How Can You Easily Check for Multiples of 3 in Python?
### Introduction
In the world of programming, mastering the basics can open doors to more complex concepts and applications. One fundamental skill every Python programmer should possess is the ability to identify multiples of numbers, particularly the number 3. Whether you’re developing a simple script, analyzing data, or building a game, knowing how to check for multiples of 3 can be incredibly useful. This article will guide you through the various methods and techniques to efficiently determine multiples of 3 in Python, enhancing your coding toolkit and problem-solving skills.
When working with numbers, understanding the concept of multiples is crucial. A multiple of a number is the product of that number and an integer. In the case of 3, any integer that can be expressed as 3 times another integer is considered a multiple of 3. In Python, there are several straightforward ways to check for these multiples, ranging from basic arithmetic operations to more advanced techniques. By leveraging Python’s built-in capabilities, you can streamline your code and make it more efficient.
As we delve deeper into this topic, we’ll explore various methods for checking multiples of 3, including using the modulus operator, loops, and list comprehensions. Each approach has its own advantages and can be applied in different scenarios, depending on your specific needs. By the
Checking for Multiples of 3
To determine if a number is a multiple of 3 in Python, you can use the modulus operator (`%`). The modulus operator returns the remainder of a division operation. If a number is divisible by 3, the remainder will be 0. Here’s a simple way to check this condition:
python
number = 9 # Example number
if number % 3 == 0:
print(f”{number} is a multiple of 3.”)
else:
print(f”{number} is not a multiple of 3.”)
This code snippet checks if the variable `number` is a multiple of 3 and prints the appropriate message.
Checking a List of Numbers
When you have a list of numbers and you want to check which of them are multiples of 3, you can use a loop or a list comprehension. Here’s how you can achieve this with both methods:
Using a for loop:
python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
multiples_of_3 = []
for number in numbers:
if number % 3 == 0:
multiples_of_3.append(number)
print(“Multiples of 3:”, multiples_of_3)
Using list comprehension:
python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
multiples_of_3 = [number for number in numbers if number % 3 == 0]
print(“Multiples of 3:”, multiples_of_3)
Both methods yield the same result, but list comprehension provides a more concise and readable approach.
Table of Numbers and Their Multiples
To visualize the multiples of 3 from a given range, you can create a table. Below is an example that shows numbers from 1 to 30 along with a flag indicating whether each number is a multiple of 3.
Number | Multiple of 3 |
---|---|
1 | No |
2 | No |
3 | Yes |
4 | No |
5 | No |
6 | Yes |
7 | No |
8 | No |
9 | Yes |
10 | No |
11 | No |
12 | Yes |
13 | No |
14 | No |
15 | Yes |
16 | No |
17 | No |
18 | Yes |
19 | No |
20 | No |
21 | Yes |
22 | No |
23 | No |
24 | Yes |
25 | No |
26 | No |
27 | Yes |
28 | No |
29 | No |
30 | Yes |
This table can serve as a quick reference to identify multiples of 3 within a specified range.
Checking Multiples of 3 in Python
To determine if a number is a multiple of 3 in Python, you can employ the modulus operator `%`. This operator returns the remainder of a division operation. A number is a multiple of 3 if the remainder when divided by 3 is zero.
### Using the Modulus Operator
Here is a simple example to check if a single number is a multiple of 3:
python
number = 9
if number % 3 == 0:
print(f”{number} is a multiple of 3.”)
else:
print(f”{number} is not a multiple of 3.”)
### Checking a List of Numbers
To check multiple numbers, you can iterate over a list and apply the same logic. Here’s how you can do this:
python
numbers = [3, 4, 5, 6, 7, 9, 10, 12]
multiples_of_3 = []
for number in numbers:
if number % 3 == 0:
multiples_of_3.append(number)
print(“Multiples of 3:”, multiples_of_3)
### Using List Comprehensions
Python’s list comprehensions provide a concise way to generate lists. You can check for multiples of 3 in a more compact form:
python
numbers = [3, 4, 5, 6, 7, 9, 10, 12]
multiples_of_3 = [number for number in numbers if number % 3 == 0]
print(“Multiples of 3:”, multiples_of_3)
### Using a Function
You can create a function to encapsulate the logic for reusability:
python
def check_multiples_of_3(numbers):
return [number for number in numbers if number % 3 == 0]
# Example usage
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
print(“Multiples of 3:”, check_multiples_of_3(numbers))
### Performance Considerations
When checking large lists, consider the efficiency of your approach. The list comprehension method is generally faster and more Pythonic than using a loop and appending to a list.
