How Can You Calculate Percentage in Python Effortlessly?
Calculating percentages is a fundamental skill that finds its application in various fields, from finance to data analysis and beyond. In the world of programming, particularly with Python, mastering the art of percentage calculation can significantly enhance your ability to manipulate and interpret data effectively. Whether you’re a beginner looking to grasp the basics or an experienced coder seeking to refine your skills, understanding how to calculate percentages in Python opens up a realm of possibilities for data-driven decision-making.
In Python, calculating a percentage is not just about applying a formula; it involves leveraging the language’s versatile syntax and built-in functions to perform operations efficiently. The process typically involves taking a part of a whole and converting it into a fraction of 100, which can be easily achieved with simple arithmetic operations. By utilizing variables, you can dynamically compute percentages based on user input or data from external sources, making your code more interactive and functional.
Moreover, Python provides various libraries that can simplify complex calculations and data manipulations, allowing for more sophisticated percentage calculations in larger datasets. From basic operations to advanced statistical analyses, understanding how to calculate percentages in Python equips you with the tools necessary to tackle a wide range of programming challenges. Get ready to dive deeper into the methods and techniques that will empower you to handle percentages like a pro!
Basic Percentage Calculation
To calculate a percentage in Python, you can use a simple mathematical formula. The percentage can be determined by dividing the part by the whole and then multiplying the result by 100. The formula is as follows:
\[ \text{Percentage} = \left( \frac{\text{Part}}{\text{Whole}} \right) \times 100 \]
In Python, this can be implemented easily with a function. Here’s a basic example:
“`python
def calculate_percentage(part, whole):
return (part / whole) * 100
“`
You can call this function with the specific values you wish to compute, for instance:
“`python
result = calculate_percentage(25, 200)
print(result) Output: 12.5
“`
Using Python’s Built-in Functions
Python provides built-in functions that can simplify calculations, especially when working with lists or arrays. For example, if you are handling a collection of values and want to calculate the percentage of each relative to the total, you can use the `sum()` function in conjunction with list comprehensions.
“`python
values = [10, 20, 30, 40]
total = sum(values)
percentages = [(value / total) * 100 for value in values]
print(percentages) Output: [10.0, 20.0, 30.0, 40.0]
“`
This snippet computes the percentage of each element in the list with respect to the total sum of the list.
Calculating Percentage Change
To calculate the percentage change between two values, you can apply the following formula:
\[ \text{Percentage Change} = \left( \frac{\text{New Value} – \text{Old Value}}{\text{Old Value}} \right) \times 100 \]
Here’s how you can implement this in Python:
“`python
def calculate_percentage_change(old_value, new_value):
return ((new_value – old_value) / old_value) * 100
“`
For example, if you want to calculate the percentage change from 50 to 75:
“`python
change = calculate_percentage_change(50, 75)
print(change) Output: 50.0
“`
Using Numpy for Large Datasets
When dealing with large datasets, using libraries like NumPy can enhance performance and simplify calculations. NumPy allows you to compute percentages across entire arrays efficiently. Here’s an example:
“`python
import numpy as np
data = np.array([100, 200, 300])
total = np.sum(data)
percentages = (data / total) * 100
print(percentages) Output: [16.66666667 33.33333333 50. ]
“`
This approach is particularly useful in data analysis and scientific computing.
Summary Table of Percentage Calculations
The following table summarizes the different methods of calculating percentages in Python:
Calculation Type | Formula | Python Code |
---|---|---|
Basic Percentage | (Part / Whole) * 100 | calculate_percentage(part, whole) |
Percentage Change | ((New – Old) / Old) * 100 | calculate_percentage_change(old_value, new_value) |
Using NumPy | (data / total) * 100 | np.array([…]) |
By leveraging these methods, you can effectively compute percentages for various applications within your Python projects.
Basic Percentage Calculation
To calculate a percentage in Python, you can use a straightforward mathematical approach. The formula for calculating a percentage is:
\[
\text{Percentage} = \left( \frac{\text{Part}}{\text{Whole}} \right) \times 100
\]
In Python, this can be implemented using simple arithmetic. Here’s an example:
“`python
part = 50
whole = 200
percentage = (part / whole) * 100
print(f”The percentage is {percentage}%”)
“`
This code snippet will output: “The percentage is 25.0%”.
Using Functions for Reusability
To enhance code reusability, you can define a function that calculates the percentage. Here’s how you might implement this:
“`python
def calculate_percentage(part, whole):
if whole == 0:
return “Whole cannot be zero.”
return (part / whole) * 100
Example usage
result = calculate_percentage(50, 200)
print(f”The percentage is {result}%”)
“`
This function checks if the `whole` is zero to avoid division errors, returning a message instead.
