How Can You Access HttpContext.Session.GetString in JavaScript?
In the dynamic world of web development, the seamless interaction between server-side and client-side technologies is crucial for creating responsive and user-friendly applications. One of the key components in this interaction is the management of session data, which allows developers to store user-specific information temporarily. With ASP.NET, accessing session data through `HttpContext.Session.GetString` is a common practice, but how do we bridge the gap to utilize that data in JavaScript? This article delves into the intricacies of accessing session data on the server side and effectively transferring it to the client side, ensuring your web applications are both efficient and interactive.
Understanding how to retrieve session data using `HttpContext.Session.GetString` is foundational for developers working with ASP.NET. This method allows you to store and retrieve string values associated with a user’s session, making it easier to maintain state across different pages. However, the challenge arises when you need to access this data in JavaScript, which operates in the browser and does not have direct access to server-side session objects.
To effectively leverage session data in JavaScript, developers must explore various techniques for passing this information from the server to the client. This could involve embedding session values directly into the HTML output, utilizing AJAX calls to fetch session data, or employing modern frameworks that
Accessing Session Data in JavaScript
To access session data stored in ASP.NET using `HttpContext.Session.GetString`, it is essential to recognize that session data is server-side and cannot be directly accessed from client-side JavaScript. However, there are methods to bridge the gap between the server-side session and client-side JavaScript.
Methods to Retrieve Session Data
There are two primary approaches to access session data in JavaScript:
- AJAX Requests: You can create an API endpoint that retrieves session data and returns it in JSON format, which can then be accessed via AJAX calls in JavaScript.
- Embedding Session Data in HTML: Another approach is to embed the session data directly into the HTML during the rendering phase. This method is suitable for small pieces of data.
Using AJAX to Fetch Session Data
To implement the AJAX method, follow these steps:
- **Create an API Endpoint**: Create a controller in your ASP.NET application that exposes session data.
csharp
[ApiController]
[Route(“api/[controller]”)]
public class SessionController : ControllerBase
{
[HttpGet(“getSessionData”)]
public IActionResult GetSessionData()
{
var sessionValue = HttpContext.Session.GetString(“YourSessionKey”);
return Ok(new { sessionValue });
}
}
- **Make an AJAX Call**: Use JavaScript (or jQuery) to fetch the session data from the created endpoint.
javascript
fetch(‘/api/session/getSessionData’)
.then(response => response.json())
.then(data => {
console.log(data.sessionValue);
// Use the session value as needed
})
.catch(error => console.error(‘Error fetching session data:’, error));
Embedding Session Data in HTML
For embedding session data directly into your HTML, you can use Razor syntax to include the session value in a `
Alternatively, you can use a data attribute in an HTML element:
Considerations
When accessing session data from JavaScript, consider the following:
- Security: Be cautious about exposing sensitive data. Ensure that only non-sensitive information is sent to the client-side.
- Data Size: Avoid sending large amounts of data as it may lead to performance issues.
Comparison of Methods
Method | Pros | Cons |
---|---|---|
AJAX Request |
|
|
Embedding in HTML |
|
|
Using these methods, you can effectively bridge the gap between server-side session data and client-side JavaScript, enabling dynamic and interactive web applications.
Accessing HttpContext.Session in JavaScript
To access values stored in `HttpContext.Session` on the server-side from JavaScript on the client-side, you need to expose the session data through a web API or a view rendering technique. Here are the primary methods to accomplish this:
Exposing Session Data via an API
- **Create a Web API Endpoint**:
- Implement a controller action that retrieves the session value and returns it as JSON.
csharp
[HttpGet]
[Route("api/session-data")]
public IActionResult GetSessionData()
{
var value = HttpContext.Session.GetString("yourKey");
return Ok(new { sessionValue = value });
}
- **Fetch Session Data in JavaScript**:
- Use the Fetch API or jQuery AJAX to call the endpoint and retrieve the session data.
javascript
fetch('/api/session-data')
.then(response => response.json())
.then(data => {
console.log(data.sessionValue); // Access your session data here
})
.catch(error => console.error('Error:', error));
Rendering Session Data in the View
If the requirement is to directly render session data within the HTML that JavaScript can access, you can embed the session value in the view.
- Use Razor Syntax:
- In your Razor view, you can access session values and render them as JavaScript variables.
