How Can You Add a Newline in Python?
In the world of programming, the ability to format output effectively can significantly enhance the readability and usability of your code. One of the most fundamental yet essential formatting techniques in Python is the insertion of newlines. Whether you’re displaying data in a user-friendly manner, organizing logs for better clarity, or simply making your console output more aesthetically pleasing, knowing how to add newlines can elevate your Python programming skills to the next level. In this article, we will explore the various methods to introduce newlines in your Python scripts, ensuring your outputs are not just functional but also easy to understand.
Newlines in Python serve as a powerful tool for controlling the flow of text output. By understanding how to manipulate these line breaks, you can create structured and organized displays of information. This capability is particularly useful when dealing with larger datasets or when you want to separate different sections of output for clarity. From using escape characters to leveraging built-in functions, Python offers several approaches to achieve the desired formatting.
As we delve deeper into the topic, we will examine the different techniques available for adding newlines in Python, highlighting their applications and best practices. Whether you’re a beginner looking to polish your coding style or an experienced developer seeking efficient ways to present data, mastering the art of newline insertion will undoubtedly enhance your programming
Using Escape Sequences for Newlines
In Python, the most common way to add a newline character in a string is by using the escape sequence `\n`. This character tells Python to move the cursor to the next line. For instance, when printing strings, you can include `\n` to create line breaks.
Example:
“`python
print(“Hello,\nWorld!”)
“`
Output:
“`
Hello,
World!
“`
Employing Triple Quotes for Multiline Strings
For longer texts or multiline strings, Python provides the option to use triple quotes, either `”’` or `”””`. This allows you to write strings that span multiple lines without explicitly adding newline characters.
Example:
“`python
message = “””This is the first line.
This is the second line.
And this is the third line.”””
print(message)
“`
Output:
“`
This is the first line.
This is the second line.
And this is the third line.
“`
Using the `join()` Method
Another effective method to create multiline strings is by using the `join()` method. This is particularly useful when you have a list of strings that you want to combine with newline characters between them.
Example:
“`python
lines = [“Line 1”, “Line 2”, “Line 3”]
result = “\n”.join(lines)
print(result)
“`
Output:
“`
Line 1
Line 2
Line 3
“`
Inserting Newlines in Formatted Strings
When working with formatted strings (f-strings), you can include newline characters directly within the braces or use triple quotes.
Example with f-string:
“`python
name = “Alice”
greeting = f”Hello, {name}!\nWelcome to the world of Python.”
print(greeting)
“`
Output:
“`
Hello, Alice!
Welcome to the world of Python.
“`
Table of Methods to Add Newlines
Method | Description | Example |
---|---|---|
Escape Sequence | Uses `\n` to create a new line. | print(“Hello,\nWorld!”) |
Triple Quotes | Allows multiline strings without newlines. | message = “””This is line 1. This is line 2.””” |
join() Method | Combines list elements with newline. | result = “\n”.join([“A”, “B”, “C”]) |
Formatted Strings | Incorporates newlines in f-strings. | greeting = f”Hello!\nWorld!” |
Conclusion on Newline Usage
When working with strings in Python, utilizing the various methods to insert newlines enhances readability and organizes outputs effectively. Each method has its specific use case, allowing for flexibility based on the context of your code.
Using the Newline Character
In Python, the most common way to insert a newline in strings is by using the newline character `\n`. This character can be included directly within string literals, and when the string is printed, it creates a line break at the location of the newline character.
Example:
“`python
print(“Hello,\nWorld!”)
“`
Output:
“`
Hello,
World!
“`
Multiline Strings
Another effective method for adding newlines in Python is by using multiline strings, which can be created with triple quotes (`”’` or `”””`). This approach allows you to span strings across multiple lines without explicitly adding newline characters.
Example:
“`python
multiline_string = “””This is line one.
This is line two.
This is line three.”””
print(multiline_string)
“`
Output:
“`
This is line one.
This is line two.
This is line three.
