How Can You Effectively Call a Dictionary in Python?
In the world of programming, dictionaries are one of the most versatile and powerful data structures available, especially in Python. Imagine having the ability to store and retrieve data in a way that mimics real-world relationships—this is precisely what dictionaries allow you to do. Whether you’re managing a collection of user profiles, tracking inventory items, or simply organizing data for easy access, understanding how to call a dictionary in Python is a fundamental skill that can elevate your coding capabilities.
At its core, a dictionary in Python is an unordered collection of key-value pairs, where each key serves as a unique identifier for its corresponding value. This structure not only facilitates efficient data retrieval but also enhances code readability and maintainability. Calling a dictionary involves accessing its elements using these keys, which opens up a world of possibilities for data manipulation and retrieval.
As we delve deeper into the intricacies of calling dictionaries, we will explore various methods and techniques that can simplify your coding experience. From basic access patterns to more advanced functionalities, understanding how to interact with dictionaries will empower you to harness their full potential in your Python projects. Get ready to unlock the secrets of this essential data structure and take your programming skills to the next level!
Accessing Dictionary Elements
In Python, dictionaries are collections of key-value pairs. You can access the values stored in a dictionary by referencing their corresponding keys. The syntax for accessing a value is straightforward:
“`python
value = my_dict[key]
“`
If `my_dict` is defined as follows:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
“`
You can retrieve the value associated with the key `’name’`:
“`python
print(my_dict[‘name’]) Output: Alice
“`
Checking for Key Existence
Before accessing a dictionary value, it is often prudent to check if the key exists to avoid `KeyError`. You can do this using the `in` keyword:
“`python
if key in my_dict:
value = my_dict[key]
else:
value = None
“`
Alternatively, the `get()` method provides a safe way to access dictionary values:
“`python
value = my_dict.get(key, default_value)
“`
The `default_value` is returned if the key does not exist, ensuring your program runs smoothly.
Iterating Over a Dictionary
You can iterate through a dictionary to access its keys, values, or key-value pairs. The following methods are commonly used:
- Iterating over keys:
“`python
for key in my_dict:
print(key)
“`
- Iterating over values:
“`python
for value in my_dict.values():
print(value)
“`
- Iterating over key-value pairs:
“`python
for key, value in my_dict.items():
print(key, value)
“`
Dictionary Methods Overview
Python dictionaries come with a variety of built-in methods that facilitate various operations. Below is a table summarizing some of the most commonly used dictionary methods:
Method | Description |
---|---|
clear() | Removes all items from the dictionary. |
copy() | Returns a shallow copy of the dictionary. |
pop(key) | Removes the specified key and returns its value. |
popitem() | Removes and returns the last inserted key-value pair. |
update(other_dict) | Updates the dictionary with elements from another dictionary. |
These methods enhance the functionality of dictionaries, enabling more efficient data management and manipulation.
The ability to access, check, and iterate over dictionary elements is fundamental in Python programming. Understanding these operations allows for effective data handling within applications.
Accessing Dictionary Elements
In Python, dictionaries are collections of key-value pairs that allow for efficient data retrieval. To call or access elements in a dictionary, you can use the following methods:
- Using Square Brackets: The most common way to access a value is by using its key inside square brackets.
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
print(my_dict[‘name’]) Output: Alice
“`
- Using the `get()` Method: This method provides a way to access values without raising an error if the key does not exist.
“`python
print(my_dict.get(‘age’)) Output: 30
print(my_dict.get(‘height’, ‘Not Found’)) Output: Not Found
“`
Iterating Through a Dictionary
To process or display all elements in a dictionary, iteration is necessary. You can do this in several ways:
- Iterating Over Keys: The default iteration will yield the keys.
“`python
for key in my_dict:
print(key, my_dict[key])
“`
- Iterating Over Values: Use the `.values()` method to iterate over values directly.
“`python
for value in my_dict.values():
print(value)
“`
- Iterating Over Key-Value Pairs: The `.items()` method allows you to access both keys and values.
“`python
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
Checking for Key Existence
Before accessing a value, it is often necessary to check if a key exists in the dictionary. This can prevent `KeyError` exceptions.
- Using the `in` Keyword: This is the most straightforward way to check for a key.
“`python
if ‘name’ in my_dict:
print(“Name exists in the dictionary.”)
