How Can You Clear a Screen in Python? A Step-by-Step Guide
In the world of programming, clarity is key. Whether you’re developing a game, building a user interface, or simply running scripts in a terminal, the ability to manage your screen output can significantly enhance the user experience. One common task that programmers often encounter is the need to clear the screen in Python. This seemingly simple action can help maintain focus, reduce clutter, and provide a fresh slate for new information. But how exactly do you achieve this in Python?
Clearing the screen in Python can be approached in various ways, depending on the environment in which your code is running. For instance, if you’re working in a command-line interface, there are specific commands that can be executed to refresh the display. On the other hand, if you’re developing a graphical user interface, the method may differ entirely. Understanding these distinctions is crucial for effective screen management and can help streamline your coding process.
Moreover, the choice of method to clear the screen can also impact the performance and responsiveness of your application. As you delve deeper into the topic, you’ll discover not only the different techniques available but also best practices that can enhance your coding efficiency. Whether you’re a beginner looking to tidy up your console output or an experienced developer aiming to optimize your applications, mastering the art of screen clearing in Python is an
Using OS Module to Clear Screen
One of the most common methods to clear the console screen in Python is by using the `os` module. This approach allows you to run system commands that are dependent on the operating system.
To implement this, you can use the following code snippet:
“`python
import os
def clear_screen():
Check the operating system
if os.name == ‘nt’: For Windows
os.system(‘cls’)
else: For macOS and Linux
os.system(‘clear’)
“`
This function checks the operating system using `os.name`. If the operating system is Windows (`’nt’`), it executes the `cls` command; otherwise, it runs the `clear` command for Unix-based systems.
Using ANSI Escape Sequences
Another method to clear the terminal screen is by using ANSI escape sequences. This technique is particularly useful for environments that support ANSI codes, such as many Linux terminals and modern Windows terminals.
You can clear the screen with the following code:
“`python
def clear_screen():
print(“\033[H\033[J”)
“`
The escape sequence `\033[H` moves the cursor to the top-left corner, and `\033[J` clears the screen from the cursor down to the bottom.
Using a Custom Function
For more control over the clearing process, you can create a custom function that combines different approaches. Below is an example of a more robust solution:
“`python
def clear_screen():
try:
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
except Exception as e:
print(“Error clearing screen:”, e)
“`
This function attempts to clear the screen and handles any exceptions that may arise during the operation.
Comparison of Methods
Here’s a table that summarizes the different methods of clearing the screen in Python:
Method | Operating System | Code Example | Usage |
---|---|---|---|
OS Module | Windows, macOS, Linux | os.system('cls'/'clear') |
General purpose |
ANSI Escape Sequences | Linux, macOS, modern Windows | print("\033[H\033[J") |
Terminal with ANSI support |
Custom Function | Windows, macOS, Linux | def clear_screen(): ... |
More control and error handling |
Each method has its own advantages and limitations, and the choice of which to use depends on the specific requirements of your application and the environment in which it runs.
Methods to Clear the Screen in Python
Clearing the screen in Python can be achieved using various methods, depending on the operating system and the context in which the Python script is running. Below are several common approaches for both console-based and GUI applications.
Clearing the Console Screen
To clear the console screen, the method used often varies between Windows and Unix-based systems (Linux, macOS). Here are the most effective techniques:
Using OS Commands
Python’s built-in `os` module can be utilized to execute system commands for clearing the screen.
- For Windows:
“`python
import os
os.system(‘cls’)
“`
- For Unix/Linux/MacOS:
“`python
import os
os.system(‘clear’)
“`
Creating a Cross-Platform Function
To make a single function that works across platforms, consider the following implementation:
“`python
import os
def clear_screen():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This function checks the operating system and executes the appropriate command to clear the screen.
Clearing the Screen in IDLE or Jupyter Notebooks
When working within environments like IDLE or Jupyter Notebooks, the methods above may not work as expected. Here are alternative strategies:
- In IDLE:
There isn’t a direct command to clear the screen. However, you can use:
“`python
from IPython.display import clear_output
clear_output(wait=True)
“`
- In Jupyter Notebooks:
Use the same `clear_output` function:
“`python
from IPython.display import clear_output
clear_output(wait=True)
“`
This will clear the output of the current cell, providing a more organized view.
