How Can You Change the Y-Axis Scale in Python Matplotlib?
Visualizing data effectively is a cornerstone of data analysis, and one of the most powerful tools at your disposal is Matplotlib, a comprehensive library in Python for creating static, animated, and interactive visualizations. Among the myriad of customization options that Matplotlib offers, adjusting the y-axis scale is crucial for enhancing the clarity and impact of your plots. Whether you’re working with financial data, scientific measurements, or any dataset that requires precise interpretation, mastering the art of y-axis scaling can transform your visual storytelling.
In this article, we will explore the importance of y-axis scaling in data visualization and how it can significantly affect the interpretation of your results. From linear to logarithmic scales, the choice of y-axis can alter the viewer’s perception and understanding of the data being presented. We will delve into the various methods available in Matplotlib for changing the y-axis scale, ensuring that your plots not only convey the intended message but also engage your audience effectively.
As we navigate through the capabilities of Matplotlib, you will discover practical techniques for customizing your plots to suit your analytical needs. Whether you’re a seasoned data scientist or a beginner looking to enhance your visualizations, understanding how to manipulate the y-axis scale will empower you to create more insightful and visually appealing graphics. Get ready to
Changing the Y-Axis Scale in Matplotlib
To modify the y-axis scale in a Matplotlib plot, you can utilize various methods depending on your specific requirements. The most common techniques include setting the limits, scaling the axis to logarithmic values, and adjusting the ticks. Each method serves a distinct purpose and can be applied to enhance data visualization.
Setting Y-Axis Limits
You can set the limits of the y-axis using the `set_ylim()` function or the `ylim()` function. This allows you to focus on a specific range of data, making it easier to interpret.
Example:
“`python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 20, 25, 30, 40]
plt.plot(x, y)
plt.ylim(15, 35) Set y-axis limits
plt.show()
“`
In this example, the y-axis is limited to the range from 15 to 35, omitting data outside this interval.
Using Logarithmic Scale
When your data spans several orders of magnitude, a logarithmic scale may provide a clearer representation. You can set the y-axis to a logarithmic scale using the `set_yscale()` method.
Example:
“`python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 100, 1000, 10000, 100000]
plt.plot(x, y)
plt.yscale(‘log’) Set y-axis to logarithmic scale
plt.show()
“`
This method is particularly useful for visualizing exponential growth or data that changes dramatically across a small range of values.
Adjusting Y-Axis Ticks
You can customize the ticks on the y-axis for better clarity using the `set_yticks()` and `set_yticklabels()` methods. This customization allows you to define where ticks appear and how they are labeled.
Example:
“`python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, 50]
plt.plot(x, y)
plt.yticks([10, 20, 30, 40, 50], [‘Ten’, ‘Twenty’, ‘Thirty’, ‘Forty’, ‘Fifty’]) Custom y-ticks
plt.show()
“`
This customization improves readability, especially when the default labels do not clearly convey the data’s meaning.
Table of Common Y-Axis Adjustments
Adjustment Type | Function/Method | Description |
---|---|---|
Set Limits | plt.ylim(lower, upper) | Defines the lower and upper limits of the y-axis. |
Logarithmic Scale | plt.yscale(‘log’) | Changes the y-axis to a logarithmic scale for better visualization of large ranges. |
Custom Ticks | plt.yticks(ticks, labels) | Sets specific tick locations and their corresponding labels on the y-axis. |
By applying these methods, you can effectively manage the y-axis scale in Matplotlib, tailoring it to the needs of your data visualization.
Adjusting the Y-Axis Scale in Matplotlib
To change the scale of the y-axis in Matplotlib, various methods can be employed depending on the desired effect. Below are the most common approaches.
Setting Fixed Limits
You can set the y-axis limits using the `set_ylim()` method or by passing limits directly to the `ylim()` function.