“`
Updating Dictionary Values
Updating values in a dictionary can be done simply by assigning a new value to an existing key.
- Direct Assignment:
“`python
my_dict[‘age’] = 31 Update age to 31
“`
- Using the `update()` Method: This allows for updating multiple key-value pairs at once.
“`python
my_dict.update({‘name’: ‘Bob’, ‘height’: 175})
“`
Removing Elements from a Dictionary
To remove elements from a dictionary, you can use several methods:
- Using `del` Statement: This removes a key-value pair.
“`python
del my_dict[‘age’]
“`
- Using the `pop()` Method: This method removes a key and returns its value, which can be useful.
“`python
age = my_dict.pop(‘age’, ‘Key not found’)
“`
- Using the `popitem()` Method: This method removes the last inserted key-value pair.
“`python
last_item = my_dict.popitem()
“`
Dictionary Comprehensions
Python supports dictionary comprehensions, which provide a concise way to create dictionaries.
- Basic Syntax:
“`python
squares = {x: x**2 for x in range(5)} Creates a dictionary of squares
“`
- Using Conditions:
“`python
even_squares = {x: x**2 for x in range(10) if x % 2 == 0}
“`
These methods and techniques offer a robust foundation for working with dictionaries in Python, enabling efficient data manipulation and retrieval.
Expert Insights on Accessing Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To call a dictionary in Python, one must first ensure that the dictionary is defined. You can access its values using the key within square brackets or the `.get()` method, which is particularly useful for avoiding KeyErrors.”
Michael Chen (Python Developer, CodeCrafters). “Utilizing dictionaries effectively in Python involves understanding their structure. When you call a dictionary, remember that keys must be unique, and accessing a value is straightforward, making dictionaries an excellent choice for associative arrays.”
Sarah Thompson (Data Scientist, Analytics Hub). “In Python, calling a dictionary is not just about retrieving values; it’s also about leveraging its methods. For instance, using `.keys()`, `.values()`, and `.items()` can provide a comprehensive understanding of the data stored within the dictionary.”
Frequently Asked Questions (FAQs)
How do I create a dictionary in Python?
To create a dictionary in Python, use curly braces `{}` with key-value pairs separated by colons. For example: `my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}`.
How can I access a value in a dictionary?
You can access a value in a dictionary by referencing its key within square brackets. For example: `value = my_dict[‘key1’]`.
What happens if I try to access a key that does not exist in the dictionary?
If you attempt to access a non-existent key, Python raises a `KeyError`. To avoid this, you can use the `get()` method, which returns `None` or a specified default value instead.
How do I add a new key-value pair to an existing dictionary?
To add a new key-value pair, simply assign a value to a new key using square brackets. For example: `my_dict[‘key3’] = ‘value3’`.
Can I update the value of an existing key in a dictionary?
Yes, you can update the value of an existing key by assigning a new value to that key. For instance: `my_dict[‘key1’] = ‘new_value1’`.
How do I iterate through a dictionary in Python?
You can iterate through a dictionary using a `for` loop. To access keys, use `for key in my_dict:`. To access key-value pairs, use `for key, value in my_dict.items():`.
In Python, a dictionary is a built-in data structure that allows for the storage of key-value pairs. To call or access a dictionary, you can utilize its keys to retrieve the corresponding values. This is done by using square brackets or the `get()` method, which provides a more flexible approach by allowing you to specify a default value if the key does not exist. Understanding how to effectively call a dictionary is essential for efficient data manipulation and retrieval in Python programming.
One of the key takeaways is the importance of using the correct syntax when accessing dictionary elements. The square bracket notation is straightforward, but it is crucial to ensure that the key exists to avoid a `KeyError`. The `get()` method serves as a safer alternative, particularly in scenarios where the presence of a key is uncertain. Additionally, dictionaries can be nested, allowing for complex data structures that can be accessed through multiple keys.
Moreover, dictionaries in Python are mutable, meaning that you can change, add, or remove key-value pairs after the dictionary has been created. This flexibility makes dictionaries an excellent choice for dynamic data storage. Overall, mastering how to call and manipulate dictionaries is fundamental for any Python developer, as they are widely used in various applications, from data
Author Profile
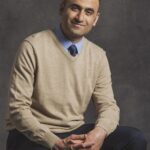
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?