How Can You Clear the Console in Python?
In the world of programming, maintaining a clean and organized workspace is crucial for efficiency and clarity. For Python developers, the console serves as a vital tool for executing code, debugging, and testing snippets of functionality. However, as you work through various iterations of your code, the console can quickly become cluttered with outputs, warnings, and error messages. This is where the ability to clear the console becomes not just a convenience, but a necessity. Whether you’re running scripts in an integrated development environment (IDE) or a terminal, knowing how to clear the console can enhance your coding experience and help you focus on what truly matters—your code.
Clearing the console in Python can vary depending on the environment you are using. For instance, the commands and methods differ between running scripts in a terminal, using an IDE like PyCharm, or working in a Jupyter Notebook. Understanding these nuances is essential for streamlining your workflow. Moreover, the ability to clear the console can aid in debugging by removing previous outputs that may distract from current results, allowing you to see only the most relevant information.
In this article, we will delve into the various methods for clearing the console in Python, equipping you with the knowledge to maintain a tidy workspace. Whether you’re a beginner looking
Methods to Clear Console in Python
When working in Python, especially during development or testing phases, it can be useful to clear the console output to enhance readability or to reset the display for new results. There are several methods to achieve this, depending on the environment you are using.
Clearing Console in Different Environments
The method for clearing the console can vary based on the operating system and the environment (like terminal, IDLE, or Jupyter Notebook) you are using. Below are some common approaches:
Using OS Commands
Python can execute operating system commands that clear the console. This is typically done using the `os` module.
- For Windows systems, you can use the following command:
“`python
import os
os.system(‘cls’)
“`
- For Unix/Linux/Mac systems, you would use:
“`python
import os
os.system(‘clear’)
“`
This method is straightforward and works effectively in terminal environments.
Using the print Function
In some cases, especially in interactive scripts, simply printing multiple new lines can simulate a clear screen, although this is not a true clear command.
“`python
print(“\n” * 100)
“`
While this does not actually clear the console, it pushes previous output off the screen, making it visually clearer.
Clearing Console in IDLE
IDLE, Python’s Integrated Development and Learning Environment, does not support the standard console clearing commands. Instead, you can clear the console by selecting the “Shell” menu and choosing “Restart Shell” or simply closing and reopening the Shell window.
Clearing Console in Jupyter Notebooks
In Jupyter Notebooks, you can clear the output of a cell by using the following command:
“`python
from IPython.display import clear_output
clear_output(wait=True)
“`
This command effectively removes all output from the cell, allowing you to start fresh without clutter.
Comparison of Methods
Method | Windows Command | Unix/Linux Command | Environment |
---|---|---|---|
OS Command (os.system) | `cls` | `clear` | Terminal |
Print New Lines | `print(“\n”*100)` | Not Applicable | Terminal, Interactive |
Restart Shell | Not Applicable | Not Applicable | IDLE |
Jupyter Clear Output | Not Applicable | Not Applicable | Jupyter Notebook |
Each of these methods serves a specific context, and choosing the right one depends on your development environment and personal preference. By utilizing these commands, you can enhance your workflow and maintain a clean console during your Python sessions.
Methods to Clear Console in Python
In Python, the method to clear the console varies depending on the operating system and the environment in which the code is executed. Below are the common approaches for different scenarios.
Using OS Commands
One of the simplest ways to clear the console is by invoking system commands through the `os` module.
For Windows:
“`python
import os
os.system(‘cls’)
“`
For Unix/Linux/Mac:
“`python
import os
os.system(‘clear’)
“`
This method works by executing the respective command to clear the terminal screen based on the OS.
Using a Function for Cross-Platform Compatibility
To create a reusable function that works on both Windows and Unix-like systems, you can define a function as follows:
“`python
import os
def clear_console():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This function checks the operating system and executes the appropriate command, allowing for seamless clearing of the console regardless of the environment.
Environment-Specific Methods
When working in specific environments, such as Jupyter notebooks or IDEs, the method to clear the console may differ.
Jupyter Notebook:
To clear the output in a Jupyter notebook, use the following code:
“`python
from IPython.display import clear_output
clear_output(wait=True)
“`
This clears the output area of the notebook and can be executed within any cell.
