How Can You Easily Check Your Python Interpreter Version?
In the ever-evolving world of programming, Python stands out as a versatile and powerful language embraced by developers across various fields. Whether you’re a seasoned programmer or just starting your coding journey, knowing the version of your Python interpreter is crucial. Different versions of Python can introduce new features, deprecate old ones, and sometimes even alter the behavior of existing code. Thus, understanding how to check your Python interpreter version is not just a matter of curiosity; it’s an essential skill that can significantly impact your development process.
When working with Python, it’s important to recognize that the language has undergone significant changes over the years. From Python 2 to Python 3, the transition marked a pivotal moment that introduced numerous enhancements and optimizations. Each version of Python comes with its own set of libraries, syntax, and functionalities, making it vital for developers to ensure compatibility with their projects. By knowing how to check your Python interpreter version, you can avoid potential pitfalls and ensure that your code runs smoothly in the intended environment.
In this article, we will explore various methods to determine the version of your Python interpreter, whether you’re using a command-line interface, an integrated development environment (IDE), or even a Jupyter notebook. Understanding these techniques will empower you to manage your Python installations effectively
Checking Python Interpreter Version in the Command Line
To determine the version of the Python interpreter you are using, the command line is one of the most straightforward methods. This can be done by executing specific commands in your terminal or command prompt.
- For Windows:
- Open the Command Prompt (cmd).
- Type the following command and press Enter:
“`
python –version
“`
or
“`
python -V
“`
- For macOS/Linux:
- Open the Terminal.
- Type the following command and press Enter:
“`
python3 –version
“`
or
“`
python3 -V
“`
This will display the version of Python that is currently set as the default interpreter in your environment.
Checking Python Version Within a Script
If you need to check the Python version programmatically within a script, you can utilize the built-in `sys` module. This is useful for ensuring compatibility in your code or logging the version for debugging purposes.
Here’s how you can do it:
“`python
import sys
print(“Python version”)
print(sys.version)
print(“Version info.”)
print(sys.version_info)
“`
This script will output the current Python version along with detailed version information, including major, minor, micro, release level, and serial number.
Using the Python REPL
Another method to check the Python version is by using the Python interactive shell, also known as the REPL (Read-Eval-Print Loop).
- To access the Python REPL:
- Open your terminal or command prompt.
- Type `python` or `python3` and press Enter.
Once in the REPL, you can check the version with the following commands:
“`python
import platform
print(platform.python_version())
“`
This will print the Python version in a user-friendly format.
Version Comparison Table
When working with Python, it’s essential to be aware of the differences between the major versions, especially Python 2 and Python 3. Below is a comparison table highlighting some key differences:
Feature | Python 2 | Python 3 |
---|---|---|
Print Statement | print “Hello” | print(“Hello”) |
Integer Division | 5 / 2 = 2 | 5 / 2 = 2.5 |
Unicode Support | ASCII by default | UTF-8 by default |
Libraries | Some libraries are deprecated | Active development and support |
Understanding these differences can significantly impact the development and execution of Python programs, especially when transitioning from Python 2 to Python 3.
Checking Python Interpreter Version in the Command Line
To determine the version of the Python interpreter you are using, the most straightforward method is through the command line interface. This can be accomplished by executing a simple command.
- Windows:
- Open Command Prompt.
- Type the following command and press Enter:
“`
python –version
“`
or
“`
python -V
“`
- macOS/Linux:
- Open the Terminal.
- Enter the following command:
“`
python –version
“`
or
“`
python -V
“`
For systems with multiple Python versions installed, you may need to specify `python3` instead of `python`.
- Example:
“`
python3 –version
“`
Checking Python Version from Within a Python Script
You can also check the Python version programmatically by using the `sys` module. This method is useful for scripts that may need to adapt behavior based on the Python version.
“`python
import sys
print(“Python version”)
print(sys.version)
print(“Version info.”)
print(sys.version_info)
“`
This script provides detailed information, including major, minor, and micro version numbers.
Using Python Shell to Check Version
If you are already in the Python interactive shell, you can check the version directly by executing the following commands:
- Start the Python shell by typing `python` or `python3` in your terminal.
