How Can You Change the Working Directory in Python?
When working with Python, one of the fundamental skills every programmer should master is the ability to manage and manipulate the working directory. Whether you’re developing a small script or a large-scale application, understanding how to change the working directory can significantly enhance your workflow and streamline your coding process. The working directory serves as the default location where Python looks for files to read and where it saves files to write. By mastering this concept, you can avoid common pitfalls related to file paths and ensure your scripts run smoothly, regardless of their location.
Changing the working directory in Python is not just a matter of convenience; it’s a crucial aspect of file management that can affect how your code interacts with various resources. Python provides built-in functions that allow you to easily navigate between directories, making it simple to access files and organize your projects effectively. This flexibility is particularly important for collaborative projects or when deploying applications across different environments, where file paths may vary.
In this article, we will delve into the methods available for changing the working directory in Python, explore best practices for managing file paths, and discuss common scenarios where this knowledge can come in handy. Whether you’re a beginner looking to understand the basics or an experienced developer seeking to refine your skills, this guide will equip you with the tools necessary to handle
Changing the Working Directory in Python
To change the working directory in Python, you can use the `os` module, which provides a portable way of using operating system-dependent functionality, such as changing the current working directory. The specific function used for this purpose is `os.chdir(path)`, where `path` is the desired directory you want to switch to.
Here are the steps to change the working directory:
- Import the os module: First, you need to import the `os` module into your Python script.
- Use os.chdir(): Call the `os.chdir()` function with the path to the desired directory.
Example code:
“`python
import os
Change to the desired directory
os.chdir(‘/path/to/directory’)
“`
You can verify the current working directory using `os.getcwd()`, which returns the absolute path of the current working directory.
Common Use Cases
Changing the working directory can be particularly useful in various scenarios:
- File Operations: When you need to read from or write to files located in different directories.
- Organizing Projects: To switch between different project folders without specifying the full path for each file operation.
- Script Execution: Ensuring that scripts are executed in a specific directory context, which can help in managing resource paths.
Example Scenario
Suppose you have a project with the following structure:
“`
project/
│
├── data/
│ └── dataset.csv
│
└── scripts/
└── analysis.py
“`
If you are running `analysis.py` and need to read `dataset.csv`, you would first change the working directory to `data/` before executing file operations.
Example code:
“`python
import os
import pandas as pd
Change to the data directory
os.chdir(‘/path/to/project/data’)
Now you can read the dataset
data = pd.read_csv(‘dataset.csv’)
“`
Handling Errors
When changing directories, it’s essential to handle potential errors that can arise, such as:
- FileNotFoundError: Raised if the specified path does not exist.
- PermissionError: Raised if you do not have permission to access the specified directory.
Here’s an example of how to handle these exceptions:
“`python
import os
try:
os.chdir(‘/path/to/directory’)
except FileNotFoundError:
print(“The specified directory does not exist.”)
except PermissionError:
print(“You do not have permission to change to this directory.”)
“`
Best Practices
- Always check if the directory exists before changing it.
- Use absolute paths to avoid confusion, especially in larger projects.
- Consider using a context manager to temporarily change the working directory if necessary.
Function | Description |
---|---|
os.getcwd() | Returns the current working directory. |
os.chdir(path) | Changes the current working directory to the specified path. |
os.listdir(path) | Lists the files and directories in the specified path. |
Changing the Working Directory in Python
To change the current working directory in Python, you can utilize the `os` module, which provides a way to interact with the operating system.
Using `os.chdir()` Function
The primary method for changing the working directory is through the `os.chdir()` function. This function takes a single argument, which is the path to the desired directory.
Example:
“`python
import os
Change the current working directory to ‘C:/Users/YourUsername/Documents’
os.chdir(‘C:/Users/YourUsername/Documents’)
“`
After executing the above code, the current working directory will be set to the specified path.
Verifying the Current Working Directory
To confirm that the working directory has been changed successfully, you can use the `os.getcwd()` function, which returns the current working directory as a string.
Example:
“`python
current_directory = os.getcwd()
print(“Current Working Directory:”, current_directory)
“`
This will output the path of the current working directory, allowing you to verify the change.
