How Can You Access and Retrieve Session Variables in JavaScript?
In the ever-evolving landscape of web development, the interplay between server-side and client-side technologies is crucial for creating dynamic and responsive applications. One of the key components of this interaction is the use of session variables, which allow developers to store user-specific data on the server for the duration of a session. However, accessing these session variables in JavaScript can be a bit of a puzzle, especially for those new to web programming. In this article, we’ll explore the intricacies of session variables and how to effectively retrieve and utilize them in your JavaScript code, enhancing your web applications’ functionality and user experience.
Understanding session variables is essential for managing user data across multiple pages and interactions within a web application. These variables are typically stored on the server and are designed to persist throughout a user’s session, making them ideal for tracking user preferences, login states, and other personalized information. However, JavaScript operates primarily on the client side, which raises the question: how can developers bridge this gap and access session variables effectively?
To tackle this challenge, we will delve into various methods and techniques that enable seamless communication between server-side session variables and client-side JavaScript. By leveraging tools such as AJAX, APIs, and server-side scripting languages, developers can retrieve session data and manipulate it
Accessing Session Variables in JavaScript
To access session variables in JavaScript, it is essential to understand that session variables are typically stored on the server side. However, you can pass these values to the client side (JavaScript) through various methods such as:
- Server-side Rendering: Embed session variable values directly into your HTML or JavaScript code upon rendering the page.
- AJAX Requests: Use asynchronous calls to retrieve session variable values from the server.
- Cookies: Store session variables in cookies, which can then be accessed by JavaScript on the client side.
Using Server-side Rendering
When rendering a webpage, you can directly embed session variables into the JavaScript code. For example, in PHP:
php
This method allows the session variable to be available immediately in your JavaScript code when the page loads.
Retrieving Session Variables with AJAX
AJAX provides a dynamic way to access session variables without reloading the page. Here is a simple example using jQuery:
javascript
$.ajax({
url: ‘getSessionVariable.php’,
type: ‘GET’,
success: function(data) {
var sessionValue = data;
console.log(sessionValue);
}
});
In this case, `getSessionVariable.php` should return the session variable value.
Using Cookies to Store Session Variables
Another way to access session variables in JavaScript is by utilizing cookies. You can set a cookie with the session variable value on the server side:
php
setcookie(“session_variable”, $_SESSION[‘your_session_variable’], time() + 3600, “/”);
Then, access this cookie in your JavaScript:
javascript
var sessionValue = getCookie(“session_variable”);
function getCookie(name) {
var value = “; ” + document.cookie;
var parts = value.split(“; ” + name + “=”);
if (parts.length == 2) return parts.pop().split(“;”).shift();
}
Comparison of Methods
The following table summarizes the advantages and disadvantages of each method for accessing session variables in JavaScript:
Method | Advantages | Disadvantages |
---|---|---|
Server-side Rendering | Immediate access, easy implementation | Can expose sensitive data |
AJAX Requests | Dynamic, no page reloads | Requires additional server-side code |
Cookies | Persistent across sessions, accessible from client | Limited size, potential security risks |
By carefully selecting the method that best fits your application’s needs, you can effectively manage session variables and enhance user interaction on your web pages.
Accessing Session Variables in JavaScript
To access session variables in JavaScript, you typically need to interact with the server-side language that manages these variables, as JavaScript runs on the client side. Session variables are not directly accessible in JavaScript unless you pass them to the client. Here are several methods to achieve this:
Using PHP to Pass Session Variables
If you are using PHP on the server side, you can easily pass session variables to JavaScript by embedding them directly in a script tag. For example:
php
This method effectively injects the session variable into the JavaScript context.
Using AJAX to Retrieve Session Variables
Another approach involves using AJAX to request session variables from the server. This method is particularly useful when you want to retrieve session data without reloading the page.
- PHP Script Example:
php
$_SESSION[‘username’]));
?>
- **AJAX Call in JavaScript**:
javascript
fetch(‘path_to_your_php_script.php’)
.then(response => response.json())
.then(data => {
console.log(data.username); // Outputs the session variable
})
.catch(error => console.error(‘Error:’, error));
This approach allows you to retrieve session data dynamically.
Storing Session Variables in Local Storage
If you need to persist session data across page reloads or sessions, consider storing the session variables in the browser’s Local Storage or Session Storage:
javascript
// Storing a session variable
sessionStorage.setItem(‘username’, ‘JohnDoe’);
// Retrieving the session variable
var username = sessionStorage.getItem(‘username’);
console.log(username); // Outputs: JohnDoe
This method ensures that session data is easily accessible in JavaScript but note that it does not share the data with the server.
