How Can You Calculate Gradient Descent in Python?
In the ever-evolving world of machine learning and optimization, gradient descent stands out as a fundamental algorithm that drives many modern applications. Whether you’re training a neural network or fine-tuning a model for predictive analytics, understanding how to calculate gradient descent in Python is crucial for achieving optimal results. This powerful technique not only helps minimize errors but also enhances the efficiency of learning algorithms, making it a cornerstone of data science and artificial intelligence.
At its core, gradient descent is a mathematical optimization method used to find the minimum of a function. By iteratively adjusting parameters in the direction of the steepest descent, it allows models to converge towards the best possible solution. Implementing gradient descent in Python is not only straightforward but also highly effective, thanks to the rich ecosystem of libraries and tools available. From NumPy to TensorFlow, Python provides a robust framework for developers and data scientists to harness the power of this algorithm.
As we delve deeper into the mechanics of gradient descent, we will explore its various forms, such as batch, stochastic, and mini-batch gradient descent, each with its unique advantages and applications. Additionally, we will discuss how to implement these techniques using Python, providing you with the knowledge and skills to optimize your models effectively. Whether you’re a seasoned programmer or a
Understanding Gradient Descent
Gradient descent is an optimization algorithm used to minimize the cost function in machine learning and deep learning models. The goal of gradient descent is to find the parameter values (weights) that minimize the cost function. The algorithm iteratively adjusts the parameters in the direction of the steepest descent as defined by the negative of the gradient.
The basic steps of gradient descent include:
- Initialization: Start with random values for the parameters.
- Compute the gradient: Calculate the gradient of the cost function with respect to the parameters.
- Update the parameters: Adjust the parameters by taking a small step in the direction of the negative gradient.
- Repeat: Continue this process until convergence, which is typically defined as when the change in the cost function is less than a specified threshold.
Implementing Gradient Descent in Python
To implement gradient descent in Python, you can use NumPy for mathematical operations. Below is a basic example illustrating how to perform gradient descent for a simple linear regression model.
“`python
import numpy as np
Define the cost function
def compute_cost(X, y, theta):
m = len(y)
predictions = X.dot(theta)
cost = (1/(2*m)) * np.sum(np.square(predictions – y))
return cost
Perform gradient descent
def gradient_descent(X, y, theta, learning_rate, iterations):
m = len(y)
cost_history = np.zeros(iterations)
for i in range(iterations):
predictions = X.dot(theta)
errors = predictions – y
theta -= (learning_rate/m) * (X.T.dot(errors))
cost_history[i] = compute_cost(X, y, theta)
return theta, cost_history
Example usage
X = np.array([[1, 1], [1, 2], [1, 3]]) Feature matrix (with intercept)
y = np.array([1, 2, 3]) Output vector
theta = np.array([0.1, 0.2]) Initial parameters
learning_rate = 0.01
iterations = 1000
theta_final, cost_history = gradient_descent(X, y, theta, learning_rate, iterations)
print(“Final parameters:”, theta_final)
print(“Cost history:”, cost_history[-10:]) Display last 10 cost values
“`
Parameters in Gradient Descent
While implementing gradient descent, several parameters must be considered to ensure optimal performance:
- Learning Rate: Determines the size of the steps taken towards the minimum. A small learning rate may lead to slow convergence, while a large learning rate might overshoot the minimum.
- Iterations: Refers to the number of times the algorithm will update the parameters. More iterations can improve convergence but may lead to longer computation times.
Parameter | Description | Typical Value |
---|---|---|
Learning Rate | Controls the step size during optimization | 0.01 – 0.1 |
Iterations | Number of times the algorithm updates parameters | 1000 – 10000 |
Initial Theta | Starting point for the parameters | Random values |
By fine-tuning these parameters, users can significantly impact the efficiency and effectiveness of the gradient descent algorithm.
Understanding Gradient Descent
Gradient descent is an optimization algorithm used to minimize the cost function in machine learning models. The algorithm iteratively adjusts parameters in the direction of the negative gradient of the cost function.
Key concepts include:
- Learning Rate: A hyperparameter that determines the step size at each iteration while moving toward a minimum.
- Cost Function: A function that measures the performance of the model; common examples include Mean Squared Error (MSE) for regression tasks.
- Iterations: The number of times the algorithm will update the parameters.
Implementing Gradient Descent in Python
To implement gradient descent in Python, you can use libraries such as NumPy for efficient numerical computations. Below is a simplified example illustrating how to perform gradient descent on a linear regression model.
