How Can You Change a Character in a String Using Python?
In the world of programming, strings are one of the most fundamental data types, serving as the backbone for text manipulation and data representation. Whether you’re crafting a simple application or developing complex algorithms, the ability to modify strings is essential. Among the myriad of operations you can perform on strings, changing a character within them is a common yet crucial task. This article will guide you through various techniques to effectively alter characters in strings using Python, one of the most popular programming languages today.
Strings in Python are immutable, meaning that once they are created, their content cannot be changed directly. This characteristic may initially seem limiting, but it opens up a wealth of creative solutions for string manipulation. To change a character in a string, you will need to explore methods that involve creating new strings based on the original. This can be achieved through indexing, slicing, and the use of built-in functions, allowing you to customize strings to meet your specific needs.
As we delve deeper into this topic, we will uncover practical examples and best practices for changing characters in strings. From simple replacements to more complex transformations, you’ll discover how to harness the power of Python to manipulate strings efficiently. Whether you’re a beginner looking to enhance your coding skills or an experienced developer seeking to refine your techniques, this guide will equip you
Methods for Changing a Character in a String
In Python, strings are immutable, meaning that once a string is created, its contents cannot be changed directly. However, there are several techniques you can utilize to change a character in a string effectively. Below are some common methods.
Using String Slicing
String slicing allows you to create a new string by concatenating parts of the original string with the new character. This method is straightforward and commonly used.
Example:
“`python
original_string = “hello”
index_to_change = 1
new_character = “a”
Change character at index 1
new_string = original_string[:index_to_change] + new_character + original_string[index_to_change + 1:]
print(new_string) Output: “hallo”
“`
In this example, the string is divided into three parts:
- The substring before the index.
- The new character.
- The substring after the index.
Using the `replace()` Method
The `replace()` method allows you to replace all occurrences of a specified character or substring with another character or substring. This is useful when you want to change multiple instances of a character.
Example:
“`python
original_string = “banana”
new_string = original_string.replace(“a”, “o”)
print(new_string) Output: “bonono”
“`
Using List Conversion
Another approach involves converting the string to a list of characters, making the desired change, and then joining the list back into a string. This method is particularly useful when you need to make multiple changes.
Example:
“`python
original_string = “world”
char_list = list(original_string)
char_list[2] = “r” Changing ‘r’ to ‘l’
new_string = ”.join(char_list)
print(new_string) Output: “wold”
“`
Comparison of Methods
The following table summarizes the advantages and disadvantages of each method:
Method | Advantages | Disadvantages |
---|---|---|
String Slicing | Simple and clear for single character changes. | Requires creating a new string; may be less efficient for large strings. |
`replace()` Method | Effective for multiple replacements. | Replaces all occurrences, not just specific indices. |
List Conversion | Flexible for multiple or complex changes. | More verbose; involves additional steps. |
These methods provide a variety of options for changing characters in strings, allowing for both simple and complex modifications based on your specific needs.
Methods to Change a Character in a String
In Python, strings are immutable, meaning you cannot change them directly. Instead, you can create a new string based on the modifications you want to apply. Below are several methods for changing a character in a string.
Using String Slicing
String slicing allows you to create a new string by concatenating parts of the original string with the desired change. Here’s how you can do it:
“`python
original_string = “hello”
index_to_change = 1
new_character = “a”
Create a new string
modified_string = original_string[:index_to_change] + new_character + original_string[index_to_change + 1:]
print(modified_string) Output: “hallo”
“`
Explanation:
- `original_string[:index_to_change]` gets the substring before the character to change.
- `new_character` is the character you want to insert.
- `original_string[index_to_change + 1:]` retrieves the substring after the character to change.
Using the String `replace()` Method
The `replace()` method can be used to replace all occurrences of a specified character with a new character. This method does not allow for selective index-based changes but is useful for bulk replacements.
“`python
original_string = “hello”
modified_string = original_string.replace(“e”, “a”)
print(modified_string) Output: “hallo”
“`
Note: If you want to replace only the first occurrence, you can specify the count:
“`python
modified_string = original_string.replace(“l”, “r”, 1)
print(modified_string) Output: “herlo”
“`
Using List Conversion
Converting the string to a list allows for mutable operations. After making changes, the list can be converted back to a string.
