basic example of its usage:
python
user_input = input(“Please enter your name: “)
print(“Hello, ” + user_input + “!”)
When the above code is executed, it displays a prompt requesting the user’s name and stores the entered value in the variable `user_input`. This value can then be used throughout the program.
Type Conversion
Since the `input()` function always returns data as a string, you may need to convert this input into other data types depending on your application’s requirements. The common types include:
- Integer: Use `int()`
- Float: Use `float()`
- Boolean: Often handled with a conditional statement
Example of converting input to an integer:
python
age = int(input(“Please enter your age: “))
print(“In five years, you will be ” + str(age + 5) + ” years old.”)
Handling Multiple Inputs
When needing to accept multiple inputs from a user, you can utilize the `split()` method to separate values. This is particularly useful for gathering data in a single line.
Example:
python
values = input(“Enter your scores separated by spaces: “).split()
scores = [int(score) for score in values]
print(“Your scores are: “, scores)
This code snippet prompts the user to enter multiple scores, splits the input into a list of strings, and converts each string to an integer.
Error Handling
To ensure your program handles unexpected input gracefully, it is advisable to implement error handling using `try` and `except` blocks. This allows your program to continue running even if the user provides invalid input.
Example:
python
try:
number = int(input(“Enter a number: “))
print(“You entered: “, number)
except ValueError:
print(“That’s not a valid number!”)
In this case, if the user inputs a non-integer value, the program will catch the error and inform the user instead of crashing.
Input Validation
When accepting user input, it’s important to validate the data to ensure it meets expected criteria. This can involve checking the length of a string, confirming that a number falls within a specific range, or using regular expressions for pattern matching.
Example of validating a username:
python
username = input(“Enter a username (3-15 characters): “)
if 3 <= len(username) <= 15:
print("Username accepted.")
else:
print("Username must be between 3 and 15 characters.")
Summary of Input Methods
The following table summarizes key methods for accepting user input in Python:
Method | Description | Example |
---|---|---|
input() | Captures user input as a string. | name = input(“Name: “) |
int(), float() | Converts input to integer or float. | age = int(input(“Age: “)) |
split() | Splits input string into a list. | values = input().split() |
try-except | Handles errors in user input. | try: number = int(input()) |
Validation | Checks if input meets criteria. | if 3 <= len(username) <= 15 |
By utilizing these methods, developers can effectively gather and manage user input in Python applications.
Using the `input()` Function
The primary method for accepting user input in Python is through the built-in `input()` function. This function reads a line from input, converts it to a string, and returns that string.
- Basic Usage:
python
user_input = input(“Please enter something: “)
- Example:
python
name = input(“What is your name? “)
print(“Hello, ” + name + “!”)
When using `input()`, it’s essential to note that all input is treated as a string. If you need to work with numerical values, type conversion is necessary.
Type Conversion
To convert the input into a different data type, such as an integer or float, you can use the `int()` or `float()` functions, respectively.
- Integer Conversion:
python
age = int(input(“Enter your age: “))
- Float Conversion:
python
height = float(input(“Enter your height in meters: “))
It is advisable to handle exceptions when converting types to avoid runtime errors if the user inputs invalid data.
- Example with Exception Handling:
python
try:
age = int(input(“Enter your age: “))
except ValueError:
print(“Please enter a valid number.”)
Accepting Multiple Inputs
To accept multiple inputs in a single line, you can use the `split()` method. This method separates the input string into a list based on spaces by default.
- Example:
python
fruits = input(“Enter your favorite fruits separated by spaces: “).split()
print(“You entered:”, fruits)
You can also customize the delimiter by passing it to the `split()` method.
- Custom Delimiter Example:
python
numbers = input(“Enter numbers separated by commas: “).split(‘,’)
numbers = [int(num) for num in numbers] # Converting to integer
print(“You entered:”, numbers)
Using Command Line Arguments
For scripts that require input directly from the command line, you can utilize the `sys` module. This approach allows for passing arguments when executing the script.
– **Example**:
python
import sys
if len(sys.argv) > 1:
print(“Arguments passed:”, sys.argv[1:])
else:
print(“No arguments were passed.”)
In this case, `sys.argv` is a list where the first element is the script name, followed by additional arguments provided.
GUI Input with Tkinter
For applications requiring a graphical user interface, Python’s `tkinter` library provides a way to accept input through dialog boxes.
- Example:
python
import tkinter as tk
from tkinter import simpledialog
root = tk.Tk()
root.withdraw() # Hide the main window
user_input = simpledialog.askstring(“Input”, “What is your name?”)
print(“Hello, ” + user_input + “!”)
This method is useful for applications that benefit from a more interactive user experience rather than a command line interface.
Best Practices
When accepting user input, consider the following best practices:
- Always validate user input to ensure it meets expected formats.
- Provide clear prompts to guide the user on what information is needed.
- Handle exceptions gracefully to improve user experience and reduce crashes.
- Use type conversion judiciously to work with the correct data types.
Expert Insights on Accepting User Input in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Accepting user input in Python is fundamental for interactive applications. Utilizing the built-in `input()` function allows developers to capture user data effectively, enabling a seamless user experience.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When accepting input from users in Python, it is crucial to validate the data to prevent errors and ensure reliability. Techniques such as try-except blocks can be employed to handle exceptions gracefully.”
Sarah Patel (Educational Technology Specialist, LearnPython.org). “For beginners, understanding how to accept input in Python through the `input()` function is essential. It serves as a gateway to creating dynamic programs that respond to user interactions.”
Frequently Asked Questions (FAQs)
How can I accept input from a user in Python?
You can use the `input()` function to accept input from a user in Python. This function prompts the user for input and returns it as a string.
What is the difference between input() and raw_input() in Python?
In Python 2, `raw_input()` is used to accept user input as a string, while `input()` evaluates the input as a Python expression. In Python 3, `raw_input()` has been removed, and `input()` behaves like `raw_input()` from Python 2.
Can I specify a prompt message when using input()?
Yes, you can specify a prompt message by passing it as an argument to the `input()` function, like this: `input(“Enter your name: “)`.
How do I convert user input to a different data type?
You can convert user input to a different data type using type conversion functions. For example, to convert input to an integer, use `int(input(“Enter a number: “))`.
What happens if the user enters invalid input?
If the user enters invalid input that cannot be converted to the specified type, a `ValueError` will be raised. It is advisable to handle such exceptions using a try-except block.
Is it possible to accept multiple inputs in one line?
Yes, you can accept multiple inputs in one line by using the `split()` method. For example: `x, y = input(“Enter two numbers: “).split()`. This will split the input string into separate variables.
In Python, accepting input from users is primarily accomplished through the built-in `input()` function. This function allows developers to prompt users for information, which can then be processed within the program. By default, the `input()` function reads input as a string, but it can be converted to other data types as needed. This flexibility makes it a fundamental tool for interactive programming in Python.
When using the `input()` function, it is important to provide a clear and concise prompt to guide users on what information is expected. Additionally, handling user input effectively often involves implementing error checking and validation to ensure that the data received is in the correct format. This not only enhances the user experience but also improves the robustness of the application.
Overall, mastering user input in Python is essential for creating dynamic and user-friendly applications. By understanding how to utilize the `input()` function and incorporating best practices for data validation, developers can significantly improve the interactivity and reliability of their programs. This knowledge lays a solid foundation for further exploration of Python’s capabilities in building comprehensive software solutions.
Author Profile
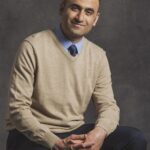
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?