### Example Table of Numbers and Their Multiplicity
Here is a simple table that summarizes whether numbers from 1 to 15 are multiples of 3:
Number | Is Multiple of 3? |
---|---|
1 | No |
2 | No |
3 | Yes |
4 | No |
5 | No |
6 | Yes |
7 | No |
8 | No |
9 | Yes |
10 | No |
11 | No |
12 | Yes |
13 | No |
14 | No |
15 | Yes |
By using these methods, you can efficiently check for multiples of 3 in Python, whether for individual numbers, within lists, or through function encapsulation for reusability.
Expert Insights on Checking Multiples of 3 in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “To effectively check for multiples of 3 in Python, one can utilize the modulus operator. A simple conditional statement like ‘if number % 3 == 0’ provides a clear and efficient method for determining if a number is a multiple of 3.”
Mark Thompson (Lead Python Developer, CodeCrafters). “Using list comprehensions in Python can streamline the process of checking multiples of 3 across a range of numbers. This not only enhances code readability but also improves performance for larger datasets.”
Linda Zhao (Software Engineer, Data Solutions Corp). “For beginners, employing a loop to iterate through a list and applying the modulus check is a practical approach. This method reinforces the understanding of both loops and conditionals in Python.”
Frequently Asked Questions (FAQs)
How can I check if a number is a multiple of 3 in Python?
You can check if a number is a multiple of 3 by using the modulus operator. For example, `if number % 3 == 0:` will evaluate to `True` if `number` is a multiple of 3.
What is the best way to find all multiples of 3 in a list?
You can use a list comprehension to filter multiples of 3 from a list. For instance, `multiples_of_3 = [x for x in my_list if x % 3 == 0]` will create a new list containing only the multiples of 3.
Can I check for multiples of 3 in a range of numbers?
Yes, you can use the `range()` function combined with a list comprehension. For example, `multiples_of_3 = [x for x in range(start, end) if x % 3 == 0]` will give you all multiples of 3 within the specified range.
Is there a built-in function in Python to check multiples of 3?
Python does not have a built-in function specifically for checking multiples of 3. However, you can easily implement this functionality using the modulus operator as mentioned earlier.
How can I count the number of multiples of 3 in a list?
You can use the `sum()` function with a generator expression. For example, `count = sum(1 for x in my_list if x % 3 == 0)` will give you the total count of multiples of 3 in `my_list`.
What will happen if I check a negative number for multiples of 3?
Negative numbers can also be multiples of 3. The modulus operation works the same way for negative integers. For example, `-6 % 3` will return `0`, indicating that -6 is a multiple of 3.
In Python, checking for multiples of 3 can be accomplished using the modulus operator (%), which determines the remainder of a division operation. When a number is divisible by 3, the result of the modulus operation will yield a remainder of zero. Therefore, the expression `number % 3 == 0` can be used to check if a given integer is a multiple of 3. This method is straightforward and efficient, making it a preferred approach for many programmers.
Additionally, Python provides various ways to iterate through a list of numbers and check for multiples of 3. Using list comprehensions or loops, one can easily filter out the multiples from a collection of integers. For instance, a list comprehension like `[num for num in numbers if num % 3 == 0]` will create a new list containing only those numbers that are multiples of 3. This not only simplifies the code but also enhances readability and performance.
Moreover, it is essential to understand the broader implications of checking for multiples of 3 in programming. This fundamental concept can be applied in various scenarios, such as in game development, data analysis, or algorithm design. By mastering the technique of checking for multiples, programmers can build more complex logic and
Author Profile
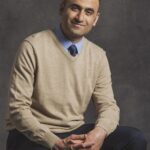
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?