How Can You Check if a Key Exists in a Python Dictionary?
In the world of Python programming, dictionaries are one of the most versatile and widely used data structures. They allow developers to store and manage data in key-value pairs, making it easy to retrieve information efficiently. However, as with any data structure, knowing how to navigate and manipulate it is crucial for effective coding. One common task that programmers frequently encounter is checking whether a specific key exists within a dictionary. This seemingly simple operation can have significant implications for data handling and control flow in your applications.
Understanding how to check for a key in a dictionary is essential for ensuring that your code runs smoothly and avoids potential errors. Whether you’re working with large datasets or just need to validate user input, knowing the right methods to employ can save you time and frustration. Python offers several straightforward techniques to check for key existence, each with its own advantages depending on the context in which you’re working.
As you delve deeper into the intricacies of Python dictionaries, you’ll discover not only the syntax involved in these checks but also best practices that can enhance your coding efficiency. By mastering this fundamental aspect of Python, you’ll be well-equipped to handle various programming challenges and harness the full power of dictionaries in your projects. Get ready to unlock the secrets of key checking in Python and elevate your coding skills to new heights
Checking for Keys in a Python Dictionary
To determine whether a specific key exists within a Python dictionary, you can utilize several approaches. Each method offers different advantages based on the context in which you are working.
Using the `in` Keyword
The most straightforward way to check for a key in a dictionary is by using the `in` keyword. This method is not only concise but also highly readable, making it a preferred choice among Python developers.
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
key_to_check = ‘age’
if key_to_check in my_dict:
print(f”{key_to_check} exists in the dictionary.”)
else:
print(f”{key_to_check} does not exist in the dictionary.”)
“`
This code will output:
“`
age exists in the dictionary.
“`
Using the `get()` Method
Another method to check for a key is by utilizing the dictionary’s `get()` method. This method retrieves the value associated with the specified key if it exists; otherwise, it returns `None` or a default value you specify.
“`python
value = my_dict.get(‘city’)
if value is not None:
print(“Key exists in the dictionary.”)
else:
print(“Key does not exist in the dictionary.”)
“`
You can also specify a default return value:
“`python
value = my_dict.get(‘country’, ‘Key not found’)
print(value) Output: Key not found
“`
Using the `keys()` Method
You can also check for the presence of a key by using the `keys()` method, which returns a view of the dictionary’s keys. While this method works, it is less efficient than the `in` keyword, as it generates a list of all keys.
“`python
if ‘name’ in my_dict.keys():
print(“Key exists.”)
else:
print(“Key does not exist.”)
“`
Comparative Summary of Methods
The following table summarizes the various methods for checking if a key exists in a dictionary:
Method | Syntax | Returns | Efficiency |
---|---|---|---|
in Keyword | key in dict | True/ | High |
get() Method | dict.get(key) | Value or None | Medium |
keys() Method | key in dict.keys() | True/ | Low |
Conclusion on Key Checking Methods
Understanding the various methods for checking the existence of keys in a Python dictionary allows developers to choose the most appropriate one for their specific use case. Each method has its strengths and weaknesses, but the `in` keyword remains the most efficient and readable option.
Checking for Key Existence in a Dictionary
In Python, dictionaries are a collection of key-value pairs that allow for efficient data retrieval. To determine if a specific key exists within a dictionary, there are several methods available, each with its own advantages.
Using the `in` Operator
The most straightforward way to check if a key exists is by using the `in` operator. This method is both intuitive and efficient.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
key_to_check = ‘b’
if key_to_check in my_dict:
print(f”{key_to_check} exists in the dictionary.”)
else:
print(f”{key_to_check} does not exist in the dictionary.”)
“`
Using the `get()` Method
The `get()` method provides another way to check for key existence while also allowing you to specify a default return value if the key is not found. This approach can help avoid KeyErrors.
“`python
value = my_dict.get(key_to_check, ‘Key not found’)
print(value) Returns the value or ‘Key not found’ if the key doesn’t exist.