“`python
import numpy as np
Hypothetical dataset
X = np.array([1, 2, 3, 4, 5])
y = np.array([2, 4, 6, 8, 10])
Parameters
m = 0 slope
b = 0 intercept
learning_rate = 0.01
n_iterations = 1000
n = len(y)
Gradient Descent Algorithm
for _ in range(n_iterations):
y_pred = m * X + b Predictions
error = y_pred – y Error calculation
cost = (1/n) * np.sum(error ** 2) Cost function (MSE)
Gradients
m_gradient = (2/n) * np.dot(X, error)
b_gradient = (2/n) * np.sum(error)
Update parameters
m -= learning_rate * m_gradient
b -= learning_rate * b_gradient
print(f”Slope: {m}, Intercept: {b}”)
“`
Key Components of the Code
- Dataset: The example uses a simple linear relationship between `X` and `y`.
- Parameters Initialization: Slope (`m`) and intercept (`b`) are initialized to zero.
- Learning Rate and Iterations: These control the speed and the number of updates, respectively.
Variable | Description |
---|---|
`X` | Independent variable (features) |
`y` | Dependent variable (target) |
`m` | Slope of the line |
`b` | Intercept of the line |
`learning_rate` | Step size for parameter updates |
`n_iterations` | Number of iterations to run gradient descent |
`n` | Number of data points |
Adjusting Parameters
When implementing gradient descent, consider adjusting the learning rate based on the convergence behavior:
- Too High: May cause divergence, leading to oscillation or divergence from the minimum.
- Too Low: Results in slow convergence, increasing computation time.
Utilize techniques like learning rate decay or adaptive methods (e.g., Adam, RMSProp) for improved performance in complex models.
Expert Insights on Calculating Gradient Descent in Python
Dr. Emily Carter (Data Scientist, AI Innovations Inc.). Gradient descent is a fundamental optimization technique used in machine learning. To calculate it in Python, one must implement the algorithm iteratively, adjusting the parameters based on the gradient of the loss function. Libraries like NumPy can facilitate matrix operations, making the implementation both efficient and straightforward.
Michael Chen (Machine Learning Engineer, Tech Solutions Group). When calculating gradient descent in Python, it’s crucial to choose an appropriate learning rate. A learning rate that is too high can cause divergence, while one that is too low can lead to slow convergence. Utilizing libraries such as TensorFlow or PyTorch can help streamline the process, as they provide built-in functions for gradient computation.
Sarah Patel (Professor of Computer Science, University of Technology). Understanding the mathematical foundation of gradient descent is essential for effective implementation in Python. One should ensure to compute the gradients accurately and consider techniques like momentum or adaptive learning rates to enhance performance. Visualization of the convergence process can also provide valuable insights into the optimization journey.
Frequently Asked Questions (FAQs)
What is gradient descent?
Gradient descent is an optimization algorithm used to minimize a function by iteratively moving towards the steepest descent direction, defined by the negative of the gradient.
How do I implement gradient descent in Python?
To implement gradient descent in Python, you define the cost function, compute the gradient, and update the parameters iteratively using the formula: `theta = theta – learning_rate * gradient`.
What libraries are commonly used for gradient descent in Python?
Common libraries include NumPy for numerical calculations, TensorFlow and PyTorch for machine learning applications, and SciPy for optimization tasks.
How do I choose the learning rate for gradient descent?
Choosing the learning rate involves experimentation. A small learning rate may lead to slow convergence, while a large one can cause divergence. Techniques like learning rate schedules can help.
What are the common variations of gradient descent?
Common variations include Batch Gradient Descent, Stochastic Gradient Descent (SGD), and Mini-batch Gradient Descent, each differing in how they use data for updates.
How can I visualize gradient descent in Python?
You can visualize gradient descent by plotting the cost function and the parameter updates over iterations using libraries like Matplotlib to show how the algorithm converges to the minimum.
Gradient descent is a fundamental optimization algorithm used in machine learning and statistics to minimize a function by iteratively moving towards the steepest descent as defined by the negative of the gradient. In Python, implementing gradient descent involves defining the function to be minimized, calculating its gradient, and updating the parameters in the direction that reduces the function’s value. This process is typically repeated until convergence is achieved, which is determined by a predefined threshold or a maximum number of iterations.
To calculate gradient descent in Python, one can utilize libraries such as NumPy for efficient numerical computations. The implementation generally requires specifying the learning rate, which controls the step size during the update process, and the initial parameters. A well-structured approach includes defining the objective function, computing the gradient, and updating the parameters in a loop until the stopping criteria are met. This method can be applied to various machine learning models, including linear regression and neural networks.
Key takeaways from the discussion on gradient descent include the importance of selecting an appropriate learning rate, as a value too high may lead to divergence, while a value too low can result in slow convergence. Additionally, using techniques such as momentum or adaptive learning rates can enhance the performance of gradient descent. Understanding the mathematical foundation behind
Author Profile
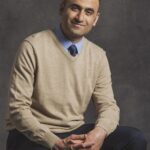
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?