How Can You Effectively Catch Errors in Axios Interceptors?
In the world of web development, managing API calls effectively is crucial for creating seamless user experiences. Axios, a popular promise-based HTTP client for JavaScript, simplifies this process with its powerful interceptors. These interceptors allow developers to intercept requests or responses before they are handled by `then` or `catch`, providing a perfect opportunity to manage errors gracefully. But how do you catch and handle those errors effectively within an interceptor? This article will guide you through the intricacies of error handling in Axios interceptors, ensuring your applications remain robust and user-friendly.
When working with Axios, interceptors serve as a powerful tool for customizing request and response handling. They can be particularly useful for logging errors, modifying requests, or even implementing authentication checks. However, with great power comes great responsibility—especially when it comes to error management. Understanding how to catch and handle errors in interceptors is essential for maintaining the integrity of your application and providing a smooth user experience.
In this article, we will explore the mechanics of Axios interceptors, focusing on how to effectively catch errors that may arise during API calls. By mastering this skill, you will not only enhance your error handling capabilities but also improve your overall development workflow. Whether you’re a seasoned developer or just starting, the insights shared
Catching Errors in Axios Interceptors
When working with Axios, interceptors provide a powerful way to intercept requests or responses before they are handled by `then` or `catch`. Capturing errors in these interceptors is essential for robust error handling and improved user experience. Here’s how to effectively manage error catching in Axios interceptors.
Setting Up Axios Interceptors
To create an interceptor, you can use `axios.interceptors.request.use` for requests and `axios.interceptors.response.use` for responses. Here’s how to set them up:
“`javascript
axios.interceptors.request.use(
(config) => {
// Modify request config before sending
return config;
},
(error) => {
// Handle request error
return Promise.reject(error);
}
);
axios.interceptors.response.use(
(response) => {
// Handle successful response
return response;
},
(error) => {
// Handle response error
return Promise.reject(error);
}
);
“`
In the above code, the second function in both `use` methods is responsible for catching errors. This allows you to implement custom error handling logic.
Handling Errors in Response Interceptor
In the response interceptor, you can catch errors and perform specific actions, such as logging or displaying notifications. Here’s an enhanced example:
“`javascript
axios.interceptors.response.use(
(response) => {
return response;
},
(error) => {
// Error handling logic
const { response } = error;
if (response) {
console.error(`Error Status: ${response.status}`);
console.error(`Error Data: ${response.data}`);
// Custom notification logic
} else {
console.error(“Network Error:”, error.message);
}
return Promise.reject(error);
}
);
“`
This code snippet demonstrates how to differentiate between server errors (where `response` is defined) and network errors.
Custom Error Handling Strategies
Implementing custom strategies can enhance the error handling experience. Here are some common approaches:
- Logging: Send error details to a logging service for further analysis.
- User Notifications: Use a UI library to display error messages to users.
- Retry Logic: Automatically retry requests upon failure, especially for network errors.
Error Handling Table
The following table summarizes different error types and suggested handling approaches:
Error Type | Suggested Action |
---|---|
Network Error | Display a user-friendly message and possibly retry the request. |
4xx Errors | Inform the user of the problem; log details for debugging. |
5xx Errors | Notify the user of a server issue; log for server-side investigation. |
By implementing these strategies in your Axios interceptors, you can effectively manage errors and enhance the reliability of your application.
Understanding Axios Interceptors
Axios interceptors allow developers to intercept requests or responses before they are handled by `then` or `catch`. This feature is particularly useful for adding headers, logging requests, or handling errors globally.
Setting Up an Axios Interceptor
To set up an interceptor, you need to use the `axios.interceptors` property. Below is a basic example of how to create an interceptor for both requests and responses.
“`javascript
import axios from ‘axios’;
axios.interceptors.request.use(
config => {
// Modify request config here
return config;
},
error => {
// Handle request error
return Promise.reject(error);
}
);
axios.interceptors.response.use(
response => {
// Handle successful response
return response;
},
error => {
// Handle response error
return Promise.reject(error);
}
);
“`
Catching Errors in Axios Interceptors
Catching errors in interceptors can be done in the response interceptor. Here are the steps:
- Access the error object: The error object contains information about the error that occurred during the request.
- Log or handle the error: You can log the error or display a user-friendly message.
- Return a rejected promise: This allows the error to be propagated to the calling function.
Error Handling Example
Here’s an example that showcases how to catch and handle errors within an Axios response interceptor:
“`javascript
axios.interceptors.response.use(
response => {
return response;
},
error => {
// Check the error response
if (error.response) {
// The request was made and the server responded with a status code
console.error(‘Error Response:’, error.response.data);
console.error(‘Status:’, error.response.status);
} else if (error.request) {
// The request was made but no response was received
console.error(‘Error Request:’, error.request);
} else {
// Something happened in setting up the request
console.error(‘Error Message:’, error.message);
}
return Promise.reject(error);
}
);
“`
Common Error Handling Scenarios
When catching errors in interceptors, consider the following scenarios:
- Network Errors: Handle cases where the network request fails entirely.
