How Can You Build a Website Using Python?
In today’s digital age, building a website has become an essential skill for individuals and businesses alike. With a myriad of tools and platforms available, it can be overwhelming to choose the right approach. Enter Python—a versatile programming language that has gained immense popularity not just for data science and automation, but also for web development. Whether you’re a seasoned coder or a curious beginner, learning how to build a website using Python can unlock a world of possibilities, enabling you to create dynamic, interactive web applications that cater to your unique needs.
At its core, web development with Python involves leveraging powerful frameworks and libraries that simplify the process of creating robust websites. From Flask, which offers a lightweight option for small projects, to Django, a comprehensive framework that supports complex applications, Python provides a range of tools tailored to different project requirements. This flexibility allows developers to focus on crafting innovative solutions without getting bogged down by the intricacies of web technologies.
Moreover, Python’s readability and straightforward syntax make it an ideal choice for newcomers to programming. As you embark on your journey to build a website, you’ll discover how Python not only enhances your coding skills but also empowers you to bring your creative visions to life. Whether you’re looking to develop a personal blog, an e-commerce platform, or a portfolio site, understanding
Choosing a Framework
When building a website using Python, selecting the right framework is crucial, as it can significantly affect the development process and the final product. Two of the most popular frameworks are Django and Flask.
Django is a high-level framework that promotes rapid development and clean, pragmatic design. It includes a wide array of built-in features, such as an ORM (Object-Relational Mapping), authentication, and an admin panel, which can accelerate the development process.
Flask, on the other hand, is a micro-framework that is lightweight and modular. It provides the essentials to get a web application running but allows developers to choose additional libraries and tools as needed, offering more flexibility.
Comparison Table
Feature | Django | Flask |
---|---|---|
Type | Full-stack | Micro-framework |
Built-in Admin Panel | Yes | No |
ORM | Yes | No (requires additional libraries) |
Flexibility | Less flexible | Highly flexible |
Learning Curve | Steeper | Gentler |
Setting Up Your Development Environment
To start building your website, you need to set up a development environment. This involves installing Python, a suitable framework, and any other necessary tools. Follow these steps:
- Install Python: Ensure you have the latest version of Python installed on your system.
- Create a Virtual Environment: This helps manage dependencies for your project:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
“`
- Install Your Framework: Depending on the chosen framework, run:
- For Django:
“`bash
pip install django
“`
- For Flask:
“`bash
pip install flask
“`
- Set Up a Code Editor: Choose a code editor or IDE like Visual Studio Code, PyCharm, or Atom, which can enhance your coding experience with features such as syntax highlighting and debugging tools.
Creating Your First Project
Once your environment is set up, you can create your first project. The steps vary slightly between Django and Flask.
For Django:
- Create a new project:
“`bash
django-admin startproject myproject
“`
- Navigate to your project directory:
“`bash
cd myproject
“`
- Start the development server:
“`bash
python manage.py runserver
“`
For Flask:
- Create a new directory for your project and navigate into it:
“`bash
mkdir myflaskapp
cd myflaskapp
“`
- Create a new file named `app.py` and add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run your application:
“`bash
python app.py
“`
This will allow you to see “Hello, World!” when accessing the root URL in your browser.
Designing Your Web Pages
After establishing a basic application, you can begin designing your web pages. Both Django and Flask support templating systems that allow you to create dynamic HTML content.
- Django uses the Django Template Language, which makes it easy to embed Python-like expressions within HTML.
- Flask utilizes Jinja2, which provides a similar functionality and is known for its simplicity and power.
Example of a Basic Template in Flask:
“`html
{{ title }}
{{ message }}
“`
Incorporating these templates into your application will enhance user experience and provide a professional look to your website.
Choosing the Right Framework
When building a website using Python, selecting the right framework is crucial. The most commonly used frameworks include:
- Django: A high-level web framework that encourages rapid development and clean, pragmatic design. It comes with an ORM, an admin panel, and built-in security features.
- Flask: A micro-framework that is lightweight and easy to get started with, ideal for smaller applications or if you prefer to add components as needed.
- FastAPI: A modern framework designed for building APIs with high performance, leveraging Python type hints to validate input data automatically.
Framework | Pros | Cons |
---|---|---|
Django | Fully featured, scalable, great for large projects | Can be complex for small projects |
Flask | Simple, flexible, good for prototyping | Requires more setup for larger applications |
FastAPI | High performance, automatic data validation | Less mature ecosystem compared to Django |
Setting Up Your Development Environment
To build a website, you must first set up your development environment. Follow these steps:
- Install Python: Ensure you have Python 3.6 or higher installed. You can download it from the official Python website.
- Set up a Virtual Environment:
- Create a virtual environment to manage dependencies:
“`bash
python -m venv myenv
“`
- Activate the environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Required Packages: Use pip to install the framework you chose. For example, for Django:
“`bash
pip install django
“`
Creating Your First Project
After setting up your environment, you can create your first project. This process varies slightly depending on the framework.
