How Can You Effectively Add Information to a Combo Box in an Excel UserForm?
When it comes to creating dynamic and user-friendly applications in Excel, user forms play a pivotal role. Among the various controls available, the combo box stands out as a powerful tool for enhancing user interaction. Whether you’re building a simple data entry form or a complex dashboard, knowing how to effectively add information to a combo box can significantly streamline the user experience. This article will guide you through the essential steps and best practices for populating combo boxes in Excel user forms, empowering you to create more intuitive and efficient applications.
Understanding the fundamentals of combo boxes is crucial for anyone looking to harness the full potential of Excel user forms. A combo box allows users to select from a predefined list of options, minimizing input errors and ensuring consistency in data entry. By adding information to a combo box, you not only make your forms more interactive but also enhance their functionality. This overview will touch on the methods available for populating combo boxes, including manual entry, dynamic data sourcing, and leveraging Excel ranges.
As we delve deeper into the topic, you will discover various techniques to manage the data within your combo boxes effectively. From setting up your user form to dynamically updating the list based on user input or other criteria, mastering these skills will elevate your Excel projects to new heights. Prepare to transform your user forms
Using the Excel VBA Editor
To add information to a combo box in an Excel UserForm, you need to access the Excel Visual Basic for Applications (VBA) editor. This environment allows you to manipulate various aspects of Excel, including UserForms and their controls.
- Open Excel and press `ALT + F11` to launch the VBA editor.
- In the Project Explorer window, locate the UserForm where your combo box resides. If you haven’t created a UserForm yet, you can do so by inserting a new UserForm from the `Insert` menu.
- Click on the UserForm to select it, then identify your combo box control.
Adding Items to the Combo Box
You can add items to a combo box either at design time or run time.
Design Time: This method allows you to predefine items in the combo box.
- Select the combo box in the UserForm.
- In the Properties window, find the `List` property.
- Click the ellipsis (`…`) button to open the List Editor, where you can manually enter items.
Run Time: This approach enables dynamic addition of items to the combo box when the UserForm is initialized or during specific events.
To add items at run time, you typically use the `UserForm_Initialize` event. Here’s a sample code snippet to illustrate:
“`vba
Private Sub UserForm_Initialize()
With Me.ComboBox1
.AddItem “Option 1”
.AddItem “Option 2”
.AddItem “Option 3”
End With
End Sub
“`
This code should be placed in the code window for your UserForm. When the UserForm is loaded, “Option 1”, “Option 2”, and “Option 3” will appear in the combo box.
Populating the Combo Box from a Range
Another effective method for filling a combo box is to populate it from a range of cells in a worksheet. This can be particularly useful when dealing with large datasets.
Here’s how to do it:
- Define the range in your worksheet that contains the items you want to add to the combo box.
- Use the following code snippet in the `UserForm_Initialize` event:
“`vba
Private Sub UserForm_Initialize()
Dim cell As Range
For Each cell In ThisWorkbook.Sheets(“Sheet1”).Range(“A1:A10”)
If cell.Value <> “” Then
Me.ComboBox1.AddItem cell.Value
End If
Next cell
End Sub
“`
In this example, the combo box will be populated with values from cells A1 to A10 on “Sheet1”.
Table of Combo Box Properties
To better understand the properties that can be manipulated for a combo box, consider the following table:
Property | Description |
---|---|
List | Contains the items displayed in the combo box. |
Style | Determines the appearance of the combo box (dropdown or simple). |
BoundColumn | Indicates which column is bound to the control in case of multi-column lists. |
Value | Displays the selected item from the list. |
By utilizing these properties and methods, you can effectively manage the items in a combo box within an Excel UserForm, enhancing the interactivity and functionality of your Excel applications.
Adding Items to a Combo Box in Excel UserForm
To populate a Combo Box in an Excel UserForm, you can either add items at design time or dynamically at runtime using VBA code. Below are detailed steps for both approaches.
Design Time: Adding Items to Combo Box
- **Open the Visual Basic for Applications (VBA) Editor**:
- Press `ALT + F11` in Excel to access the VBA editor.
- **Insert a UserForm**:
- Right-click on any of the objects for your workbook in the Project Explorer.
- Select `Insert > UserForm`.
- Add a Combo Box:
- From the Toolbox, select the Combo Box control and draw it on the UserForm.
- Configure the Combo Box:
- Right-click on the Combo Box, and select `Properties`.
- In the properties window, find the `List` property.
- Click on the ellipsis `(…)` button next to `List` to open the List Box Editor.
- Enter the items you want to include, each on a new line.
- Close the properties window:
- Save your changes.
Runtime: Adding Items Using VBA Code
To dynamically populate the Combo Box when the UserForm is initialized, you can use the following steps:
- Open the Code Window:
- In the UserForm, double-click on it to open the code window.
