How Can You Easily Add Rows to a DataFrame in Python?
Adding rows to a DataFrame in Python is a fundamental skill that can significantly enhance your data manipulation capabilities. Whether you’re working with datasets in data science, analytics, or machine learning, knowing how to efficiently manage and expand your DataFrame is crucial. As you delve into the world of data, you may find yourself needing to append new data, merge datasets, or even create entirely new rows based on existing information. This article will guide you through the essential techniques to seamlessly add rows to a DataFrame, empowering you to handle your data with confidence and precision.
In the realm of data manipulation, Python’s Pandas library stands out as a powerful tool for managing tabular data. Understanding how to add rows to a DataFrame not only allows you to expand your datasets but also enables you to perform complex data transformations and analyses. Whether you’re appending a single row, adding multiple rows at once, or integrating data from other sources, the process is both straightforward and flexible.
Throughout this article, we will explore various methods for adding rows to a DataFrame, highlighting the advantages and potential pitfalls of each approach. From using built-in functions to leveraging more advanced techniques, you’ll gain insights that will enhance your data handling skills and streamline your workflow. Prepare to unlock the full potential of your
Appending Rows to a DataFrame
One of the most common methods to add rows to a DataFrame in Python is by using the `append()` method. This method allows you to add rows from another DataFrame or from a dictionary, where the keys correspond to the column names of the DataFrame.
To use the `append()` method, you can follow this syntax:
“`python
import pandas as pd
Original DataFrame
df = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
New row to append
new_row = pd.DataFrame({‘A’: [5], ‘B’: [6]})
Appending the new row
df = df.append(new_row, ignore_index=True)
“`
It’s important to note that the `append()` method returns a new DataFrame and does not modify the original DataFrame in place unless you reassign it back.
Using `loc` to Add Rows
Another effective way to add rows is by using the `loc` indexer. This method is particularly useful when you want to add rows at specific indices or if you want to add multiple rows at once.
Here’s how you can do it:
“`python
import pandas as pd
Original DataFrame
df = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
Adding a new row using loc
df.loc[len(df)] = [5, 6]
“`
This method allows for more flexibility and can be less memory-intensive than the `append()` method, especially when adding multiple rows.
Concatenating DataFrames
The `pd.concat()` function is another powerful option for adding rows, particularly when you have multiple DataFrames to concatenate. This function can handle both vertical and horizontal concatenation.
Here’s how to concatenate two DataFrames:
“`python
import pandas as pd
Original DataFrame
df1 = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
New DataFrame to concatenate
df2 = pd.DataFrame({
‘A’: [5, 6],
‘B’: [7, 8]
})
Concatenating DataFrames
df = pd.concat([df1, df2], ignore_index=True)
“`
Creating a New DataFrame from Existing Data
In some scenarios, you may want to create a new DataFrame based on specific criteria from an existing DataFrame. This can be done using boolean indexing or filtering methods.
For instance, if you want to add rows based on a condition:
“`python
import pandas as pd
Original DataFrame
df = pd.DataFrame({
‘A’: [1, 2, 3, 4],
‘B’: [5, 6, 7, 8]
})
Filtered DataFrame based on a condition
new_rows = df[df[‘A’] > 2]
“`
This will create a new DataFrame containing only the rows where column ‘A’ has values greater than 2.
Method | Use Case | Example |
---|---|---|
append() | Adding a single row or DataFrame | df.append(new_row) |
loc | Adding rows at specific indices | df.loc[len(df)] = [5, 6] |
concat() | Concatenating multiple DataFrames | pd.concat([df1, df2]) |
Boolean Indexing | Creating new DataFrames based on conditions | df[df[‘A’] > 2] |
Methods for Adding Rows to a DataFrame
Adding rows to a DataFrame in Python, particularly using the Pandas library, can be accomplished through various methods. Each method serves different use cases, depending on the desired outcome.
Using `loc` to Append Rows
The `loc` indexer can be used to append a new row to an existing DataFrame. This method is straightforward and allows for adding a single row effectively.
“`python
import pandas as pd
Example DataFrame
df = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
Adding a new row
df.loc[len(df)] = [5, 6]
“`
- This method uses the current length of the DataFrame to find the next available index.
- Ensure that the new row’s data matches the DataFrame’s structure.
Using `append` Method
The `append()` method provides an easy way to add rows. It creates a new DataFrame by appending the specified rows.
“`python
new_row = pd.Series({‘A’: 7, ‘B’: 8})
df = df.append(new_row, ignore_index=True)
“`
- The `ignore_index` parameter resets the index for the resulting DataFrame.
- Note that `append()` is deprecated in future versions of Pandas, so consider alternatives.