- Once in the shell, type:
“`python
import platform
print(platform.python_version())
“`
This will output the version of the Python interpreter currently in use.
Checking Version in Jupyter Notebooks
If you are utilizing a Jupyter Notebook, you can check the Python version by executing the following code in a cell:
“`python
import sys
sys.version
“`
Alternatively, you can use the `!` operator to run shell commands directly:
“`python
!python –version
“`
Table of Common Commands to Check Python Version
Operating System | Command | Description |
---|---|---|
Windows | `python –version` | Displays the Python version. |
Windows | `python -V` | Alternative command to check version. |
macOS/Linux | `python –version` | Displays the Python version. |
macOS/Linux | `python3 –version` | Checks version for Python 3 specifically. |
Python Shell | `import sys; print(sys.version)` | Displays detailed version info. |
Jupyter Notebook | `import sys; sys.version` | Provides the Python version in Jupyter. |
Jupyter Notebook | `!python –version` | Executes shell command for version check. |
Each of these methods provides a reliable way to ascertain the version of the Python interpreter, ensuring that your development environment is set up correctly for your projects.
Expert Insights on Checking Python Interpreter Version
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To check the Python interpreter version, one can simply execute the command ‘python –version’ or ‘python3 –version’ in the terminal. This method is straightforward and provides immediate clarity on the version being used, which is crucial for compatibility in development projects.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Utilizing the built-in ‘sys’ module is another effective way to determine the Python version. By running ‘import sys’ followed by ‘print(sys.version)’, developers can access detailed version information directly from their scripts, which is particularly useful in environments where command line access is limited.”
Sarah Patel (Python Educator, LearnPythonNow). “For beginners, I recommend checking the Python version using the command line, as it is the most accessible method. However, understanding how to retrieve version information programmatically using ‘platform.python_version()’ can empower learners to write more versatile and adaptable code.”
Frequently Asked Questions (FAQs)
How can I check the Python interpreter version from the command line?
You can check the Python interpreter version by opening your command line interface and typing `python –version` or `python3 –version`, depending on your installation. This will display the version number of the Python interpreter currently in use.
Is there a way to check the Python version within a script?
Yes, you can check the Python version within a script by using the `sys` module. Include the following code:
“`python
import sys
print(sys.version)
“`
This will print the version of Python that is executing the script.
What command should I use in a Jupyter notebook to check the Python version?
In a Jupyter notebook, you can check the Python version by executing the following command in a cell:
“`python
!python –version
“`
Alternatively, you can use:
“`python
import sys
sys.version
“`
Can I check the Python version in an interactive shell?
Yes, you can check the Python version in an interactive shell by simply typing `python` or `python3` to start the interpreter, and then using the command `import sys` followed by `print(sys.version)`.
What is the difference between `python` and `python3` commands?
The `python` command typically refers to Python 2.x versions, while `python3` explicitly refers to Python 3.x versions. The specific behavior may depend on your system configuration and how Python is installed.
How can I check the version of Python packages I have installed?
You can check the version of installed Python packages by using the command `pip list` in your command line. This will display a list of all installed packages along with their respective versions.
In summary, checking the version of the Python interpreter is an essential task for developers and users alike, as it ensures compatibility with libraries and frameworks. There are several straightforward methods to determine the version of Python installed on a system. The most common approach is to use the command line or terminal, where users can execute the command `python –version` or `python3 –version`. This will display the version number directly in the console.
Another method involves using Python’s interactive shell. By launching the interpreter and executing the command `import sys` followed by `print(sys.version)`, users can retrieve detailed information about the Python version, including additional build information. This method is particularly useful for those who prefer working within the Python environment itself.
Additionally, integrated development environments (IDEs) and code editors often provide built-in features to check the Python version being used. Users can typically find this information in the settings or about section of the IDE. Understanding which version of Python is in use is crucial for effective development and troubleshooting.
knowing how to check the Python interpreter version is a fundamental skill for anyone working with Python. Whether through command line commands, the interactive shell, or IDE features, users have multiple
Author Profile
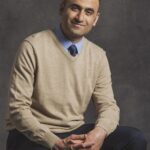
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?