How Can You Change CSS Using JavaScript?
### Introduction
In the dynamic world of web development, the ability to manipulate styles on-the-fly can transform a static webpage into an interactive experience. Whether you’re looking to create a responsive design, enhance user engagement, or simply add a touch of flair, changing CSS with JavaScript opens up a realm of possibilities. This powerful combination allows developers to respond to user actions, adapt layouts, and create visually stunning effects that can captivate audiences. If you’ve ever wondered how to harness the full potential of CSS and JavaScript together, you’re in the right place.
Changing CSS with JavaScript is not just a technical skill; it’s a creative tool that empowers developers to bring their visions to life. By leveraging JavaScript, you can dynamically alter styles, toggle classes, and even create animations that respond to user interactions. This seamless integration between the two languages enables a more fluid and responsive user experience, making it essential for modern web applications.
As we delve deeper into this topic, we’ll explore various methods and techniques for manipulating CSS through JavaScript. From simple style changes to complex animations, understanding how to effectively use JavaScript for CSS manipulation can elevate your web development skills and enhance the overall functionality of your projects. Get ready to unlock the potential of your web pages and engage
Accessing and Modifying CSS Properties
To change CSS styles using JavaScript, you typically interact with the Document Object Model (DOM). Each HTML element can be selected and manipulated, allowing you to modify its style properties dynamically. The most common method for accessing CSS properties is through the `style` property of a DOM element.
You can change individual CSS properties by directly assigning a new value to a specific property. Here’s an example:
javascript
document.getElementById(“myElement”).style.backgroundColor = “blue”;
In this case, the background color of the element with the ID `myElement` is changed to blue. You can also set multiple properties at once:
javascript
const element = document.getElementById(“myElement”);
element.style.color = “white”;
element.style.fontSize = “20px”;
element.style.margin = “10px”;
Using CSS Classes for Style Changes
Instead of modifying individual CSS properties, you can apply or remove CSS classes. This approach is more efficient, especially when dealing with multiple styles. Here’s how you can toggle a class:
javascript
const element = document.getElementById(“myElement”);
element.classList.add(“newClass”); // Add a class
element.classList.remove(“oldClass”); // Remove a class
element.classList.toggle(“active”); // Toggle a class
Utilizing classes helps maintain cleaner code and allows for better separation of concerns, where CSS handles styling and JavaScript manages behavior.
Dynamic Style Changes Based on Conditions
JavaScript allows you to dynamically change styles based on conditions. For example, you might want to change the styling of an element based on user interaction:
javascript
const button = document.getElementById(“toggleButton”);
const box = document.getElementById(“box”);
button.addEventListener(“click”, function() {
if (box.style.display === “none”) {
box.style.display = “block”;
} else {
box.style.display = “none”;
}
});
In this example, clicking the button toggles the visibility of a box.
Table of Common CSS Properties and JavaScript Syntax
CSS Property | JavaScript Syntax |
---|---|
background-color | element.style.backgroundColor = “red”; |
font-size | element.style.fontSize = “16px”; |
margin | element.style.margin = “20px”; |
display | element.style.display = “none”; |
color | element.style.color = “blue”; |
By understanding how to manipulate CSS through JavaScript, you can create interactive and visually dynamic web applications that respond to user actions and other events.
Accessing and Modifying CSS Styles
To change CSS styles using JavaScript, you first need to access the DOM elements you wish to modify. This can be achieved through various methods such as `getElementById`, `getElementsByClassName`, or `querySelector`. Once you have a reference to the desired element, you can manipulate its style properties directly.
Example: Changing a Single Style Property
javascript
document.getElementById(‘myElement’).style.color = ‘blue’;
In this example, the text color of the element with the ID `myElement` is changed to blue.
Changing Multiple CSS Properties
To change multiple CSS properties at once, you can either set each property individually or use the `cssText` property to modify the entire style string.
Setting Multiple Properties Individually:
javascript
let element = document.getElementById(‘myElement’);
element.style.backgroundColor = ‘yellow’;
element.style.fontSize = ’20px’;
element.style.border = ‘1px solid black’;
Using `cssText`:
javascript
document.getElementById(‘myElement’).style.cssText = ‘background-color: yellow; font-size: 20px; border: 1px solid black;’;
Using Classes to Change Styles
Another effective method of changing CSS styles is by adding or removing classes. This approach is beneficial for maintaining cleaner JavaScript code and utilizing existing CSS rules.
Adding a Class:
javascript
document.getElementById(‘myElement’).classList.add(‘newClass’);
Removing a Class:
javascript
document.getElementById(‘myElement’).classList.remove(‘oldClass’);
Toggling a Class:
javascript
document.getElementById(‘myElement’).classList.toggle(‘active’);
Responsive CSS Changes with JavaScript
JavaScript can be particularly useful for responsive design, allowing you to apply different styles based on the viewport size or other conditions. You can listen for events such as `resize` to trigger style changes dynamically.
