How Can You Clear an Array in JavaScript Effectively?
### Introduction
In the dynamic world of JavaScript programming, arrays serve as one of the most fundamental data structures, allowing developers to store and manipulate collections of data with ease. However, as projects evolve and requirements change, there often comes a time when you need to clear an array—removing all its elements to start fresh. Whether you’re optimizing memory usage, resetting state in a web application, or simply managing data more efficiently, knowing how to clear an array effectively is an essential skill for any JavaScript developer. In this article, we will explore various methods to achieve this, ensuring you have the right tools at your disposal for any coding scenario.
When it comes to clearing an array in JavaScript, there are several approaches you can take, each with its own advantages and use cases. From simple assignment techniques to more robust methods that preserve references, understanding these options will empower you to choose the most suitable one for your needs. Not only will we delve into the syntax and mechanics behind each method, but we will also discuss common pitfalls and best practices to enhance your coding efficiency.
As we navigate through the intricacies of array manipulation, you’ll gain insight into how these techniques fit into broader programming concepts. By the end of this article, you’ll be equipped with the knowledge to clear arrays
Methods to Clear an Array in JavaScript
To clear an array in JavaScript, developers have multiple methods at their disposal, each with its own use cases and implications. Below are several effective techniques for achieving this.
Using the Length Property
One of the simplest ways to clear an array is by setting its `length` property to `0`. This method is straightforward and efficient, as it modifies the original array in place.
javascript
let myArray = [1, 2, 3, 4, 5];
myArray.length = 0; // The array is now empty
Using the Splice Method
The `splice` method can also be utilized to remove elements from an array. When called with parameters `0` and the array’s current length, it effectively clears the array.
javascript
let myArray = [1, 2, 3, 4, 5];
myArray.splice(0, myArray.length); // The array is now empty
Reassigning the Array
Another approach is to reassign the variable that holds the array to a new empty array. This method, however, does not modify the original array if other references exist.
javascript
let myArray = [1, 2, 3, 4, 5];
myArray = []; // The variable now points to a new empty array
Using the Filter Method
You can also use the `filter` method to return an empty array, effectively clearing its contents. While not the most conventional method, it is still valid.
javascript
let myArray = [1, 2, 3, 4, 5];
myArray = myArray.filter(() => ); // The array is now empty
Comparison of Methods
Below is a comparison of the different methods for clearing an array, highlighting their characteristics:
Method | Modifies Original Array | Performance | Example |
---|---|---|---|
Length Property | Yes | Fast | myArray.length = 0; |
Splice Method | Yes | Moderate | myArray.splice(0, myArray.length); |
Reassignment | No | Fast | myArray = []; |
Filter Method | No | Slow | myArray = myArray.filter(() => ); |
Selecting the appropriate method for clearing an array in JavaScript depends on the specific requirements of your application, such as performance considerations and whether you need to maintain references to the original array. Each method presented here serves a distinct purpose, allowing developers to choose the most suitable approach for their needs.
Methods to Clear an Array in JavaScript
There are several effective ways to clear an array in JavaScript. Each method has its own use case and performance implications, depending on the specific requirements of the application.
Using Length Property
One of the simplest ways to clear an array is by setting its `length` property to zero. This method is straightforward and efficient.
javascript
let arr = [1, 2, 3, 4];
arr.length = 0;
console.log(arr); // Output: []
Using Splice Method
The `splice()` method can also be used to remove all elements from an array. This method modifies the original array and can be particularly useful if the array is referenced elsewhere in the code.
javascript
let arr = [1, 2, 3, 4];
arr.splice(0, arr.length);
console.log(arr); // Output: []
Using Pop Method in a Loop
Another method involves using a loop to repeatedly call `pop()` until the array is empty. This is less efficient than the previous methods but can be useful in certain scenarios where you need to perform actions on each removed element.
javascript
let arr = [1, 2, 3, 4];
while (arr.length > 0) {
arr.pop();
}
console.log(arr); // Output: []
Reassigning to a New Array
You can also clear an array by reassigning it to a new empty array. This method creates a new reference, which can be beneficial if you want to ensure that no other references to the original array exist.
javascript
let arr = [1, 2, 3, 4];
arr = [];
console.log(arr); // Output: []
Performance Considerations
When deciding which method to use for clearing an array, consider the following points:
Method | Performance | Reference Change | Use Case |
---|---|---|---|
Length Property | Fast | No | Best for clearing without affecting references |
Splice Method | Moderate | No | Useful when needing to maintain references |
Pop in a Loop | Slow | No | When actions need to be taken on each element |
Reassigning to New Array | Fast | Yes | Best for creating a completely new context |
Choosing the right method depends on your specific needs, including performance requirements and whether maintaining references is crucial.
Expert Insights on Clearing Arrays in JavaScript
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To clear an array in JavaScript, the most efficient method is to set its length property to zero. This approach is not only straightforward but also ensures that the original array reference remains intact, which is crucial in many coding scenarios.”
Michael Chen (JavaScript Developer Advocate, Tech Innovations Inc.). “Using the splice method is another effective way to clear an array. By calling array.splice(0, array.length), you can remove all elements from the array while maintaining the original reference. This method is particularly useful when you need to manipulate the array in place.”
Sarah Johnson (Lead Frontend Developer, Web Solutions Group). “While there are multiple ways to clear an array, using the array.length = 0 method is the most commonly recommended. It is concise and performs well in terms of speed. However, developers should be aware of the implications for any references to the original array.”
Frequently Asked Questions (FAQs)
How can I clear an array in JavaScript?
You can clear an array in JavaScript by setting its length property to zero, like this: `array.length = 0;`. This effectively removes all elements from the array.
Is there a method to remove all elements from an array without affecting its reference?
Yes, you can use the `splice` method to remove all elements while maintaining the reference. For example: `array.splice(0, array.length);` removes all elements from the array.
What is the difference between setting the length to zero and using the `splice` method?
Setting the length to zero (`array.length = 0;`) is a more efficient operation, while `splice` can be used to remove elements selectively, allowing for more control over the array’s contents.
Can I use the `pop` method in a loop to clear an array?
Yes, you can use a `while` loop with the `pop` method to remove elements one by one until the array is empty. However, this method is less efficient than setting the length or using `splice`.
What happens to references of the original array when I clear it?
When you clear an array by modifying its length or using `splice`, all references to the original array will see the change, as they point to the same array object in memory.
Are there any performance considerations when clearing large arrays?
Yes, for large arrays, setting the length to zero is generally more performant than using methods like `splice` or `pop`, as it minimizes the number of operations and memory reallocations.
In JavaScript, clearing an array can be accomplished through various methods, each with its own implications and use cases. The most straightforward approach is to set the array’s length property to zero, effectively removing all elements. This method is efficient and maintains the reference to the original array, which is particularly useful when the array is referenced elsewhere in the code.
Another common method is to assign a new empty array to the variable holding the original array. While this also clears the contents, it creates a new reference, which may lead to issues if other parts of the code are relying on the original array reference. Additionally, using the `splice` method allows for more granular control, as it can remove elements from specific indices, but it may be less efficient for clearing an entire array.
Ultimately, the choice of method depends on the specific requirements of the application and the importance of maintaining references. Understanding the nuances of each approach helps developers make informed decisions, ensuring that they choose the most appropriate method for their needs. By considering factors such as performance, reference integrity, and code clarity, developers can effectively manage arrays in JavaScript.
Author Profile
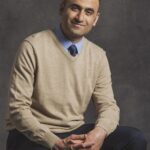
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?