How Can You Effectively Cache HTTP Requests in Angular 12? An Example Guide
In the fast-paced world of web development, optimizing performance is crucial for delivering a seamless user experience. One powerful technique that Angular developers can leverage is caching HTTP requests. By storing the results of previous requests, applications can significantly reduce load times, enhance responsiveness, and minimize unnecessary server calls. In this article, we will explore how to implement caching for HTTP requests in Angular 12, providing you with practical insights and examples to elevate your application’s efficiency.
Caching HTTP requests in Angular 12 not only improves performance but also reduces the strain on backend services. When a user navigates through an application, repeated data fetching can lead to delays and increased latency. By caching responses, developers can ensure that frequently accessed data is readily available, allowing for quicker rendering of components and a more fluid user experience. This approach is particularly beneficial for applications that rely on static or infrequently changing data.
In this guide, we will delve into the various strategies for implementing HTTP request caching in Angular 12. From leveraging built-in services to creating custom caching mechanisms, we will cover essential concepts and provide practical examples that illustrate how to effectively manage and utilize cached data. Whether you are building a small application or a large-scale enterprise solution, understanding how to cache HTTP requests will empower you to create more efficient Angular applications.
Implementing HTTP Request Caching in Angular 12
To effectively cache HTTP requests in Angular 12, you can utilize the `HttpClient` service along with RxJS operators. This allows you to store responses and avoid unnecessary network calls for the same requests.
Using Interceptors for Caching
Angular’s `HttpInterceptor` is a powerful feature that can be leveraged for caching HTTP requests. By implementing an interceptor, you can intercept outgoing requests and incoming responses, allowing you to manage caching logic seamlessly.
Steps to Implement an HTTP Interceptor:
- Create an Interceptor: Generate a new service using Angular CLI.
“`bash
ng generate service interceptors/cache
“`
- Implement Caching Logic: In the created service, implement the caching logic as shown below:
“`typescript
import { Injectable } from ‘@angular/core’;
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from ‘@angular/common/http’;
import { Observable } from ‘rxjs’;
import { tap } from ‘rxjs/operators’;
@Injectable()
export class CacheInterceptor implements HttpInterceptor {
private cache = new Map
intercept(req: HttpRequest
if (req.method !== ‘GET’) {
return next.handle(req);
}
const cachedResponse = this.cache.get(req.url);
if (cachedResponse) {
return new Observable(observer => {
observer.next(cachedResponse);
observer.complete();
});
}
return next.handle(req).pipe(
tap(event => {
if (event.type === HttpEventType.Response) {
this.cache.set(req.url, event);
}
})
);
}
}
“`
- Register the Interceptor: Register the interceptor in your application module.
“`typescript
import { HTTP_INTERCEPTORS } from ‘@angular/common/http’;
import { CacheInterceptor } from ‘./interceptors/cache.service’;
@NgModule({
providers: [
{
provide: HTTP_INTERCEPTORS,
useClass: CacheInterceptor,
multi: true
}
]
})
export class AppModule {}
“`
Additional Caching Strategies
While interceptors provide a robust method for caching, consider these additional strategies:
- Using Local Storage: Store responses in local storage for persistence across sessions.
- Memory Caching: Keep frequently accessed data in memory to reduce response times.
Example of Local Storage Caching
You can enhance the caching mechanism by storing responses in local storage. Below is an example of how to implement this.
“`typescript
intercept(req: HttpRequest
const cachedResponse = localStorage.getItem(req.url);
if (cachedResponse) {
return of(JSON.parse(cachedResponse));
}
return next.handle(req).pipe(
tap(event => {
if (event.type === HttpEventType.Response) {
localStorage.setItem(req.url, JSON.stringify(event));
}
})
);
}
“`
Performance Comparison Table
The following table summarizes the performance of different caching strategies in Angular.
Strategy | Advantages | Disadvantages |
---|---|---|
HTTP Interceptor |
|
|
Local Storage |
|
|
Memory Caching |
|
|
By implementing these techniques, you can optimize your Angular applications for better performance and user experience through effective caching of HTTP requests.
Understanding HTTP Request Caching in Angular 12
Caching HTTP requests in Angular can significantly improve application performance by reducing the number of network calls and speeding up response times. Angular provides several strategies to implement caching effectively.
Using HttpClient with Interceptors
HttpClient interceptors allow you to intercept HTTP requests and responses globally. By creating a caching interceptor, you can store responses in memory and return them for subsequent requests.
Creating a Caching Interceptor
- Generate an Interceptor:
Use Angular CLI to create an interceptor.
“`bash
ng generate interceptor caching
“`
- Implement the Interceptor:
In the generated file, implement the caching logic as follows:
“`typescript
import { Injectable } from ‘@angular/core’;
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from ‘@angular/common/http’;
import { Observable, of } from ‘rxjs’;
import { tap } from ‘rxjs/operators’;
@Injectable()
export class CachingInterceptor implements HttpInterceptor {
private cache: Map
intercept(req: HttpRequest
const cachedResponse = this.cache.get(req.url);
if (cachedResponse) {
return of(cachedResponse);
}
return next.handle(req).pipe(
tap(event => {
if (event instanceof HttpResponse) {
this.cache.set(req.url, event);
}
})
);
}
}
“`
- Register the Interceptor:
Register the interceptor in your app module.