Using `concat` Function
The `concat()` function is versatile for combining multiple DataFrames or adding rows.
“`python
new_rows = pd.DataFrame({‘A’: [9, 10], ‘B’: [11, 12]})
df = pd.concat([df, new_rows], ignore_index=True)
“`
- This method is efficient for adding multiple rows at once.
- It can also combine DataFrames along different axes.
Using `DataFrame.loc` with Lists
If you want to add multiple rows at once, you can use `loc` with a list of lists or a DataFrame.
“`python
additional_rows = [[13, 14], [15, 16]]
for row in additional_rows:
df.loc[len(df)] = row
“`
- This method allows for flexibility in adding multiple rows incrementally.
Performance Considerations
When adding rows to a DataFrame, performance can vary based on the method chosen. Here are some points to consider:
Method | Performance | Use Case |
---|---|---|
`loc` | Fast for few rows | Incremental additions |
`append` | Slower, deprecated | Single row addition |
`concat` | Efficient for many rows | Bulk additions |
- For extensive DataFrame operations, prefer using `concat()` to minimize overhead.
- Frequent use of `append()` can lead to performance degradation due to DataFrame copies.
Selecting the appropriate method for adding rows to a DataFrame depends on the specific requirements of the task. Consider factors such as the number of rows to add, performance implications, and future compatibility with Pandas.
Expert Insights on Adding Rows to DataFrames in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When adding rows to a DataFrame in Python, utilizing the `pandas` library is essential. The `append()` method is straightforward for adding single or multiple rows, but for larger datasets, consider using `concat()` for efficiency. This approach minimizes memory overhead and enhances performance.”
Michael Thompson (Senior Software Engineer, Data Solutions Group). “In my experience, using `loc` or `iloc` to assign values directly to a new index is a highly efficient way to add rows to a DataFrame. This method allows for dynamic row addition without the overhead of creating a new DataFrame each time, which can be crucial in real-time data processing applications.”
Sarah Patel (Machine Learning Engineer, AI Analytics Corp.). “For those dealing with large datasets, I recommend leveraging the `pd.concat()` function over `append()`, as it is more performant when combining multiple DataFrames. Additionally, always ensure that the DataFrame’s structure remains consistent to avoid complications during the merging process.”
Frequently Asked Questions (FAQs)
How can I add a single row to a DataFrame in Python?
You can add a single row to a DataFrame using the `loc` method or the `append` method. For example, `df.loc[len(df)] = [value1, value2, value3]` or `df = df.append(new_row, ignore_index=True)`.
What is the difference between `append()` and `concat()` for adding rows?
The `append()` method is used to add a single DataFrame to another, while `concat()` can combine multiple DataFrames along a particular axis. `concat()` is generally more efficient for adding multiple rows.
Can I add multiple rows to a DataFrame at once?
Yes, you can add multiple rows at once using the `concat()` function. For example, `df = pd.concat([df, new_rows], ignore_index=True)` allows you to concatenate the existing DataFrame with another DataFrame containing new rows.
Is it possible to add rows based on conditions?
Yes, you can add rows based on conditions by filtering your DataFrame and then appending the filtered data. Use boolean indexing to select the desired rows and then append them accordingly.
What happens to the index when I add rows to a DataFrame?
When adding rows, the index can be adjusted based on the method used. If you use `ignore_index=True`, the index will be reset. Otherwise, the new rows will retain their original indices unless specified otherwise.
Are there performance considerations when adding rows to a DataFrame?
Yes, adding rows in a loop using methods like `append()` can be inefficient due to the need for DataFrame reallocation. It is advisable to collect all rows in a list and then create a DataFrame or use `concat()` for better performance.
In Python, adding rows to a DataFrame can be accomplished using various methods, primarily through the Pandas library. The most common techniques include using the `append()` method, the `concat()` function, and the `loc[]` indexer. Each of these methods has its own advantages and is suited for different scenarios, depending on the requirements of the data manipulation task at hand.
The `append()` method allows for the addition of a single row or multiple rows by passing a DataFrame or a dictionary representing the new data. However, it is worth noting that `append()` is being deprecated in future versions of Pandas, which makes it less favorable for long-term use. On the other hand, the `concat()` function is a more versatile option that can efficiently combine multiple DataFrames along a specified axis, making it ideal for adding rows in bulk.
Another effective approach is using the `loc[]` indexer, which enables direct assignment to a new index. This method is particularly useful when you want to add rows iteratively or when working with existing DataFrames. It is essential to consider the performance implications of each method, especially when dealing with large datasets, as some methods may lead to inefficient memory usage and slower execution times
Author Profile
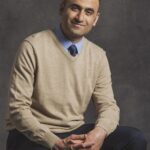
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?