How Can You Add a String to a List in Python?
In the world of programming, particularly in Python, managing data efficiently is crucial for building robust applications. One of the fundamental tasks you’ll encounter is the need to manipulate collections of data, such as lists. Whether you’re developing a simple script or a complex system, knowing how to add a string to a list can significantly enhance your ability to organize and process information. This seemingly basic operation opens the door to a plethora of possibilities, allowing you to dynamically update your data structures as your program runs.
Adding a string to a list in Python is a straightforward yet essential skill that every programmer should master. Lists in Python are versatile and can hold a variety of data types, including strings, integers, and even other lists. By understanding how to append strings to these lists, you can create more interactive and responsive applications. This operation not only enriches your data collection but also allows for greater flexibility in how you manage and utilize that data throughout your code.
As we delve deeper into this topic, we will explore various methods for adding strings to lists, including the built-in functions and techniques that Python offers. Whether you’re looking to enhance your coding repertoire or seeking solutions for specific programming challenges, mastering the art of list manipulation will empower you to write cleaner, more efficient code. Get ready to
Methods to Add a String to a List
In Python, there are several methods to add a string to a list, each serving different use cases. The most common methods include `append()`, `insert()`, and `extend()`. Understanding when and how to use these methods can enhance your efficiency in managing lists.
Using the append() Method
The `append()` method is used to add a single string to the end of a list. This method modifies the list in place and does not return a new list.
Example:
“`python
my_list = [‘apple’, ‘banana’]
my_list.append(‘cherry’)
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Using the insert() Method
The `insert()` method allows you to add a string at a specific position in the list. This method takes two arguments: the index at which to insert the item and the item itself.
Example:
“`python
my_list = [‘apple’, ‘banana’]
my_list.insert(1, ‘cherry’) Insert ‘cherry’ at index 1
print(my_list) Output: [‘apple’, ‘cherry’, ‘banana’]
“`
Using the extend() Method
The `extend()` method is used to add multiple strings to a list at once. This method takes an iterable (like another list) and appends each element to the end of the existing list.
Example:
“`python
my_list = [‘apple’, ‘banana’]
my_list.extend([‘cherry’, ‘date’])
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’, ‘date’]
“`
Comparison of Methods
Choosing the appropriate method depends on the specific requirement of your task. The following table summarizes the differences:
Method | Description | Usage |
---|---|---|
append() | Adds a single item to the end of the list | my_list.append(‘item’) |
insert() | Adds an item at a specified index | my_list.insert(index, ‘item’) |
extend() | Adds multiple items from an iterable | my_list.extend([‘item1’, ‘item2’]) |
Best Practices
When working with lists in Python, consider the following best practices:
- Use `append()` when you simply need to add a single item.
- Use `insert()` sparingly, as it can be less efficient for larger lists due to the need to shift elements.
- Use `extend()` when you want to merge another iterable into your list to keep your code concise and readable.
- Always be mindful of the data type being added to ensure consistency within the list.
Methods to Add a String to a List in Python
In Python, there are several methods to add a string to a list, each serving different use cases. Below are the primary methods:
Using the `append()` Method
The `append()` method adds a single element to the end of a list. This is the most straightforward way to add a string.
“`python
my_list = [‘apple’, ‘banana’]
my_list.append(‘cherry’)
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Key Points:
- Adds the string as a single element.
- Modifies the original list in place.
Using the `extend()` Method
The `extend()` method allows you to add multiple elements to a list. If you want to add a string as an iterable, you can use this method.
“`python
my_list = [‘apple’, ‘banana’]
my_list.extend(‘cherry’)
print(my_list) Output: [‘apple’, ‘banana’, ‘c’, ‘h’, ‘e’, ‘r’, ‘r’, ‘y’]
“`
Key Points:
- The string is treated as an iterable, adding each character separately.
- Use `extend()` if you want to add elements from another iterable, like a list or a tuple.
Using the `insert()` Method
The `insert()` method allows you to add a string at a specific index in the list.
“`python
my_list = [‘apple’, ‘banana’]
my_list.insert(1, ‘cherry’)
print(my_list) Output: [‘apple’, ‘cherry’, ‘banana’]
“`
Key Points:
- The first argument is the index where the string will be inserted.
