How Can You Clear the Python Shell for a Fresh Start?
For many Python enthusiasts and developers, the interactive Python shell is a powerful tool that allows for quick experimentation and immediate feedback. However, as you dive deeper into coding, the shell can quickly become cluttered with old code snippets, error messages, and output that can obscure your view of the current task at hand. If you’ve ever found yourself overwhelmed by a jumbled mess of previous commands, you’re not alone. Knowing how to clear the Python shell can significantly enhance your coding experience, making it easier to focus on what truly matters—your code.
In this article, we’ll explore the various methods to clear the Python shell, whether you’re using the built-in interpreter, an integrated development environment (IDE), or a Jupyter notebook. Each environment has its own quirks and commands, and understanding these can streamline your workflow. Clearing the shell is not just about tidiness; it can also help prevent confusion and errors that arise from residual outputs or commands that linger in your session.
As we delve into the topic, you’ll discover practical tips and tricks that will not only help you clear the clutter but also improve your overall efficiency in coding with Python. Whether you’re a beginner just starting your programming journey or an experienced developer looking to refine your skills, mastering the art of shell management is a
Methods to Clear the Python Shell
Clearing the Python shell can enhance the readability of your workspace and help you focus on new output without the distraction of previous commands. Below are several methods to achieve this.
Using the Built-in Commands
In the Python interactive shell, you can use built-in commands to clear the screen. While there isn’t a specific command in Python to clear the shell, you can utilize system calls based on your operating system.
- For Windows, use the `cls` command:
“`python
import os
os.system(‘cls’)
“`
- For Unix/Linux and MacOS, use the `clear` command:
“`python
import os
os.system(‘clear’)
“`
This approach ensures that your command window is cleared and the cursor returns to the top, providing a clean slate for your next commands.
Keyboard Shortcuts
Most terminal interfaces offer keyboard shortcuts that can quickly clear the screen without needing to execute a command. Here are common shortcuts for various platforms:
Operating System | Shortcut |
---|---|
Windows | Ctrl + L |
Unix/Linux | Ctrl + L |
MacOS | Command + K |
These shortcuts may vary depending on your terminal or shell setup. Always check the specific documentation for your terminal if these don’t work.
Using IDE Features
If you are using an Integrated Development Environment (IDE) such as PyCharm, Jupyter Notebook, or VSCode, they typically offer a built-in option to clear the console.
– **PyCharm**: Right-click in the console and select “Clear All.”
– **Jupyter Notebook**: Use the menu option: `Cell` -> `All Output` -> `Clear`.
- VSCode: Use the command palette (Ctrl + Shift + P) and type “Clear” to find the clear terminal command.
This feature is particularly useful in a development environment where you might be running extensive scripts and need to manage output effectively.
Incorporating these methods into your Python workflow can significantly streamline your coding experience. Regularly clearing your Python shell not only keeps your workspace organized but also improves your focus on the current tasks at hand.
Methods to Clear the Python Shell
Clearing the Python shell can enhance your coding experience by providing a clean workspace. There are various methods available depending on your environment.
Using Keyboard Shortcuts
In many interactive Python shells, you can use keyboard shortcuts to clear the screen. The specific shortcuts may vary based on your operating system and shell type:
- IDLE:
- Press `Ctrl + L` to clear the shell window.
- Windows Command Prompt:
- Use `cls` command and press `Enter`.
- Linux/Mac Terminal:
- Type `clear` and press `Enter`.
Using Python Commands
You can also clear the shell programmatically using Python commands. Here are two common methods:
- Using `os` Module:
“`python
import os
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
This command checks the operating system and executes the corresponding command to clear the screen.
- Using `subprocess` Module:
“`python
import subprocess
subprocess.call(‘cls’ if os.name == ‘nt’ else ‘clear’, shell=True)
“`
This method is similar to the `os` module but is often preferred for its flexibility.
Clearing the Shell in Jupyter Notebooks
In Jupyter Notebooks, clearing the output is slightly different:
– **Clear All Output**:
- Navigate to the menu bar and select `Cell` -> `All Output` -> `Clear`.
