How Can You Easily Check for Duplicates in a Python List?
In the world of programming, data integrity is paramount, and ensuring that your lists are free from duplicates is a critical aspect of maintaining that integrity. Whether you’re processing user inputs, analyzing datasets, or simply organizing information, the presence of duplicate entries can lead to erroneous results and skewed analyses. In Python, a language renowned for its simplicity and versatility, checking for duplicates in a list is not only straightforward but also an essential skill for any developer. This article will delve into various methods to identify and handle duplicates, equipping you with the tools you need to keep your data pristine.
When working with lists in Python, you may encounter situations where duplicate values can disrupt your workflow. Understanding how to efficiently check for these duplicates is crucial, especially in scenarios involving data manipulation or aggregation. Python offers several built-in functionalities and data structures that can help streamline this process, allowing you to focus on the logic of your application rather than getting bogged down by repetitive entries.
From utilizing sets to employing list comprehensions, the techniques available for checking duplicates are as diverse as the challenges they address. Each method presents its own advantages and trade-offs, making it essential to choose the right approach based on your specific needs. As we explore these strategies, you’ll gain insights into not just identifying duplicates, but also
Using Sets to Identify Duplicates
One of the most efficient ways to check for duplicates in a list in Python is by using a set. A set is a data structure that inherently does not allow duplicate elements. By converting a list to a set, you can easily determine if duplicates exist by comparing the length of the list and the set.
“`python
def check_duplicates_with_set(lst):
return len(lst) != len(set(lst))
“`
In this example, if the lengths differ, it indicates the presence of duplicates. This method is both time-efficient and straightforward.
Utilizing Collections for Duplicates
Python’s `collections` module provides a `Counter` class that allows for more detailed analysis of duplicates. The `Counter` object counts the frequency of each element in the list, which can then be used to identify duplicates.
“`python
from collections import Counter
def find_duplicates_with_counter(lst):
counts = Counter(lst)
return {item: count for item, count in counts.items() if count > 1}
“`
This function returns a dictionary containing the duplicate items and their counts, providing more insight into the data.
Looping through the List
Another method to find duplicates is by iterating through the list and maintaining a separate set to track seen elements. This approach is more manual but can be useful in specific scenarios.
“`python
def check_duplicates_with_loop(lst):
seen = set()
duplicates = set()
for item in lst:
if item in seen:
duplicates.add(item)
else:
seen.add(item)
return duplicates
“`
This function will return a set of duplicates found in the list.
Comparison of Methods
The following table summarizes the different methods for checking duplicates in a list:
Method | Complexity | Output | Details |
---|---|---|---|
Using Sets | O(n) | Boolean | Simple length comparison |
Using Counter | O(n) | Dictionary | Counts occurrences of each item |
Looping | O(n) | Set | Tracks seen items and duplicates |
By selecting the appropriate method based on your specific needs, you can efficiently handle duplicate entries in a Python list. Each method offers unique advantages, depending on whether you require just a boolean check, detailed counts, or a simple list of duplicates.
Using Python Sets to Identify Duplicates
One of the most efficient ways to check for duplicates in a list is by utilizing Python’s built-in `set` data structure. A set inherently disallows duplicate entries, which makes it an ideal choice for this task.
To find duplicates using sets, follow these steps:
- Convert the list to a set.
- Compare the length of the list with the length of the set.
- If the lengths are different, duplicates exist.
Here’s a sample code snippet:
“`python
def has_duplicates(lst):
return len(lst) != len(set(lst))
Example usage
my_list = [1, 2, 3, 4, 5, 1]
print(has_duplicates(my_list)) Output: True
“`
Using Collections to Count Duplicates
The `collections` module provides a `Counter` class that can be used to count occurrences of each element in a list. This method allows you to identify duplicates and see how many times each element appears.
Here’s how to implement it:
“`python
from collections import Counter
def find_duplicates(lst):
counts = Counter(lst)
return [item for item, count in counts.items() if count > 1]
Example usage
my_list = [1, 2, 2, 3, 4, 4, 5]
print(find_duplicates(my_list)) Output: [2, 4]
“`
Using List Comprehension
List comprehension can also be used to identify duplicates, although this method may be less efficient for large lists. The idea is to iterate through the list and check if an element appears more than once.