- Considerations:
- Ensure that the session data does not contain sensitive information when rendering it directly in the view.
- Escape the session data if necessary to avoid JavaScript injection issues.
Security Considerations
When exposing session data to JavaScript, it is crucial to take security precautions:
- Validate User Permissions:
- Ensure that only authorized users can access the session data.
- Use HTTPS:
- Always serve your application over HTTPS to protect data in transit.
- Limit Data Exposure:
- Only expose the necessary session variables to minimize potential risks.
Alternatives to Session Storage
If the session data does not need to persist beyond a browser session, consider using alternative storage mechanisms:
- Local Storage:
- For data that needs to persist even after the browser is closed.
- Session Storage:
- For data that should only persist for the duration of the page session.
Each of these storage options can be accessed directly from JavaScript without server-side intervention, offering a more straightforward method for client-side data handling.
Accessing HttpContext.Session Data in JavaScript: Expert Insights
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). As a seasoned software architect, I emphasize the importance of server-client communication. To access `HttpContext.Session` data in JavaScript, you typically need to expose the session data through an API endpoint. This way, you can retrieve the session values using AJAX calls, ensuring a secure and efficient data transfer between the server and client.
Michael Chen (Full Stack Developer, CodeCraft Solutions). In my experience, directly accessing `HttpContext.Session` from JavaScript is not feasible due to the separation of server-side and client-side technologies. Instead, I recommend creating a dedicated endpoint that returns the session data in JSON format. This allows you to use `fetch` or `XMLHttpRequest` in JavaScript to get the session values seamlessly.
Sara Patel (Web Development Consultant, Digital Strategies Group). It is crucial to understand that session management should prioritize security. When accessing session data in JavaScript, ensure that you implement proper authentication and authorization checks in your API. This prevents unauthorized access and maintains the integrity of your application’s session data.
Frequently Asked Questions (FAQs)
How can I access session data stored in HttpContext in JavaScript?
To access session data stored in HttpContext from JavaScript, you need to expose the session data via an API endpoint or a server-side rendered page. JavaScript cannot directly access server-side session data.
What is HttpContext.Session.GetString in ASP.NET?
HttpContext.Session.GetString is a method in ASP.NET that retrieves a string value from the session state associated with the current HTTP request. It is commonly used to store user-specific data during a web session.
Can I directly read HttpContext.Session values in client-side JavaScript?
No, you cannot directly read HttpContext.Session values in client-side JavaScript. You must first send the session data to the client through an API or by rendering it within the HTML of the page.
What is the best way to pass session data to JavaScript?
The best way to pass session data to JavaScript is by using AJAX calls to a server-side endpoint that returns the session data in JSON format. Alternatively, you can embed the data in the HTML as a JavaScript variable during server-side rendering.
Is it secure to expose session data to JavaScript?
Exposing session data to JavaScript can pose security risks, especially if sensitive information is involved. Always ensure that only non-sensitive data is shared and implement proper security measures, such as authentication and authorization checks.
How do I set a session variable in ASP.NET?
To set a session variable in ASP.NET, use the following syntax: `HttpContext.Session.SetString("key", "value");`. This stores the specified value in the session state, which can later be retrieved using `HttpContext.Session.GetString("key");`.
Accessing session data stored in HttpContext.Session in a .NET application and utilizing it in JavaScript involves a few critical steps. The HttpContext.Session.GetString method allows developers to retrieve string values stored in the session state on the server side. However, since JavaScript operates on the client side, a direct call to HttpContext.Session from JavaScript is not possible. Instead, developers must pass the session data to the client side through a server-side rendering approach or an API endpoint.
One effective method to access session data in JavaScript is by embedding the session values into the HTML rendered by the server. This can be done by using Razor syntax in ASP.NET to output the session values into JavaScript variables. Alternatively, developers can create an API endpoint that retrieves the session data and returns it as a JSON object, which can then be fetched using AJAX or Fetch API in JavaScript. This approach allows for a more dynamic interaction with the session data without requiring a full page reload.
In summary, while HttpContext.Session.GetString is a powerful tool for managing session state in .NET applications, accessing this data in JavaScript requires a strategic approach. By either embedding session data directly into the rendered HTML or creating a dedicated API endpoint,
Author Profile
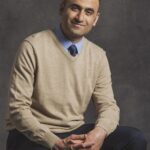
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?