- Clear Specific Cell Output:
- Click on the output area of the cell, and a small “X” will appear on the right. Click it to remove the output.
- Keyboard Shortcut:
- You can also use `Esc` to enter command mode, then press `00` (double zero) to restart the kernel, which clears all outputs.
Custom Functions for Clearing Shell
If you frequently need to clear the shell, you can create a custom function for convenience:
“`python
def clear_shell():
os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)
“`
You can call `clear_shell()` anytime you want to clear the screen, making your workflow more efficient.
Using Integrated Development Environments (IDEs)
Different IDEs have their own methods for clearing the console:
- PyCharm:
- Click on the “Clear All” button in the console toolbar or use `Ctrl + K`.
- Visual Studio Code:
- Use `Ctrl + Shift + P` to open the command palette, then type `Clear` and select `Clear Console`.
- Spyder:
- Click on the “Clear Console” button or use the shortcut `Ctrl + L`.
Each of these methods provides a quick way to maintain a tidy coding environment, allowing for better focus and organization as you work in Python.
Expert Insights on Clearing the Python Shell
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). “To clear the Python shell effectively, one can utilize the built-in command `import os` followed by `os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)`. This method is efficient for both Windows and Unix-based systems, ensuring a clean workspace.”
Michael Chen (Lead Python Developer, CodeCrafters). “For those using the interactive Python shell, simply typing `exit()` or `quit()` will clear the current session. However, it’s important to note that this will close the shell entirely, which may not be desirable for ongoing projects.”
Sarah Thompson (Technical Writer, Python Programming Journal). “In environments like Jupyter Notebook, clearing the output can be done through the menu: ‘Cell’ > ‘All Output’ > ‘Clear’. This approach helps maintain an organized workspace without losing your code.”
Frequently Asked Questions (FAQs)
How do I clear the Python shell in IDLE?
To clear the Python shell in IDLE, you can use the shortcut `Ctrl + L` or navigate to the menu and select `Shell` > `Restart Shell`. This will clear the output and reset the shell environment.
Is there a command to clear the Python shell in the command line interface?
In the command line interface, you can clear the Python shell by using the command `cls` on Windows or `clear` on Unix/Linux systems. This will clear the terminal screen but not the Python environment.
Can I clear the Python shell using a script?
Yes, you can clear the Python shell using a script by calling the `os` module. Use `import os` followed by `os.system(‘cls’ if os.name == ‘nt’ else ‘clear’)` to clear the screen programmatically.
Does clearing the Python shell affect the variables and functions defined?
Clearing the Python shell does not affect the variables and functions defined. It only removes the visible output from the shell, while the current session remains intact.
What happens if I use the `clear()` function in the Python shell?
The `clear()` function is not a built-in function in Python. If you attempt to use it, you will receive a `NameError`. Instead, use the appropriate commands or methods to clear the screen as mentioned above.
Are there any third-party libraries that can help clear the Python shell?
Yes, libraries like `curses` can be used to manipulate the terminal screen, including clearing it. However, this is more complex and typically used for creating text-based user interfaces rather than simply clearing the shell.
Clearing the Python shell can enhance the user experience by providing a clean workspace, especially during interactive sessions. There are several methods to achieve this, depending on the environment in which Python is being executed. For instance, using the `os` module’s `system` function can effectively clear the shell in a terminal or command prompt. Additionally, in environments like Jupyter Notebooks, clearing the output can be accomplished using specific commands or shortcuts.
It is important to note that the method of clearing the shell may vary based on the operating system. For example, on Windows, the command `cls` is used, while on Unix-based systems, the command is `clear`. Understanding these differences is crucial for executing the appropriate command in the respective environment. Furthermore, integrated development environments (IDEs) like PyCharm or Visual Studio Code may have built-in options to clear the console, which can streamline the process.
In summary, clearing the Python shell is a straightforward process that can be accomplished through various methods tailored to the user’s environment. Familiarity with these methods not only improves efficiency but also enhances the overall coding experience. By utilizing the right commands and understanding the tools available, users can maintain a more organized and productive workspace while working with Python
Author Profile
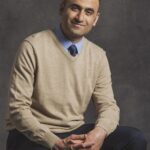
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?