“`
Using `keys()` Method
You can also use the `keys()` method to retrieve a view object of the dictionary’s keys and check for membership. This method is less efficient compared to the `in` operator, as it creates a view object.
“`python
if key_to_check in my_dict.keys():
print(f”{key_to_check} exists in the dictionary.”)
“`
Using `setdefault()` Method
The `setdefault()` method checks for a key and returns its value if present. If the key is not found, it inserts the key with a specified default value. This method can be useful when you need to check and modify the dictionary simultaneously.
“`python
value = my_dict.setdefault(key_to_check, ‘Default value’)
print(value) Prints the value associated with the key or ‘Default value’ if the key didn’t exist.
“`
Performance Considerations
While all methods provide a way to check for key existence, performance can vary:
Method | Time Complexity | Notes |
---|---|---|
`in` operator | O(1) | Fastest and most efficient method. |
`get()` | O(1) | Useful for returning a default value. |
`keys()` | O(n) | Slower as it creates a keys view. |
`setdefault()` | O(1) | Useful for checking and setting default. |
Choosing the right method depends on the specific requirements of your application, such as whether you need to handle default values or modify the dictionary simultaneously.
Expert Insights on Checking Keys in Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To efficiently check if a key exists in a Python dictionary, the most straightforward method is using the ‘in’ keyword. This approach is not only readable but also optimized for performance, making it a preferred choice among seasoned developers.”
Michael Chen (Data Scientist, Analytics Hub). “Using the ‘get()’ method is another effective way to check for a key in a dictionary. This method allows you to specify a default return value if the key is not found, thus providing flexibility in handling missing keys without raising an error.”
Lisa Tran (Python Educator, Code Academy). “For beginners, I recommend starting with the ‘in’ operator for key checks. It is intuitive and aligns well with Python’s philosophy of simplicity and readability, which is crucial for those new to programming.”
Frequently Asked Questions (FAQs)
How can I check if a key exists in a Python dictionary?
You can check if a key exists in a dictionary using the `in` keyword. For example, `if key in my_dict:` returns `True` if the key is present.
What is the difference between using `in` and the `get()` method?
Using `in` checks for the existence of a key, while the `get()` method retrieves the value associated with the key. If the key does not exist, `get()` returns `None` or a specified default value, whereas `in` returns a boolean.
Can I check for multiple keys in a dictionary at once?
You can check for multiple keys using a loop or a list comprehension. For example, `[key in my_dict for key in keys]` creates a list of boolean values indicating the presence of each key.
Is there a performance difference between using `in` and `get()`?
Using `in` is generally faster for checking key existence, as it directly checks the dictionary’s hash table. The `get()` method involves a lookup and returns the value, which may be slightly slower.
What happens if I check for a key that is not in the dictionary?
If you check for a key that is not present using `in`, it will return “. If you use `get()`, it will return `None` or the specified default value if provided.
Are there any alternative methods to check for keys in a dictionary?
Yes, you can also use the `keys()` method, like `if key in my_dict.keys():`, but this is less efficient than simply using `in`, as it creates a list of keys.
In Python, checking if a key exists in a dictionary can be accomplished through several straightforward methods. The most common approach is to use the `in` keyword, which allows for a clean and efficient way to determine if a specified key is present. For example, the expression `key in my_dict` returns `True` if the key exists and “ otherwise. This method is both readable and efficient, making it the preferred choice for many developers.
Another method involves using the `get()` function, which retrieves the value associated with a key if it exists, or returns a default value if it does not. While this method can also indicate the presence of a key, it is generally used when the value of the key is needed, rather than solely for existence checking. Additionally, the `keys()` method can be employed, but it is less efficient than using the `in` keyword, as it creates a view of all keys in the dictionary.
In summary, the most effective and Pythonic way to check for the presence of a key in a dictionary is by utilizing the `in` keyword. This method is not only concise but also enhances code readability. Understanding these methods is crucial for efficient dictionary manipulation and can significantly improve
Author Profile
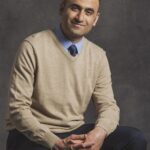
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?