Clearing the Screen in GUI Applications
For GUI applications using libraries like Tkinter, you might want to clear the content of a window rather than the entire screen. Here’s how:
“`python
import tkinter as tk
def clear_window(window):
for widget in window.winfo_children():
widget.destroy()
“`
This function will remove all widgets from the specified Tkinter window, effectively clearing it for new content.
Using ANSI Escape Codes
For terminal applications that support ANSI escape codes, you can clear the screen by printing an escape sequence:
“`python
print(“\033[H\033[J”)
“`
This sequence moves the cursor to the top left of the terminal and clears the screen.
Performance Considerations
When implementing screen clearing in a loop or a frequently called function, consider the following:
- Avoid excessive use of screen clearing, as it can affect performance and user experience.
- Use it judiciously to enhance readability without overwhelming the user with constant changes.
By selecting the appropriate method based on your development environment and requirements, you can effectively manage screen output in your Python applications.
Expert Insights on Clearing a Screen in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). “To clear a screen in Python, one commonly used method is to utilize the `os` module. By calling `os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)`, developers can effectively clear the console screen across different operating systems.”
James Liu (Python Developer and Educator, Tech Academy). “In interactive Python environments like Jupyter Notebooks, the command `from IPython.display import clear_output; clear_output(wait=True)` serves as an efficient way to clear the output area, allowing for a cleaner presentation of results.”
Linda Thompson (Lead Python Instructor, CodeMaster Training). “For beginners, it is essential to understand that while clearing the screen can enhance readability, it is not a built-in function in Python. Instead, leveraging system commands or specific libraries is crucial for achieving this functionality.”
Frequently Asked Questions (FAQs)
How can I clear the console screen in Python on Windows?
To clear the console screen in Python on Windows, you can use the command `os.system(‘cls’)`. Ensure you import the `os` module before executing this command.
What command is used to clear the screen in Python on Unix/Linux systems?
On Unix/Linux systems, you can clear the screen by executing `os.system(‘clear’)`. This command will refresh the terminal display.
Is there a cross-platform way to clear the screen in Python?
Yes, you can use the following code snippet for a cross-platform solution:
“`python
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This checks the operating system and executes the appropriate command.
Can I clear the screen in a Python script without using the os module?
While the `os` module is the most common method, you can also use libraries like `curses` for terminal manipulation, but this is more complex and not recommended for simple screen clearing.
Does clearing the screen affect the program’s execution in Python?
No, clearing the screen does not affect the execution of the program. It merely removes the previous output from the console, allowing for a cleaner display of new information.
Are there any third-party libraries for clearing the screen in Python?
Yes, libraries like `colorama` or `rich` can provide enhanced terminal capabilities, including screen clearing, but they are not specifically designed just for that purpose.
Clearing the screen in Python can be accomplished through various methods, depending on the operating system and the environment in which the code is executed. The most common approaches include using the ‘os’ module to execute system commands or utilizing libraries like ‘curses’ for more advanced terminal control. For instance, on Windows, the command ‘cls’ is used, while on Unix-like systems, ‘clear’ serves the same purpose. Understanding these distinctions is crucial for effective screen management in Python applications.
Another important aspect to consider is the context in which the screen clearing is required. In interactive console applications, clearing the screen can enhance user experience by providing a clean slate for output. However, in graphical user interfaces (GUIs), such as those created with Tkinter or Pygame, screen clearing is typically handled through specific methods provided by those libraries, rather than through system commands.
In summary, the method chosen to clear a screen in Python should align with the specific requirements of the project and the environment. By leveraging the appropriate techniques, developers can ensure that their applications maintain a professional appearance and provide a seamless user experience. Mastery of these methods not only improves the functionality of Python programs but also enhances the overall efficiency of code execution.
Author Profile
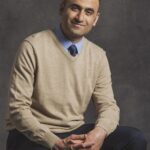
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?