How Can You Safely Store API Keys in a Docker Container?
In today’s digital landscape, the security of sensitive information is paramount, especially for developers working with APIs. API keys serve as the gatekeepers to various services, enabling applications to communicate and function effectively. However, with the rise of containerization technologies like Docker, the challenge of securely storing these keys has become increasingly complex. As developers embrace the flexibility and scalability that Docker offers, it’s crucial to adopt best practices for managing API keys to safeguard against unauthorized access and potential data breaches. This article delves into the most effective strategies for securely storing API keys within Docker containers, ensuring that your applications remain both functional and secure.
When deploying applications in Docker, the way you handle API keys can significantly impact your security posture. Traditional methods of storing sensitive data, such as hardcoding keys directly into your source code, are not only risky but also counterproductive in a containerized environment. Instead, developers must explore alternative approaches that prioritize security without compromising the ease of deployment. From leveraging environment variables to utilizing secret management tools, there are several strategies to consider that can help mitigate risks associated with API key exposure.
Moreover, understanding the implications of each storage method is essential for maintaining a secure application lifecycle. As we navigate through the best practices for storing API keys in Docker containers, we will highlight the importance
Environment Variables
Storing API keys as environment variables is one of the most common practices when working with Docker containers. This method keeps sensitive information out of your codebase and allows for easy configuration.
- How to set environment variables:
- Use the `-e` flag with the `docker run` command:
“`bash
docker run -e API_KEY=your_api_key your_image
“`
- Alternatively, you can define them in a `.env` file and use the `–env-file` option:
“`bash
docker run –env-file .env your_image
“`
This approach allows for flexibility and security as you can manage different keys for different environments (development, testing, production) without changing the code.
Docker Secrets
For applications that require a higher level of security, Docker Secrets can be utilized. This feature is available in Docker Swarm mode and allows you to manage sensitive data securely.
- Steps to use Docker Secrets:
- Create a secret:
“`bash
echo “your_api_key” | docker secret create api_key –
“`
- Deploy a service with the secret:
“`bash
docker service create –name your_service –secret api_key your_image
“`
- Access the secret inside the container:
Secrets are mounted as files in `/run/secrets/` by default. You can read the API key from this file.
This method ensures that sensitive information is not exposed in the environment variables or in the container’s image.
Docker Compose
Using Docker Compose allows for defining services, networks, and volumes in a single file, making it easier to manage configuration.
- Example of using environment variables in `docker-compose.yml`:
“`yaml
version: ‘3.8’
services:
app:
image: your_image
environment:
- API_KEY=${API_KEY}
env_file:
- .env
“`
- Using Docker Secrets in Docker Compose:
“`yaml
version: ‘3.8’
services:
app:
image: your_image
secrets:
- api_key
secrets:
api_key:
external: true
“`
This enables you to keep API keys outside the codebase while still allowing easy deployment and configuration management.
Best Practices
To enhance security and maintainability when storing API keys in Docker containers, consider the following best practices:
- Do not hard-code keys: Avoid placing API keys directly in your application code or Dockerfile.
- Use a dedicated configuration file: Store sensitive information in a separate, secure configuration file that is not included in version control.
- Limit access: Ensure that only necessary services have access to the API keys. Use Docker’s role-based access control (RBAC) features.
- Rotate keys regularly: Regularly update and rotate your API keys to minimize the risk of exposure.
Method | Pros | Cons |
---|---|---|
Environment Variables | Simple, easy to configure | Potentially visible to all processes in the container |
Docker Secrets | Enhanced security, not visible in environment variables | Requires Docker Swarm |
Docker Compose | Structured configuration, easy management | Still may expose variables if not configured correctly |
Implementing these methods and adhering to best practices can help secure API keys effectively in Docker environments.
Environment Variables
Storing API keys as environment variables is a common approach due to its simplicity and security. Docker allows you to set environment variables in several ways:
- Dockerfile: Use the `ENV` instruction to define variables.
- Docker Compose: Define environment variables in the `docker-compose.yml` file.
- Runtime: Pass environment variables at runtime using the `-e` flag.
Example of defining an environment variable in Docker Compose:
“`yaml
version: ‘3.8’
services:
app:
image: your-image
environment:
- API_KEY=${API_KEY}
“`
This approach allows you to keep sensitive data outside your codebase. Use a `.env` file to manage environment variables locally, ensuring it is included in your `.gitignore`.
Secrets Management with Docker Swarm
For applications running in Docker Swarm, utilizing Docker Secrets provides a robust mechanism for handling sensitive data such as API keys. This method encrypts secrets and only makes them available to the services that need them.
- Create a secret:
“`bash
echo “your_api_key” | docker secret create api_key –
“`
- Use the secret in your service:
“`yaml
version: ‘3.8’
services:
app:
image: your-image
secrets:
- api_key
secrets:
api_key:
external: true
“`
Secrets are stored securely and are not exposed in the container’s environment, making this a highly recommended approach for production environments.
