How Can You Check If a Number is Even in JavaScript?
In the realm of programming, understanding how to manipulate and evaluate numbers is fundamental, especially in languages like JavaScript. One of the most basic yet essential tasks you might encounter is determining whether a number is even or odd. This seemingly simple operation can have significant implications in various applications, from controlling flow in algorithms to managing data sets in web development. Whether you’re a novice coder just starting your journey or an experienced developer brushing up on your skills, mastering this concept will enhance your ability to write efficient and effective code.
At its core, checking if a number is even involves a straightforward mathematical principle: even numbers are divisible by two without a remainder. In JavaScript, this can be accomplished using a few different methods, each with its own advantages and nuances. Understanding these techniques not only equips you with the tools to perform this check but also deepens your grasp of JavaScript’s syntax and logic.
As we delve deeper into this topic, we will explore various approaches to determine the evenness of a number in JavaScript. From utilizing the modulus operator to leveraging built-in functions, each method offers unique insights into the language’s capabilities. By the end of this article, you’ll be well-prepared to implement these techniques in your own coding projects, enhancing your programming toolkit and boosting your confidence in
Methods to Check if a Number is Even
In JavaScript, determining if a number is even can be accomplished through several methods, each with its own advantages. Here are the most common approaches:
Using the Modulus Operator
The most straightforward way to check if a number is even is by using the modulus operator (`%`). This operator returns the remainder of a division operation. For even numbers, the remainder when divided by 2 is always zero.
javascript
function isEven(num) {
return num % 2 === 0;
}
### Example
javascript
console.log(isEven(4)); // true
console.log(isEven(5)); //
Using Bitwise Operators
Another efficient method is using the bitwise AND operator (`&`). In this approach, you check if the least significant bit of the number is 0. This is a low-level operation that can be faster in performance-critical applications.
javascript
function isEven(num) {
return (num & 1) === 0;
}
### Example
javascript
console.log(isEven(10)); // true
console.log(isEven(11)); //
Checking for NaN and Infinity
When validating numbers, it is crucial to ensure the input is a valid number. JavaScript provides the `isNaN()` function and the `Number.isFinite()` method to check for non-numeric values and infinite numbers.
javascript
function isEven(num) {
if (typeof num !== ‘number’ || !Number.isFinite(num)) {
return ; // Not a valid number
}
return num % 2 === 0;
}
### Example
javascript
console.log(isEven(NaN)); //
console.log(isEven(Infinity)); //
console.log(isEven(0)); // true
Performance Comparison
While all methods are effective, performance can vary based on context. The following table summarizes the execution time and complexity of each method:
Method | Time Complexity | Space Complexity |
---|---|---|
Modulus Operator | O(1) | O(1) |
Bitwise Operator | O(1) | O(1) |
Validation with NaN & Infinity Check | O(1) | O(1) |
Each method serves its purpose and can be selected based on the specific requirements of the application.
Methods to Check if a Number is Even in JavaScript
In JavaScript, determining whether a number is even can be accomplished through various methods. The primary technique involves using the modulus operator. Below are some common approaches:
Using the Modulus Operator
The most straightforward method to check if a number is even is by using the modulus operator (`%`). This operator returns the remainder of a division operation. If a number is divisible by 2, it is even.
javascript
function isEven(number) {
return number % 2 === 0;
}
In this example:
- If `number` is even, `number % 2` will equal `0`, and the function will return `true`.
- If `number` is odd, it will return “.
Using Bitwise AND Operator
Another efficient way to check for even numbers is by using the bitwise AND operator (`&`). This method operates on the binary representation of numbers.
javascript
function isEven(number) {
return (number & 1) === 0;
}
- This approach checks the least significant bit of the number. If it is `0`, the number is even; if it is `1`, the number is odd.
Using Division
You can also check for evenness by dividing the number by `2` and checking for a remainder. However, this method is less efficient than using the modulus operator.
javascript
function isEven(number) {
return Math.floor(number / 2) * 2 === number;
}
- Here, the number is divided by `2`, floored (rounded down), and multiplied back by `2`. If the result equals the original number, it is even.
