How Can You Effectively Clear a JavaScript Array?
### Introduction
In the dynamic world of JavaScript programming, managing data efficiently is crucial for optimal performance and functionality. Arrays, a fundamental data structure, play a pivotal role in storing collections of data. However, there are times when you may find yourself needing to clear an array, whether to reset its contents for new data or to free up memory. Understanding how to clear a JavaScript array effectively can enhance your coding skills and streamline your applications. In this article, we will explore various methods to clear arrays, each with its own advantages and use cases, ensuring you have the right tools at your disposal for any programming challenge.
When it comes to clearing a JavaScript array, developers have several options at their fingertips. Each method varies in terms of performance and implications for memory management, making it essential to choose the right approach based on your specific scenario. From simple assignments to more advanced techniques, the way you clear an array can impact the behavior of your code, especially when dealing with references and shared data structures.
As we delve deeper into this topic, you’ll discover not only how to clear an array but also the nuances of each method. By the end of this article, you will be equipped with a comprehensive understanding of the best practices for managing arrays in JavaScript, enabling you
Methods to Clear a JavaScript Array
Clearing a JavaScript array can be accomplished in several ways, each with its own implications regarding performance and memory management. Below are the most common methods.
Using the Length Property
One of the simplest ways to clear an array is by setting its length property to zero. This method is efficient and immediately removes all elements from the array.
javascript
let arr = [1, 2, 3, 4, 5];
arr.length = 0;
console.log(arr); // Output: []
Using the splice() Method
The `splice()` method can also be employed to remove all elements from an array. This method is useful if you need to retain the array reference but want to empty its contents.
javascript
let arr = [1, 2, 3, 4, 5];
arr.splice(0, arr.length);
console.log(arr); // Output: []
Using the pop() Method in a Loop
Another approach is to use a loop that continuously calls the `pop()` method until the array is empty. This method is less efficient but demonstrates how elements can be removed one at a time.
javascript
let arr = [1, 2, 3, 4, 5];
while (arr.length > 0) {
arr.pop();
}
console.log(arr); // Output: []
Using the filter() Method
You can also clear an array by using the `filter()` method. This method allows for a more functional programming approach but may not be as intuitive for simply clearing an array.
javascript
let arr = [1, 2, 3, 4, 5];
arr = arr.filter(() => );
console.log(arr); // Output: []
Performance Comparison
The table below summarizes the performance characteristics of the different methods discussed:
Method | Time Complexity | Memory Management |
---|---|---|
Length Property | O(1) | Releases memory immediately |
splice() | O(n) | Releases memory after operation |
pop() in Loop | O(n) | Releases memory gradually |
filter() | O(n) | Creates a new array, old memory released after GC |
Each method has its own context of use depending on the specific requirements of your application, such as performance needs or memory management considerations.
Methods to Clear a JavaScript Array
Clearing a JavaScript array can be accomplished through several methods, each with its own advantages and use cases. Below are the most common techniques:
Using the Length Property
One of the simplest methods to clear an array is by setting its `length` property to `0`. This effectively removes all elements from the array.
javascript
let arr = [1, 2, 3, 4];
arr.length = 0; // arr is now []
Advantages:
- Very efficient, as it directly modifies the existing array.
- No need for additional methods or functions.
Using the Splice Method
The `splice()` method can also be utilized to remove elements from an array. By specifying the starting index and the number of elements to remove, you can clear the entire array.
javascript
let arr = [1, 2, 3, 4];
arr.splice(0, arr.length); // arr is now []
Advantages:
- Allows for more control over which elements to remove.
- Can be used in scenarios where partial clearing is needed.
Using the Shift Method in a Loop
For scenarios where you may want to process elements before clearing, the `shift()` method can be applied in a loop until the array is empty.
javascript
let arr = [1, 2, 3, 4];
while (arr.length > 0) {
arr.shift(); // Removes the first element repeatedly
} // arr is now []
Advantages:
- Useful when additional processing on each element is required before removal.