Example of Responsive Style Changes:
javascript
window.addEventListener(‘resize’, function() {
let element = document.getElementById(‘myElement’);
if (window.innerWidth < 600) {
element.style.fontSize = '14px';
} else {
element.style.fontSize = '20px';
}
});
Using JavaScript Libraries
For more complex style manipulations, consider using JavaScript libraries such as jQuery or frameworks like React, which provide built-in methods for styling.
jQuery Example:
javascript
$(‘#myElement’).css({
‘background-color’: ‘red’,
‘font-size’: ’18px’
});
React Inline Styles:
In React, styles can be applied directly within components using an object.
javascript
const myStyle = {
backgroundColor: ‘green’,
fontSize: ’22px’
};
function MyComponent() {
return
;
}
Performance Considerations
When manipulating CSS through JavaScript, it is crucial to consider performance:
- Minimize DOM accesses to reduce reflows and repaints.
- Batch multiple style changes to limit rendering overhead.
- Use classes for style changes where possible, as it allows for better optimization by the browser.
By understanding these methods and considerations, you can effectively change CSS with JavaScript to enhance user experience and maintainability of your code.
Expert Insights on Changing CSS with JavaScript
Jessica Lin (Front-End Developer, Tech Innovations Inc.). “To effectively change CSS with JavaScript, one must understand the Document Object Model (DOM). By selecting elements using methods like `document.getElementById` or `document.querySelector`, developers can manipulate styles dynamically, allowing for responsive and interactive web designs.”
Michael Carter (Web Development Instructor, Code Academy). “Utilizing JavaScript to alter CSS properties can enhance user experience significantly. For instance, using the `style` property of an HTML element, developers can change attributes such as `backgroundColor` or `display` directly, providing a seamless transition between styles.”
Sarah Thompson (UI/UX Designer, Creative Solutions). “When changing CSS with JavaScript, it is crucial to consider performance implications. Excessive manipulation of styles can lead to reflows and repaints, negatively impacting page performance. Therefore, it is advisable to batch style changes and utilize CSS classes for bulk updates whenever possible.”
Frequently Asked Questions (FAQs)
How can I change the style of an element using JavaScript?
You can change the style of an element by accessing its `style` property in JavaScript. For example, `document.getElementById(‘myElement’).style.color = ‘red’;` changes the text color of the element with the ID `myElement` to red.
What is the difference between inline styles and CSS classes in JavaScript?
Inline styles are applied directly to the element using the `style` property, while CSS classes are defined in a stylesheet and can be added or removed using the `classList` property. Inline styles have higher specificity than classes.
Can I change multiple CSS properties at once with JavaScript?
Yes, you can change multiple CSS properties at once by setting them individually or by using the `cssText` property. For example, `element.style.cssText = ‘color: blue; background-color: yellow;’;` applies both styles simultaneously.
How do I add or remove a CSS class using JavaScript?
To add a CSS class, use `element.classList.add(‘className’);`. To remove a class, use `element.classList.remove(‘className’);`. This method allows for better management of styles without directly manipulating inline styles.
Is it possible to change styles based on events in JavaScript?
Yes, you can change styles based on events by adding event listeners. For example, `element.addEventListener(‘click’, function() { element.style.display = ‘none’; });` hides the element when it is clicked.
What are the performance implications of changing CSS with JavaScript?
Frequent changes to CSS properties can lead to performance issues, especially if they cause reflows or repaints in the browser. It is advisable to batch style changes or use CSS transitions for smoother effects.
In summary, changing CSS with JavaScript is a powerful technique that allows developers to dynamically manipulate the appearance of web pages. By utilizing the Document Object Model (DOM), JavaScript can access and modify CSS properties directly, enabling responsive designs and interactive user experiences. Developers can change styles in various ways, including modifying inline styles, adding or removing CSS classes, and even creating new style rules through JavaScript.
Key takeaways from this discussion include the importance of understanding the different methods available for altering CSS with JavaScript. Each method, whether it be setting styles directly on elements, using class manipulation, or leveraging CSS variables, has its own advantages and use cases. Additionally, performance considerations should be taken into account, as frequent style changes can lead to reflows and repaints that may affect page performance.
Ultimately, mastering the integration of CSS and JavaScript not only enhances the visual appeal of web applications but also improves user engagement. As web technologies continue to evolve, staying informed about best practices for manipulating styles will remain essential for developers aiming to create modern, interactive web experiences.
Author Profile
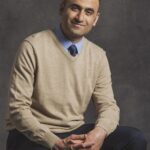
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?