Calculating Percentage Increase or Decrease
When calculating percentage change, you can use the formula:
\[
\text{Percentage Change} = \left( \frac{\text{New Value} – \text{Old Value}}{\text{Old Value}} \right) \times 100
\]
Here’s how to implement this in Python:
“`python
def percentage_change(old_value, new_value):
if old_value == 0:
return “Old value cannot be zero.”
return ((new_value – old_value) / old_value) * 100
Example usage
change = percentage_change(100, 150)
print(f”The percentage change is {change}%”)
“`
This snippet calculates and prints the percentage increase from an old value to a new value.
Handling Percentages in DataFrames
For applications involving data analysis, such as with the Pandas library, calculating percentages across data frames is common. Here’s an example of how to calculate the percentage of a column relative to the sum of that column:
“`python
import pandas as pd
data = {‘Values’: [10, 20, 30, 40]}
df = pd.DataFrame(data)
df[‘Percentage’] = (df[‘Values’] / df[‘Values’].sum()) * 100
print(df)
“`
This will add a new column to the DataFrame with the percentage of each value relative to the total sum.
Using Numpy for Efficient Calculations
For numerical calculations, the Numpy library can be utilized for efficient percentage calculations, especially with large datasets:
“`python
import numpy as np
values = np.array([10, 20, 30, 40])
percentages = (values / np.sum(values)) * 100
print(percentages)
“`
This method leverages Numpy’s optimized functions for calculations and can handle larger datasets more efficiently than traditional loops.
Through these examples, you can implement various methods to calculate percentages in Python, from basic arithmetic to leveraging libraries like Pandas and Numpy for more complex datasets.
Expert Insights on Calculating Percentages in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Calculating percentages in Python can be efficiently done using simple arithmetic operations. By dividing the part by the whole and multiplying by 100, you can derive the percentage. Utilizing libraries like NumPy can further streamline this process for larger datasets.”
Michael Chen (Software Engineer, CodeCraft Solutions). “In Python, the calculation of percentages can be straightforward. You can create a function to encapsulate the logic, ensuring reusability. Additionally, leveraging Python’s built-in functions allows for cleaner and more maintainable code.”
Sarah Patel (Python Educator, LearnPython Academy). “Teaching how to calculate percentages in Python is crucial for beginners. I emphasize using clear examples and visualizations to help students understand the concept. It’s also beneficial to explore how percentages apply in real-world scenarios, enhancing their learning experience.”
Frequently Asked Questions (FAQs)
How do I calculate the percentage of a number in Python?
To calculate the percentage of a number in Python, use the formula: `(part / total) * 100`. For example, to find what percentage 25 is of 200, you would write: `percentage = (25 / 200) * 100`.
What Python function can I use to calculate percentages?
There is no built-in function specifically for calculating percentages in Python. However, you can create a simple function to encapsulate the percentage calculation logic:
“`python
def calculate_percentage(part, total):
return (part / total) * 100
“`
Can I calculate percentages using NumPy in Python?
Yes, you can use NumPy to calculate percentages efficiently, especially with arrays. For example, if you have a NumPy array, you can calculate the percentage of each element relative to the sum of the array:
“`python
import numpy as np
arr = np.array([10, 20, 30])
percentages = (arr / np.sum(arr)) * 100
“`
How can I format the percentage output in Python?
You can format the percentage output using Python’s string formatting methods. For example:
“`python
percentage = 25.0
formatted_percentage = “{:.2f}%”.format(percentage)
“`
This will display the percentage with two decimal places.
Is it possible to calculate percentage change in Python?
Yes, you can calculate percentage change using the formula: `((new_value – old_value) / old_value) * 100`. For example:
“`python
old_value = 50
new_value = 75
percentage_change = ((new_value – old_value) / old_value) * 100
“`
What libraries can assist with percentage calculations in Python?
While basic percentage calculations can be done using standard Python, libraries like Pandas can simplify operations on datasets. For instance, you can use Pandas to calculate percentages across DataFrame columns easily.
Calculating percentages in Python is a straightforward process that can be accomplished using basic arithmetic operations. The fundamental formula for calculating a percentage is to divide the part by the whole and then multiply the result by 100. This can be easily implemented in Python using simple expressions, making it accessible for both beginners and experienced programmers.
To perform percentage calculations, one can utilize Python’s built-in data types such as integers and floats. For example, if you have a part value and a whole value, you can compute the percentage by using the expression `(part / whole) * 100`. This method is efficient and can be adapted for various applications, including financial calculations, data analysis, and statistical evaluations.
Moreover, Python provides libraries such as NumPy and Pandas that can enhance percentage calculations, especially when dealing with large datasets. These libraries offer functions that streamline operations and improve performance, making them valuable tools for data scientists and analysts. Understanding how to calculate percentages in Python not only aids in numerical analysis but also enhances one’s programming skills in handling mathematical computations.
Author Profile
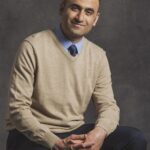
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?