How Can You Make Text Bold in Python?
In the world of programming, the ability to manipulate text formatting can significantly enhance the readability and presentation of your output. Whether you’re creating a command-line application, generating reports, or developing a web application, knowing how to bold text in Python can add a professional touch to your work. This simple yet powerful formatting technique can help emphasize important information, making it stand out to users and stakeholders alike.
Python, with its rich ecosystem of libraries and frameworks, offers various ways to achieve bold text formatting, depending on the context in which you’re working. From console applications to web development, each environment provides unique methods to highlight your text. For instance, when working in a terminal, you might utilize ANSI escape codes to change the appearance of your output. Alternatively, if you’re developing a web application using frameworks like Flask or Django, you might leverage HTML and CSS to style your text effectively.
As you delve deeper into the topic, you’ll discover the various approaches and tools available for bolding text in Python, allowing you to choose the method that best suits your project. Whether you’re a beginner looking to enhance your skills or an experienced developer seeking to refine your text formatting techniques, understanding how to bold in Python is a valuable asset that can elevate your programming game.
Using ANSI Escape Codes
In terminal applications, you can achieve bold text by utilizing ANSI escape codes. These codes allow you to format text in different styles, including bold.
To make text bold in a terminal, you can use the following escape sequence:
“`python
print(“\033[1mThis text is bold\033[0m”)
“`
In this example:
- `\033[1m` initiates bold text.
- `\033[0m` resets the formatting back to normal.
Here is a brief overview of common ANSI codes:
Code | Description |
---|---|
`\033[0m` | Reset |
`\033[1m` | Bold |
`\033[2m` | Dim |
`\033[3m` | Italic |
`\033[4m` | Underline |
Using Rich Library
For more advanced text formatting, consider using the `rich` library, which simplifies the process of styling text in Python applications. First, ensure that the library is installed:
“`bash
pip install rich
“`
You can then use it as follows:
“`python
from rich.console import Console
console = Console()
console.print(“This text is bold”, style=”bold”)
“`
The `rich` library supports various styles and allows for more complex formatting, making it a preferred choice for applications that require enhanced text presentation.
Formatting in Jupyter Notebooks
In Jupyter Notebooks, you can use Markdown syntax to bold text. To bold text in a Markdown cell, simply wrap the text in double asterisks or double underscores. For example:
“`markdown
This text is bold
“`
or
“`markdown
__This text is bold__
“`
When you run the cell, it will render the text as bold.
Using HTML in Python
If you’re generating HTML content, you can easily create bold text using HTML tags. For example:
“`python
html_content = “This text is bold”
“`
When rendered in a web browser, the text will appear in bold. This approach is particularly useful when working with web frameworks like Flask or Django, where HTML content is generated dynamically.
In Python, achieving bold text can be accomplished through various methods depending on the context, such as terminal applications, Jupyter Notebooks, or web development. Each approach has its specific syntax and use cases, offering flexibility for developers to present text effectively.
Using Bold Text in Python
In Python, the method for displaying bold text depends on the context in which you are working. Below are various ways to achieve bold text formatting in different scenarios.
Bold Text in Console Output
When working in a console or terminal, you can use ANSI escape codes to format text. For bold text, the escape code is `\033[1m` to start and `\033[0m` to reset.
Example:
“`python
print(“\033[1mThis is bold text\033[0m”)
“`
Bold Text in GUI Applications
For GUI applications, libraries such as Tkinter or PyQt can be used to render bold text.
Tkinter Example:
“`python
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text=”This is bold text”, font=(“Helvetica”, 16, “bold”))
label.pack()
root.mainloop()
“`
PyQt Example:
“`python
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel(“This is bold text”)
label.setStyleSheet(“font-weight: bold;”)
label.show()
app.exec_()
“`
Bold Text in Web Applications
When generating HTML content in a web application, you can use the `` or `` tags for bold text.
Example using Flask:
“`python
from flask import Flask, render_template_string
app = Flask(__name__)
@app.route(‘/’)
def home():
return render_template_string(“
This is bold text
“)
if __name__ == ‘__main__’:
app.run()
“`
Bold Text in Markdown
If you are working with Markdown (for example, in Jupyter Notebooks), you can use double asterisks or double underscores for bold formatting.
