How to Assert ‘Error Nil Got’ in Your Go Code: Tips and Best Practices?
In the world of programming, error handling is a crucial aspect that can make or break the robustness of an application. Among the myriad of challenges developers face, one common question arises: how to assert that an error is nil when it is expected to be? This seemingly simple task can often lead to confusion, especially for those new to coding or transitioning between languages. Understanding how to effectively manage errors not only enhances code quality but also improves the overall user experience by ensuring that applications behave as intended.
When working with languages like Go, asserting that an error is nil can be a fundamental part of control flow. Developers must learn to differentiate between expected and unexpected errors, as well as how to handle them appropriately. This involves not just checking the error value but also understanding the context in which the error may arise. By mastering this skill, programmers can write cleaner, more efficient code that gracefully handles potential pitfalls.
Moreover, the implications of asserting error values extend beyond mere syntax. It encompasses best practices in software development, such as maintaining code readability and ensuring that error handling is both consistent and logical. As we delve deeper into this topic, we will explore various techniques and strategies that can help developers confidently assert that an error is nil, equipping them with the tools necessary to write resilient applications.
Understanding Error Handling in Go
In Go, errors are a fundamental part of programming, especially when dealing with functions that can fail. When an error occurs, it’s common to check for it, and if the error is not `nil`, appropriate actions can be taken. The typical pattern for error handling involves returning both a result and an error, which allows the caller to decide how to handle the situation.
When you encounter a situation where you need to assert that an error is `nil`, it’s essential to understand the implications of this assertion. The assertion can be performed using simple conditional statements.
Checking for Errors
To assert that an error is `nil`, you can use the following pattern:
“`go
result, err := someFunction()
if err != nil {
// Handle the error
}
“`
This pattern checks if `err` is `nil`. If it is not, you can proceed to handle the error accordingly.
Here are some common practices for error handling:
- Logging the error: Always log the error to track what went wrong.
- Returning the error: If you cannot handle it at your current level, consider returning the error to the caller.
- Using a custom error type: This allows you to include more context about the error.
Assertion Techniques
When you want to assert that an error is `nil`, particularly in tests, you can use the `t.Errorf` method to report failures in a clear manner. Here’s an example of how you might structure a test function:
“`go
func TestSomeFunction(t *testing.T) {
result, err := someFunction()
if err != nil {
t.Errorf(“expected error to be nil, got: %v”, err)
}
// Additional assertions on the result can be performed here
}
“`
This approach ensures that if the error is not `nil`, the test will fail with a descriptive message.
Best Practices for Asserting Errors
When asserting that an error is `nil`, consider the following best practices:
- Provide context: When logging or returning errors, include context to help identify where the failure occurred.
- Use assertions in tests: Utilize testing frameworks to assert errors cleanly and clearly.
- Document error expectations: Clearly document when and why errors might be returned by your functions.
Method | Description |
---|---|
Check for `nil` | Use if statements to verify that the error variable is `nil`. |
Log the error | Record the error using a logging framework for later analysis. |
Return the error | Propagate the error up the call stack for handling at a higher level. |
Using these techniques and practices will enhance your error handling capabilities in Go, ensuring that you properly manage and respond to errors throughout your application.
Understanding Error Handling in Go
In Go, error handling is a critical aspect of writing robust applications. The typical pattern involves returning an error as the last return value from a function. This necessitates the caller to assert whether the error is `nil` or if it contains some information that needs to be handled.
Common Practices for Asserting Errors
When dealing with errors in Go, it is essential to perform checks to ensure that you are correctly handling potential issues. The following practices can be employed:
- Check for nil: Always check if the error returned from a function is `nil` before proceeding with further logic.
- Use the `errors.Is` and `errors.As` functions: These functions help in handling specific error types more gracefully.
Example code snippet:
“`go
result, err := someFunction()
if err != nil {
// Handle the error
log.Fatalf(“Error occurred: %v”, err)
}
// Proceed with using result
“`
Asserting Specific Errors
To assert specific errors, you can use type assertions or the `errors.As` function. This allows you to inspect the nature of the error more thoroughly.