Handling Non-integer Values
When working with non-integer values, it’s essential to decide how to handle them. You may want to convert the number to an integer before checking for evenness.
javascript
function isEven(number) {
const intNumber = Math.floor(number); // Convert to integer
return intNumber % 2 === 0;
}
This ensures that any decimal values are rounded down, allowing for a proper check against evenness.
Practical Examples
Here are practical examples of using the `isEven` function:
javascript
console.log(isEven(4)); // Output: true
console.log(isEven(7)); // Output:
console.log(isEven(0)); // Output: true
console.log(isEven(-2)); // Output: true
console.log(isEven(-3)); // Output:
console.log(isEven(4.5)); // Output: true (after conversion)
Performance Considerations
- The modulus and bitwise methods are both efficient, with bitwise operations typically being faster.
- Choose the method based on code readability and maintainability, especially in collaborative environments.
- For large datasets or performance-critical applications, benchmarking different methods may be beneficial.
Each method has its own merits, and the choice may depend on the specific context or requirements of your application.
Expert Insights on Checking Even Numbers in JavaScript
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To determine if a number is even in JavaScript, one can utilize the modulus operator. By checking if `number % 2 === 0`, developers can efficiently ascertain the evenness of a number, which is a fundamental operation in programming.”
Michael Chen (JavaScript Developer Advocate, Tech Innovations). “In JavaScript, the simplicity of using the modulus operator for checking even numbers cannot be overstated. This method not only enhances code readability but also ensures optimal performance in numerical computations.”
Sarah Johnson (Lead Instructor, JavaScript Academy). “Teaching new programmers how to check if a number is even is crucial. The expression `if (num % 2 === 0)` serves as a perfect example of logical conditions in JavaScript, illustrating both syntax and the importance of understanding basic arithmetic operations.”
Frequently Asked Questions (FAQs)
How can I determine if a number is even in JavaScript?
You can determine if a number is even by using the modulus operator. If `number % 2 === 0`, the number is even.
What is the modulus operator in JavaScript?
The modulus operator (`%`) returns the remainder of a division operation. It is commonly used to check for even or odd numbers.
Can I check if a number is even using a function in JavaScript?
Yes, you can create a function that takes a number as an argument and returns `true` if it is even and “ otherwise. For example:
javascript
function isEven(num) {
return num % 2 === 0;
}
Does JavaScript treat negative numbers differently when checking for evenness?
No, JavaScript treats negative numbers the same way. A negative number is considered even if it satisfies the condition `number % 2 === 0`.
What happens if I check a non-integer number for evenness?
If you check a non-integer number, the modulus operator will still return a result. However, only whole numbers can be classified as even or odd. For example, `2.5 % 2` will yield `0.5`, indicating it is neither even nor odd.
Is there a built-in method in JavaScript to check for even numbers?
No, JavaScript does not have a built-in method specifically for checking even numbers. You must implement the check using the modulus operator or a custom function.
In JavaScript, determining whether a number is even can be efficiently accomplished using the modulus operator (%). This operator returns the remainder of a division operation. Specifically, a number is classified as even if it yields a remainder of zero when divided by two. Therefore, the expression `number % 2 === 0` serves as a straightforward method to check for evenness.
Additionally, it is important to consider the type of the variable being evaluated. JavaScript is a loosely typed language, meaning that variables can hold values of any type. To ensure accurate results, it is advisable to confirm that the variable in question is indeed a number. This can be achieved using the `typeof` operator, which can help prevent errors when non-numeric values are inadvertently checked.
In summary, checking if a number is even in JavaScript can be succinctly done with a simple conditional statement combined with the modulus operator. By adhering to best practices, such as verifying the variable type, developers can write robust and error-free code. This approach not only enhances code reliability but also improves overall performance in applications where numerical evaluations are frequent.
Author Profile
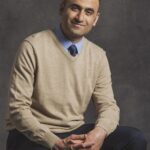
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?