How Can You Effectively Clean a Dataset in Python?
In the world of data science, the quality of your dataset can make or break your analysis. A clean dataset is the foundation upon which insightful conclusions are built, yet many practitioners find themselves grappling with messy, incomplete, or inconsistent data. Whether you’re a seasoned data scientist or a newcomer eager to dive into the realm of data analysis, mastering the art of dataset cleaning in Python is an essential skill that can elevate your projects and enhance your understanding of data manipulation.
Cleaning a dataset involves a series of systematic steps aimed at identifying and rectifying issues such as missing values, duplicates, and erroneous entries. Python, with its robust libraries like Pandas and NumPy, offers powerful tools to streamline this process. By leveraging these libraries, you can efficiently transform raw data into a polished product, ready for analysis. Understanding the common pitfalls and best practices in dataset cleaning not only saves time but also ensures that your analyses yield accurate and reliable results.
As you embark on this journey to clean your dataset in Python, you’ll discover a wealth of techniques and methodologies that can be applied to various types of data. From basic operations like filtering and sorting to more advanced strategies such as outlier detection and data normalization, the possibilities are vast. By honing your skills in dataset cleaning, you’ll empower yourself to unlock the
Identifying Missing Values
Identifying missing values is a crucial step in data cleaning. Missing data can skew results and lead to misleading interpretations. In Python, the `pandas` library provides efficient methods to detect and handle missing values.
To identify missing values in a DataFrame, the `isnull()` function can be employed. This function returns a DataFrame of the same shape as the original, indicating `True` for missing values and “ for non-missing values.
Here is how to use it:
“`python
import pandas as pd
df = pd.read_csv(‘data.csv’)
missing_values = df.isnull()
print(missing_values)
“`
To obtain a summary of missing values in each column, you can use the `sum()` function combined with `isnull()`:
“`python
missing_summary = df.isnull().sum()
print(missing_summary)
“`
Handling Missing Values
After identifying missing values, the next step is to decide how to handle them. There are several common strategies:
- Removing Missing Values: This is suitable when the dataset is large, and the proportion of missing values is small. You can use:
“`python
df_cleaned = df.dropna()
“`
- Imputing Missing Values: If you choose to fill in missing values, you can use methods such as mean, median, or mode imputation. For example, to fill missing values with the mean:
“`python
df[‘column_name’].fillna(df[‘column_name’].mean(), inplace=True)
“`
- Using Interpolation: This method estimates missing values based on other data points. For instance:
“`python
df[‘column_name’].interpolate(method=’linear’, inplace=True)
“`
Removing Duplicates
Duplicates can lead to biased analysis. The `drop_duplicates()` function in `pandas` is a straightforward way to remove duplicate rows in a DataFrame. Here’s an example:
“`python
df_unique = df.drop_duplicates()
“`
You can also specify which columns to consider for identifying duplicates:
“`python
df_unique = df.drop_duplicates(subset=[‘column1’, ‘column2’])
“`
Standardizing Data Formats
Data often comes in various formats, especially when collected from different sources. Standardizing formats ensures consistency and accuracy. This can include:
- Date Formats: Convert all date entries to a standard format using `pd.to_datetime()`:
“`python
df[‘date_column’] = pd.to_datetime(df[‘date_column’], format=’%Y-%m-%d’)
“`
- String Case Normalization: To ensure consistent casing, you can convert strings to lowercase:
“`python
df[‘text_column’] = df[‘text_column’].str.lower()
“`
- Trimming Whitespace: Remove leading and trailing whitespace from string entries:
“`python
df[‘text_column’] = df[‘text_column’].str.strip()
“`
Data Type Conversion
Ensuring that each column has the correct data type is essential for effective analysis. The `astype()` method in `pandas` allows for easy conversion:
“`python
df[‘numeric_column’] = df[‘numeric_column’].astype(float)
df[‘categorical_column’] = df[‘categorical_column’].astype(‘category’)
“`
You can check the data types of all columns using:
“`python
print(df.dtypes)
“`
Example of a Cleaned DataFrame
The following table illustrates a simple example of a cleaned DataFrame after applying the aforementioned techniques.
