How Do You Call a Class in Python? A Step-by-Step Guide
### Introduction
In the world of Python programming, classes serve as the blueprint for creating objects, encapsulating data, and defining behaviors. Whether you’re building a simple script or a complex application, understanding how to effectively call a class is a fundamental skill that can elevate your coding prowess. As you dive into the realm of object-oriented programming, mastering this concept will not only enhance your ability to organize and manage code but also empower you to write more modular and reusable software.
When you call a class in Python, you are essentially creating an instance of that class, allowing you to access its properties and methods. This process is straightforward yet powerful, enabling you to harness the full potential of your code. From instantiating objects to invoking their functionalities, the process of calling a class is the gateway to leveraging the principles of encapsulation and abstraction that object-oriented programming offers.
As you explore the nuances of calling classes in Python, you’ll discover various techniques and best practices that can streamline your development process. Whether you’re a novice programmer or an experienced developer looking to refine your skills, understanding how to call classes effectively will open up new avenues for creativity and efficiency in your coding journey. Get ready to unlock the secrets of Python classes and transform the way you approach programming!
Creating a Class in Python
To effectively call a class in Python, one must first define the class. A class is a blueprint for creating objects, encapsulating data for the object and methods to manipulate that data. Here’s a basic example of how to create a class:
python
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return f”{self.name} says Woof!”
In this example, the `Dog` class has an initializer method `__init__`, which sets the `name` and `age` attributes for each instance of the class, and a method `bark()` that returns a string.
Instantiating a Class
To call a class, you instantiate it by creating an object. This is done by calling the class name followed by parentheses. For the `Dog` class defined earlier, you would instantiate it like this:
python
my_dog = Dog(“Buddy”, 3)
Here, `my_dog` is an instance of the `Dog` class, with the name “Buddy” and age 3.
Accessing Attributes and Methods
Once you have an instance of a class, you can access its attributes and methods using the dot notation:
python
print(my_dog.name) # Output: Buddy
print(my_dog.bark()) # Output: Buddy says Woof!
### Key Points to Remember
- Class Definition: Use the `class` keyword followed by the class name.
- Constructor: The `__init__` method initializes the object’s attributes.
- Method Calls: Use dot notation to access methods and attributes of the instance.
Example of Multiple Instances
You can create multiple instances of a class with different attributes. For instance:
python
dog1 = Dog(“Max”, 5)
dog2 = Dog(“Bella”, 2)
print(dog1.bark()) # Output: Max says Woof!
print(dog2.bark()) # Output: Bella says Woof!
Table of Class Characteristics
Characteristic | Description |
---|---|
Attributes | Variables that hold data specific to the object. |
Methods | Functions defined within the class that operate on the object’s data. |
Encapsulation | Bundling of data and methods that operate on that data. |
Inheritance | Ability to create a new class based on an existing class. |
By understanding these components, you can effectively call and utilize classes in Python to create complex programs and systems.
Creating a Class in Python
To call a class in Python, one must first define it. A class is created using the `class` keyword followed by the class name and a colon. Here is an example of a simple class definition:
python
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f”{self.name} says Woof!”
In this example, `Dog` is a class with an initializer method `__init__` that sets the dog’s name and a method `bark` that returns a string indicating the dog is barking.
Instantiating a Class
Once a class is defined, you can create an instance (or object) of that class. This is done by calling the class as if it were a function, passing any required arguments.
Example of instantiation:
python
my_dog = Dog(“Buddy”)
Here, `my_dog` is an instance of the `Dog` class with the name “Buddy”.
Calling Class Methods
To call a method defined in a class, use the instance followed by a dot and then the method name, including parentheses.
Example of calling the `bark` method:
python
print(my_dog.bark()) # Output: Buddy says Woof!
This line calls the `bark` method on the `my_dog` instance and prints the output.
Accessing Class Attributes
You can access an instance’s attributes directly through the instance:
python
print(my_dog.name) # Output: Buddy
This retrieves the `name` attribute of the `my_dog` instance.
