How Can You Easily Clear an Array in JavaScript?
### Introduction
In the dynamic world of JavaScript programming, arrays serve as a fundamental data structure, enabling developers to store and manipulate collections of items with ease. However, there are times when you may find yourself needing to clear an array, whether to free up memory, reset values, or prepare for new data. Understanding how to efficiently clear an array is an essential skill that can enhance your coding efficiency and streamline your applications. In this article, we will explore various methods to clear an array in JavaScript, equipping you with the knowledge to choose the best approach for your specific needs.
When it comes to clearing an array, JavaScript offers several techniques that vary in efficiency and readability. From setting the length of the array to zero, to using built-in methods, each approach has its own advantages and potential drawbacks. As you delve deeper into the nuances of these methods, you’ll discover how to effectively manage array data and optimize your code for performance.
Moreover, understanding the implications of each method is crucial, especially in scenarios where references to the original array might still exist. By the end of this article, you will not only know how to clear an array but also understand the best practices to ensure your code remains clean and maintainable. Get ready to unlock the secrets of array
Methods to Clear an Array in JavaScript
There are several ways to clear an array in JavaScript, each with its own use cases and implications for performance and memory management. Below are the most common methods.
Using Length Property
One of the simplest and most efficient ways to clear an array is by setting its `length` property to `0`. This method directly modifies the original array and is efficient in terms of memory usage.
javascript
let arr = [1, 2, 3, 4, 5];
arr.length = 0;
console.log(arr); // Output: []
Using Splice Method
The `splice()` method can be employed to remove elements from the array. By specifying the starting index as `0` and the number of elements to remove as the length of the array, you can effectively clear the array.
javascript
let arr = [1, 2, 3, 4, 5];
arr.splice(0, arr.length);
console.log(arr); // Output: []
Using the Pop Method in a Loop
You can also clear an array by repeatedly using the `pop()` method until the array is empty. This approach is less efficient, especially for large arrays, as it modifies the array one element at a time.
javascript
let arr = [1, 2, 3, 4, 5];
while (arr.length > 0) {
arr.pop();
}
console.log(arr); // Output: []
Using the Filter Method
If you want to create a new empty array while leaving the original array untouched, you can use the `filter()` method to filter out all elements, resulting in an empty array.
javascript
let arr = [1, 2, 3, 4, 5];
arr = arr.filter(() => );
console.log(arr); // Output: []
Comparison of Methods
The following table summarizes the different methods to clear an array, highlighting their efficiency and impact on the original array.
Method | Modifies Original Array | Efficiency |
---|---|---|
Length Property | Yes | High |
Splice Method | Yes | Medium |
Pop in Loop | Yes | Low |
Filter Method | No | Medium |
Choosing the right method to clear an array in JavaScript depends on the specific requirements of your application, such as whether you need to preserve the original array or prioritize performance. Each method has its advantages and is suited for different scenarios.
Methods to Clear an Array in JavaScript
JavaScript provides several effective methods to clear an array, each with its own use cases and implications. Below are the most common techniques.
Using the Length Property
One of the simplest ways to clear an array is by setting its `length` property to `0`. This method is straightforward and effective.
javascript
let array = [1, 2, 3, 4, 5];
array.length = 0; // Now array is []
Advantages:
- Direct and easy to understand.
- It modifies the original array.
Using the Splice Method
The `splice()` method can also be used to remove all elements from an array. This method is particularly useful if you want to maintain references to the original array.
javascript
let array = [1, 2, 3, 4, 5];
array.splice(0, array.length); // Now array is []
Advantages:
- Retains the original array reference.
- Can also be used to remove specific elements, if needed.
Using the Pop Method in a Loop
Another method involves using the `pop()` method in a loop until the array is empty. This approach is less efficient but demonstrates how to iteratively remove elements.
javascript
let array = [1, 2, 3, 4, 5];
while (array.length > 0) {
array.pop(); // Removes last element until array is empty
}
Advantages:
- Demonstrates the iterative removal of elements.
- Useful in scenarios where cleanup operations are needed for each element.
Reassigning to a New Array
You can also clear an array by reassigning it to a new empty array. This method does not affect other references to the original array.
javascript
let array = [1, 2, 3, 4, 5];
array = []; // array is now a new empty array
Advantages:
- Creates a new instance, freeing up memory.
- Does not alter existing references to the original array.
Comparison of Methods
Method | Modifies Original Array | Retains Reference | Performance |
---|---|---|---|
Length Property | Yes | Yes | Fast |
Splice | Yes | Yes | Fast |
Pop in Loop | Yes | Yes | Slower |
Reassigning | No | No | Fast |
Each method has its own merits depending on the specific requirements of your application, such as performance considerations and reference management.
Expert Insights on Clearing Arrays in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “To effectively clear an array in JavaScript, developers can utilize the array’s length property by setting it to zero. This method is efficient and maintains the reference to the original array, which is crucial in many applications.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Another approach to clear an array is to assign a new empty array to the variable. However, this method creates a new reference, which may lead to issues if other parts of the code rely on the original array reference.”
Sarah Johnson (JavaScript Educator, Web Development Academy). “Using the splice method is also a viable option for clearing an array. By invoking array.splice(0, array.length), developers can remove all elements while keeping the original reference intact, making it a versatile choice.”
Frequently Asked Questions (FAQs)
How can I clear an array in JavaScript?
You can clear an array in JavaScript by setting its length property to zero, like this: `array.length = 0;`. This effectively removes all elements from the array.
What is the difference between setting the length to zero and reassigning a new array?
Setting the length to zero modifies the original array in place, while reassigning a new array creates a new reference. If other references to the original array exist, they will not reflect the changes made by reassignment.
Can I use the splice method to clear an array?
Yes, you can use the splice method to clear an array by calling `array.splice(0, array.length);`. This removes all elements from the array starting from index 0.
Is there a performance difference between the methods to clear an array?
Setting the length to zero is generally more efficient than using splice or creating a new array, as it directly modifies the array without iterating through its elements.
What happens to the references of the original array when I clear it?
When you clear an array by setting its length to zero, all references to that array will see the cleared state. However, if you reassign a new array, other references will still point to the original array with its previous elements.
Are there any methods to clear an array that are considered best practice?
Using `array.length = 0;` is often considered the best practice for clearing an array due to its simplicity and efficiency. It is widely supported and clearly communicates the intent to clear the array.
In JavaScript, clearing an array can be accomplished through various methods, each with its own implications and use cases. The most common approaches include setting the array’s length to zero, assigning a new empty array to the variable, and using the splice method. Each method effectively removes all elements from the array, but they differ in terms of performance and impact on references to the original array.
One of the simplest and most efficient ways to clear an array is to set its length property to zero. This method is direct and modifies the original array in place, making it a suitable choice when other references to the array exist. Alternatively, reassigning the variable to a new empty array is straightforward but will not affect other references to the original array, which may lead to unintended consequences if those references are still in use.
Using the splice method is another viable option, as it allows for the removal of elements from the array while maintaining its reference. This method can be particularly useful when you want to clear an array while ensuring that any other variables referencing the same array remain updated. Ultimately, the choice of method will depend on the specific requirements of the application and the desired behavior regarding references and performance.
Author Profile
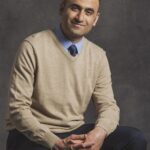
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?