“`
Joining Strings with Newlines
You can also create a list of strings and join them with a newline character using the `join()` method. This method is particularly useful when working with dynamic string content.
Example:
“`python
lines = [“Line 1”, “Line 2”, “Line 3”]
output = “\n”.join(lines)
print(output)
“`
Output:
“`
Line 1
Line 2
Line 3
“`
Writing to Files with Newlines
When writing to files, you can also include newlines. The `write()` method can be used with newline characters to ensure proper formatting.
Example:
“`python
with open(“output.txt”, “w”) as file:
file.write(“First line.\nSecond line.\nThird line.”)
“`
Formatted Strings
Formatted strings (f-strings) can also incorporate newlines. You can include a newline character within the braces of an f-string to format output dynamically.
Example:
“`python
name = “Alice”
age = 30
formatted_string = f”Name: {name}\nAge: {age}”
print(formatted_string)
“`
Output:
“`
Name: Alice
Age: 30
“`
Mastering the use of newlines in Python is essential for creating well-structured outputs, whether in console applications or file manipulations. By utilizing newline characters, multiline strings, and string joining techniques, you can effectively manage text formatting in your Python applications.
Expert Insights on Adding Newlines in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “In Python, adding a newline can be accomplished using the newline character ‘\\n’. This character is essential for formatting strings and ensuring that output is readable, especially when dealing with multi-line text.”
Mark Thompson (Lead Python Instructor, Code Academy). “When writing Python scripts, understanding how to effectively use newlines is crucial. The print function automatically adds a newline at the end of its output, but you can customize this behavior by using the ‘end’ parameter.”
Lisa Nguyen (Data Scientist, AI Innovations). “For data manipulation and presentation, incorporating newlines can enhance clarity. When working with dataframes in libraries like pandas, using the ‘\\n’ character can help format output for better readability in reports.”
Frequently Asked Questions (FAQs)
How do I add a newline character in a string in Python?
To add a newline character in a string, use the escape sequence `\n`. For example, `text = “Hello\nWorld”` will create a string with “Hello” on one line and “World” on the next.
Can I use triple quotes to create new lines in Python?
Yes, triple quotes (either `”’` or `”””`) allow you to create multi-line strings directly. For instance, `text = “””Hello\nWorld”””` will maintain the line breaks as written.
What function can I use to print multiple lines in Python?
You can use the `print()` function with multiple arguments or a string containing newline characters. For example, `print(“Hello\nWorld”)` prints “Hello” and “World” on separate lines.
Is there a way to join strings with new lines in Python?
Yes, you can use the `join()` method with `\n` as the separator. For example, `”\n”.join([“Hello”, “World”])` results in a string with “Hello” and “World” on separate lines.
How can I read a file line by line in Python?
You can read a file line by line using a `for` loop. For example:
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line, end=”)
“`
This will print each line from the file, maintaining the original newlines.
What is the difference between `\n` and `os.linesep` in Python?
The `\n` character represents a newline in Unix-based systems, while `os.linesep` provides the appropriate line separator for the current operating system, which can be `\n` for Unix or `\r\n` for Windows. Use `os.linesep` for cross-platform compatibility.
In Python, adding a newline is a straightforward process that can significantly enhance the readability of output and the organization of data. The most common method to insert a newline is by using the newline character, represented as `\n`. This character can be included in strings, allowing developers to format their output across multiple lines easily.
Another approach to add newlines is by using the `print()` function, which automatically adds a newline after each call unless specified otherwise. By utilizing the `end` parameter of the `print()` function, developers can customize how output is displayed, including controlling newline behavior. Additionally, using triple quotes for multi-line strings is an effective way to include newlines without the need for explicit newline characters.
Overall, understanding how to manipulate newlines in Python is essential for effective programming. It not only improves the presentation of data but also aids in debugging and logging processes. Mastering these techniques allows developers to create cleaner and more user-friendly outputs, ultimately enhancing the overall quality of their code.
Author Profile
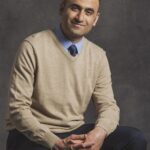
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?