“`python
original_string = “hello”
index_to_change = 1
new_character = “a”
Convert to list
string_as_list = list(original_string)
string_as_list[index_to_change] = new_character
Convert back to string
modified_string = ”.join(string_as_list)
print(modified_string) Output: “hallo”
“`
Using a Loop for Selective Changes
If you need to change characters based on certain conditions (e.g., only changing vowels), you can use a loop.
“`python
original_string = “hello”
new_character = “x”
modified_string = “”
for char in original_string:
if char in “aeiou”:
modified_string += new_character
else:
modified_string += char
print(modified_string) Output: “hxllx”
“`
Summary of Methods
Method | Description | Example Usage |
---|---|---|
String Slicing | Creates a new string by combining slices | `original_string[:index] + new_char + original_string[index + 1:]` |
`replace()` | Replaces all or specified occurrences of a character | `original_string.replace(“old”, “new”, count)` |
List Conversion | Converts string to a list for mutable operations | `list(original_string)[index] = new_char` |
Loop for Conditions | Iterates and modifies based on specific criteria | `for char in original_string: …` |
Each method has its unique advantages depending on the context and requirements of the modification needed.
Expert Insights on Modifying Strings in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations). “To change a character in a string in Python, one must remember that strings are immutable. A common approach is to convert the string into a list, modify the desired character, and then join it back into a string. This method ensures that the original string remains unchanged while allowing for the necessary modifications.”
Mark Thompson (Python Developer Advocate, Tech Solutions). “Using string slicing is a highly efficient way to change a character in a string. By slicing the string around the character you wish to replace and concatenating it with the new character, you can achieve the desired result in a clean and readable manner. This technique is both elegant and Pythonic.”
Lisa Chen (Data Scientist, Analytics Corp). “For those who need to perform multiple character replacements, utilizing the `str.replace()` method can be very effective. This built-in function allows for replacing all occurrences of a specified character with a new one, streamlining the process and enhancing code readability, especially in data manipulation tasks.”
Frequently Asked Questions (FAQs)
How can I change a specific character in a string in Python?
You can change a specific character in a string by converting the string into a list, modifying the desired index, and then joining the list back into a string. For example:
“`python
s = “hello”
s_list = list(s)
s_list[1] = ‘a’
new_string = ”.join(s_list) Result: ‘hallo’
“`
Is it possible to change multiple characters in a string at once?
Yes, you can change multiple characters by using a loop or a comprehension to modify the desired indices. For instance:
“`python
s = “hello”
s_list = list(s)
for index in [1, 3]:
s_list[index] = ‘a’
new_string = ”.join(s_list) Result: ‘hallo’
“`
Can I use string slicing to change a character in a string?
String slicing allows you to create a new string by combining parts of the original string. You can replace a character using slicing like this:
“`python
s = “hello”
new_string = s[:1] + ‘a’ + s[2:] Result: ‘hallo’
“`
What method can I use to replace all occurrences of a character in a string?
You can use the `str.replace()` method to replace all occurrences of a character in a string. For example:
“`python
s = “hello”
new_string = s.replace(‘l’, ‘x’) Result: ‘hexxo’
“`
Are strings mutable or immutable in Python?
Strings in Python are immutable, meaning you cannot change them directly. Any modification creates a new string instead of altering the original.
What are some common errors when trying to change a character in a string?
Common errors include attempting to assign a value to an index of a string directly, which raises a `TypeError`, and trying to access an index that is out of range, which raises an `IndexError`.
In Python, strings are immutable, meaning that once they are created, their contents cannot be changed directly. To change a character in a string, one must create a new string that reflects the desired modifications. This can be achieved through various methods, such as string slicing, concatenation, or using built-in functions. Understanding these techniques is essential for effective string manipulation in Python programming.
One common approach to change a character in a string is to use slicing. By slicing the string into parts before and after the character to be changed, you can concatenate these parts with the new character. For example, if you want to replace the character at a specific index, you can slice the string up to that index, add the new character, and then append the remainder of the string. This method is straightforward and leverages Python’s powerful string manipulation capabilities.
Another valuable insight is the use of the `replace()` method, which allows for replacing all occurrences of a specified substring with a new substring. This method is particularly useful when multiple characters need to be changed throughout the string. However, it is important to note that this method will not modify the original string but will return a new string with the changes applied.
In summary
Author Profile
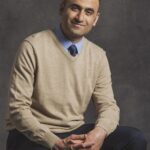
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?