How Can You Build a Website Using Python: A Step-by-Step Guide?
Building a website can seem like a daunting task, especially if you’re venturing into the world of programming for the first time. However, with Python—a versatile and powerful programming language—creating a dynamic and engaging website is not only achievable but also enjoyable. Whether you’re looking to showcase your portfolio, start a blog, or develop a full-fledged web application, Python offers a plethora of frameworks and tools that make the process seamless. In this article, we’ll explore how to harness the power of Python to bring your web development dreams to life.
At its core, building a website with Python involves understanding the fundamental components of web development, including front-end and back-end technologies. Python frameworks like Flask and Django provide robust structures that simplify the development process, allowing you to focus on creating an exceptional user experience. These frameworks come equipped with features that streamline routing, database management, and templating, making it easier for developers to build scalable and maintainable applications.
Moreover, Python’s extensive libraries and community support empower developers to integrate various functionalities into their websites, from user authentication to data visualization. As you embark on your journey to create a website with Python, you’ll discover that the language not only enhances productivity but also fosters creativity, enabling you to craft unique and interactive online experiences. Get ready to dive
Choosing a Web Framework
Selecting the right web framework is crucial when building a website with Python. Frameworks streamline development by providing tools and libraries that simplify common tasks. Here are some popular Python web frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with an ORM (Object-Relational Mapping), authentication, and an admin panel out of the box.
- Flask: A lightweight and flexible micro-framework that is easy to use for small applications. It allows for more control over components.
- FastAPI: Designed for building APIs quickly, FastAPI is highly performant and uses asynchronous programming, making it suitable for applications that require high concurrency.
Each framework has its strengths and weaknesses, so consider the project requirements and your familiarity with the framework when making a choice.
Setting Up Your Development Environment
To begin building your website, you must set up a development environment. This typically includes:
- Python Installation: Ensure you have Python installed on your machine. You can download it from the official Python website.
- Virtual Environment: Create a virtual environment to manage dependencies for your project. Use the following commands:
“`bash
Create a virtual environment
python -m venv myenv
Activate the virtual environment
On Windows
myenv\Scripts\activate
On macOS/Linux
source myenv/bin/activate
“`
- Package Manager: Use `pip` to install necessary packages. For example, to install Django, run:
“`bash
pip install django
“`
Building the Application Structure
Once your environment is ready, you can start structuring your application. Below is an example directory structure for a Django project:
Directory/File | Description |
---|---|
myproject/ | Root directory of your project. |
manage.py | Command-line utility for managing the project. |
myapp/ | Your application directory, containing models, views, and templates. |
templates/ | Directory for HTML templates. |
static/ | Directory for static files (CSS, JavaScript, images). |
This structure helps in organizing your code and assets, making it easier to manage as your application grows.
Creating Views and Templates
Views are Python functions that handle requests and return responses, while templates are HTML files that define the layout of your web pages. For example, in Django, you can create a view like this:
“`python
from django.http import HttpResponse
def home(request):
return HttpResponse(“Welcome to my website!”)
“`
To connect this view to a URL, update your `urls.py`:
“`python
from django.urls import path
from .views import home
urlpatterns = [
path(”, home, name=’home’),
]
“`
For templates, create a file called `home.html` in the `templates` directory:
“`html
Welcome to my website!
“`
Render this template in your view:
“`python
from django.shortcuts import render
def home(request):
return render(request, ‘home.html’)
“`
This approach separates logic from presentation, promoting cleaner code and easier maintenance.
Choosing the Right Framework
When building a website with Python, selecting the appropriate framework is crucial. The choice of framework can significantly influence the development speed, scalability, and overall functionality of your website. Here are some popular Python frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It includes an ORM (Object-Relational Mapping), admin panel, and built-in security features.
- Flask: A micro-framework that is lightweight and easy to use, perfect for smaller applications or when you want more control over components.
- FastAPI: Ideal for building APIs quickly with automatic documentation generation and performance comparable to Node.js and Go.
Framework | Type | Ideal Use Case |
---|---|---|
Django | Full-stack | Large applications with complex requirements |
Flask | Micro | Small to medium applications |
FastAPI | API-centric | High-performance APIs |
Setting Up Your Development Environment
To start developing your website, you need to set up a suitable development environment:
- Install Python: Ensure you have the latest version of Python installed. You can download it from the official Python website.
- Choose a Code Editor: Popular choices include Visual Studio Code, PyCharm, and Sublime Text.
- Create a Virtual Environment:
“`bash
python -m venv myenv
“`
This command creates an isolated environment for your project.