Assigning a New Empty Array
Another straightforward method is to simply assign a new empty array to the variable. This approach, however, only clears the reference for that specific variable.
javascript
let arr = [1, 2, 3, 4];
arr = []; // arr now points to a new empty array
Disadvantages:
- This does not affect other references to the original array. Any other variables referencing the original array will remain unchanged.
Using the Filter Method
The `filter()` method can be applied to create a new array based on a condition. To clear an array, you can filter for a condition that will never be true.
javascript
let arr = [1, 2, 3, 4];
arr = arr.filter(() => ); // arr is now []
Advantages:
- Functional programming approach that can be integrated into larger codebases.
Comparison of Methods
Method | Modifies Original Array | Creates New Array | Performance |
---|---|---|---|
Length Property | Yes | No | Very High |
Splice | Yes | No | High |
Shift in a Loop | Yes | No | Moderate |
Assigning New Array | No | Yes | High |
Filter | No | Yes | Moderate |
Each method has its specific use cases and should be chosen based on the needs of the application and the desired outcome.
Expert Insights on Clearing JavaScript Arrays
Emily Carter (Senior JavaScript Developer, CodeCraft Solutions). “To clear a JavaScript array efficiently, one can simply set its length property to zero. This method is both straightforward and effective, ensuring that the array is emptied without creating a new reference.”
James Liu (Lead Software Engineer, Tech Innovations Inc.). “Another common approach to clear an array is to use the splice method. By invoking array.splice(0, array.length), developers can remove all elements while maintaining the original array reference, which is crucial for certain applications.”
Maria Gonzalez (JavaScript Educator, WebDev Academy). “Using the array.length = 0 method is often recommended for its simplicity, but it is essential to consider the context. If other references to the array exist, they will still point to the original data, which may lead to unintended side effects.”
Frequently Asked Questions (FAQs)
How can I clear a JavaScript array?
You can clear a JavaScript array by setting its length property to zero, using the `array.length = 0;` method. This effectively removes all elements from the array.
Is there a method to remove all elements from an array without altering its reference?
Yes, you can use the `splice` method. For example, `array.splice(0, array.length);` will remove all elements while keeping the original reference intact.
Can I use the `pop` method in a loop to clear an array?
Yes, you can use a loop with the `pop` method to remove elements one by one. However, this method is less efficient than setting the length to zero or using `splice`.
What is the difference between setting the length to zero and using `splice`?
Setting the length to zero is a faster operation as it directly modifies the array’s length property. In contrast, `splice` creates a new array with the specified elements removed, which may be less efficient.
Will clearing an array affect other references to it?
If you clear an array by modifying its length or using `splice`, all references to that array will reflect the changes since they point to the same object in memory.
Are there any performance considerations when clearing large arrays?
Yes, for large arrays, setting the length to zero is generally more efficient than using methods like `splice` or `pop` in a loop, as it minimizes the number of operations performed on the array.
Clearing a JavaScript array can be accomplished through several methods, each with its own advantages and implications. The most common techniques include setting the length of the array to zero, using the splice method, and reassigning the array to a new empty array. Each of these methods effectively removes all elements from the array, but they may differ in performance and behavior, especially in the context of references to the original array.
One of the simplest and most efficient ways to clear an array is by setting its length property to zero. This method is straightforward and retains the original array reference, which is particularly useful when other parts of your code hold references to the same array. Alternatively, the splice method allows for more granular control over which elements to remove, but it is generally less efficient for clearing an entire array.
Reassigning the array to a new empty array is another viable approach, but it results in the loss of the original reference. This can lead to issues if other variables or functions depend on the original array. Therefore, it is essential to choose the method that best fits the specific requirements of your application, considering factors such as performance, memory management, and reference integrity.
Author Profile
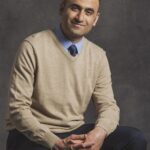
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?