How Can You Easily Check the Version of a Python Library?
When working with Python, one of the most crucial aspects of maintaining your projects is ensuring that you are using the correct versions of libraries. Whether you’re developing a new application or troubleshooting an existing one, knowing the version of the libraries you’re working with can save you time and prevent compatibility issues. In a world where software is constantly evolving, understanding how to check the version of your Python libraries is an essential skill for any developer.
In this article, we will explore the various methods available for checking the version of Python libraries, ranging from simple command-line instructions to utilizing built-in functions within your code. We’ll discuss the importance of version control in your projects, especially when collaborating with others or deploying applications in different environments. By the end of this guide, you will have a clear understanding of how to efficiently verify library versions, ensuring that your Python projects run smoothly and as intended.
Whether you are a beginner just starting your coding journey or an experienced developer looking to brush up on best practices, this article will provide you with the tools and knowledge needed to manage your Python library versions effectively. Get ready to dive into the world of version management and enhance your programming prowess!
Checking the Version of a Python Library
To determine the version of a Python library that is currently installed in your environment, there are several methods available. Each approach serves different use cases, whether you are working in a script, an interactive shell, or a package management tool.
Using the Command Line
One of the most straightforward methods to check the version of an installed Python library is through the command line interface. The following commands can be utilized:
- Using pip: This is the package manager for Python. You can check the version of a specific library by using the following command:
“`bash
pip show
For example, to check the version of NumPy, you would run:
“`bash
pip show numpy
“`
- Listing installed packages: If you want to see all installed packages along with their versions, you can use:
“`bash
pip list
“`
This will display a table of all installed libraries and their respective versions.
Using Python Code
If you prefer to check the version programmatically within a Python script or interactive shell, you can use the following methods:
- Using `__version__` attribute: Many libraries have a `__version__` attribute that you can access directly. For example:
“`python
import numpy
print(numpy.__version__)
“`
- Using the `pkg_resources` module: This module from the `setuptools` package allows you to access package metadata, including version information:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“numpy”).version
print(version)
“`
Example Table of Library Versions
The following table illustrates how different libraries expose their versioning information:
Library | Method to Check Version | Example Output |
---|---|---|
NumPy | numpy.__version__ | 1.21.0 |
Pandas | pandas.__version__ | 1.3.1 |
Requests | requests.__version__ | 2.26.0 |
Matplotlib | matplotlib.__version__ | 3.4.3 |
Using Environment Management Tools
For users employing virtual environments or environment management tools like Anaconda, checking library versions can be done through specific commands:
- Anaconda Navigator: Open the Anaconda Navigator and navigate to the “Environments” tab. You can view all installed packages and their versions in the selected environment.
- Conda command line: You can also use the command line to check installed packages with:
“`bash
conda list
“`
This command provides a comprehensive list of packages, similar to `pip list`.
By utilizing these methods, you can efficiently verify the version of any Python library, ensuring compatibility and aiding in debugging processes.
Checking Python Library Version via Command Line
One of the most straightforward methods to check the version of a Python library is by using the command line interface. This can be done using either `pip` or `python` commands.
Using pip:
- Open your command prompt or terminal.
- Enter the following command:
“`
pip show
Replace `
Example:
“`
pip show numpy
“`
Output:
“`
Name: numpy
Version: 1.21.2
Summary: NumPy is the fundamental package for array computing with Python.
“`
Using pip list:
- To see a list of all installed libraries along with their versions, you can run:
“`
pip list
“`
This will display all installed packages in a tabulated format.
Checking Python Library Version within a Python Script
You can also determine the version of a library programmatically by importing the library in a Python script. Most libraries have a `__version__` attribute that stores their version number.
Example:
“`python
import numpy
print(numpy.__version__)
“`
If the library does not have a `__version__` attribute, you can often find the version using the following method:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“library_name”).version
print(version)
“`
Replace `”library_name”` with the name of the library.
Checking Library Version in Jupyter Notebooks
In a Jupyter Notebook, you can use similar techniques to check library versions. You can run the following commands in a cell:
Using pip:
“`python
!pip show numpy
“`
Using the library’s `__version__`:
“`python
import pandas as pd
pd.__version__
“`
This will display the version of the Pandas library directly in the output cell.
Using Anaconda Navigator
If you are using Anaconda, you can check the library versions through the Anaconda Navigator interface:
- Open Anaconda Navigator.
- Go to the “Environments” tab.
- Select your environment.
- You will see a list of installed packages along with their versions.
This is particularly useful for managing libraries across multiple environments.
Verifying Version Compatibility
When checking library versions, it is crucial to ensure compatibility with other libraries or your project. You can refer to the documentation of the specific library for compatibility information, typically found in the installation or requirements sections. Additionally, tools like `pipdeptree` can help visualize dependencies and their versions:
Installation:
“`
pip install pipdeptree
“`
Usage:
“`
pipdeptree
“`
This will create a tree structure showing all installed packages and their dependencies, allowing you to easily assess version compatibility.
Expert Insights on Checking Python Library Versions
Dr. Emily Carter (Senior Software Engineer, Python Software Foundation). “To check the version of a Python library, one can utilize the command line interface by executing ‘pip show
‘. This command provides detailed information about the library, including its version, which is essential for ensuring compatibility with your project.”
Michael Chen (Data Scientist, Tech Innovations Inc.). “Another effective method to check the version of a Python library is by importing the library in a Python script and printing its version attribute. For example, using ‘import
‘ followed by ‘print( .__version__)’ gives you direct access to the version information.”
Laura Smith (Python Developer Advocate, CodeCraft). “For those using Jupyter notebooks, the command ‘!pip show
‘ can be executed directly in a cell. This approach is particularly convenient for data science workflows, allowing for quick checks without leaving the notebook environment.”
Frequently Asked Questions (FAQs)
How can I check the version of a specific Python library?
You can check the version of a specific Python library by using the command `pip show
Is there a way to check the version of all installed Python libraries?
Yes, you can check the version of all installed Python libraries by running the command `pip list`. This will provide a list of all installed packages along with their respective versions.
Can I check the version of a library from within a Python script?
Yes, you can check the version of a library from within a Python script by importing the library and accessing its `__version__` attribute, if available. For example:
“`python
import library_name
print(library_name.__version__)
“`
What command do I use to check the version of pip itself?
To check the version of pip, use the command `pip –version` or `pip -V` in your terminal or command prompt. This will display the pip version along with the Python version it is associated with.
Are there any differences in checking versions for libraries installed in a virtual environment?
No, the method to check library versions remains the same in a virtual environment. Ensure you activate the virtual environment first, then use `pip show
What should I do if a library does not have a version attribute?
If a library does not have a `__version__` attribute, consult the library’s documentation or its setup.py file for version information. Alternatively, you can use `pip show
Checking the version of a Python library is an essential task for developers and data scientists alike. It ensures compatibility with other packages and helps in troubleshooting issues that may arise due to version discrepancies. There are several methods to determine the version of a library installed in your Python environment, including using the command line, the Python interpreter, and package management tools such as pip.
One of the most straightforward methods is to use the command line with pip. By executing the command `pip show
In summary, knowing how to check the version of a Python library is crucial for maintaining a stable and functional development environment. Whether through command line tools or Python code, these methods provide clarity and ensure that developers can manage dependencies effectively. Staying informed about library versions not only aids in debugging but also enhances collaboration within teams by ensuring that everyone is working with compatible software versions.
Author Profile
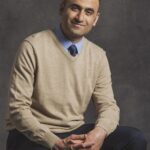
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?