How Can You Effectively Clean Data in Python?
In the age of big data, the ability to glean insights from raw information has never been more critical. However, before any analysis can take place, one crucial step stands between you and meaningful results: data cleaning. Often regarded as the unsung hero of data science, cleaning data in Python is not just about removing errors; it’s about transforming chaotic datasets into well-organized, reliable sources of truth. Whether you’re a budding analyst or an experienced data scientist, mastering the art of data cleaning can significantly enhance the quality of your work and the accuracy of your conclusions.
Data cleaning involves a series of processes aimed at identifying and correcting inaccuracies, inconsistencies, and missing values within your datasets. In Python, a plethora of libraries such as Pandas and NumPy provide powerful tools to streamline this process, allowing you to manipulate and prepare your data efficiently. From handling duplicates and outliers to standardizing formats and filling in gaps, the techniques you employ can drastically affect the outcomes of your analyses.
As you delve deeper into the world of data cleaning, you’ll discover that it’s not merely a technical task but also a critical thinking exercise. Understanding the context of your data and the implications of your cleaning choices can lead to more insightful analyses and better decision-making. By leveraging Python’s capabilities
Understanding the Importance of Data Cleaning
Data cleaning is a crucial step in the data preprocessing pipeline, ensuring that the data used for analysis or modeling is accurate, consistent, and reliable. Clean data enhances the quality of insights derived from data analysis and can significantly improve the performance of machine learning models.
Common issues that necessitate data cleaning include:
- Missing Values: Incomplete data entries can distort analysis.
- Duplicates: Redundant records may skew results.
- Inconsistent Formatting: Variations in data formats can lead to errors.
- Outliers: Extreme values can mislead statistical interpretations.
Techniques for Data Cleaning in Python
Python offers several libraries and techniques for effective data cleaning. The most widely used library for data manipulation is Pandas, which provides tools to handle various data issues.
Handling Missing Values
Missing data can be handled in several ways:
- Removal: Eliminate rows or columns with missing values.
- Imputation: Fill missing values using statistical methods, such as mean, median, or mode.
- Forward/Backward Fill: Use previous or next valid observations to fill gaps.
Example code for handling missing values:
“`python
import pandas as pd
Load dataset
df = pd.read_csv(‘data.csv’)
Remove rows with missing values
df_cleaned = df.dropna()
Impute missing values with mean
df[‘column_name’].fillna(df[‘column_name’].mean(), inplace=True)
“`
Removing Duplicates
Duplicated entries can be removed using the `drop_duplicates()` function in Pandas. This function identifies and removes duplicate rows based on all or specific columns.
Example code:
“`python
Remove duplicate rows
df_cleaned = df.drop_duplicates()
“`
Standardizing Data Formats
Inconsistent data formats can be standardized using string methods or datetime conversions. For example, converting all text to lowercase or formatting date columns uniformly can help ensure consistency.
Example code for standardizing text data:
“`python
Convert all entries in a column to lowercase
df[‘column_name’] = df[‘column_name’].str.lower()
“`
Identifying and Handling Outliers
Outliers can be detected using statistical methods such as Z-scores or IQR (Interquartile Range). Once identified, outliers can be removed or replaced based on the context of the analysis.
Example code to remove outliers using IQR:
“`python
Q1 = df[‘column_name’].quantile(0.25)
Q3 = df[‘column_name’].quantile(0.75)
IQR = Q3 – Q1
Remove outliers
df_cleaned = df[(df[‘column_name’] >= (Q1 – 1.5 * IQR)) & (df[‘column_name’] <= (Q3 + 1.5 * IQR))]
```
Summary of Data Cleaning Techniques
The following table summarizes key techniques for data cleaning in Python:
Technique | Description |
---|---|
Handling Missing Values | Remove or fill missing data using statistical methods. |
Removing Duplicates | Eliminate repeated entries from the dataset. |
Standardizing Formats | Ensure consistency in data formats across the dataset. |
Identifying Outliers | Detect and handle anomalies in the data. |
These techniques provide a comprehensive approach to cleaning data in Python, ensuring the dataset is ready for analysis or modeling.
Understanding Data Cleaning
Data cleaning is a crucial step in the data analysis process. It involves identifying and correcting errors or inconsistencies in data to improve its quality. This process ensures that the data is accurate, complete, and reliable for analysis.
Common Data Cleaning Techniques in Python
Python provides various libraries and methods for effective data cleaning. Here are some widely used techniques:
- Handling Missing Values:
- Use `pandas` to identify missing values:
“`python
import pandas as pd
df.isnull().sum()
“`
- Fill missing values with the mean, median, or mode:
“`python
df[‘column_name’].fillna(df[‘column_name’].mean(), inplace=True)
“`
- Removing Duplicates:
- Identify and remove duplicate entries:
“`python
df.drop_duplicates(inplace=True)
“`
- Filtering Outliers:
- Use statistical methods to detect outliers, such as Z-score or IQR:
“`python
from scipy import stats
df = df[(np.abs(stats.zscore(df[‘column_name’])) < 3)]
```
Utilizing Libraries for Data Cleaning
Several Python libraries can assist in the data cleaning process. Below is a table summarizing key libraries and their functionalities:
Library | Functionality |
---|---|
Pandas | Data manipulation and analysis |
NumPy | Numerical operations and array handling |
OpenCV | Image data cleaning |
Scikit-learn | Preprocessing tools for machine learning |
Numpy | Array manipulation and mathematical functions |
Example of Data Cleaning Workflow
An effective data cleaning workflow typically follows these steps:
- Loading the Data:
“`python
df = pd.read_csv(‘data.csv’)
“`
- Exploratory Data Analysis (EDA):
- Understand data distribution using visualizations like histograms and box plots.