IDEs (like PyCharm or VSCode):
Most integrated development environments do not have a built-in command to clear the console via Python code. Instead, users typically rely on keyboard shortcuts:
- PyCharm: `Ctrl + K` (Windows/Linux) or `Cmd + K` (Mac)
- VSCode: `Ctrl + Shift + P`, then type “Clear Terminal”
Considerations for Interactive Python Shell
In interactive Python shells (like IDLE), clearing the console is not directly supported through Python code. Users can manually clear the console using the following keyboard shortcuts:
- IDLE: `Ctrl + L` (Windows/Linux) or `Cmd + L` (Mac)
Summary of Methods
Environment | Command/Function |
---|---|
Windows Terminal | `os.system(‘cls’)` |
Unix/Linux/Mac | `os.system(‘clear’)` |
Cross-Platform | `clear_console()` function |
Jupyter Notebook | `clear_output(wait=True)` |
IDLE | `Ctrl + L` |
PyCharm | `Ctrl + K` |
VSCode | `Ctrl + Shift + P` |
By utilizing the appropriate method based on your environment, you can efficiently manage the console output in Python.
Expert Insights on Clearing the Console in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Clearing the console in Python is often overlooked, yet it can significantly enhance the user experience during interactive sessions. Utilizing the ‘os’ module to call ‘os.system(“cls” if os.name == “nt” else “clear”)’ is a widely accepted method that works across different operating systems.”
James Liu (Lead Python Instructor, Code Academy). “For beginners, understanding how to clear the console can simplify debugging and improve code readability. I recommend using the ‘clear’ command in Unix-based systems or ‘cls’ in Windows, but be aware that this is not a built-in Python function; it requires importing the ‘os’ module.”
Sarah Mitchell (Data Scientist, Tech Innovations Inc.). “In data-driven applications, clearing the console can help in managing output clutter. Implementing a function to clear the console can streamline the presentation of results, especially when running multiple iterations of data analysis scripts.”
Frequently Asked Questions (FAQs)
How can I clear the console in Python on Windows?
To clear the console in Python on Windows, you can use the command `os.system(‘cls’)`. Ensure you import the `os` module before executing this command.
What command should I use to clear the console in Python on macOS or Linux?
On macOS or Linux, you can clear the console by using the command `os.system(‘clear’)`. Like with Windows, you need to import the `os` module first.
Is there a cross-platform way to clear the console in Python?
Yes, you can use the following code snippet for a cross-platform solution:
“`python
import os
import platform
def clear_console():
os.system(‘cls’ if platform.system() == ‘Windows’ else ‘clear’)
“`
This function will determine the operating system and execute the appropriate command.
Can I use a library to clear the console in Python?
Yes, libraries like `colorama` can be used to manage console output, but they do not directly provide a clear function. You can still use the `os` commands mentioned earlier.
What happens if I try to clear the console in an IDE like PyCharm or Jupyter Notebook?
In IDEs like PyCharm, the console may not clear using `os.system()` commands. Instead, you can use the built-in functionality of the IDE to clear the output. In Jupyter Notebook, you can use the command `from IPython.display import clear_output; clear_output()` to clear the output cell.
Are there any limitations to clearing the console in Python?
Yes, clearing the console may not work as expected in certain environments such as IDEs or integrated terminals, where the output is managed differently. Additionally, the clear command may not have a visual effect in some contexts, such as when running scripts in a non-interactive mode.
In Python, clearing the console can be achieved through various methods, depending on the operating system and the environment in which the code is executed. For instance, in a Windows environment, the command `os.system(‘cls’)` is commonly used, while in Unix-based systems like macOS and Linux, the command `os.system(‘clear’)` serves the same purpose. These commands utilize the operating system’s built-in functionalities to refresh the console display, thereby removing previous outputs.
Additionally, users working within integrated development environments (IDEs) or interactive shells may encounter different methods for clearing the console. For example, many IDEs, such as PyCharm or Jupyter Notebook, provide built-in options or shortcuts that allow users to clear the output window or console without needing to write specific code. Understanding these nuances can enhance the user experience and streamline the coding process.
In summary, while clearing the console in Python is straightforward, it is essential to choose the appropriate method based on the user’s environment. Familiarity with the commands and features specific to the operating system and IDE can significantly improve efficiency and productivity during development. By leveraging these techniques, programmers can maintain a clean workspace that facilitates better focus and organization in their coding tasks.
Author Profile
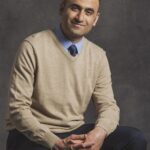
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?