Here’s a code example:
“`python
def check_duplicates(lst):
return list(set([x for x in lst if lst.count(x) > 1]))
Example usage
my_list = [1, 2, 3, 4, 2, 3]
print(check_duplicates(my_list)) Output: [2, 3]
“`
Using a Loop and a Dictionary
For more control over the duplicates found, a loop coupled with a dictionary can be employed. This method allows for tracking the frequency of each element.
Here’s a detailed approach:
“`python
def find_duplicates_with_dict(lst):
seen = {}
duplicates = []
for item in lst:
if item in seen:
seen[item] += 1
else:
seen[item] = 1
for item, count in seen.items():
if count > 1:
duplicates.append(item)
return duplicates
Example usage
my_list = [1, 2, 3, 1, 2, 4]
print(find_duplicates_with_dict(my_list)) Output: [1, 2]
“`
Performance Considerations
Different methods for checking duplicates have varying performance characteristics. Here’s a brief comparison:
Method | Time Complexity | Space Complexity |
---|---|---|
Using sets | O(n) | O(n) |
Using collections.Counter | O(n) | O(n) |
List comprehension | O(n^2) | O(n) |
Loop with dictionary | O(n) | O(n) |
Choosing the right method depends on the size of the list and the specific requirements of your application. For most general purposes, using sets or `Counter` will yield the best performance.
Expert Insights on Checking for Duplicates in Python Lists
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When checking for duplicates in a Python list, using a set is one of the most efficient methods. By converting the list to a set, you automatically remove duplicates, which can then be compared to the original list to identify any missing items.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For larger datasets, I recommend using the collections.Counter class. It provides a straightforward way to count occurrences of each element in the list, allowing you to easily identify duplicates and their frequencies.”
Sarah Patel (Python Developer, Open Source Community). “Another effective approach is to utilize list comprehensions along with a dictionary to track seen items. This method is both memory-efficient and fast, particularly for lists with a significant number of elements.”
Frequently Asked Questions (FAQs)
How can I check for duplicates in a list in Python?
You can check for duplicates in a list by converting the list to a set and comparing its length to the original list. If the lengths differ, duplicates exist. For example: `len(my_list) != len(set(my_list))`.
What is the most efficient way to find duplicates in a list?
Using a set to track seen elements is often the most efficient method. Iterate through the list, adding each element to the set. If an element is already in the set, it is a duplicate.
Can I use list comprehension to find duplicates?
Yes, you can utilize list comprehension combined with a set to identify duplicates. For example: `[item for item in my_list if my_list.count(item) > 1]` will yield a list of duplicates.
Is there a built-in Python function to check for duplicates?
Python does not have a specific built-in function for checking duplicates, but you can use the `collections.Counter` class to count occurrences of each element and identify duplicates.
How can I remove duplicates from a list in Python?
You can remove duplicates by converting the list to a set and back to a list: `my_list = list(set(my_list))`. Note that this method does not preserve the original order of elements.
What libraries can I use to handle duplicates in larger datasets?
For larger datasets, consider using libraries like `pandas`, which provides powerful data manipulation tools, including functions to identify and remove duplicates efficiently.
In Python, checking for duplicates in a list can be accomplished through various methods, each with its own advantages and use cases. One of the most straightforward approaches is to utilize a set, which inherently disallows duplicate entries. By converting the list to a set and comparing its length to the original list, one can easily determine if duplicates exist. This method is efficient and concise, making it a popular choice among developers.
Another common technique involves using a loop to iterate through the list while maintaining a separate collection to track seen items. This method allows for more control over the process and can be tailored for specific requirements, such as counting occurrences of each element. Additionally, using Python’s built-in libraries, such as the `collections.Counter`, provides a powerful way to tally elements and identify duplicates in a single line of code.
In summary, the choice of method for checking duplicates in a list depends on the specific needs of the task at hand. Whether opting for the simplicity of sets, the flexibility of loops, or the convenience of built-in libraries, Python offers versatile solutions to efficiently handle duplicate detection. Understanding these methods equips developers with the tools necessary to maintain data integrity and optimize performance in their applications.
Author Profile
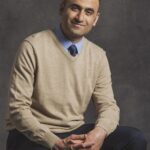
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?