Using Class Variables
Classes can also have class variables, which are shared among all instances. Here is how to define and access them:
python
class Dog:
species = “Canis lupus familiaris” # Class variable
def __init__(self, name):
self.name = name
print(Dog.species) # Output: Canis lupus familiaris
In this example, `species` is a class variable that can be accessed using the class name.
Example with Multiple Instances
You can create multiple instances of a class, each with its own attributes while sharing class variables.
python
dog1 = Dog(“Buddy”)
dog2 = Dog(“Max”)
print(dog1.bark()) # Output: Buddy says Woof!
print(dog2.bark()) # Output: Max says Woof!
print(Dog.species) # Output: Canis lupus familiaris
Each instance retains its own state, while the class variable remains constant across instances.
Inheritance in Classes
Python supports inheritance, allowing one class to inherit the attributes and methods of another. This is done by defining a class that specifies its parent class in parentheses.
Example of inheritance:
python
class Puppy(Dog):
def wag_tail(self):
return f”{self.name} is wagging its tail!”
Here, `Puppy` inherits from `Dog` and adds a new method `wag_tail`.
To call methods from both the parent and child class:
python
puppy = Puppy(“Charlie”)
print(puppy.bark()) # Output: Charlie says Woof!
print(puppy.wag_tail()) # Output: Charlie is wagging its tail!
This demonstrates how classes can be extended, allowing for greater flexibility and code reuse in Python programming.
Understanding Class Invocation in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To call a class in Python, one must first instantiate it by creating an object. This is done by using the class name followed by parentheses, which can include any necessary arguments defined in the class’s constructor.”
Michael Chen (Python Developer, Open Source Advocate). “When calling a class in Python, it is essential to understand the difference between calling the class itself and calling its methods. The class can be called to create an instance, while methods can be invoked on that instance to perform specific actions.”
Sarah Johnson (Technical Writer, Python Programming Journal). “Properly calling a class involves not just instantiation but also understanding inheritance and how it affects method resolution. This is crucial for utilizing polymorphism effectively in your Python applications.”
Frequently Asked Questions (FAQs)
How do I define a class in Python?
To define a class in Python, use the `class` keyword followed by the class name and a colon. Include an `__init__` method to initialize attributes. For example:
python
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
How do I create an instance of a class in Python?
To create an instance of a class, call the class name followed by parentheses. For example:
python
my_instance = MyClass(‘value’)
How can I access attributes of a class instance?
Access attributes using dot notation. For example, if you have an instance `my_instance`, you can access its attribute like this:
python
print(my_instance.attribute)
What is the purpose of the `self` parameter in a class method?
The `self` parameter refers to the instance of the class itself. It allows access to instance attributes and methods within class methods. It must be the first parameter of any instance method.
Can I call a class method without creating an instance?
Yes, you can call a class method without creating an instance by using the class name followed by the method name. However, the method must be defined as a class method using the `@classmethod` decorator. For example:
python
class MyClass:
@classmethod
def my_class_method(cls):
return ‘Hello’
MyClass.my_class_method()
What is the difference between instance methods and class methods?
Instance methods operate on an instance of the class and can access instance variables. Class methods operate on the class itself and can access class variables. Instance methods require an instance to be called, while class methods can be called on the class directly.
In Python, calling a class involves creating an instance of that class by using its constructor, which is defined by the `__init__` method. This process allows you to access the class’s attributes and methods, enabling you to utilize the functionality encapsulated within the class. To call a class, you simply use the class name followed by parentheses, optionally passing any required arguments that the constructor expects.
Understanding how to call a class is fundamental to object-oriented programming in Python. It allows developers to create reusable code components that can model real-world entities. By instantiating a class, you can maintain state and behavior specific to that instance, which promotes better organization and modularity in your codebase.
Moreover, leveraging classes effectively can lead to improved code maintainability and scalability. By encapsulating data and behavior, classes facilitate the implementation of complex systems while keeping the code clean and manageable. Therefore, mastering the technique of calling classes is essential for any Python developer aiming to write efficient and effective object-oriented code.
Author Profile
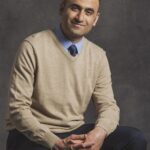
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?