How Can You Add Commas in a Slice in Golang?
In the world of Go programming, managing data structures efficiently is crucial for building robust applications. One common task that developers encounter is the need to format slices—especially when it comes to presenting data in a human-readable format. A frequent requirement is adding commas between elements in a slice, which can enhance clarity and improve the overall presentation of information. Whether you’re crafting a user interface, generating output for logs, or preparing data for APIs, knowing how to seamlessly insert commas into slices can elevate your coding skills and streamline your development process.
When working with slices in Go, you may find yourself needing to convert them into a string format that is both readable and aesthetically pleasing. The challenge lies in ensuring that commas are placed correctly, without trailing or leading unnecessary punctuation. This task may seem straightforward, but it involves understanding Go’s string manipulation capabilities and slice operations. By mastering this technique, you can transform raw data into a polished format that meets your project’s requirements.
In this article, we will explore various methods to add commas between elements in a slice, providing you with practical examples and best practices. Whether you’re a seasoned Go developer or just starting out, you’ll gain valuable insights that will enhance your coding repertoire. Get ready to dive into the world of Go slices and discover how to make
Adding Commas in a Slice in Go
In Go, adding commas to elements in a slice can be achieved through various methods, depending on the desired output format. Below are some techniques for formatting slice elements with commas.
Using String Join
The most straightforward method to add commas between elements of a slice is to use the `strings.Join` function. This function concatenates the elements of a slice into a single string, inserting a specified separator between each element.
Here’s how to implement it:
“`go
package main
import (
“fmt”
“strings”
)
func main() {
slice := []string{“apple”, “banana”, “cherry”}
result := strings.Join(slice, “, “)
fmt.Println(result) // Output: apple, banana, cherry
}
“`
In this example, the slice contains three fruit names, and `strings.Join` combines them into a single string with commas separating each item.
Using a Loop
Alternatively, if you need more control over the formatting or want to customize the output further, you can build the string manually using a loop:
“`go
package main
import (
“fmt”
)
func main() {
slice := []string{“apple”, “banana”, “cherry”}
var result string
for i, fruit := range slice {
if i > 0 {
result += “, ”
}
result += fruit
}
fmt.Println(result) // Output: apple, banana, cherry
}
“`
In this example, a loop iterates through the slice, appending a comma before each element except the first.
Handling Edge Cases
When adding commas, consider edge cases such as empty slices or slices with a single element. Here’s how to handle these scenarios:
“`go
package main
import (
“fmt”
“strings”
)
func formatSlice(slice []string) string {
if len(slice) == 0 {
return “”
}
return strings.Join(slice, “, “)
}
func main() {
emptySlice := []string{}
singleElementSlice := []string{“apple”}
fmt.Println(formatSlice(emptySlice)) // Output: (empty)
fmt.Println(formatSlice(singleElementSlice)) // Output: apple
}
“`
This function checks the length of the slice before attempting to join its elements, ensuring that it returns an appropriate response for empty or single-element slices.
Performance Considerations
When working with large slices, it’s important to consider performance. The `strings.Join` method is generally more efficient than manually concatenating strings in a loop, as it avoids multiple allocations.
Method | Performance | Use Case |
---|---|---|
`strings.Join` | High | Large slices, general use |
Loop | Moderate | Custom formatting requirements |
In summary, depending on your specific needs, you can choose between `strings.Join` for straightforward cases or a manual loop for more complex formatting tasks.
Adding Commas in a Slice in Go
To add commas between elements in a slice in Go, you can use the `strings.Join` function from the `strings` package. This function concatenates the elements of a slice into a single string, inserting a specified separator between each element.
Example Code
Here’s a simple example demonstrating how to add commas between elements of a string slice:
“`go
package main
import (
“fmt”
“strings”
)
func main() {
fruits := []string{“apple”, “banana”, “cherry”}
result := strings.Join(fruits, “, “)
fmt.Println(result) // Output: apple, banana, cherry
}
“`
In this example, the `strings.Join` function takes two arguments:
- The slice of strings (`fruits`).
- The string that will serve as the separator (in this case, a comma followed by a space `”, “`).
Detailed Explanation
- Importing the Package: Ensure you import the `strings` package to access the `Join` function.
- Slice Declaration: Declare a slice of strings containing the elements you want to join.
- Using `strings.Join`: Call `strings.Join` with your slice and the desired separator to create a single string.