Example:
“`go
var customErr *CustomError
if errors.As(err, &customErr) {
// Handle the specific CustomError type
fmt.Println(“Caught a custom error:”, customErr)
}
“`
Using Test Cases to Assert Error Behavior
In unit tests, you can assert that a function returns the expected error. This can be achieved using the `errors.Is` function or direct comparison. Below is an illustration:
“`go
func TestFunction(t *testing.T) {
_, err := someFunction()
if !errors.Is(err, expectedError) {
t.Fatalf(“expected error %v, got %v”, expectedError, err)
}
}
“`
Creating Custom Errors
Defining custom error types can greatly enhance error handling. By implementing the `Error()` method, you provide more context about the error.
Example of a custom error type:
“`go
type CustomError struct {
Message string
}
func (e *CustomError) Error() string {
return e.Message
}
“`
Usage in a function:
“`go
func someFunction() error {
return &CustomError{Message: “an error occurred”}
}
“`
Best Practices in Error Handling
Adhering to best practices in error handling can lead to cleaner, more maintainable code. Consider the following:
- Return errors to the caller: Functions should return errors instead of logging them internally.
- Provide context: Use wrapping with `fmt.Errorf` to add context to errors.
- Document error conditions: Clearly document what errors can be expected from a function.
Example of returning a wrapped error:
“`go
func anotherFunction() error {
err := someFunction()
if err != nil {
return fmt.Errorf(“anotherFunction failed: %w”, err)
}
return nil
}
“`
Implementing these strategies ensures that error handling in your Go applications is both effective and informative.
Expert Insights on Error Handling in Programming
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “In programming, particularly in languages like Go, asserting that an error is nil is crucial for robust error handling. It ensures that the function executed successfully before proceeding with further logic. Always check for errors immediately after a function call to maintain code integrity.”
Michael Tran (Senior Developer, CodeCraft Solutions). “When you encounter an error assertion, such as ‘error nil got’, it indicates that the expected error was not returned. This situation should prompt a thorough investigation of the function’s behavior and its return values, ensuring that all edge cases are properly handled.”
Sarah Johnson (Lead Architect, Reliable Systems). “Asserting that an error is nil is not just a best practice; it is essential for preventing runtime failures. Developers should implement comprehensive logging and testing strategies to capture unexpected behaviors, which can lead to better insights into why an error was not nil when it was anticipated.”
Frequently Asked Questions (FAQs)
What does “assert error nil got” mean in programming?
This phrase typically refers to a testing assertion that checks whether an error variable is nil. If the assertion fails, it indicates that an unexpected error occurred.
How can I use assertions to check for nil errors in Go?
In Go, you can use the `assert` package from libraries like `stretchr/testify` to verify that an error variable is nil. The syntax usually involves `assert.Nil(t, err)` where `err` is the variable being checked.
What happens if an assertion for a nil error fails?
If the assertion fails, it usually triggers a test failure, providing feedback that an error was returned when none was expected. This helps identify issues in the code.
Can I customize the error message for failed assertions?
Yes, many assertion libraries allow you to provide a custom error message that will be displayed when the assertion fails. This can help clarify the context of the failure.
Are there best practices for asserting errors in tests?
Best practices include always checking for nil errors when they are expected, using clear and descriptive assertion messages, and grouping related assertions to maintain test readability.
What is the significance of checking for nil errors in unit tests?
Checking for nil errors is crucial in unit tests as it ensures that functions behave as expected. It helps maintain code reliability and prevents unexpected behaviors in production.
In programming, particularly in languages like Go, handling errors effectively is crucial for building robust applications. The phrase “assert error nil got” typically refers to a situation where a function is expected to return an error value of nil, indicating success, but instead returns a non-nil error. This discrepancy can lead to unexpected behavior and bugs in the application. Understanding how to assert that an error is nil is essential for validating the correctness of function outputs and ensuring that error handling is implemented appropriately.
To assert that an error is nil, developers often use conditional statements to check the error value returned by a function. For instance, in Go, a common pattern involves checking if the error variable is nil immediately after a function call. If the error is not nil, developers can handle it accordingly, such as logging the error or returning it to the caller. This practice not only helps in debugging but also contributes to maintaining the integrity of the application by preventing the propagation of errors.
Key takeaways from this discussion include the importance of rigorous error checking in programming. Developers should always anticipate potential errors and implement checks to assert that they are nil when expected. Additionally, understanding the context in which errors occur can help in crafting better error messages and handling strategies.
Author Profile
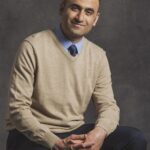
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?