“`python
import matplotlib.pyplot as plt
plt.plot(x, y)
plt.ylim(0, 10) Sets y-axis limits to 0 and 10
plt.show()
“`
Alternatively, using `set_ylim()`:
“`python
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_ylim(0, 10) Sets y-axis limits
plt.show()
“`
Changing the Scale Type
Matplotlib allows for different scale types such as linear, logarithmic, or symmetric log. To switch to a logarithmic scale, use the `set_yscale()` method:
“`python
plt.plot(x, y)
plt.yscale(‘log’) Changes y-axis to logarithmic scale
plt.show()
“`
For symmetric logarithmic scale:
“`python
plt.yscale(‘symlog’) Changes y-axis to symmetric log scale
plt.show()
“`
Customizing Tick Marks and Labels
You can customize the tick marks and labels on the y-axis using the `set_yticks()` and `set_yticklabels()` methods:
“`python
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_yticks([0, 2, 4, 6, 8, 10]) Custom y-ticks
ax.set_yticklabels([‘0’, ‘Two’, ‘Four’, ‘Six’, ‘Eight’, ‘Ten’]) Custom labels
plt.show()
“`
Using Logarithmic Scale with Custom Base
To specify a logarithmic scale with a custom base, you can pass the `base` parameter to `set_yscale()`:
“`python
plt.plot(x, y)
plt.yscale(‘log’, base=2) Logarithmic scale with base 2
plt.show()
“`
Visualizing Data with Inverted Y-Axis
In some cases, it is beneficial to invert the y-axis. This can be done with the `invert_yaxis()` method:
“`python
plt.plot(x, y)
plt.gca().invert_yaxis() Inverts the y-axis
plt.show()
“`
Combining Multiple Axes with Different Scales
For complex visualizations, you may want to plot multiple y-axes with different scales. This can be achieved using `twinx()`:
“`python
fig, ax1 = plt.subplots()
ax1.plot(x, y1, ‘g-‘) First y-axis
ax1.set_ylabel(‘Y1 Axis’, color=’g’)
ax2 = ax1.twinx() Create a second y-axis
ax2.plot(x, y2, ‘b-‘) Second y-axis
ax2.set_ylabel(‘Y2 Axis’, color=’b’)
plt.show()
“`
Example Summary Table
Method | Code Example | Description |
---|---|---|
Set Fixed Limits | `plt.ylim(0, 10)` | Define specific limits for y-axis. |
Logarithmic Scale | `plt.yscale(‘log’)` | Change to logarithmic scale. |
Custom Tick Marks | `ax.set_yticks([…])` | Define custom tick marks. |
Inverted Y-Axis | `plt.gca().invert_yaxis()` | Invert the y-axis direction. |
Multiple Axes | `ax1.twinx()` | Create a second y-axis. |
These methods provide robust options for customizing the y-axis in Matplotlib, enabling greater flexibility in data visualization.
Expert Insights on Modifying Y-Axis Scale in Matplotlib
Dr. Emily Chen (Data Visualization Specialist, Tech Insights Journal). “To effectively change the y-axis scale in Matplotlib, one can utilize the `set_ylim()` function, allowing for precise control over the range of values displayed. This is crucial for enhancing clarity in data representation.”
Michael Torres (Senior Software Engineer, Data Science Innovations). “When adjusting the y-axis scale, it is important to consider the context of your data. Using logarithmic scales can be beneficial for datasets with exponential growth, which can be easily implemented with `plt.yscale(‘log’)`.”
Lisa Patel (Machine Learning Researcher, AI Analytics Group). “For more advanced customization, the `matplotlib.ticker` module provides various formatting options for the y-axis. This allows for tailored tick marks and labels, enhancing the interpretability of complex datasets.”
Frequently Asked Questions (FAQs)
How do I change the y-axis scale in a Matplotlib plot?
To change the y-axis scale in a Matplotlib plot, use the `plt.ylim()` function to set the limits of the y-axis. For example, `plt.ylim(bottom, top)` sets the lower and upper limits.
Can I change the y-axis to a logarithmic scale in Matplotlib?
Yes, you can change the y-axis to a logarithmic scale by using the `plt.yscale(‘log’)` function. This is useful for visualizing data that spans several orders of magnitude.
How can I customize the ticks on the y-axis in Matplotlib?
You can customize the ticks on the y-axis using the `plt.yticks()` function. This allows you to specify the locations and labels of the ticks, enhancing the readability of your plot.
Is it possible to invert the y-axis in Matplotlib?
Yes, you can invert the y-axis by using the `plt.gca().invert_yaxis()` method. This is particularly useful for certain types of data visualization where higher values need to appear at the bottom.
What should I do if my y-axis labels overlap in Matplotlib?
If y-axis labels overlap, you can adjust the rotation of the labels using `plt.yticks(rotation=angle)` or increase the figure size with `plt.figure(figsize=(width, height))` to provide more space for the labels.
How do I add a secondary y-axis in Matplotlib?
To add a secondary y-axis, use the `twinx()` method. This creates a new y-axis that shares the same x-axis, allowing for the display of different data scales on the same plot.
Changing the y-axis scale in Python’s Matplotlib is a straightforward process that can significantly enhance the readability and interpretability of your data visualizations. Users can manipulate the y-axis scale through various methods, including setting limits, using logarithmic scales, and adjusting tick marks. Each of these methods allows for tailored visualizations that can accommodate different types of data and analytical needs.
One of the primary methods to change the y-axis scale is by using the `set_ylim()` function, which allows users to specify the minimum and maximum values for the y-axis. This is particularly useful when you want to focus on a specific range of data. Additionally, the `yscale()` function enables the use of logarithmic scales, which can be beneficial for datasets that span several orders of magnitude. This approach can help in visualizing relationships that may not be apparent with a linear scale.
Furthermore, customizing the y-axis ticks and labels can enhance clarity. Functions such as `set_yticks()` and `set_yticklabels()` provide control over the appearance of tick marks, allowing for a more informative presentation of the data. Overall, understanding how to manipulate the y-axis scale in Matplotlib empowers users to create more effective and insightful visualizations, catering
Author Profile
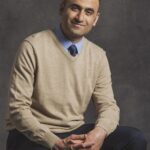
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?