- Identifying and Handling Missing Values:
- Use descriptive statistics and visualizations to understand the extent of missing data.
- Data Transformation:
- Normalize or standardize data if necessary:
“`python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
df[[‘column_name’]] = scaler.fit_transform(df[[‘column_name’]])
“`
- Data Type Conversion:
- Ensure columns have appropriate data types:
“`python
df[‘date_column’] = pd.to_datetime(df[‘date_column’])
“`
- Final Review:
- Check the cleaned data for any remaining issues before analysis.
Best Practices for Data Cleaning
Implementing best practices can enhance the efficiency and effectiveness of your data cleaning efforts:
- Document the Cleaning Process: Maintain clear documentation of all cleaning steps taken for reproducibility.
- Automate Repetitive Tasks: Use functions to automate common cleaning tasks, reducing manual errors.
- Collaborate with Domain Experts: Engage with stakeholders to understand the context and nuances of the data.
- Test for Consistency: Regularly validate the cleaned data against known benchmarks or datasets.
By following these guidelines, you can ensure a robust data cleaning process in Python that enhances the integrity and usability of your datasets.
Expert Insights on Data Cleaning Techniques in Python
Dr. Lisa Chen (Data Scientist, Analytics Innovations). “Data cleaning in Python is essential for ensuring the integrity of your analysis. Libraries such as Pandas provide powerful tools for handling missing values, removing duplicates, and transforming data types, which are critical steps in preparing your dataset for further analysis.”
James Patel (Machine Learning Engineer, Tech Solutions Inc.). “When cleaning data in Python, it’s important to adopt a systematic approach. Utilizing functions like `dropna()` for missing values and `replace()` for correcting anomalies can significantly enhance the quality of your dataset. Always visualize your data before and after cleaning to understand the impact of your changes.”
Sarah Gomez (Data Analyst, Insightful Analytics). “I recommend using the `clean` and `transform` methods from the `pandas` library to streamline the data cleaning process in Python. Additionally, integrating validation checks during the cleaning phase can help catch errors early, ensuring that the final dataset is both accurate and reliable for analysis.”
Frequently Asked Questions (FAQs)
How can I remove missing values from a dataset in Python?
You can remove missing values using the `dropna()` method from the Pandas library. This method allows you to drop rows or columns with missing data based on your specified criteria.
What libraries are commonly used for data cleaning in Python?
The most commonly used libraries for data cleaning in Python include Pandas, NumPy, and OpenRefine. These libraries provide various functions to manipulate and clean data effectively.
How do I handle outliers in my dataset using Python?
Outliers can be handled by identifying them using statistical methods such as Z-scores or IQR (Interquartile Range). Once identified, you can choose to remove them or transform them based on your analysis requirements.
What is the purpose of the `fillna()` function in Pandas?
The `fillna()` function in Pandas is used to fill missing values in a DataFrame with specified values, such as the mean, median, or a constant value, thereby maintaining the integrity of the dataset.
How can I standardize or normalize data in Python?
Data can be standardized or normalized using the `StandardScaler` or `MinMaxScaler` from the Scikit-learn library. Standardization transforms data to have a mean of zero and a standard deviation of one, while normalization scales data to a range of [0, 1].
What are some common data cleaning techniques in Python?
Common data cleaning techniques include removing duplicates, handling missing values, correcting data types, standardizing formats, and filtering out irrelevant data. These techniques ensure the dataset is accurate and ready for analysis.
Cleaning data in Python is an essential step in the data analysis process, as it ensures that the dataset is accurate, consistent, and ready for analysis. The process typically involves several key steps, including handling missing values, removing duplicates, correcting data types, and addressing inconsistencies in the data. Libraries such as Pandas and NumPy are invaluable tools for performing these tasks efficiently, allowing users to manipulate and clean their datasets with ease.
One of the primary techniques for cleaning data is the identification and treatment of missing values. This can involve filling in missing entries with appropriate values, such as the mean or median, or removing rows or columns that contain excessive missing data. Additionally, removing duplicate records helps maintain the integrity of the dataset, ensuring that each entry is unique and contributes meaningful information to the analysis.
Another significant aspect of data cleaning is the correction of data types and formats. Ensuring that each column in a dataset is of the correct data type—such as integers, floats, or strings—is crucial for accurate analysis. Furthermore, standardizing formats, such as date formats or categorical variables, enhances the consistency of the dataset and facilitates easier analysis and visualization.
mastering data cleaning techniques in Python is vital for
Author Profile
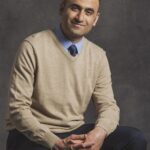
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?