- Shifts the subsequent elements to the right.
Using List Concatenation
You can also concatenate lists to add a string, which creates a new list.
“`python
my_list = [‘apple’, ‘banana’]
new_list = my_list + [‘cherry’]
print(new_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Key Points:
- Creates a new list; the original list remains unchanged.
- Suitable for adding multiple strings at once by concatenating lists.
Using List Comprehension
List comprehension can be used when you want to create a new list based on an existing one, including the new string.
“`python
my_list = [‘apple’, ‘banana’]
new_list = [x for x in my_list] + [‘cherry’]
print(new_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Key Points:
- Provides a flexible way to modify or build lists.
- Useful for applying conditions or transformations while adding.
Performance Considerations
The choice of method may impact performance based on the list size and the number of elements being added. Here’s a brief comparison:
Method | In-place Modification | Time Complexity | Suitable for Adding Multiple Elements |
---|---|---|---|
`append()` | Yes | O(1) | No |
`extend()` | Yes | O(k) | Yes |
`insert()` | Yes | O(n) | No |
Concatenation | No | O(n) | Yes |
Select the method that best fits your requirements based on the context of use and performance considerations.
Expert Insights on Adding Strings to Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To add a string to a list in Python, one can utilize the `append()` method, which is specifically designed for this purpose. This method efficiently adds the string as the last element of the list, ensuring that the list structure remains intact.”
Michael Chen (Python Developer, CodeMaster Solutions). “Another effective way to add a string to a list is by using the `insert()` method. This method allows for more flexibility, as it enables the user to specify the index at which the string should be added, thus providing control over the list’s order.”
Lisa Patel (Data Scientist, Analytics Hub). “For scenarios where multiple strings need to be added at once, the `extend()` method is highly recommended. This method takes an iterable as an argument and appends each element to the list, making it a powerful tool for batch additions.”
Frequently Asked Questions (FAQs)
How can I add a string to an existing list in Python?
You can use the `append()` method to add a string to the end of a list. For example, `my_list.append(“new_string”)` will add “new_string” to `my_list`.
Is there a way to insert a string at a specific position in a list?
Yes, you can use the `insert()` method to add a string at a specific index. For example, `my_list.insert(1, “new_string”)` will insert “new_string” at index 1.
Can I add multiple strings to a list at once?
Yes, you can use the `extend()` method to add multiple strings. For example, `my_list.extend([“string1”, “string2”])` will add both “string1” and “string2” to `my_list`.
What happens if I add a string to a list that already contains it?
If you add a string to a list that already contains it, the string will be added again, resulting in duplicate entries. Lists in Python allow duplicates.
How do I check if a string is already in a list before adding it?
You can use the `in` keyword to check for existence. For example, `if “new_string” not in my_list: my_list.append(“new_string”)` will add “new_string” only if it is not already present.
What is the difference between `append()` and `extend()` methods?
The `append()` method adds a single element to the end of the list, while the `extend()` method adds multiple elements from an iterable to the end of the list.
In Python, adding a string to a list can be accomplished using several methods, each suited for different scenarios. The most straightforward approach is to use the `append()` method, which adds the string as a single element at the end of the list. Alternatively, the `insert()` method allows for adding a string at a specific index, providing greater control over the list’s structure. For cases where multiple strings need to be added, the `extend()` method can be utilized, which merges another iterable into the list.
Additionally, list concatenation using the `+` operator can be employed to create a new list that combines the original list with the new string. It is important to note that while `append()` and `extend()` modify the list in place, concatenation produces a new list, which may have implications for memory usage and performance in larger applications.
In summary, Python offers flexible methods for adding strings to lists, including `append()`, `insert()`, `extend()`, and concatenation. Understanding the nuances of each method allows developers to choose the most efficient approach based on their specific needs and the context of their code. This knowledge is essential for effective list manipulation and contributes to writing clean, efficient Python code.
Author Profile
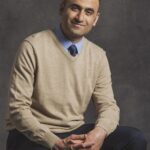
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?