Docker Volume for Configuration Files
Another method is to store API keys in configuration files located on a Docker volume. This allows for easier management and updating of keys without needing to rebuild your container.
- Create a configuration file:
Store your API keys in a `.env` or a custom configuration file.
- Mount the volume:
Use Docker volumes to mount the directory containing your configuration file into the container.
Example in `docker-compose.yml`:
“`yaml
version: ‘3.8’
services:
app:
image: your-image
volumes:
- ./config:/app/config
“`
Ensure that sensitive files are secured and not included in source control.
Using Third-party Secret Management Tools
Integrating third-party secret management tools can enhance security practices when handling API keys in Docker containers. Consider the following options:
- HashiCorp Vault: Provides secure storage and tight access control for secrets.
- AWS Secrets Manager: Ideal for applications running on AWS, allowing you to retrieve secrets programmatically.
- Azure Key Vault: For Azure-hosted applications, providing secure management of API keys.
These tools offer advanced features such as automatic rotation of secrets, which can significantly reduce the risk of key exposure.
Tool | Key Features |
---|---|
HashiCorp Vault | Dynamic secrets, access policies, audit logging |
AWS Secrets Manager | Automatic rotation, fine-grained access control |
Azure Key Vault | Integration with Azure services, RBAC management |
Best Practices for API Key Management
When managing API keys within Docker containers, adhere to the following best practices:
- Limit Scope: Use API keys with the least privileges necessary.
- Rotate Keys Regularly: Implement a schedule for rotating keys to minimize exposure risk.
- Monitor Usage: Keep track of API key usage and set up alerts for unusual activity.
- Avoid Hardcoding: Never hardcode API keys in your application code or Dockerfiles.
- Secure Your Environment: Ensure that your Docker host and containers are properly secured against unauthorized access.
By following these practices, you can significantly enhance the security of your API keys when using Docker containers.
Best Practices for Storing API Keys in Docker Containers
Emily Carter (DevOps Engineer, CloudSecure Solutions). “The most secure way to store API keys in a Docker container is to utilize Docker secrets. This feature allows you to manage sensitive data separately from your application code, ensuring that your keys are encrypted and only accessible to the services that need them.”
James Lin (Security Architect, CyberShield Inc.). “Environment variables are commonly used for storing API keys in Docker containers, but they can be exposed through various means. I recommend using a combination of environment variables and a secrets management tool like HashiCorp Vault to enhance security and manage access more effectively.”
Sarah Thompson (Cloud Solutions Consultant, TechSavvy Group). “When deploying applications in Docker, avoid hardcoding API keys directly into your Dockerfiles. Instead, leverage external configuration files or environment variable files that are not included in your version control system, ensuring that sensitive information remains protected.”
Frequently Asked Questions (FAQs)
What are the best practices for storing API keys in a Docker container?
Store API keys as environment variables or in a secure secrets management tool. Avoid hardcoding keys in your application code or Dockerfile to enhance security.
Can I use Docker secrets to manage API keys securely?
Yes, Docker secrets are designed for managing sensitive data. They provide a secure way to store and access API keys without exposing them in the container environment.
How do I set environment variables for API keys in a Docker container?
You can set environment variables using the `-e` flag in the `docker run` command or by specifying them in a Docker Compose file under the `environment` section.
Is it safe to use a `.env` file for storing API keys in Docker?
Using a `.env` file can be safe if properly secured. Ensure the file is included in your `.gitignore` to prevent accidental exposure in version control.
What should I avoid when storing API keys in Docker containers?
Avoid hardcoding API keys in your source code or Docker images. Also, refrain from using publicly accessible storage solutions that could expose your keys.
How can I rotate API keys in a Docker environment?
To rotate API keys, update the keys in your secrets management tool or environment variables, then restart your Docker containers to apply the changes without downtime.
Storing API keys securely within a Docker container is a critical aspect of maintaining the integrity and security of applications. It is essential to avoid hardcoding sensitive information directly into the application code or Docker images, as this can expose the keys to unauthorized access. Instead, leveraging environment variables, Docker secrets, or external configuration management tools can provide a more secure approach to managing API keys.
Utilizing environment variables is one of the simplest methods for storing API keys in Docker. By defining these variables in a `.env` file or directly in the Docker Compose file, developers can ensure that sensitive information is not embedded in the source code. However, it is crucial to manage access to these files and avoid including them in version control systems.
For enhanced security, Docker secrets offer a more robust solution, especially in a Swarm mode environment. Docker secrets allow for the secure storage and management of sensitive data, which can be accessed only by specific services. This method minimizes the risk of exposure and provides better control over who can access the API keys. Additionally, integrating external tools such as HashiCorp Vault or AWS Secrets Manager can further streamline the management of secrets across multiple environments.
the best practices for storing API keys in
Author Profile
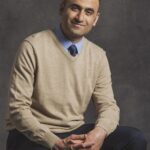
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?