Handling Exceptions
When changing the working directory, it is prudent to handle potential exceptions. For instance, if the specified directory does not exist, a `FileNotFoundError` will be raised. You can manage this using a try-except block.
Example:
“`python
try:
os.chdir(‘C:/Invalid/Path’)
except FileNotFoundError as e:
print(“Error: The specified directory does not exist.”, e)
“`
This approach ensures that your program can gracefully handle errors related to directory changes.
Working with Relative Paths
In addition to absolute paths, you can use relative paths to change the working directory. This is particularly useful when working within a project folder.
Example:
“`python
Change to a subdirectory called ‘data’
os.chdir(‘data’)
“`
This command will change the working directory to the `data` subdirectory relative to the current directory.
Listing Files in the Current Directory
Once the working directory has been changed, you may want to list the files within it. The `os.listdir()` function can be employed for this purpose.
Example:
“`python
files = os.listdir()
print(“Files in the current directory:”, files)
“`
This will output a list of files and directories in the current working directory.
Common Use Cases
Changing the working directory is often useful in scenarios such as:
- Data Processing: When working with datasets stored in specific folders.
- File Management: Organizing output files in designated directories.
- Script Automation: Running scripts that require access to files in various directories.
By understanding how to manipulate the working directory, you can enhance your efficiency in file handling and management within Python.
Expert Insights on Changing Working Directory in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Changing the working directory in Python is crucial for managing file paths effectively. Utilizing `os.chdir()` allows users to set the current working directory dynamically, enabling seamless access to project files without hardcoding paths.”
James Liu (Python Developer and Educator, Code Academy). “It’s important to understand that the working directory can significantly affect file operations in Python. By using `os.getcwd()` in conjunction with `os.chdir()`, developers can ensure they are operating in the correct context, which minimizes errors related to file access.”
Sarah Thompson (Software Engineer, Data Solutions Corp.). “For those working in collaborative environments, changing the working directory programmatically can enhance workflow efficiency. By incorporating relative paths after setting the working directory with `os.chdir()`, teams can maintain consistency across different systems.”
Frequently Asked Questions (FAQs)
How can I change the working directory in Python?
You can change the working directory in Python using the `os` module. Import the module and use `os.chdir(‘path/to/directory’)` to set the desired directory.
What function do I use to get the current working directory?
To retrieve the current working directory, use `os.getcwd()`. This function returns the path of the current working directory as a string.
What happens if I change the working directory in a script?
Changing the working directory affects all subsequent file operations in that script. Any relative file paths will be resolved based on the new working directory.
Can I change the working directory to a network path?
Yes, you can change the working directory to a network path. Ensure that the network path is accessible and properly formatted according to your operating system’s conventions.
Is it possible to change the working directory temporarily in a Python session?
Yes, you can use a context manager to temporarily change the working directory. The `contextlib` module provides a way to create a context manager that restores the original directory after use.
How do I check if a directory exists before changing to it?
You can check if a directory exists using `os.path.exists(‘path/to/directory’)`. This function returns `True` if the directory exists, allowing you to avoid errors when changing directories.
Changing the working directory in Python is a fundamental task that allows developers to manage file paths effectively. The primary method for altering the working directory is through the `os` module, specifically using the `os.chdir()` function. This function takes a string argument that specifies the path to the desired directory. It is crucial to ensure that the path is valid; otherwise, Python will raise an error. Additionally, using `os.getcwd()` allows users to retrieve the current working directory, which can be useful for verification purposes.
Another important aspect to consider is the difference between absolute and relative paths when changing directories. Absolute paths provide the complete location from the root of the file system, while relative paths are based on the current working directory. Understanding these distinctions is vital for effective file management and avoiding potential errors in file handling.
Furthermore, developers should be aware of the implications of changing the working directory within scripts or applications. It can affect how files are accessed and can lead to confusion if not managed carefully. Therefore, it is advisable to document changes to the working directory within code and ensure that any changes are made intentionally and with a clear understanding of their impact on the overall program.
Author Profile
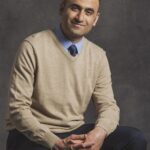
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?