How Can You Easily Add Elements to a Set in Python?
In the world of programming, data structures play a crucial role in how we store and manipulate information. Among these structures, sets in Python stand out for their simplicity and efficiency. If you’ve ever needed a way to manage a collection of unique items—be it a list of user IDs, a collection of tags, or even a group of friends—sets offer an elegant solution. But how do you effectively add elements to a set in Python? Whether you’re a beginner eager to learn or an experienced coder looking to refine your skills, understanding the nuances of set operations can significantly enhance your coding toolkit.
Sets are unordered collections that automatically handle duplicates, making them a powerful choice for various applications. In Python, adding elements to a set is not just about inserting values; it’s about leveraging the unique properties of sets to maintain data integrity and optimize performance. As you delve deeper into the mechanics of set manipulation, you’ll discover the different methods available for adding items, each with its own advantages and use cases.
From the straightforward addition of single elements to the more complex merging of multiple sets, mastering these techniques will empower you to handle data in a more organized and efficient manner. So, whether you’re looking to streamline your code or simply explore the capabilities of Python’s set data type, this
Adding Elements to a Set
In Python, sets are a versatile collection type that allows for the storage of unique elements. There are several methods to add elements to a set, each suited for different scenarios.
Using the `add()` Method
The simplest way to add a single element to a set is by using the `add()` method. This method will insert the specified element into the set if it is not already present.
“`python
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) Output: {1, 2, 3, 4}
“`
Using the `update()` Method
When you need to add multiple elements to a set at once, the `update()` method is the most efficient choice. This method can accept any iterable, such as a list, tuple, or even another set.
“`python
my_set = {1, 2, 3}
my_set.update([4, 5, 6])
print(my_set) Output: {1, 2, 3, 4, 5, 6}
“`
Examples of Adding Elements
The following table summarizes different methods to add elements to a set in Python, highlighting the method and usage.
Method | Usage | Example |
---|---|---|
`add()` | Adds a single element | `my_set.add(7)` |
`update()` | Adds multiple elements | `my_set.update([8, 9])` |
`update()` with another set | Adds elements from another set | `my_set.update({10, 11})` |
Handling Duplicate Elements
A key characteristic of sets is that they automatically handle duplicate elements. When you attempt to add an element that already exists in the set, Python will ignore the addition without raising an error.
“`python
my_set = {1, 2, 3}
my_set.add(2) No effect since 2 is already in the set
print(my_set) Output: {1, 2, 3}
“`
Conclusion on Adding Elements
Understanding how to effectively add elements to a set in Python will enhance your ability to manipulate collections. The `add()` and `update()` methods provide flexibility depending on whether you are adding single or multiple elements. This functionality is integral to leveraging the full potential of sets in your programming tasks.
Adding Elements to a Set in Python
In Python, sets are mutable collections that store unique elements. There are several methods available for adding elements to a set, each suited for different scenarios.
Using the `add()` Method
The `add()` method is the most straightforward way to insert a single element into a set. If the element already exists in the set, the method will not add it again, ensuring the uniqueness of set elements.
“`python
my_set = {1, 2, 3}
my_set.add(4) Adds 4 to the set
my_set.add(2) Does not add, as 2 is already present
print(my_set) Output: {1, 2, 3, 4}
“`
Using the `update()` Method
When you need to add multiple elements at once, the `update()` method is ideal. This method can accept any iterable, including lists, tuples, and other sets.
“`python
my_set = {1, 2, 3}
my_set.update([4, 5]) Adds 4 and 5 to the set
my_set.update((6, 7)) Adds 6 and 7 as well
print(my_set) Output: {1, 2, 3, 4, 5, 6, 7}
“`
Adding Elements from Another Set
When combining two sets, the `update()` method can also be used to add all elements from another set.
“`python
set_a = {1, 2, 3}
set_b = {3, 4, 5}
set_a.update(set_b) Merges set_b into set_a
print(set_a) Output: {1, 2, 3, 4, 5}
“`
Using `|=` Operator
The `|=` operator provides a shorthand for the `update()` method, allowing for an in-place union of sets.
“`python
set_a = {1, 2, 3}
set_b = {3, 4, 5}
set_a |= set_b Adds all elements from set_b to set_a
print(set_a) Output: {1, 2, 3, 4, 5}
“`
Performance Considerations
- Time Complexity:
- `add()`: O(1) for a single element.
- `update()`: O(n) for adding multiple elements, where n is the number of elements being added.
- Space Complexity: Sets dynamically resize, but the space used is proportional to the number of unique elements.
Common Use Cases
- Data Deduplication: Use sets to store elements from a list while removing duplicates.
- Membership Testing: Quickly check for the existence of an element due to the average O(1) time complexity for lookups in sets.
By leveraging these methods, you can efficiently manage and manipulate sets in Python, ensuring optimal performance and clarity in your code.
Expert Insights on Adding Elements to a Set in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Adding elements to a set in Python is straightforward using the `add()` method, which allows for the insertion of a single element. Additionally, the `update()` method can be utilized to add multiple elements from an iterable, making it a versatile choice for developers.”
Michael Thompson (Python Developer, CodeCraft Solutions). “When working with sets in Python, it is crucial to remember that they are unordered collections. Thus, while adding elements, duplicates are automatically ignored, ensuring that each element remains unique. This feature is particularly beneficial for data integrity in applications.”
Lisa Chen (Data Scientist, Analytics Hub). “In Python, the immutability of sets means that once created, their size can change only through methods like `add()` and `update()`. This characteristic is essential for managing large datasets efficiently, as it allows for dynamic updates without the overhead of managing duplicates.”
Frequently Asked Questions (FAQs)
How do I add a single element to a set in Python?
You can add a single element to a set using the `add()` method. For example, `my_set.add(element)` will add `element` to `my_set`.
Can I add multiple elements to a set at once?
Yes, you can add multiple elements to a set using the `update()` method. For instance, `my_set.update([element1, element2, element3])` will add all specified elements to `my_set`.
What happens if I try to add a duplicate element to a set?
Sets automatically handle duplicates by ignoring any duplicate elements. If you attempt to add an existing element, the set remains unchanged.
Is there a difference between `add()` and `update()` methods?
Yes, `add()` is used for adding a single element, while `update()` can add multiple elements from an iterable, such as a list or another set.
Can I add elements of different data types to a set?
Yes, sets in Python can contain elements of different data types, including integers, strings, and tuples, as long as the elements are hashable.
What types of objects cannot be added to a set?
Unhashable objects, such as lists and dictionaries, cannot be added to a set. Only immutable types, like strings and tuples, are allowed.
In Python, sets are a built-in data type that allows for the storage of unique elements. To add elements to a set, the most common method is using the `add()` method, which appends a single element to the set. If you need to add multiple elements at once, the `update()` method is available, which can accept an iterable such as a list, tuple, or another set. It is important to note that sets automatically handle duplicates, meaning that if an element already exists in the set, it will not be added again.
When working with sets, understanding the difference between `add()` and `update()` is crucial for efficient data manipulation. The `add()` method is straightforward and effective for individual elements, while `update()` provides flexibility for bulk additions. Additionally, since sets are unordered collections, the order of elements does not matter, which can simplify operations when the order is not a concern.
In summary, adding elements to a set in Python can be efficiently accomplished using either the `add()` or `update()` methods, depending on the specific needs of the operation. This functionality, combined with the inherent properties of sets, makes them a powerful tool for managing unique collections of data in Python programming.
Author Profile
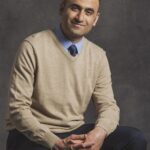
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?