How Can You Check the Version of a Python Package?
In the ever-evolving world of programming, keeping your tools and libraries up to date is crucial for maintaining the functionality and security of your projects. Python, with its rich ecosystem of packages, offers developers a plethora of options to enhance their coding experience. However, as you dive deeper into your projects, you may find yourself asking: “How do I check the version of a Python package?” This seemingly simple question holds the key to ensuring compatibility, leveraging new features, and troubleshooting issues that may arise from outdated libraries.
Understanding how to check the version of a Python package is an essential skill for both novice and experienced developers alike. Whether you’re working on a personal project or collaborating within a team, knowing the specific version of a package can help you avoid conflicts and ensure that your code runs smoothly. Python provides several methods to retrieve this information, each suited to different scenarios and preferences. From command-line tools to programmatic approaches, there are various ways to stay informed about the packages you rely on.
As we explore the different techniques for checking package versions, you’ll discover the importance of version management in your development workflow. Armed with this knowledge, you’ll be better equipped to make informed decisions about updates, compatibility, and the overall health of your Python environment. Join us as we delve into the practical
Using the Command Line
One of the most straightforward methods to check the version of a Python package is through the command line interface. This can be done using the `pip` tool, which is the package installer for Python. To check the version of an installed package, you can use the following command:
“`bash
pip show package_name
“`
Replace `package_name` with the actual name of the package you are interested in. This command will return several details about the package, including its version number.
Alternatively, you can list all installed packages along with their versions by using:
“`bash
pip list
“`
This command provides a comprehensive overview of all packages installed in your Python environment.
Using Python Code
Another method to check the version of a package is to use Python code directly. This can be particularly useful in scripts or when working within an interactive Python shell. You can access the version of an installed package by importing it and checking its `__version__` attribute. For example:
“`python
import package_name
print(package_name.__version__)
“`
If the package does not define a `__version__` attribute, you might need to consult the documentation or use alternative methods to determine the version.
Checking Version with `pkg_resources`
Python’s `pkg_resources` module, part of the `setuptools` package, allows for more advanced version checks. Here’s how to use it:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“package_name”).version
print(version)
“`
This method is beneficial for checking the version of a package programmatically, especially when dealing with multiple dependencies in a project.
Table of Version Checking Methods
Method | Command/Code | Output |
---|---|---|
Command Line (pip show) | pip show package_name |
Displays package details including version |
Command Line (pip list) | pip list |
Lists all installed packages with their versions |
Python Code (import) | import package_name |
Displays the version if defined |
Using pkg_resources | import pkg_resources |
Returns the version of the specified package |
Using Anaconda Navigator
For users working within the Anaconda environment, Anaconda Navigator provides a graphical interface to check package versions. To find the version of a package:
- Open Anaconda Navigator.
- Navigate to the “Environments” tab.
- Select the environment where the package is installed.
- In the list of installed packages, locate your package and view its version number displayed alongside it.
This method is particularly user-friendly for those who prefer not to use the command line.
Checking the Version of a Python Package
To determine the version of a Python package, there are several methods available. Below are the most common techniques used in various environments.
Using pip
The `pip` command is widely used for package management in Python. You can check the version of an installed package by executing the following command in your terminal or command prompt:
“`bash
pip show package_name
“`
Replace `package_name` with the name of the package you wish to check. The output will include details about the package, including its version. For example:
“`bash
pip show requests
“`
This will yield output similar to:
“`
Name: requests
Version: 2.25.1
Summary: Python HTTP for Humans.
…
“`
Using Python Code
You can also check the version of a package programmatically within a Python script or interpreter. Here’s how to do it:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“package_name”).version
print(version)
“`
Replace `package_name` with the desired package name. For instance, to check the version of the `numpy` package:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“numpy”).version
print(version)
“`
Using the __version__ Attribute
Many Python packages include a `__version__` attribute that can be accessed directly. This method is straightforward but may not be available for every package. Here’s an example:
“`python
import numpy
print(numpy.__version__)
“`
This will directly output the version number of `numpy`.
Using conda
If you are using Anaconda to manage your packages, you can check the version of a package using the following command:
“`bash
conda list package_name
“`
For example:
“`bash
conda list pandas
“`
This will display a list of installed packages, including the version of `pandas`.
Using a Requirements File
If you have a `requirements.txt` file, you can also check the versions of all listed packages by running:
“`bash
pip freeze
“`
This command will output a list of all installed packages along with their versions in the format:
“`
package_name==version
“`
For example:
“`
requests==2.25.1
numpy==1.19.5
“`
Version Comparison with pip
You can compare package versions directly using pip. To check if a package is up to date or if a specific version is installed, you can use:
“`bash
pip list –outdated
“`
This will list all packages that have updates available, along with the current and latest versions.
By employing these methods, you can efficiently check the version of Python packages in your environment and ensure your projects are using the correct dependencies.
Expert Insights on Checking Python Package Versions
Dr. Emily Carter (Senior Software Engineer, Python Software Foundation). “To check the version of a Python package, the most reliable method is to use the command `pip show package_name`. This command provides detailed information about the package, including its version, which is essential for ensuring compatibility in your projects.”
Mark Thompson (Lead Developer, Open Source Initiative). “Another effective way to check a package version is by using the Python interpreter itself. You can run `import package_name; print(package_name.__version__)`. This method is particularly useful when you want to verify the version programmatically within your code.”
Lisa Chen (Data Scientist, AI Innovations). “For those who prefer a graphical interface, tools like Anaconda Navigator allow users to view installed packages and their versions easily. This can be particularly helpful for data science projects where managing dependencies is crucial.”
Frequently Asked Questions (FAQs)
How can I check the version of a specific Python package?
You can check the version of a specific Python package using the command `pip show package_name`, replacing `package_name` with the name of the package. This command will display detailed information, including the version.
Is there a way to check the version of all installed Python packages?
Yes, you can check the version of all installed Python packages by running the command `pip list`. This will provide a list of all packages along with their respective versions.
Can I check the version of a Python package programmatically?
Yes, you can check the version of a Python package programmatically by importing the package and accessing its `__version__` attribute, if available. For example, `import package_name; print(package_name.__version__)`.
What command should I use to check the version of a package in a virtual environment?
To check the version of a package in a virtual environment, first activate the virtual environment, then use the command `pip show package_name` or `pip list` to see the installed packages and their versions.
Are there any differences in checking package versions between pip and conda?
Yes, while `pip` uses commands like `pip show` and `pip list`, `conda` uses commands such as `conda list` to check package versions. Each package manager has its own syntax and methods for version checking.
What should I do if the version of a package is not displayed?
If the version of a package is not displayed, ensure that the package is installed correctly. You can reinstall the package using `pip install package_name –upgrade` to ensure you have the latest version.
Checking the version of a Python package is an essential task for developers and data scientists, as it ensures compatibility and helps manage dependencies effectively. There are several methods to achieve this, including using the command line interface, Python’s built-in functions, or package management tools like pip and conda. Each of these methods provides a straightforward way to retrieve version information, catering to different user preferences and environments.
One of the most common approaches is to use the pip command in the terminal. By executing `pip show
In summary, understanding how to check the version of a Python package is crucial for maintaining a stable development environment. Whether through command line tools or within Python scripts, these methods empower users to manage their packages effectively. This knowledge not only aids in troubleshooting but also enhances the overall efficiency of software development practices.
Author Profile
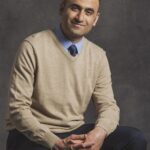
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?