How Can You Access Session Variables in JavaScript?
In the dynamic world of web development, managing user sessions effectively is crucial for creating a seamless and personalized experience. One of the key components in this process is the use of session variables, which allow developers to store and retrieve user-specific data as they navigate through a website. But how do you access these session variables using JavaScript? This article will guide you through the essential techniques and best practices for working with session variables, empowering you to enhance your web applications.
Session variables play a vital role in maintaining state across different pages of a web application. They enable developers to store information such as user preferences, authentication status, and shopping cart contents, ensuring that users have a consistent experience throughout their visit. While session variables are typically managed on the server side, JavaScript offers various methods to access and manipulate this data on the client side, making it an essential skill for modern web developers.
As we delve deeper into the topic, we will explore the different ways to interact with session variables, including the use of cookies, the sessionStorage API, and server-side techniques. By understanding how to effectively access and utilize session variables in JavaScript, you will be better equipped to create responsive and user-friendly web applications that cater to the unique needs of your audience. Get ready to unlock the potential of
Accessing Session Variables in JavaScript
To access session variables in JavaScript, it’s essential to understand how session storage works within the context of web browsers. Session storage is a type of web storage that allows you to store data for the duration of the page session. This means that data persists as long as the browser tab is open, but it is cleared once the tab is closed.
Session variables can be accessed using the `sessionStorage` object. The following methods are commonly used to interact with session variables:
- setItem(key, value): Adds a key-value pair to the session storage.
- getItem(key): Retrieves the value associated with the specified key.
- removeItem(key): Removes the specified key and its associated value.
- clear(): Clears all session storage data.
Here is an example of how to use these methods:
javascript
// Setting a session variable
sessionStorage.setItem(‘username’, ‘JohnDoe’);
// Accessing a session variable
let username = sessionStorage.getItem(‘username’);
console.log(username); // Output: JohnDoe
// Removing a session variable
sessionStorage.removeItem(‘username’);
// Clearing all session variables
sessionStorage.clear();
Understanding Session Storage Limitations
Session storage has some limitations that developers should be aware of:
- Storage Size: Most browsers limit session storage to about 5-10 MB per origin.
- Data Type: All values stored in session storage are strings. You may need to serialize objects to store them and deserialize them when retrieving.
- Browser Support: Session storage is well-supported in modern browsers, but older versions may not support it.
Using Session Storage for Temporary Data
Session storage is particularly useful for storing temporary data that is relevant only during the user’s session. Some common use cases include:
- User Authentication Tokens: Storing tokens to maintain user sessions without needing to re-authenticate.
- Form Data: Keeping track of user inputs in multi-step forms, allowing users to navigate back without losing data.
- Temporary Application State: Storing application state that should be preserved while the user navigates through the app.
Best Practices for Using Session Storage
When working with session storage, consider the following best practices:
- Use Descriptive Keys: Ensure that keys are descriptive to avoid conflicts and improve code readability.
- Limit Data Storage: Store only essential data to optimize performance.
- Handle Serialization: Use JSON.stringify() and JSON.parse() for storing and retrieving non-string data types.
Method | Description | Example |
---|---|---|
setItem | Adds a key-value pair | sessionStorage.setItem(‘key’, ‘value’); |
getItem | Retrieves a value by key | sessionStorage.getItem(‘key’); |
removeItem | Removes a key-value pair | sessionStorage.removeItem(‘key’); |
clear | Clears all session storage | sessionStorage.clear(); |
By adhering to these practices, developers can effectively manage session variables within their applications, ensuring a seamless user experience while maintaining optimal performance.
Accessing Session Variables in JavaScript
Session variables are typically stored on the server side, but JavaScript can interact with them through various methods, depending on the technology stack being used. Here’s how to access session variables effectively.
Using JavaScript with PHP Session Variables
When using PHP to manage session variables, you can access them in JavaScript by outputting them directly into your HTML. This can be achieved by echoing the session variable into a JavaScript variable.
php
Accessing Session Variables with AJAX
If you need to access session variables without refreshing the page, AJAX calls can be used to retrieve session data from the server. This is commonly done in combination with a backend script (like PHP) that returns the session data in JSON format.