- Write the Initialization Code:
- Use the `UserForm_Initialize` event to add items to the Combo Box. Here is an example of the code:
“`vba
Private Sub UserForm_Initialize()
With Me.ComboBox1
.AddItem “Option 1”
.AddItem “Option 2”
.AddItem “Option 3”
.AddItem “Option 4”
End With
End Sub
“`
- Run the UserForm:
- Close the code window and run the UserForm to see the Combo Box populated with the specified items.
Adding Items from a Range
You can also populate a Combo Box with data from an Excel worksheet range. This is useful for larger datasets:
- Using a Range:
- Modify the `UserForm_Initialize` event to read from a specified range in your worksheet:
“`vba
Private Sub UserForm_Initialize()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets(“Sheet1”) ‘ Specify your sheet name
Dim cell As Range
For Each cell In ws.Range(“A1:A10”) ‘ Specify your range
If Not IsEmpty(cell.Value) Then
Me.ComboBox1.AddItem cell.Value
End If
Next cell
End Sub
“`
- Ensure Data Integrity:
- Before running the UserForm, ensure that the specified range contains no blank cells if you want all entries in the Combo Box.
Additional Considerations
- Clearing Existing Items: If you need to clear existing items before adding new ones, use the following line in your initialization code:
“`vba
Me.ComboBox1.Clear
“`
- Error Handling: Implement error handling to manage potential issues, such as an invalid range or empty data sources.
- Dynamic Update: If you need to update the Combo Box items based on user actions or other events, consider adding a separate subroutine to manage those changes dynamically.
By following these methods, you can effectively add information to a Combo Box in an Excel UserForm, enhancing user interaction and data input efficiency.
Expert Insights on Adding Information to Combo Boxes in Excel UserForms
Dr. Emily Carter (Excel VBA Specialist, Tech Solutions Inc.). “To effectively add information to a combo box in an Excel UserForm, it is essential to utilize the List property of the combo box control. This allows for dynamic data entry and enhances user interaction significantly.”
Michael Thompson (Data Management Consultant, DataWise Group). “Using the AddItem method in VBA is a straightforward approach to populate combo boxes with data from a range. This method ensures that users can easily access and select the necessary options, streamlining the data entry process.”
Sarah Jenkins (Software Development Trainer, Code Academy). “Incorporating error handling while adding items to a combo box is crucial. It prevents runtime errors and ensures that the user experience remains smooth, especially when dealing with large datasets.”
Frequently Asked Questions (FAQs)
How do I add items to a combo box in an Excel UserForm?
To add items to a combo box in an Excel UserForm, you can use the `AddItem` method in the UserForm’s code. For example, in the UserForm’s `Initialize` event, you can write: `ComboBox1.AddItem “Item1″` to add “Item1” to the combo box.
Can I populate a combo box from a range in an Excel worksheet?
Yes, you can populate a combo box from a range in an Excel worksheet by looping through the cells in the specified range and using the `AddItem` method. For instance, you can use a `For Each` loop to add each cell’s value to the combo box.
Is it possible to clear items from a combo box in Excel UserForm?
Yes, you can clear items from a combo box by using the `Clear` method. For example, you can use `ComboBox1.Clear` in your code to remove all existing items from the combo box.
How can I set a default value for a combo box in an Excel UserForm?
To set a default value for a combo box, you can assign a value to the combo box’s `Value` property after populating it. For example, `ComboBox1.Value = “DefaultItem”` sets “DefaultItem” as the selected value.
Can I allow users to type in the combo box in Excel UserForm?
Yes, you can allow users to type in the combo box by setting its `Style` property to `fmStyleDropDown`. This enables users to either select from the list or enter their own value.
What event should I use to handle changes in the combo box selection?
To handle changes in the combo box selection, you should use the `Change` event. This event triggers whenever the user selects a different item from the combo box, allowing you to execute specific code based on the selection.
Incorporating information into a combo box within an Excel userform is a fundamental task that enhances user interactivity and data selection efficiency. To achieve this, one can utilize the properties of the combo box control, specifically the ‘AddItem’ method, which allows for the dynamic addition of items during runtime. This process can be executed in the userform’s initialization event, ensuring that the combo box is populated with the desired options as soon as the form is loaded.
Furthermore, it is essential to understand the various ways to populate a combo box. Data can be added manually through code, or it can be sourced from a worksheet range, providing flexibility depending on the application’s requirements. Utilizing the ‘RowSource’ property is another effective method for linking a combo box directly to a range of cells in a worksheet, allowing for automatic updates whenever the source data changes.
mastering the addition of information to a combo box in an Excel userform not only streamlines data entry but also significantly improves the user experience. By leveraging the appropriate methods and properties, developers can create intuitive and responsive forms that cater to their specific data handling needs. Understanding these techniques is crucial for anyone looking to enhance their Excel-based applications.
Author Profile
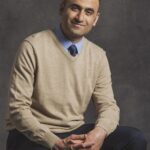
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?