How Can You Build a Website Using Python? A Step-by-Step Guide
### Introduction
In today’s digital age, having a strong online presence is essential for individuals and businesses alike. Whether you’re a budding entrepreneur, a creative artist, or simply someone looking to share your passions, building a website can open up a world of opportunities. While there are countless tools and platforms available to create a website, leveraging the power of Python can offer unparalleled flexibility and control. If you’ve ever wondered how to harness this versatile programming language to craft your own website, you’re in the right place.
Building a website with Python not only allows you to customize every aspect of your site but also equips you with valuable programming skills that can enhance your career prospects. From simple personal blogs to complex web applications, Python’s robust frameworks like Flask and Django provide the tools needed to bring your vision to life. In this article, we will explore the fundamental concepts of web development with Python, highlighting the benefits of using this language and the steps you need to take to get started.
As we delve deeper into the world of Python web development, you’ll discover the essential components that make up a website, from setting up a server to managing databases and deploying your project online. Whether you’re a complete novice or someone with a bit of coding experience, this guide will equip you with
Choosing a Framework
When building a website with Python, selecting the right framework is critical. Two of the most popular frameworks are Flask and Django, each catering to different project needs.
Flask is a micro-framework, ideal for small to medium-sized applications. It provides the essentials, allowing for flexibility and scalability through extensions. Django, on the other hand, is a high-level framework that follows the “batteries-included” philosophy, providing a plethora of built-in features, which is suitable for larger applications.
Comparison of Flask and Django:
Feature | Flask | Django |
---|---|---|
Type | Micro-framework | Full-stack framework |
Flexibility | Highly flexible, minimal structure | Structured, opinionated |
Built-in Features | Minimal | Rich set of features |
Learning Curve | Gentler | Steeper |
Use Case | Small to medium apps | Large, complex apps |
Setting Up Your Development Environment
Establishing a proper development environment is crucial for a smooth development process. Here are the necessary steps:
- Install Python: Ensure Python is installed on your machine. You can download it from the official Python website.
- Create a Virtual Environment: This step is essential for managing dependencies. You can create a virtual environment using the following command:
bash
python -m venv myenv
- Activate the Virtual Environment:
- On Windows:
bash
myenv\Scripts\activate
- On macOS/Linux:
bash
source myenv/bin/activate
- Install Required Packages: Depending on your chosen framework, install the necessary packages using pip. For Flask, you might use:
bash
pip install Flask
For Django:
bash
pip install Django
Building Your Application
After setting up your environment, you can start developing your application. The process slightly differs based on the chosen framework.
For Flask:
- Create a new file, `app.py`, and add the following code:
python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == ‘__main__’:
app.run(debug=True)
For Django:
- Start a new project with:
bash
django-admin startproject myproject
- Navigate into your project directory and run:
bash
python manage.py runserver
- This creates a basic Django project and starts the development server.
Implementing Frontend Technologies
Integrating frontend technologies with your Python backend is essential for a complete web experience. You can use HTML, CSS, and JavaScript alongside your Python framework.
- HTML: Structure your web pages.
- CSS: Style your pages for better aesthetics.
- JavaScript: Add interactivity to your site.
Consider using templating engines such as Jinja2 with Flask or Django’s built-in templating system to generate dynamic HTML pages.
Key Points to Remember:
- Keep your frontend and backend code organized.
- Use version control, like Git, to manage your codebase effectively.
- Always test your application locally before deploying it to production.
Choosing the Right Framework
When building a website with Python, selecting an appropriate web framework is crucial. The choice of framework impacts development speed, scalability, and ease of maintenance. Here are some popular frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with built-in features like an ORM, authentication, and an admin panel.
- Flask: A micro-framework that is lightweight and flexible, perfect for small to medium applications. It provides essential features and allows you to add extensions as needed.
- FastAPI: A modern framework focused on building APIs quickly and efficiently. It supports asynchronous programming and offers automatic generation of OpenAPI documentation.
Framework | Use Case | Key Features |
---|---|---|
Django | Full-fledged web applications | Built-in admin panel, ORM, authentication |
Flask | Microservices, small apps | Lightweight, extensible, easy to learn |
FastAPI | APIs, microservices | Asynchronous support, auto-doc generation |
Setting Up Your Development Environment
Establishing a conducive development environment is essential. Follow these steps to set up your Python web development environment:
- Install Python: Ensure you have Python installed on your machine. Use the command `python –version` to verify.