Example using jQuery AJAX:
javascript
$.ajax({
url: ‘getSessionData.php’,
method: ‘GET’,
success: function(data) {
console.log(data.username); // Outputs the username from the session
}
});
In `getSessionData.php`:
php
$_SESSION[‘username’]]);
?>
Using Node.js and Express for Session Management
In a Node.js environment using Express, session variables can be accessed through the `req.session` object. Here’s how you can handle it:
- **Set Up Express Session**:
Ensure you have the `express-session` middleware configured in your Express application.
javascript
const session = require(‘express-session’);
app.use(session({
secret: ‘your-secret-key’,
resave: ,
saveUninitialized: true
}));
- **Accessing Session Variables**:
You can then access the session variable in your routes and send it to the client.
javascript
app.get(‘/getSessionData’, (req, res) => {
res.json({ username: req.session.username });
});
In the client-side JavaScript, you can use AJAX to fetch this data similarly to the previous example.
Considerations for Security
When working with session variables, it’s essential to consider security implications:
- Data Exposure: Avoid exposing sensitive session data directly in JavaScript.
- CSRF Protection: Implement Cross-Site Request Forgery (CSRF) protection when making AJAX calls that modify session data.
- Secure Cookies: Use secure and HttpOnly flags for session cookies to enhance security.
Best Practices
- Limit Session Lifespan: Define a reasonable timeout for session variables to enhance security.
- Use HTTPS: Always use HTTPS to protect session data during transmission.
- Validate Input: Sanitize any data retrieved from session variables before using it in your application.
By following these methods and practices, you can effectively access and manage session variables in JavaScript while ensuring the security and integrity of your web applications.
Expert Insights on Accessing Session Variables in JavaScript
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “To effectively access session variables in JavaScript, developers should utilize the `sessionStorage` object. This allows for data to be stored in a session-specific context, ensuring that the information persists across page reloads but is cleared when the tab is closed.”
Mark Thompson (Senior Software Engineer, CodeCraft Solutions). “Using session variables in JavaScript primarily involves understanding the `sessionStorage` API. By calling `sessionStorage.getItem(‘key’)`, developers can retrieve stored values, making it essential for maintaining user state during a session.”
Linda Zhao (Frontend Developer, Digital Dynamics). “It’s crucial to remember that session variables are specific to the browser tab. Therefore, when implementing sessionStorage, developers must ensure that they handle data retrieval and storage appropriately to avoid data loss during navigation.”
Frequently Asked Questions (FAQs)
What are session variables in JavaScript?
Session variables are temporary storage mechanisms used to hold data specific to a user’s session. They are typically stored on the server and are accessible throughout the user’s interaction with a web application.
How can I set session variables in JavaScript?
JavaScript itself does not manage session variables directly. Instead, session variables are usually set on the server-side using languages like PHP, Node.js, or Python. JavaScript can interact with these variables through AJAX requests or by using cookies.
Can I access session variables directly in JavaScript?
No, session variables cannot be accessed directly in JavaScript. However, you can retrieve their values through server-side scripts and pass them to the client-side using JSON or other data formats.
What is the difference between session storage and session variables?
Session storage is a web storage feature that allows you to store data in the browser for the duration of the page session. In contrast, session variables are stored on the server and can persist across multiple pages in a web application.
How do I use session storage in JavaScript?
You can use the `sessionStorage` object in JavaScript to store data. For example, use `sessionStorage.setItem(‘key’, ‘value’)` to set a value and `sessionStorage.getItem(‘key’)` to retrieve it.
Are session variables secure in JavaScript?
Session variables are generally secure as they are stored on the server. However, data passed to the client-side should be handled carefully to avoid exposure to client-side attacks such as XSS (Cross-Site Scripting).
Accessing session variables in JavaScript typically involves utilizing the capabilities of the web storage API, specifically the sessionStorage object. Session variables are designed to store data for the duration of a page session, meaning they persist as long as the browser tab remains open. This feature is particularly useful for maintaining state information across different pages or during user interactions within a single tab.
To access session variables, developers can use the sessionStorage API, which provides methods such as setItem, getItem, and removeItem. By calling setItem with a key-value pair, developers can store data, while getItem allows them to retrieve that data later. It is important to note that sessionStorage is limited to the specific tab and does not share data across different tabs or windows, which distinguishes it from localStorage.
In summary, effectively managing session variables in JavaScript involves understanding the sessionStorage API and its methods. This approach allows developers to enhance user experience by preserving necessary data throughout a session. By leveraging these capabilities, applications can become more interactive and responsive to user actions, leading to improved overall functionality.
Author Profile
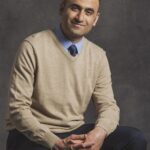
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?