Using Cookies to Store Session Data
Cookies can also be utilized to store session variables, especially when you want to maintain them across different pages:
javascript
// Setting a cookie
document.cookie = “username=JohnDoe; path=/”;
// Retrieving a cookie
function getCookie(name) {
let cookieArr = document.cookie.split(“;”);
for (let i = 0; i < cookieArr.length; i++) {
let cookiePair = cookieArr[i].split("=");
if (name == cookiePair[0].trim()) {
return decodeURIComponent(cookiePair[1]);
}
}
return null;
}
var username = getCookie("username");
console.log(username); // Outputs: JohnDoe
Utilizing cookies allows session variables to be shared across different pages and browser sessions.
Summary of Methods
Method | Description |
---|---|
PHP Embedding | Inject session variable directly into JavaScript. |
AJAX | Fetch session variables dynamically from the server. |
Local Storage | Store session variables in the browser for persistence. |
Cookies | Utilize cookies for cross-page and session sharing. |
Each method has its use case, and the choice depends on the requirements of the application and the desired user experience.
Expert Insights on Accessing Session Variables in JavaScript
Dr. Emily Carter (Web Development Specialist, Tech Innovations). “Accessing session variables in JavaScript typically involves using server-side languages to pass the data to the client. For instance, PHP can store session variables that can be accessed through AJAX calls, allowing seamless integration with JavaScript.”
Michael Chen (Senior Software Engineer, Cloud Solutions Inc.). “One effective way to handle session variables is by utilizing the `sessionStorage` object in JavaScript. This allows you to store data on the client side, which can be synchronized with server-side session variables for a more dynamic user experience.”
Laura Smith (JavaScript Frameworks Expert, Frontend Masters). “To retrieve session variables in JavaScript, consider implementing a RESTful API that sends the session data as JSON. This method not only enhances security but also provides a structured way to manage session data across various components of your application.”
Frequently Asked Questions (FAQs)
How can I set session variables in PHP?
Session variables in PHP can be set using the `$_SESSION` superglobal array. Start the session with `session_start()` and then assign values like this: `$_SESSION[‘variable_name’] = ‘value’;`.
How do I access session variables in JavaScript?
JavaScript cannot directly access PHP session variables. However, you can pass session variable values to JavaScript by embedding them in the HTML output or using AJAX calls to retrieve them from a PHP script.
Can I use AJAX to get session variables in JavaScript?
Yes, you can use AJAX to retrieve session variables. Create a PHP script that outputs the session variable values, then use an AJAX call from JavaScript to fetch and use those values.
What is the difference between session variables and cookies?
Session variables are stored on the server and are temporary, lasting only for the duration of the session. Cookies are stored on the client’s browser and can persist beyond the session, based on their expiration settings.
Are session variables secure for sensitive data?
Session variables are generally secure as they are stored on the server. However, it is essential to implement proper session management practices, such as using HTTPS and regenerating session IDs, to enhance security.
How can I clear session variables in PHP?
You can clear session variables in PHP by using `unset($_SESSION[‘variable_name’]);` for specific variables or `session_destroy();` to clear all session data and terminate the session.
Accessing session variables in JavaScript typically involves a combination of server-side and client-side programming. Session variables are stored on the server and are not directly accessible from the client-side JavaScript. Instead, developers often use server-side languages such as PHP, Python, or Node.js to retrieve session variables and then pass them to the client-side code through various methods, such as embedding them in the HTML or using AJAX calls.
One common approach is to render session variables into the HTML output during the server-side processing. This can be done by embedding the variable values within script tags or data attributes in the HTML elements. Another method is to create an API endpoint that the JavaScript can call to fetch the session variable values dynamically. This allows for a more interactive experience, as the client-side code can request updated session data without needing a full page reload.
It is crucial to handle session data securely, as exposing sensitive information can lead to security vulnerabilities. Developers should ensure that any data passed to the client is sanitized and that appropriate access controls are in place. Additionally, understanding the lifecycle of session variables and how they are managed on the server is essential for effective implementation.
In summary, while JavaScript cannot directly access session variables
Author Profile
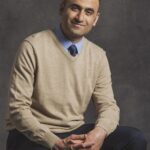
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?