Column Name | Original Values | Cleaned Values |
---|---|---|
Date | 01/01/2020, 02/02/2020, NaT | 2020-01-01, 2020-02-02, 2020-01-01 (imputed) |
Category | Cat, Dog, cat, | cat, dog, cat |
Sales | 100, NaN, 150 | 100, 125 (imputed), 150 |
By systematically applying these techniques, you can effectively clean your dataset, leading to more reliable analyses and insights.
Understanding the Importance of Data Cleaning
Data cleaning is crucial in data analysis as it ensures the accuracy, consistency, and reliability of the dataset. A well-cleaned dataset leads to better modeling results and insights. The common issues that necessitate data cleaning include:
- Missing values
- Duplicates
- Incorrect data types
- Outliers
- Irrelevant features
Identifying Missing Values
Missing values can skew results and lead to misleading conclusions. In Python, the `pandas` library provides tools to identify and handle these values effectively.
“`python
import pandas as pd
Load dataset
df = pd.read_csv(‘your_dataset.csv’)
Check for missing values
missing_values = df.isnull().sum()
print(missing_values)
“`
To handle missing values, consider the following strategies:
- Remove rows with missing values:
“`python
df.dropna(inplace=True)
“`
- Fill missing values with a specific value or statistic:
“`python
df.fillna(0, inplace=True) Replace with 0
df.fillna(df.mean(), inplace=True) Replace with mean
“`
Removing Duplicates
Duplicates can distort analysis and lead to inaccurate results. You can easily identify and remove duplicates using `pandas`.
“`python
Identify duplicates
duplicates = df.duplicated().sum()
print(f’Duplicates: {duplicates}’)
Remove duplicates
df.drop_duplicates(inplace=True)
“`
Correcting Data Types
Data types must be appropriate for analysis. For instance, categorical data should be of type `category`, and numerical data should be in integer or float format.
“`python
Check data types
print(df.dtypes)
Convert data types
df[‘column_name’] = df[‘column_name’].astype(‘category’)
df[‘numeric_column’] = pd.to_numeric(df[‘numeric_column’], errors=’coerce’)
“`
Handling Outliers
Outliers can significantly affect statistical analyses. Identifying outliers can be done using methods such as the Z-score or IQR.
“`python
Using IQR to identify outliers
Q1 = df[‘column_name’].quantile(0.25)
Q3 = df[‘column_name’].quantile(0.75)
IQR = Q3 – Q1
Filter out outliers
df = df[(df[‘column_name’] >= (Q1 – 1.5 * IQR)) & (df[‘column_name’] <= (Q3 + 1.5 * IQR))]
```
Standardizing and Normalizing Data
Standardization and normalization can improve model performance. Use `StandardScaler` for standardization and `MinMaxScaler` for normalization.
“`python
from sklearn.preprocessing import StandardScaler, MinMaxScaler
Standardization
scaler = StandardScaler()
df[[‘numeric_column’]] = scaler.fit_transform(df[[‘numeric_column’]])
Normalization
min_max_scaler = MinMaxScaler()
df[[‘numeric_column’]] = min_max_scaler.fit_transform(df[[‘numeric_column’]])
“`
Encoding Categorical Variables
Categorical variables must be encoded to be used in machine learning models. Common methods include One-Hot Encoding and Label Encoding.
“`python
One-Hot Encoding
df = pd.get_dummies(df, columns=[‘categorical_column’], drop_first=True)
Label Encoding
from sklearn.preprocessing import LabelEncoder
le = LabelEncoder()
df[‘categorical_column’] = le.fit_transform(df[‘categorical_column’])
“`
Final Checks and Exporting Cleaned Data
After cleaning the dataset, conduct final checks for consistency and completeness.