- Output the Result: Use `fmt.Println` to display the output.
Handling Different Data Types
If you are working with a slice of different data types (e.g., integers), you will need to convert each element to a string before using `strings.Join`. Here’s how to do it:
“`go
package main
import (
“fmt”
“strconv”
“strings”
)
func main() {
numbers := []int{1, 2, 3}
strNumbers := make([]string, len(numbers))
for i, num := range numbers {
strNumbers[i] = strconv.Itoa(num) // Convert int to string
}
result := strings.Join(strNumbers, “, “)
fmt.Println(result) // Output: 1, 2, 3
}
“`
In this modified example:
- Conversion: The `strconv.Itoa` function is used to convert each integer to a string.
- String Slice: A new slice (`strNumbers`) is created to hold the string representations of the numbers.
Performance Considerations
When working with large slices or in performance-sensitive applications, consider the following:
- Memory Allocation: `strings.Join` is efficient as it preallocates memory for the final string, minimizing allocations.
- Element Type: Ensure that the elements are of the same type (or convertible to a common type) to avoid unnecessary type assertions or conversions.
Common Use Cases
Adding commas in slices is commonly used in scenarios such as:
- Formatting output for logging or display.
- Creating CSV strings for file writing.
- Generating query parameters in URLs.
Utilizing `strings.Join` provides a clean and efficient way to achieve this in Go.
Expert Insights on Adding Commas in Slices in Golang
Emily Chen (Senior Software Engineer, GoLang Solutions). “In Go, to add a comma between elements in a slice, you can utilize the `strings.Join` function, which allows you to concatenate the elements of the slice into a single string with a specified separator. This is particularly useful for formatting output.”
Michael Thompson (Golang Developer Advocate, Tech Innovations). “When working with slices in Go, it is essential to remember that commas are not inherently part of the slice structure. To format the output with commas, you should convert the slice to a string using `fmt.Sprintf` or `strings.Join`, ensuring that the desired formatting is achieved.”
Sarah Patel (Lead Golang Instructor, Code Academy). “Adding commas in a slice is a common requirement for displaying data. Utilizing a loop to iterate through the slice and appending a comma conditionally can also be effective, but leveraging built-in functions like `strings.Join` is more efficient and cleaner.”
Frequently Asked Questions (FAQs)
How can I format a slice of strings to include commas in Go?
To format a slice of strings with commas, you can use the `strings.Join()` function from the `strings` package. This function takes a slice of strings and a separator (in this case, a comma) and returns a single string with the elements joined by the specified separator.
What is the syntax for using strings.Join() in Go?
The syntax for using `strings.Join()` is as follows:
“`go
result := strings.Join(slice, “, “)
“`
Here, `slice` is your slice of strings, and `”, “` is the separator you want to use.
Can I add a comma after the last element in a slice in Go?
Typically, adding a comma after the last element is not standard practice in string formatting. If you require this, you can manually concatenate a comma to the result after using `strings.Join()`.
Is there a way to add different separators between elements in a slice?
Yes, you can achieve this by iterating through the slice and manually constructing the string. Alternatively, you can use a loop to append elements with different separators as needed.
How do I handle empty slices when adding commas in Go?
If the slice is empty, `strings.Join()` will return an empty string. You can check if the slice is empty before calling `strings.Join()` to handle such cases appropriately.
Can I use a custom function to format slices with commas in Go?
Yes, you can define a custom function to format slices with commas or any other desired formatting. This function can iterate through the slice and apply your specific formatting logic.
In Golang, adding a comma to elements within a slice can be accomplished through various methods, depending on the desired output format. One common approach is to iterate over the slice and concatenate each element with a comma. This can be efficiently achieved using the `strings.Join` function, which simplifies the process by allowing developers to specify a separator, such as a comma, while joining the elements of a slice into a single string.
Another method involves using a loop to build a new string manually, appending each element followed by a comma. However, this approach requires careful handling to avoid trailing commas, which can lead to formatting issues. Developers should also consider edge cases, such as empty slices or slices with a single element, to ensure that the output remains clean and user-friendly.
Ultimately, the choice of method will depend on the specific requirements of the application and the complexity of the data being handled. Utilizing built-in functions like `strings.Join` not only enhances code readability but also improves performance by reducing the overhead associated with string concatenation in loops.
Author Profile
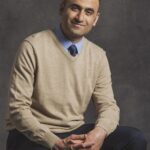
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?