- Create a Virtual Environment: This isolates your project dependencies. Run the following commands:
bash
python -m venv myprojectenv
source myprojectenv/bin/activate # On Windows use: myprojectenv\Scripts\activate
- Install the Chosen Framework: Use pip to install your selected framework. For example, for Django:
bash
pip install django
Building Your First Application
After setting up your environment, you can start building your application. Here is a step-by-step guide using Django as an example:
- Create a Project:
bash
django-admin startproject myproject
cd myproject
- Create an App:
bash
python manage.py startapp myapp
- Define Models: In `myapp/models.py`, define your data models:
python
from django.db import models
class Item(models.Model):
name = models.CharField(max_length=100)
description = models.TextField()
- Register Models: In `myapp/admin.py`, register your models to make them accessible in the admin panel:
python
from django.contrib import admin
from .models import Item
admin.site.register(Item)
- Migrate Database: Apply migrations to create database tables:
bash
python manage.py makemigrations
python manage.py migrate
- Run the Development Server: Start your server to view the application:
bash
python manage.py runserver
Creating Frontend Interfaces
Frontend development is vital for user interaction. You can create HTML templates to serve dynamic content. Here’s how to set it up in Django:
- Create a Templates Directory: Inside your app directory, create a folder named `templates/myapp/`.
- Create an HTML Template: Add an HTML file (e.g., `index.html`) with the following structure:
Welcome to My App
-
{% for item in items %}
- {{ item.name }}: {{ item.description }}
{% endfor %}
- Render the Template in Views: In `myapp/views.py`, create a view to render your template:
python
from django.shortcuts import render
from .models import Item
def index(request):
items = Item.objects.all()
return render(request, ‘myapp/index.html’, {‘items’: items})
- Map URL to View: In `myapp/urls.py`, connect your view to a URL:
python
from django.urls import path
from .views import index
urlpatterns = [
path(”, index, name=’index’),
]
- Include App URLs in Project: In `myproject/urls.py`, include your app’s URLs:
python
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path(‘admin/’, admin.site.urls),
path(”, include(‘myapp.urls’)),
]
Following these steps will help you create a functional web application using Python.
Expert Insights on Building a Website with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Building a website with Python can be both efficient and scalable, particularly when utilizing frameworks like Django or Flask. These frameworks provide built-in features that simplify the development process, allowing developers to focus more on functionality rather than boilerplate code.”
Michael Tran (Web Development Instructor, Code Academy). “For beginners, I recommend starting with Flask due to its simplicity and flexibility. It allows for quick prototyping and is excellent for small to medium-sized applications. Once you grasp the basics, transitioning to Django for larger projects becomes a natural progression.”
Lisa Chen (Lead Developer, Digital Solutions Group). “When building a website with Python, it’s crucial to consider the deployment environment. Utilizing platforms like Heroku or AWS can streamline the deployment process. Additionally, integrating CI/CD practices can enhance the overall efficiency of your development lifecycle.”
Frequently Asked Questions (FAQs)
What are the basic steps to build a website with Python?
To build a website with Python, start by choosing a web framework such as Flask or Django. Set up your development environment, create your project structure, define your routes and views, implement templates for the frontend, and finally, deploy your application to a web server.
Do I need to know HTML and CSS to build a website with Python?
Yes, knowledge of HTML and CSS is essential for building a website with Python. HTML is used for structuring your web pages, while CSS is used for styling them. Understanding these technologies will help you create a more visually appealing and functional website.
What web frameworks are recommended for building websites with Python?
Popular web frameworks for building websites with Python include Django, Flask, and FastAPI. Django is suitable for larger applications with built-in features, Flask is ideal for smaller projects due to its simplicity, and FastAPI is excellent for building APIs with high performance.
How can I deploy my Python website?
You can deploy your Python website using various platforms such as Heroku, AWS, or DigitalOcean. Choose a hosting provider that supports Python applications, configure your server environment, and upload your code. Ensure you set up a web server like Gunicorn or Nginx to handle requests.
Is it necessary to use a database for a Python web application?
While not strictly necessary, using a database is highly recommended for most web applications that require data storage and retrieval. Popular database options for Python applications include SQLite, PostgreSQL, and MySQL, which can be easily integrated using ORM tools like SQLAlchemy or Django’s ORM.
What are some common challenges when building a website with Python?
Common challenges include managing dependencies, ensuring security, optimizing performance, and handling scalability. Additionally, debugging issues and integrating third-party services can also pose difficulties. Addressing these challenges requires careful planning and a good understanding of web development practices.
Building a website with Python involves several key steps, including selecting the appropriate framework, setting up the development environment, and understanding the fundamental components of web development. Popular frameworks such as Django and Flask offer powerful tools for creating dynamic web applications, each catering to different project requirements and developer preferences. By leveraging these frameworks, developers can streamline the process of building robust websites while adhering to best practices in coding and architecture.
Additionally, it is essential to grasp the basics of front-end and back-end development. Front-end technologies like HTML, CSS, and JavaScript are crucial for creating an engaging user interface, while back-end development with Python handles server-side logic, data management, and application functionality. Understanding how these components interact is vital for delivering a seamless user experience.
Furthermore, deploying the website is a critical phase that involves selecting a hosting service and ensuring the application is accessible to users. Familiarity with version control systems like Git and continuous integration practices can significantly enhance collaboration and project management. By following these steps and utilizing the right tools, developers can effectively build and maintain a website using Python.
Author Profile
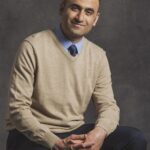
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?