- Activate the Virtual Environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Install Necessary Packages:
Use pip to install the chosen framework and other dependencies:
“`bash
pip install django
“`
Creating Your First Application
Once your environment is set up, you can start creating your application. The steps vary slightly depending on the framework:
- For Django:
- Create a new project:
“`bash
django-admin startproject myproject
“`
- Navigate into your project directory:
“`bash
cd myproject
“`
- Start the development server:
“`bash
python manage.py runserver
“`
- For Flask:
- Create a new Python file (e.g., `app.py`).
- Add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
- Run the application:
“`bash
python app.py
“`
Building Out Features
When building out the features of your website, consider the following components:
- Routing: Define how URLs correspond to your application’s logic.
- Templates: Use templating engines like Jinja2 (for Flask) or Django’s built-in templating system to create dynamic HTML pages.
- Database Integration: Use ORMs like Django ORM or SQLAlchemy to manage data.
- User Authentication: Implement user registration and login functionalities for secure access.
Testing Your Application
Testing is vital to ensure your application functions as expected. Both Django and Flask come with testing tools:
- Django: Use the built-in testing framework to write unit tests.
- Flask: Use the `unittest` module or Flask-Testing to create tests for your app.
Deploying Your Website
After development and testing, deploying your website is the next step. Common deployment options include:
- Heroku: A cloud platform that allows you to deploy applications easily.
- AWS: Provides extensive services for hosting and managing applications.
- DigitalOcean: A simpler cloud hosting provider that is beginner-friendly.
Steps to deploy on Heroku:
- Install the Heroku CLI.
- Create a `requirements.txt` and `Procfile`.
- Use Git to push your code to Heroku.
Following these structured steps will guide you through building a functional website using Python, ensuring you cover essential aspects of development, testing, and deployment.
Expert Insights on Building Websites with Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Building a website with Python can be highly efficient, especially when utilizing frameworks like Django or Flask. These frameworks provide robust tools for rapid development and scalability, making them ideal for both startups and established businesses.”
Michael Thompson (Web Development Instructor, Code Academy). “For beginners, I recommend starting with Flask due to its simplicity and flexibility. It allows developers to grasp the fundamentals of web development without being overwhelmed by too many features at once.”
Sarah Lee (Lead Developer, Digital Solutions Group). “When building a website with Python, it is crucial to focus on security and performance. Implementing best practices such as input validation and using secure libraries can significantly enhance the integrity of your web application.”
Frequently Asked Questions (FAQs)
What are the basic requirements to build a website with Python?
To build a website with Python, you need a Python environment set up on your machine, a web framework such as Flask or Django, knowledge of HTML, CSS, and JavaScript, and a text editor or IDE for coding.
Which Python web frameworks are recommended for beginners?
Flask is highly recommended for beginners due to its simplicity and flexibility. Django is another excellent choice, offering a more comprehensive framework with built-in features for larger applications.
How do I set up a local development environment for Python web development?
You can set up a local development environment by installing Python, setting up a virtual environment using `venv`, and installing your chosen web framework via pip. Additionally, you may want to install a database like SQLite or PostgreSQL.
Can I deploy a Python website on a cloud platform?
Yes, you can deploy a Python website on various cloud platforms such as Heroku, AWS, Google Cloud Platform, and DigitalOcean. Each platform provides documentation for deploying Python applications.
What are some common challenges when building a website with Python?
Common challenges include managing dependencies, handling database migrations, ensuring security practices, optimizing performance, and debugging issues that arise during development.
Is it necessary to learn front-end technologies when building a website with Python?
Yes, understanding front-end technologies such as HTML, CSS, and JavaScript is essential for creating a complete web application, as they are crucial for designing user interfaces and enhancing user experience.
Building a website with Python involves several key steps, including selecting the appropriate framework, setting up the development environment, and deploying the application. Popular frameworks such as Django and Flask offer robust tools for web development, catering to different project requirements. Django is ideal for larger applications due to its built-in features and scalability, while Flask provides flexibility for smaller projects or microservices.
To begin, developers must establish a suitable development environment, which typically includes installing Python, the chosen web framework, and any necessary libraries or dependencies. Utilizing version control systems like Git can greatly enhance collaboration and project management. Additionally, understanding the basics of HTML, CSS, and JavaScript is essential for creating an engaging front-end experience.
Once the website is developed, deploying it on a web server is the final step. This can be achieved through various platforms, such as Heroku, AWS, or DigitalOcean, which offer different levels of service and scalability. Ensuring that the website is secure and optimized for performance is crucial for user experience and search engine visibility.
In summary, building a website with Python is a structured process that requires careful planning, the right tools, and an understanding of both back-end and front-end technologies. By leveraging Python’s
Author Profile
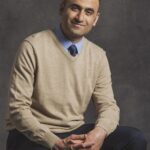
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?