“`python
Final checks
print(df.info())
Export cleaned dataset
df.to_csv(‘cleaned_dataset.csv’, index=)
“`
Expert Strategies for Cleaning Datasets in Python
Dr. Emily Carter (Data Scientist, Insight Analytics Group). “When cleaning datasets in Python, it is crucial to utilize libraries such as Pandas for efficient data manipulation. Start by identifying and handling missing values, either by imputation or removal, and ensure to standardize data formats to maintain consistency across the dataset.”
Michael Chen (Machine Learning Engineer, Tech Innovations Inc.). “Data cleaning is a foundational step in any data analysis process. I recommend using the ‘drop_duplicates()’ function in Pandas to eliminate redundant entries, followed by applying ‘str.replace()’ for correcting inconsistent text formats, which often leads to improved model performance.”
Sarah Johnson (Big Data Consultant, Future Data Solutions). “An effective data cleaning strategy in Python involves not just fixing errors but also understanding the context of the data. Employing visualization tools like Matplotlib or Seaborn can help identify outliers and anomalies, allowing for more informed decisions on how to handle them during the cleaning process.”
Frequently Asked Questions (FAQs)
What are the common steps to clean a dataset in Python?
The common steps include handling missing values, removing duplicates, correcting data types, normalizing or standardizing data, and addressing outliers. Libraries such as Pandas are typically used for these tasks.
How can I handle missing values in a dataset using Python?
Missing values can be handled by using methods such as imputation (filling in missing values with mean, median, or mode), dropping rows or columns with missing values, or using interpolation techniques. The Pandas library provides functions like `fillna()` and `dropna()` for this purpose.
What libraries are recommended for data cleaning in Python?
The most recommended libraries for data cleaning in Python are Pandas for data manipulation, NumPy for numerical operations, and Scikit-learn for preprocessing tasks. Additionally, libraries like OpenRefine can be useful for more complex cleaning tasks.
How can I remove duplicates from a dataset in Python?
To remove duplicates, you can use the `drop_duplicates()` function from the Pandas library. This function allows you to specify which columns to consider for identifying duplicates and whether to keep the first or last occurrence.
What techniques can be used to standardize data in Python?
Data can be standardized using techniques such as Min-Max scaling or Z-score normalization. The `StandardScaler` and `MinMaxScaler` classes from the Scikit-learn library can be employed to achieve this.
How do I detect and handle outliers in a dataset using Python?
Outliers can be detected using statistical methods such as the Z-score method or IQR (Interquartile Range) method. Once identified, outliers can be handled by removing them, transforming the data, or capping their values. Libraries like Pandas and NumPy facilitate these operations.
Cleaning a dataset in Python is a critical step in the data analysis process, ensuring that the data is accurate, consistent, and usable. The process typically involves several key tasks, including handling missing values, correcting data types, removing duplicates, and addressing outliers. Utilizing libraries such as Pandas and NumPy significantly streamlines these tasks, providing powerful tools for data manipulation and analysis.
One of the primary insights from the discussion on dataset cleaning is the importance of understanding the nature of the data before applying any cleaning techniques. This involves exploratory data analysis (EDA) to identify issues such as missing entries or inconsistencies. By gaining a comprehensive understanding of the dataset, practitioners can make informed decisions on the most appropriate cleaning methods to apply, thereby enhancing the quality of the analysis.
Additionally, automation of the cleaning process can save considerable time and effort. By writing reusable functions or utilizing data cleaning libraries, data scientists can efficiently manage large datasets and ensure that cleaning procedures are consistently applied. This not only improves efficiency but also helps maintain the integrity of the data throughout the analysis process.
effective dataset cleaning in Python is essential for producing reliable and accurate results in data analysis. By leveraging the right tools and methodologies, data professionals
Author Profile
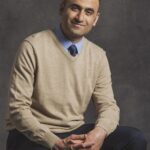
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?