How Can You Effectively Break Out of a While Loop?
How to Break a While Loop: Mastering Control Flow in Programming
In the world of programming, control flow is a fundamental concept that dictates how a program executes its instructions. Among the various control structures, the while loop stands out as a powerful tool for repetitive tasks. However, there are times when you may need to break free from the confines of a while loop, whether due to meeting a specific condition, encountering an error, or simply realizing that the task at hand has changed. Understanding how to effectively break a while loop is essential for writing efficient and clean code, allowing developers to manage their programs with precision and flexibility.
Breaking a while loop can be achieved through various techniques, each suited to different scenarios and programming languages. The most common method involves using a break statement, which immediately exits the loop when a certain condition is met. This not only enhances code readability but also improves performance by preventing unnecessary iterations. Additionally, some programming languages offer alternative mechanisms, such as flags or exceptions, that can be employed to exit a loop gracefully.
As you delve deeper into the intricacies of while loops and their control mechanisms, you’ll discover the importance of structuring your code to avoid infinite loops and ensure that your programs run smoothly. Mastering the art of breaking while loops will empower you
Understanding Loop Control Statements
In programming, a while loop continues to execute a block of code as long as a specified condition remains true. However, there are scenarios where it is necessary to exit the loop prematurely. This can be accomplished using loop control statements, which enhance the flexibility of your code.
The primary control statements used to break out of a while loop are `break` and `return`. Understanding how to implement these statements effectively is crucial for managing loop execution.
Using the Break Statement
The `break` statement is a powerful tool that allows a programmer to exit a while loop immediately. When executed, it transfers control to the statement following the loop.
Syntax:
“`python
while condition:
Code block
if some_condition:
break
“`
This example illustrates the basic usage of the `break` statement. The loop will terminate as soon as `some_condition` evaluates to true, regardless of the original loop condition.
Example:
“`python
count = 0
while count < 10:
print(count)
count += 1
if count == 5:
break
```
In this example, the loop will print numbers from 0 to 4 and then stop when `count` reaches 5.
Using the Return Statement
The `return` statement can also be utilized to exit a while loop, especially within a function. When a return statement is executed, it exits not only the loop but also the entire function.
Syntax:
“`python
def example_function():
while condition:
Code block
if some_condition:
return
“`
Example:
“`python
def count_to_ten():
count = 0
while count < 10:
print(count)
count += 1
if count == 5:
return
```
In this case, calling `count_to_ten()` will output numbers from 0 to 4 and then exit the function entirely.
Conditional Logic for Loop Control
Proper use of conditional logic can significantly enhance loop control. Here are some common strategies:
- Flag Variables: Use a boolean flag to control loop execution based on certain conditions.
- Nested Loops: Combine multiple loops and use a break statement in an inner loop to affect an outer loop.
- Exception Handling: Utilize try-except blocks to manage errors that may necessitate breaking out of a loop.
Table: Comparison of Control Statements
Control Statement | Exits | Use Case |
---|---|---|
break | Only the loop | Immediate exit based on condition |
return | Entire function | Exiting a loop when performing a function |
Understanding these mechanisms allows programmers to write more efficient and maintainable code, enabling them to manage loop execution dynamically based on runtime conditions.
Understanding the While Loop
A while loop is a fundamental control structure in programming that executes a block of code as long as a specified condition evaluates to true. Breaking out of a while loop can be essential for managing program flow, particularly when the loop must terminate based on dynamic conditions.
Methods to Break a While Loop
There are several methods to effectively break out of a while loop, depending on the programming language being used and the specific requirements of your application. Below are the most common techniques:
Using the Break Statement
The `break` statement is a direct way to exit a while loop prematurely. It can be used when a certain condition is met within the loop. Here’s how it generally works:
- Syntax Example (in Python):
“`python
while condition:
if some_condition:
break Exit the loop
Other code
“`
- Key Points:
- The `break` statement immediately terminates the loop.
- Control passes to the next statement following the loop.
Using a Flag Variable
A flag variable can be employed to control the execution of the loop. This method is particularly useful when multiple conditions can lead to breaking the loop.
- Example:
“`python
flag = True
while flag:
if some_condition:
flag = Change the flag to exit
Other code
“`
- Advantages:
- Provides clear control over loop termination.
- Can be used to manage complex loop exit conditions.