- Django:
- Create a new project:
“`bash
django-admin startproject myproject
“`
- Navigate to the project directory:
“`bash
cd myproject
“`
- Start the development server:
“`bash
python manage.py runserver
“`
- Flask:
- Create a new file `app.py` and add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the application:
“`bash
python app.py
“`
- FastAPI:
- Create `main.py` and add the following code:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
def read_root():
return {“Hello”: “World”}
“`
- Run the application using Uvicorn:
“`bash
uvicorn main:app –reload
“`
Building Your Website Structure
With your project up and running, you can start building the website structure. This typically involves setting up routes, creating templates, and connecting to a database.
- Define Routes: Use the framework’s routing capabilities to define URL endpoints.
- Create Templates: Use HTML with templating engines like Jinja2 (Flask, FastAPI) or Django’s template system to render dynamic content.
- Database Integration: Use ORM tools like Django ORM or SQLAlchemy with Flask and FastAPI to interact with your database.
Deploying Your Website
Once your website is ready, you need to deploy it. Common deployment methods include:
- Using Cloud Providers: Platforms like Heroku, AWS, and DigitalOcean allow easy deployment.
- Containerization: Docker can be used to create containers for your application, making it easier to manage dependencies and deployment.
- Static Hosting: For static sites, services like Netlify or GitHub Pages can be used.
Deployment Option | Pros | Cons |
---|---|---|
Cloud Providers | Scalable, easy to manage | Costs can add up |
Containerization | Consistent environments | Learning curve |
Static Hosting | Free for static sites | Limited interactivity |
Expert Insights on Building a Website Using Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Building a website using Python can be a rewarding experience, especially with frameworks like Django and Flask. These tools not only streamline the development process but also enhance security and scalability, making them ideal for both beginners and experienced developers.”
Michael Chen (Web Development Instructor, Code Academy). “For those new to web development, Python offers a gentle learning curve. Utilizing libraries such as Flask for smaller applications or Django for larger projects allows developers to focus on building functionality without getting bogged down by complex configurations.”
Sarah Thompson (Lead Developer, Web Solutions Group). “When constructing a website with Python, it is essential to understand the importance of RESTful APIs and database integration. Leveraging Python’s ORM capabilities can significantly simplify data management, allowing developers to create robust web applications efficiently.”
Frequently Asked Questions (FAQs)
What are the basic requirements to build a website using Python?
To build a website using Python, you need a basic understanding of Python programming, knowledge of web frameworks like Flask or Django, and familiarity with HTML, CSS, and JavaScript. Additionally, a code editor and a local development environment are essential.
Which Python web framework is best for beginners?
Flask is often recommended for beginners due to its simplicity and minimalistic approach. It allows developers to start small and gradually add features as needed. Django is another option, offering more built-in features but with a steeper learning curve.
How do I set up a local development environment for Python web development?
To set up a local development environment, install Python on your machine, choose a web framework (like Flask or Django), and set up a virtual environment using `venv` or `virtualenv`. This isolates project dependencies and avoids conflicts with other projects.
Can I use Python for front-end development?
While Python is primarily used for back-end development, you can use frameworks like Brython or Transcrypt to write Python code that compiles to JavaScript for front-end development. However, traditional front-end languages like HTML, CSS, and JavaScript are more commonly used.
How do I deploy a Python web application?
To deploy a Python web application, choose a hosting service that supports Python, such as Heroku, AWS, or DigitalOcean. After setting up your environment on the server, you can use tools like Git for version control and a WSGI server like Gunicorn to serve your application.
What are some common challenges when building a website with Python?
Common challenges include managing dependencies, ensuring security, optimizing performance, and debugging issues. Additionally, understanding the differences between synchronous and asynchronous programming can be crucial for building scalable applications.
Building a website using Python involves several key steps that leverage the language’s versatility and powerful frameworks. Initially, it is essential to choose the right web framework, with popular options including Flask and Django. Flask is lightweight and ideal for smaller applications, while Django offers a more comprehensive solution with built-in features for larger projects. Understanding the framework’s structure and how to set up the development environment is crucial for a smooth development process.
Once the framework is selected, the next step is to design the website’s architecture. This includes defining the models, views, and templates that will dictate how data is handled and presented. Utilizing Python’s object-oriented capabilities can enhance code organization and reusability. Additionally, integrating front-end technologies such as HTML, CSS, and JavaScript is vital to create a visually appealing and interactive user experience.
Testing and deployment are the final stages in building a website with Python. It is important to conduct thorough testing to identify and resolve any bugs before launching. For deployment, various platforms such as Heroku, AWS, or DigitalOcean can be utilized to host the application. Understanding how to manage databases and server configurations is also essential for maintaining the website post-launch.
In summary, building a website using Python
Author Profile
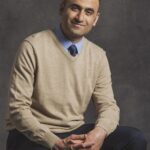
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?