“`typescript
import { HTTP_INTERCEPTORS } from ‘@angular/common/http’;
@NgModule({
…
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: CachingInterceptor, multi: true }
]
})
export class AppModule { }
“`
Cache Expiration Strategy
Implementing a cache expiration strategy ensures that stale data does not persist indefinitely. Here are a few approaches to consider:
- Time-based Expiration:
Store a timestamp along with the cached data and check the elapsed time on subsequent requests.
- Size-based Expiration:
Limit the number of cached entries and remove the least recently used (LRU) item when the limit is reached.
- Manual Invalidation:
Provide methods to invalidate the cache when data updates are known.
Example of Time-based Expiration
Modify the caching logic to include expiration:
“`typescript
private cache: Map
private cacheTimeLimit = 5 * 60 * 1000; // 5 minutes
intercept(req: HttpRequest
const cachedEntry = this.cache.get(req.url);
const currentTime = Date.now();
if (cachedEntry && (currentTime – cachedEntry.timestamp) < this.cacheTimeLimit) {
return of(cachedEntry.response);
}
return next.handle(req).pipe(
tap(event => {
if (event instanceof HttpResponse) {
this.cache.set(req.url, { response: event, timestamp: currentTime });
}
})
);
}
“`
Using Angular Services for Caching
Creating a dedicated service for managing cached data can enhance code modularity and reusability. This service can handle fetching data and caching it as needed.
Example of a Caching Service
- Generate a Service:
Use Angular CLI to create a caching service.
“`bash
ng generate service cache
“`
- Implement the Service:
Here is a simple example of a caching service.
“`typescript
import { Injectable } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
import { Observable } from ‘rxjs’;
@Injectable({
providedIn: ‘root’
})
export class CacheService {
private cache = new Map
constructor(private http: HttpClient) {}
getData(url: string): Observable
if (this.cache.has(url)) {
return of(this.cache.get(url));
} else {
return this.http.get(url).pipe(tap(data => this.cache.set(url, data)));
}
}
}
“`
- **Usage**:
Inject the caching service in your components to use cached data.
“`typescript
constructor(private cacheService: CacheService) {}
this.cacheService.getData(‘api/data’).subscribe(data => {
console.log(data);
});
“`
Implementing HTTP request caching in Angular 12 using interceptors or services can significantly enhance your application’s performance while ensuring data freshness through appropriate expiration strategies.
Expert Insights on Caching HTTP Requests in Angular 12
Dr. Emily Carter (Senior Software Engineer, Angular Development Group). “Caching HTTP requests in Angular 12 can significantly enhance application performance. By utilizing Angular’s built-in HttpClient service alongside RxJS operators, developers can implement caching mechanisms that store responses and reduce the number of network calls.”
Mark Thompson (Lead Frontend Architect, Tech Innovations Inc.). “To effectively cache HTTP requests in Angular 12, I recommend creating a service that handles caching logic. This service can store responses in memory or local storage and check for existing cached data before making a new request, thus optimizing load times and user experience.”
Sarah Jenkins (Full-Stack Developer, CodeCraft Solutions). “Implementing caching in Angular 12 requires careful consideration of data freshness. It’s essential to set appropriate cache expiration strategies to ensure that users receive up-to-date information while still benefiting from the performance improvements that caching provides.”
Frequently Asked Questions (FAQs)
How can I implement HTTP request caching in Angular 12?
You can implement HTTP request caching in Angular 12 by using the `HttpClient` service along with an in-memory caching mechanism, such as a service that stores responses in a JavaScript object or a more sophisticated solution like RxJS operators.
What is the purpose of caching HTTP requests?
Caching HTTP requests improves application performance by reducing the number of network calls, decreasing load times, and enhancing user experience by serving previously fetched data quickly.
Can I use Angular’s built-in services for caching?
Angular does not provide built-in caching for HTTP requests, but you can create a custom service that utilizes RxJS operators, such as `shareReplay`, to cache responses for reuse.
How do I clear the cache for HTTP requests in Angular 12?
To clear the cache, you can implement a method in your caching service that removes specific entries or clears the entire cache object when necessary, such as upon user logout or data refresh.
Is it possible to cache only specific HTTP requests?
Yes, you can selectively cache HTTP requests by adding logic in your caching service to determine which requests to cache based on the request URL, method, or other criteria.
What are some libraries that can help with HTTP request caching in Angular?
Libraries like `ngx-cacheable` or `angular-cache` can assist with HTTP request caching, providing decorators and services to simplify the implementation of caching strategies in Angular applications.
In Angular 12, caching HTTP requests is a valuable technique that can significantly enhance application performance and user experience. By storing the results of HTTP requests, applications can reduce the need for repeated network calls, leading to faster data retrieval and lower latency. Implementing caching effectively involves utilizing Angular’s built-in services, such as HttpClient, alongside custom caching strategies that can be tailored to specific application needs.
One common approach to caching in Angular is to create a service that manages HTTP requests and their cached responses. This service can check if a requested resource is already available in the cache before making a network call. If the resource is present, it can return the cached data, thereby avoiding unnecessary HTTP requests. Conversely, if the resource is not cached, the service can fetch the data from the server and store it for future use. This method not only improves performance but also optimizes bandwidth usage.
Additionally, developers should consider cache invalidation strategies to ensure that the application displays the most up-to-date information. This can involve setting expiration times for cached data or implementing mechanisms to refresh the cache when certain conditions are met, such as user actions or specific events. By balancing caching efficiency with data accuracy, developers can create robust applications that deliver a seamless
Author Profile
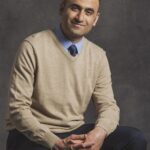
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?