- Server Errors: Manage responses with status codes in the 5xx range.
- Client Errors: Handle responses with status codes in the 4xx range.
Best Practices for Error Handling
To enhance error handling in Axios interceptors, consider these best practices:
- Centralized Error Logging: Implement a logging mechanism to centralize error reporting.
- User Notifications: Display notifications to users for critical errors.
- Retry Logic: Implement retry logic for transient errors.
- Graceful Degradation: Provide fallback options when requests fail.
Best Practice | Description |
---|---|
Centralized Error Logging | Log errors to a centralized service or file |
User Notifications | Inform users of errors gracefully |
Retry Logic | Automatically retry failed requests |
Graceful Degradation | Offer alternative features when errors occur |
Implementing error handling in Axios interceptors is essential for building robust applications. By following the outlined methods and best practices, developers can ensure that their applications handle errors effectively and provide a seamless user experience.
Expert Strategies for Error Handling in Axios Interceptors
Jessica Lane (Senior Software Engineer, Tech Innovations Inc.). “To effectively catch errors in Axios interceptors, it’s crucial to implement a centralized error handling mechanism. This allows you to intercept responses and handle errors uniformly, ensuring that all API errors are processed consistently across your application.”
Michael Chen (Lead Frontend Developer, CodeCraft Solutions). “Utilizing Axios interceptors for error handling not only improves code readability but also enhances maintainability. I recommend logging errors in the response interceptor and providing user-friendly feedback, which can be achieved by customizing the error messages based on the status codes received.”
Sarah Patel (Full Stack Developer, WebTech Dynamics). “Implementing a retry mechanism within your Axios interceptors can significantly improve user experience during transient errors. By catching errors and retrying the request a limited number of times, you can reduce the likelihood of user disruption and maintain application stability.”
Frequently Asked Questions (FAQs)
How can I set up an error interceptor in Axios?
You can set up an error interceptor in Axios by using the `axios.interceptors.response.use()` method. This method takes two arguments: the first is a function that handles successful responses, and the second is a function that handles errors. For example:
“`javascript
axios.interceptors.response.use(
response => response,
error => {
// Handle the error
return Promise.reject(error);
}
);
“`
What is the purpose of using an error interceptor in Axios?
The purpose of using an error interceptor is to centralize error handling for all Axios requests. This allows you to manage error responses consistently, log errors, or perform specific actions based on the error type without duplicating code in each request.
Can I access the original request in the error interceptor?
Yes, you can access the original request in the error interceptor. The error object passed to the interceptor contains a `config` property, which holds the configuration of the original request, including headers, URL, and other settings.
How do I differentiate between different types of errors in an interceptor?
You can differentiate between error types by examining the properties of the error object, such as `error.response`, `error.request`, and `error.message`. For instance, if `error.response` exists, it indicates a response was received but there was an error (like a 404 or 500 status code).
Is it possible to retry a request after catching an error in the interceptor?
Yes, it is possible to retry a request after catching an error in the interceptor. You can implement a retry logic by checking the error type and conditionally making a new request within the error handler. Ensure to handle potential infinite loops by setting a maximum retry limit.
How do I handle global error notifications in an Axios interceptor?
To handle global error notifications, you can integrate a notification library within the error interceptor. When an error is caught, you can trigger a notification with the error message or status. This provides users with feedback without interrupting the application flow.
In summary, catching errors in Axios interceptors is a crucial aspect of managing HTTP requests and responses effectively. Axios provides a robust mechanism to handle errors globally through its interceptors, which allows developers to centralize error handling logic. By implementing error-catching logic within the response interceptor, developers can ensure that any errors encountered during API calls are processed uniformly, leading to a more maintainable codebase.
One of the key takeaways is the importance of distinguishing between different types of errors. Axios interceptors can capture network errors, server errors, and client-side errors, allowing developers to implement tailored responses for each scenario. For instance, handling a 404 error differently from a 500 error can enhance user experience and provide clearer feedback. This differentiation can be achieved by examining the error object within the interceptor and applying conditional logic based on the status code.
Additionally, integrating logging mechanisms within interceptors can aid in debugging and monitoring application performance. By logging errors to a centralized service or console, developers can gain insights into the frequency and types of errors occurring, which can inform future improvements. Overall, leveraging Axios interceptors for error handling not only streamlines the process but also contributes to building more resilient applications.
Author Profile
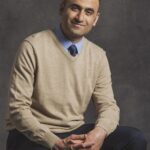
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?