Conditional Check in the Loop
Implementing a conditional check at the beginning of the loop can effectively manage when to exit. This is often done by modifying the loop condition itself.
- Example:
“`python
i = 0
while i < 10:
if i == 5:
break Exit when i equals 5
i += 1
```
- Considerations:
- Ensure the condition is updated correctly to avoid infinite loops.
- This method is straightforward and easy to implement.
Using Return Statement in Functions
In scenarios where a while loop is encapsulated within a function, the `return` statement can be utilized to exit both the loop and the function simultaneously.
- Example:
“`python
def example_function():
while True:
if some_condition:
return Exit the loop and function
Other code
“`
- Usage:
- This is effective for functions that require immediate termination based on specific conditions.
- It enhances code readability by clearly indicating an exit point.
Practical Considerations
When breaking out of a while loop, it is crucial to consider the following:
- Avoid Infinite Loops: Ensure that your loop has a clear exit strategy.
- Maintain Code Readability: Use comments or clear variable names to indicate the purpose of breaking the loop.
- Testing: Thoroughly test the loop exit conditions to ensure reliability and correctness in various scenarios.
Implementing effective strategies to break a while loop enhances control over program execution. By employing methods such as the break statement, flag variables, conditional checks, and return statements, developers can create robust and maintainable code.
Expert Insights on Breaking While Loops in Programming
Dr. Emily Carter (Computer Science Professor, Tech University). “Breaking a while loop effectively requires a clear understanding of the loop’s exit conditions. It is crucial to ensure that the break statement is placed within the loop’s body and is triggered by a specific condition to avoid infinite loops.”
James Liu (Senior Software Engineer, CodeCraft Solutions). “Utilizing flags or counters can significantly enhance control over while loops. By implementing a boolean flag that changes state based on certain criteria, developers can break out of the loop gracefully and maintain code readability.”
Maria Gonzalez (Lead Developer, Innovative Tech Corp). “It is essential to document the reasons for breaking a while loop within the code. This practice not only aids in debugging but also improves collaboration among team members by clarifying the logic behind loop termination.”
Frequently Asked Questions (FAQs)
How can I break out of a while loop in Python?
You can use the `break` statement to exit a while loop in Python. When the `break` statement is executed, the loop terminates immediately, and control is transferred to the statement following the loop.
What conditions can I use to break a while loop?
You can use any boolean expression as a condition to break a while loop. This typically involves checking a variable’s value or state within the loop, allowing you to terminate the loop when specific criteria are met.
Can I use multiple break statements in a while loop?
Yes, you can use multiple `break` statements within a while loop. Each `break` can be conditional, allowing for different exit points based on various conditions evaluated during the loop’s execution.
Is there an alternative to using break in a while loop?
Yes, you can control the loop’s execution by modifying the loop condition itself. By setting the condition to “ when you want to exit, you can avoid using the `break` statement altogether.
How does a break statement affect nested while loops?
A `break` statement will only exit the innermost loop in which it is called. If you have nested while loops, you may need to use additional logic or flags to exit outer loops if necessary.
Are there any performance implications of using break in a while loop?
Using a `break` statement generally does not have significant performance implications. However, excessive or unnecessary use of `break` can lead to less readable code, which may complicate maintenance and debugging efforts.
In programming, breaking out of a while loop is a fundamental concept that allows developers to control the flow of their code effectively. A while loop continues to execute as long as a specified condition remains true. However, there are scenarios where it becomes necessary to exit the loop prematurely. This can be achieved using various methods, with the most common being the `break` statement, which immediately terminates the loop regardless of the condition.
Additionally, other techniques can be employed to manage loop execution. For instance, modifying the loop’s condition within the loop body can lead to a natural termination of the loop. Furthermore, using flags or counters to monitor the loop’s state can provide more granular control over when to exit the loop. These strategies enhance code readability and maintainability, allowing developers to write more efficient and understandable loops.
Ultimately, understanding how to effectively break a while loop is crucial for any programmer. It not only aids in preventing infinite loops but also contributes to better performance and resource management in applications. By mastering these techniques, developers can ensure their code runs smoothly and meets the intended logic without unnecessary delays or complications.
Author Profile
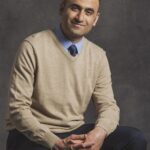
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?