Example:
“`markdown
This is bold text
“`
or
“`markdown
__This is bold text__
“`
Bold Text in Reports (Using Libraries)
Libraries such as Matplotlib and ReportLab allow you to create reports with bold text.
Matplotlib Example:
“`python
import matplotlib.pyplot as plt
plt.title(“This is bold title”, fontweight=’bold’)
plt.xlabel(“X-axis”, fontweight=’bold’)
plt.ylabel(“Y-axis”, fontweight=’bold’)
plt.show()
“`
ReportLab Example:
“`python
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
c = canvas.Canvas(“example.pdf”, pagesize=letter)
c.setFont(“Helvetica-Bold”, 12)
c.drawString(100, 750, “This is bold text”)
c.save()
“`
Common Use Cases
Context | Method of Bold Formatting |
---|---|
Console Output | ANSI escape codes |
GUI Applications | Tkinter, PyQt |
Web Applications | HTML tags (``, ``) |
Markdown | Double asterisks or underscores |
Reports | Matplotlib, ReportLab |
By employing the appropriate method for bold text based on your specific application context, you can enhance the visibility and emphasis of your messages effectively.
Expert Insights on Formatting Text in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To bold text in Python, particularly when working with libraries like Matplotlib or Jupyter Notebooks, you can use Markdown syntax or specific formatting functions provided by these libraries. For example, in Jupyter, wrapping text in double asterisks ‘**’ or using HTML tags like ‘‘ will render the text in bold.”
Michael Chen (Lead Developer, PyTech Innovations). “In Python, the concept of ‘bolding’ text is often context-dependent. For terminal outputs, using ANSI escape codes can achieve bold text. However, in GUI applications like Tkinter, you would set the font weight to ‘bold’ when creating text elements.”
Sarah Thompson (Data Visualization Expert, Visualize It Inc.). “When creating visualizations in Python, libraries such as Seaborn and Matplotlib allow you to customize the appearance of text, including making it bold. This can be done by specifying the font weight in the text properties, enhancing the readability of your charts and graphs.”
Frequently Asked Questions (FAQs)
How can I make text bold in a Python console?
You can use ANSI escape codes to format text in the console. For example, `print(“\033[1mThis text is bold\033[0m”)` will display “This text is bold” in bold.
Is there a way to bold text in Jupyter notebooks?
In Jupyter notebooks, you can use Markdown. To make text bold, wrap the text in double asterisks or double underscores, like `This text is bold` or `__This text is bold__`.
Can I bold text in a Python GUI application?
Yes, in GUI frameworks like Tkinter, you can set the font weight to bold. For example, use `font=(“Helvetica”, 12, “bold”)` when creating a label.
How do I bold text in a web application using Python?
In web applications using frameworks like Flask or Django, you can use HTML tags. For instance, use `This text is bold` within your templates.
Are there libraries in Python that support rich text formatting?
Yes, libraries like Rich and PrettyTable allow for advanced formatting, including bold text. For example, `from rich.console import Console; console = Console(); console.print(“This is bold”, style=”bold”)` will print bold text.
Can I bold text in Python’s output files like CSV or TXT?
Text files such as CSV or TXT do not support formatting like bold. However, you can create formatted documents using libraries like ReportLab or Python-docx for PDFs and Word documents, respectively.
In Python, the concept of “bold” can vary based on the context in which it is applied. When dealing with text output in the console, Python does not natively support text formatting such as bold. However, developers can achieve bold text effects in various ways, depending on the environment. For example, when using terminal emulators that support ANSI escape codes, one can use specific codes to format text as bold. Additionally, libraries like `rich` or `colorama` can be utilized to enhance console output with bold and other styles.
In graphical user interface (GUI) applications, such as those created with Tkinter or PyQt, bold text can be easily implemented by specifying font properties. These libraries allow developers to set font weights to bold when creating labels, buttons, or other text elements. Furthermore, when generating HTML content, using the `` or `` tags will render text in bold when displayed in a web browser.
In summary, while Python does not provide a direct method for bolding text in all contexts, there are multiple approaches available depending on the output medium. Understanding the environment and the tools at your disposal is crucial for effectively formatting text. Utilizing libraries and frameworks that support text styling can significantly
Author Profile
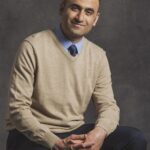
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?